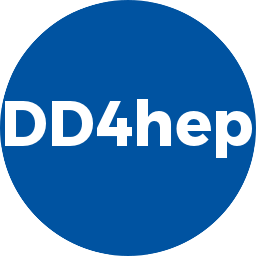 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef XML_XMLELEMENTS_H
14 #define XML_XMLELEMENTS_H
26 #define RAD_2_DEGREE 57.295779513082320876798154814105
29 #define M_PI 3.14159265358979323846
60 static size_t length(
const char* s);
89 if ( &c !=
this ) msg = c.msg;
95 typedef const std::string&
CSTR;
112 std::string
_toString(
const std::string& s);
114 std::string
_toString(
unsigned long i,
const char* fmt =
"%lu");
116 std::string
_toString(
unsigned int i,
const char* fmt =
"%u");
118 std::string
_toString(
int i,
const char* fmt =
"%d");
120 std::string
_toString(
long i,
const char* fmt =
"%ld");
122 std::string
_toString(
float d,
const char* fmt =
"%.17e");
124 std::string
_toString(
double d,
const char* fmt =
"%.17e");
126 std::string
_ptrToString(
const void* p,
const char* fmt =
"%p");
128 template <
typename T> std::string
_toString(
const T* p,
const char* fmt =
"%p")
157 std::string
getEnviron(
const std::string& env);
220 Strng_t
operator+(
const Strng_t& a,
const char* b);
222 Strng_t
operator+(
const Strng_t& a,
const std::string& b);
224 Strng_t
operator+(
const Strng_t& a,
const Strng_t& b);
226 Strng_t
operator+(
const char* a,
const Strng_t& b);
228 Strng_t
operator+(
const std::string& a,
const Strng_t& b);
278 Tag_t(
const std::string&
v,
const std::string& s,
void (*register_func)(
const std::string&,
Tag_t*))
280 register_func(
v,
this);
300 operator const std::string&()
const {
304 const std::string&
str()
const {
309 return m_str.c_str();
314 Tag_t
operator+(
const Tag_t& a,
const char* b);
316 Tag_t
operator+(
const char* a,
const Tag_t& b);
320 Tag_t
operator+(
const Tag_t& a,
const Strng_t& b);
322 Tag_t
operator+(
const Tag_t& a,
const std::string& b);
364 XmlElement*
next()
const;
455 return this->attr<T>(this->
attr_name(a));
464 template <
class T> T
attr(
const XmlChar* name, T default_value)
const;
481 bool hasAttr(
const char* t)
const {
486 template <
class T> T
attr(
const char* name)
const {
487 return this->attr<T>(
Strng_t(name));
490 template <
class T> T
attr(
const char* name,
const T& default_value)
const {
492 return this->
hasAttr(tag) ? this->attr<T>(
tag) : default_value;
522 unsigned int checksum(
unsigned int param,
unsigned int (fcn)(
unsigned int param,
const XmlChar*,
size_t)=0)
const;
525 #define INLINE inline
536 template <>
INLINE bool Handle_t::attr<bool>(
const XmlChar* tag_value)
const {
540 template <>
INLINE int Handle_t::attr<int>(
const XmlChar* tag_value)
const {
544 template <>
INLINE unsigned int Handle_t::attr<unsigned int>(
const XmlChar* tag_value)
const {
548 template <>
INLINE long Handle_t::attr<long>(
const XmlChar* tag_value)
const {
552 template <>
INLINE unsigned long Handle_t::attr<unsigned long>(
const XmlChar* tag_value)
const {
556 template <>
INLINE float Handle_t::attr<float>(
const XmlChar* tag_value)
const {
560 template <>
INLINE double Handle_t::attr<double>(
const XmlChar* tag_value)
const {
564 template <>
INLINE std::string Handle_t::attr<std::string>(
const XmlChar* tag_value)
const {
570 return a ? a : std::move(default_value);
573 template <>
INLINE bool Handle_t::attr<bool>(
const XmlChar* tag_value,
bool default_value)
const {
578 template <>
INLINE int Handle_t::attr<int>(
const XmlChar* tag_value,
int default_value)
const {
583 template <>
INLINE unsigned int Handle_t::attr<unsigned int>(
const XmlChar* tag_value,
unsigned int default_value)
const {
588 template <>
INLINE long Handle_t::attr<long>(
const XmlChar* tag_value,
long default_value)
const {
593 template <>
INLINE unsigned long Handle_t::attr<unsigned long>(
const XmlChar* tag_value,
unsigned long default_value)
const {
598 template <>
INLINE float Handle_t::attr<float>(
const XmlChar* tag_value,
float default_value)
const {
603 template <>
INLINE double Handle_t::attr<double>(
const XmlChar* tag_value,
double default_value)
const {
608 template <>
INLINE std::string Handle_t::attr<std::string>(
const XmlChar* tag_value, std::string default_value)
const {
610 return a ?
