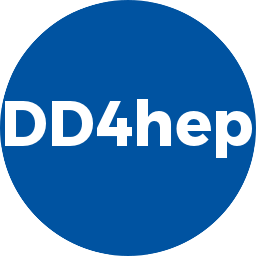 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_DDCOND_CONDITIONSREPOSITORYWRITER_H
14 #define DD4HEP_DDCOND_CONDITIONSREPOSITORYWRITER_H
118 using dd4hep::printout;
125 static dd4hep::PrintLevel s_printLevel = dd4hep::INFO;
132 class PropertyDumper {
136 void operator()(
const std::pair<std::string, dd4hep::Property>& p)
const {
138 std::string val = p.second.str();
139 if ( val[0] ==
'\'' ) val = p.second.str().c_str()+1;
140 if ( !val.empty() && val[val.length()-1] ==
'\'' ) val[val.length()-1] = 0;
151 std::string nam = c.
name();
152 std::string cn = nam.substr(nam.find(
'#')+1);
160 const dd4hep::Translation3D::Vector&
v = tr.Vect();
176 rot.GetComponents(z,y,x);
186 v.setAttr(
_U(value),data.
str());
191 press.
setAttr(
_U(value),c.
get<
double>()/(100e0*units::pascal));
234 size_t len = ::strlen(match);
235 size_t idx = typ.find(match);
236 size_t idq = typ.find(
',',idx+len);
237 if ( idx != std::string::npos && idq != std::string::npos ) {
238 std::string subtyp = tag;
239 subtyp +=
"["+typ.substr(idx+len,idq-idx-len)+
"]";
240 v.setAttr(
_U(type),subtyp);
244 dd4hep::except(
"Writer",
"++ Unknwon XML conversion to type: %s",typ.c_str());
249 return _seq(
v,c,
"vector",
"::vector<");
253 return _seq(
v,c,
"list",
"::list<");
257 return _seq(
v,c,
"set",
"::set<");
263 printout(INFO,
"ConditionsXMLWriter",
"++ Summary: Converted %ld conditions. %ld conditions without recipe.",
288 const IOV& validity = slice.
pool->validity();
293 std::snprintf(text,
sizeof(text),
"%ld,%ld#%s",
315 for (
const auto t : iovs ) {
333 std::vector<Condition> conds;
335 if ( !conds.empty() ) {
336 std::stringstream comment;
340 printout(s_printLevel,
"Writer",
"++ Conditions of DE %s [%d entries]",
341 detector.
path().c_str(),
int(conds.size()));
342 comment <<
" DDCond conditions for DetElement " << detector.
path()
343 <<
" Total of " << int(conds.size()) <<
" Entries. ";
346 for(
const auto& c : conds ) {
347 std::string nam = c.
name();
348 std::string cn = nam.substr(nam.find(
'#')+1);
349 detail::ReferenceBitMask<Condition::mask_type> msk(c->
flags);
351 printout(s_printLevel,
"Writer",
"++ Condition %s [%16llX] -> %s",
352 cn.c_str(), c.
key(), c.
name());
354 conditions.
append(_convert<temperature>(conditions,c));
358 conditions.
append(_convert<pressure>(conditions,c));
369 const std::type_info& typ = data.
typeInfo();
370 #if defined(DD4HEP_HAVE_ALL_PARSERS)
371 if ( typ ==
typeid(
char) || typ ==
typeid(
unsigned char) ||
372 typ ==
typeid(
short) || typ ==
typeid(
unsigned short) ||
373 typ ==
typeid(
int) || typ ==
typeid(
unsigned int) ||
374 typ ==
typeid(
long) || typ ==
typeid(
unsigned long) ||
375 typ ==
typeid(
float) || typ ==
typeid(
double) ||
376 typ ==
typeid(
bool) || typ ==
typeid(std::string) )
378 if ( typ ==
typeid(
int) || typ ==
typeid(
long) ||
379 typ ==
typeid(
float) || typ ==
typeid(
double) ||
380 typ ==
typeid(
bool) || typ ==
typeid(std::string) )
383 conditions.
append(_convert<value>(conditions,c));
386 else if ( ::strstr(data.
dataType().c_str(),
"::vector<") ) {
387 conditions.
append(_convert<std::vector<void*> >(conditions,c));
390 else if ( ::strstr(data.
dataType().c_str(),
"::list<") ) {
391 conditions.
append(_convert<std::list<void*> >(conditions,c));
394 else if ( ::strstr(data.
dataType().c_str(),
"::set<") ) {
395 conditions.