_toString(attr_value(a)) : std::move(default_value);
614 template<>
INLINE bool Handle_t::attr<bool>(
const Attribute tag_value)
const
617 template<>
INLINE int Handle_t::attr<int>(
const Attribute tag_value)
const
620 template<>
INLINE float Handle_t::attr<float>(
const Attribute tag_value)
const
623 template<>
INLINE double Handle_t::attr<double>(
const Attribute tag_value)
const
626 template<>
INLINE std::string Handle_t::attr<std::string>(
const Attribute tag_value)
const
627 {
return _toString(attr_value(tag_value));}
726 std::string
uri()
const;
787 operator bool()
const {
835 void text(
const std::string value)
const {
851 template <
class T> T
attr(
const XmlAttr* att)
const {
859 template <
class T> T
attr(
const XmlChar* tag_value, T default_value)
const {
863 template <
class T> T
attr(
const char* name)
const {
865 return this->attr<T>(
Strng_t(name));
868 template <
class T> T
attr(
const char* name, T default_value)
const {
869 return this->attr<T>(
Strng_t(name), default_value);
904 template <
class T>
void setValue(
const T& val)
const {
938 void addComment(
const std::string& text_value)
const;
989 #endif // XML_XMLELEMENTS_H
void throw_loop_exception(const std::exception &e) const
Helper function to throw an exception.
void setValue(const XmlChar *text) const
Set the element's value.
Tag_t(const std::string &s)
Constructor from STL string.
static XmlChar * replicate(const XmlChar *c)
Replicate string: internally allocates new string, which must be free'ed with release.
bool hasChild(const XmlChar *tag_value) const
Check the existence of a child with a given tag name.
Document document() const
Access the hosting document handle of this DOM element.
Class to support the access to collections of XmlNodes (or XmlElements)
Handle_t setChild(const XmlChar *tag) const
Check if a child with the required tag exists - if not create it and add it to the current node.
std::string m_str
STL string buffer.
void setAttrs(Handle_t e) const
Remove own attributes and copy all attributes from handle 'e'.
const XmlChar * getRef(const XmlChar *tag) const
Access the value of the reference attribute of the node (attribute ref="ref-name")
Tag_t(const std::string &v, const std::string &s, void(*register_func)(const std::string &, Tag_t *))
XmlElement * next() const
Advance to next element.
DocumentHolder(DOC d)
Constructor.
std::string text() const
Access the tag name of this DOM element.
std::string tag() const
Text access to the element's tag.
std::string uri() const
Acces the document URI.
double _toDouble(const XmlChar *value)
Conversion function from raw unicode string to double.
T attr(const XmlChar *name, T default_value) const
Access typed attribute value by its unicode name. If not existing returns default value.
void setName(const XmlChar *new_name)
Change/set the object's name.
xercesc::DOMException XmlException
const XmlChar * cpXmlChar
Element(const Element &e)
Constructor from XmlElement handle.
void append(Handle_t handle) const
Append a new element to the existing tree.
DOC operator->() const
Accessot to DOM document behaviour using arrow operator.
NodeList & operator=(const NodeList &l)
Assignment operator.
Class describing a list of XML nodes.
Handle_t clone(XmlDocument *new_doc) const
Clone the DOMelement - with the option of a deep copy.
void exception(const std::string &src, const std::string &msg)
unsigned int _toUInt(const XmlChar *value)
Conversion function from raw unicode string to unsigned int.
const XmlChar * attr_value_nothrow(const XmlChar *attr) const
Access attribute value by the attribute's unicode name (no exception thrown if not present)
Handle_t m_element
The underlying object holding the XmlElement pointer.
const XmlAttr * Attribute
XmlElement * previous() const
Go back to previous element.
std::string tag() const
Access the tag name of this DOM element.
Handle_t setRef(const XmlChar *tag, const XmlChar *ref)
Add reference child as a new child node. The obj must have the "name" attribute!
void append(Handle_t e) const
Append a DOM element to the current node.
Tag_t & operator=(const char *s)
Assignment of a normal ASCII string.
Handle_t parent() const
Access the element's parent element.
long _toLong(const XmlChar *value)
Conversion function from raw unicode string to long.
Handle_t addChild(const XmlChar *tag) const
Add a new child to the DOM node.
size_t numChildren(const XmlChar *tag_value, bool exc=true) const
Access the number of children of this DOM element with a given tag name.
const XmlChar * attr_value(const Attribute a) const
Access attribute value by the attribute (throws exception if not present)
static size_t length(const char *s)
String length in native representation.
Handle_t createElt(const XmlChar *tag) const
Create DOM element.
Handle_t remove(Handle_t e) const
Remove a single child node identified by its handle from the tree of the element.
const char * c_str() const
Access data buffer of STL string.
Class to easily access the properties of single XmlElements.
DocumentHolder()=default
Default Constructor.
bool enableEnvironResolution(bool new_value)
Enable/disable environment resolution when parsing strings.
void setAttrs(Handle_t e) const
Set attributes as in argument handle.
Handle_t(Elt_t e=0)
Initializing constructor.
XmlElement * reset()
Reset the nodelist - e.g. restart iteration from beginning.
void _toDictionary(const XmlChar *name, const XmlChar *value)
Helper function to populate the evaluator dictionary.
~NodeList()
Default destructor.
Strng_t(const std::string &c)
Initializing constructor from STL string.
void setValue(const T &val) const
Set element value.
size_t numChildren(const XmlChar *tag, bool throw_exception) const
Access the number of children of this DOM element with a given tag name.
Tag_t(const Tag_t &c)
Copy constructor.
Handle_t::Elt_t Elt_t
Simplification type declarations.
Element & operator=(const Element &c)
Assignment operator.
const XmlChar * attr_name(const Attribute attr) const
Access attribute name (throws exception if not present)
NodeList(const NodeList &l)
Copy constructor.
const XmlChar * rawText() const
Unicode text access to the element's text.
Class to support both way translation between C++ and XML strings.
bool hasChild(const XmlChar *tag) const
Check the existence of a child with a given tag name.
int get_float_precision()
Access floating point precision on conversion to string.
const XmlChar * refName() const
Access the object's reference name in unicode (same as name)
Collection_t & reset()
Reset the collection object to restart the iteration.
void removeChildren(const XmlChar *tag) const
Remove children with a given tag name from the DOM node.
~Document()=default
Destructor.
XmlChar * m_xml
Pointer to unicode string.
const XmlChar * attr_value(const Attribute attr) const
Access attribute value by the attribute (throws exception if not present)
void for_each(T oper) const
Loop processor using function object.
T attr(const XmlChar *name) const
Access typed attribute value by its unicode name.
Handle_t child(const Strng_t &tag_value, bool except=true) const
Access child by tag name. Thow an exception if required in case the child is not present.
bool operator==(const std::string &c, const Tag_t &b)
Equality operator between tag object and STL string.
int set_float_precision(int precision)
Change floating point precision on conversion to string.
int _toInt(const XmlChar *value)
Conversion function from raw unicode string to int.
virtual ~DocumentHolder()
Standard destructor - releases the document.
Strng_t(const XmlChar *c)
Initializing constructor from unicode string.
Namespace for the AIDA detector description toolkit supporting XML utilities.
Attribute setRef(const XmlChar *tag, const XmlChar *refname) const
Set the reference attribute to the node (adds attribute ref="ref-name")
T attr(const char *name, const T &default_value) const
Access typed attribute value by its name.
Attribute m_name
Attribute holding thre name.
NodeList children(const XmlChar *tag) const
Access a group of children identified by the same tag name.
void removeAttrs() const
Remove all attributes of this element.
const XmlChar * name() const
Access the object's name in unicode.
DOC ptr() const
Accessot to DOM document behaviour.
static void release(char **p)
Release string.
Attribute attr_ptr(const XmlChar *attr) const
Access attribute pointer by the attribute's unicode name (throws exception if not present)
Elt_t ptr() const
Access to XmlElement pointer.
void for_each(const XmlChar *tag_name, T oper) const
Loop processor using function object.
Element(const Handle_t &e)
Constructor from XmlElement handle.
Attribute getAttr(const XmlChar *name) const
Access single attribute by its name.
T attr(const char *name, T default_value) const
Access typed attribute value by its name.
std::string _toString(const Attribute attr)
Convert xml attribute to STL string.
size_t length() const
String length in native representation.
static XmlChar * transcode(const char *c)
Transcode string.