append(_convert<std::set<void*> >(conditions,c));
400 comment <<
"\n ** Unconverted condition: "
401 <<
"Unknown data type of condition: " << cn
402 <<
" [" << (
void*)c.
key() <<
"] -> "
403 << c.
name() <<
" Flags:" << (
unsigned int)c->
flags <<
"\n";
405 printout(ERROR,
"Writer",comment.str());
414 conditions.
append(_convert<Alignment>(conditions,cond_align));
417 else if ( cond_delta.
isValid() ) {
418 conditions.
append(_convert<dd4hep::Delta>(conditions,cond_delta));
422 for (
const auto& i : detector.
children())
423 count +=
collect(root,slice,i.second);
432 long ret = docH.
output(doc, output);
433 if ( !output.empty() ) {
434 printout(INFO,
"Writer",
"++ Successfully wrote %ld conditions (%ld unconverted) to file: %s",
446 static long write_repository_conditions(
dd4hep::Detector& description,
int argc,
char** argv) {
453 for(
int i=0; i<argc; ++i) {
454 if ( ::strncmp(argv[i],
"-iov_type",7) == 0 )
455 iovtype = manager.
iovType(argv[++i]);
456 else if ( ::strncmp(argv[i],
"-iov_value",7) == 0 )
457 iovvalue = ::atol(argv[++i]);
458 else if ( ::strncmp(argv[i],
"-output",4) == 0 && argc>i+1)
460 else if ( ::strncmp(argv[i],
"-manager",4) == 0 )
462 else if ( ::strncmp(argv[i],
"-help",2) == 0 ) {
463 printout(dd4hep::ALWAYS,
"Plugin-Help",
"Usage: dd4hep_XMLConditionsRepositoryWriter --opt [--opt] ");
464 printout(dd4hep::ALWAYS,
"Plugin-Help",
" -output <string> Output file name. Default: stdout ");
465 printout(dd4hep::ALWAYS,
"Plugin-Help",
" -manager <string> Add manager properties to the output. ");
466 printout(dd4hep::ALWAYS,
"Plugin-Help",
" -iov_type <string> IOV type to be selected. ");
467 printout(dd4hep::ALWAYS,
"Plugin-Help",
" -iov_value <string> IOV value to create the conditions snapshot.");
472 dd4hep::except(
"ConditionsPrepare",
"++ Unknown IOV type supplied.");
474 dd4hep::except(
"ConditionsPrepare",
475 "++ Unknown IOV value supplied for iov type %s.",iovtype->
str().c_str());
479 std::shared_ptr<ConditionsSlice> slice(
new ConditionsSlice(manager,content));
482 printout(dd4hep::INFO,
"Conditions",
483 "++ Selected conditions: %7ld conditions (S:%ld,L:%ld,C:%ld,M:%ld) for IOV:%-12s",
485 iovtype ? iov.str().c_str() :
"???");
490 doc = writer.
dump(manager);
491 writer.
collect(doc.root(), *slice);
494 doc = writer.
dump(*slice);
496 writer.
write(doc, output);
499 DECLARE_APPLY(DD4hep_ConditionsXMLRepositoryWriter,write_repository_conditions)
509 static long write_repository_manager(
dd4hep::Detector& description,
int argc,
char** argv) {
513 for(
int i=0; i<argc; ++i) {
514 if ( ::strncmp(argv[i],
"-output",4) == 0 && argc>i+1)
516 else if ( ::strncmp(argv[i],
"-help",2) == 0 ) {
517 printout(dd4hep::ALWAYS,
"Plugin-Help",
"Usage: dd4hep_XMLConditionsManagerWriter --opt [--opt] ");
518 printout(dd4hep::ALWAYS,
"Plugin-Help",
" -output <string> Output file name. Default: stdout ");
524 writer.
write(doc, output);
527 DECLARE_APPLY(DD4hep_ConditionsXMLManagerWriter,write_repository_manager)
const IOVType * iovType(const std::string &iov_name) const
Access IOV by its name.
const Children & children() const
Access to the list of children.
const std::string & path() const
Path of the detector element (not necessarily identical to placement path!)
Document create(const char *tag, const char *comment=0) const
Create new XML document by parsing empty xml buffer.
Document document() const
Access the hosting document handle of this DOM element.
virtual DetElement world() const =0
Return reference to the top-most (world) detector element.
std::string name
String name.
AlignmentCondition::Object * cond
void for_each(FUNCTOR &func)
Apply functor on properties.
long m_numUnconverted
Debug counters: unconverted conditions.
mask_type flags() const
Flag operations: Get condition flags.
void append(Handle_t handle) const
Append a new element to the existing tree.
ConditionsCollector< typename std::remove_reference< T >::type > conditionsCollector(ConditionsMap &m, T &&conditions)
Creator utility function for ConditionsCollector objects.
Main handle class to hold an alignment conditions object.
Detector & detectorDescription() const
Access the detector description.
#define DECLARE_APPLY(name, func)
ConditionsManager manager
Reference to the conditions manager.
std::string str() const
Conversion to string.
bool isValid() const
Check the validity of the object held by the handle.
std::string _toString(bool value)
String conversions: boolean value to string.
key_type key() const
Hash identifier.
Class to easily access the properties of single XmlElements.
const char * name() const
Access the object name (or "" if not supported by the object)
Helper union to interprete conditions keys.
Class describing the interval of validty type.
const std::vector< const IOVType * > iovTypesUsed() const
Access the used/registered IOV types.
Class describing an condition to re-adjust an alignment.
ROOT::Math::Translation3D Translation3D
Main condition object handle.
static ConditionsManager from(T &host)
Static accessor if installed as an extension.
Namespace for implementation details of the AIDA detector description toolkit.
Conditions content object. Defines which conditions should be loaded by the ConditionsManager.
Class describing the interval of validty.
Result prepare(const IOV &required_validity, ConditionsSlice &slice, ConditionUpdateUserContext *ctxt=0) const
Prepare all updates to the clients with the defined IOV.
Handle class describing a detector element.
AlignmentData & data()
Data accessor for the use of decorators.
static const Condition::itemkey_type deltaKey
Key value of a delta condition "alignment_delta".
static const std::string deltaName
Key name of a delta condition "alignment_delta".
Delta delta
Alignment changes.
PropertyManager & properties() const
Access to the property manager.
Key keyData
IOV key (if second==first, discrete, otherwise range)
Namespace for the AIDA detector description toolkit supporting XML utilities.
Conditions slice object. Defines which conditions should be loaded by the ConditionsManager.
Class describing an opaque data block.
virtual ~ConditionsXMLRepositoryWriter()
Default destructor.
T & get()
Generic getter. Specify the exact type, not a polymorph type.
static std::string defaultComment()
Default comment string.
ConditionsXMLRepositoryWriter()=default
Default constructor.
void setText(const XmlChar *text) const
Set the element's text.
unsigned long long int key_type
Forward definition of the key type.
long write(xml::Document doc, const std::string &output) const
Write the XML document structure to a file.
const std::type_info & typeInfo() const
Access type id of the condition.
Class supporting the basic functionality of an XML document.
Manager class for condition handles.
ROOT::Math::XYZVector Position
std::unique_ptr< UserPool > pool
Reference to the user pool managing all conditions of this slice.
virtual int output(Document doc, const std::string &fname) const
Write xml document to output file (stdout if file name empty)
Result of a prepare call to the conditions manager.
long m_numConverted
Debug counters: converted conditions.
Class supporting to read and parse XML documents.
Manager to ease the handling of groups of properties.
size_t size() const
Access total number of properties.
User abstraction class to manipulate XML elements within a document.
const IOVType * iovType
Reference to IOV type.
Namespace for the AIDA detector description toolkit.
Attribute setAttr(const XmlChar *nam, const T &val) const
Set single attribute.
void fill_content(ConditionsManager mgr, ConditionsContent &content, const IOVType &typ)
Populate the conditions slice from the conditions manager (convenience)
xml::Document dump(ConditionsSlice &slice)
Dump the tree content into a XML document structure.
unsigned int hash(unsigned int initialSeed, unsigned int eventNumber, unsigned int runNumber)
calculate hash from initialSeed, eventID and runID
The main interface to the dd4hep detector description package.
const std::string & dataType() const
Access type name of the condition data block.
std::string str() const
Create string representation of the data block.
ROOT::Math::RotationZYX RotationZYX
size_t collect(xml::Element root, ConditionsSlice &slice, DetElement detector)
Dump the tree content into an existing XML document structure.
ConditionsXMLRepositoryWriter(const ConditionsXMLRepositoryWriter ©)=default
Copy constructor (Special, partial copy only. Hence no assignment!)
OpaqueDataBlock & data() const
Access the opaque data block.
Conditions slice object. Defines which conditions should be loaded by the ConditionsManager.
void addComment(const XmlChar *text) const
Add comment node to the element.
Handle_t root() const
Access the ROOT eleemnt of the DOM document.