Document & operator=(const Document &d)=default
Assignment.
Strng_t & operator=(const XmlChar *s)
Assignment opertor from unicode string.
Helper class to encapsulate a unicode string.
Class supporting the basic functionality of an XML document including ownership.
Handle_t child(const XmlChar *tag, bool throw_exception=true) const
Access a single child by its tag name (unicode)
Handle_t clone(const Document &new_doc) const
Clone the DOMelement.
void setText(const XmlChar *text) const
Set the element's text.
T attr(const XmlChar *tag_value, T default_value) const
Access attribute with implicit return type conversion.
bool hasAttr(const XmlChar *name) const
Check for the existence of a named attribute.
Handle_t clone(Handle_t source) const
Clone a DOM element / sub-tree.
DocumentHolder & assign(DOC d)
Assign new document. Old document is dropped.
Class supporting the basic functionality of an XML document.
Document()
Default Constructor.
RefElement(const Handle_t &e)
Construction from existing object handle.
Strng_t operator+(const Strng_t &a, const char *b)
Unicode string concatenation of a normal ASCII string from right side.
Elt_t parentElement() const
Access the XmlElements parent.
const XmlChar * attr_name(const Attribute a) const
Access attribute name (throws exception if not present)
RefElement & operator=(const RefElement &e)
Assignment operator.
size_t size() const
Access the collection size. Avoid this call – sloooow!
Tag_t(const char *s)
Constructor from normal ASCII string.
Elt_t m_node
The pointer to the XmlElement.
User abstraction class to manipulate named XML elements (references) within a document.
T attr(const char *name) const
Access typed attribute value by its name.
const XmlChar * ptr() const
Accessor to unicode string.
Document(DOC d)
Initializing Constructor.
unsigned long _toULong(const XmlChar *value)
Conversion function from raw unicode string to unsigned long.
DocumentHolder & operator=(const DocumentHolder ©)=delete
Assignment operator.
const XmlChar * tagName() const
Access the tag name of this DOM element.
~Strng_t()
Default destructor - release unicode string.
Class supporting to read and parse XML documents.
Collection_t(Handle_t node, const XmlChar *tag)
Constructor over XmlElements with a given tag name.
Tag_t(const Strng_t &s)
Constructor from internal XML string.
bool operator!() const
operator NOT: check handle validity
const XmlChar * rawTag() const
Unicode text access to the element's tag. Tis must be wrong ....
Handle_t remove(Handle_t node) const
Remove a child node identified by its handle.
float _toFloat(const XmlChar *value)
Conversion function from raw unicode string to float.
XERCES_XMLCH_T XmlChar
Use the definition from the autoconf header of Xerces:
User abstraction class to manipulate XML elements within a document.
void dump_tree(Handle_t elt)
Dump partial or full XML trees to stdout.
Namespace for the AIDA detector description toolkit.
const std::string & str() const
Access writable STL string.
std::vector< Attribute > attributes() const
Retrieve a collection of all attributes of this DOM element.
Attribute setAttr(const XmlChar *nam, const T &val) const
Set single attribute.
T attr(const XmlChar *tag_value) const
Access attribute with implicit return type conversion.
Definition of the XmlString class.
std::string _ptrToString(const void *p, const char *fmt="%p")
Format void pointer (64 bits) to string with arbitrary format.
std::string getEnviron(const std::string &env)
Helper function to lookup environment from the expression evaluator.
Document(const Document &d)=default
Copy constructor.
Element & operator=(Handle_t handle)
Assignment operator.
NodeList m_children
Reference to the list of child nodes.
Attribute setAttr(const XmlChar *t, const XmlChar *v) const
Generic attribute setter with unicode value.
void dumpTree(XmlDocument *doc)
Dump DOM tree of a document.
unsigned int checksum(unsigned int param, unsigned int(fcn)(unsigned int param, const XmlChar *, size_t)=0) const
Checksum (sub-)tree of a xml document/tree. Default will pick up the adler32 checksum.
bool hasAttr(const XmlChar *t) const
Check for the existence of a named attribute.
std::vector< Attribute > attributes() const
Retrieve a collection of all attributes of this DOM element.
void operator++() const
Operator to advance the collection (pre increment)
Handle_t parent() const
Access the XmlElements parent.
const XmlChar * rawValue() const
Unicode text access to the element's value.
DocumentHolder(const DocumentHolder ©)=delete
Default Constructor.
T attr(const Attribute a) const
Access typed attribute value by the XmlAttr.
std::string value() const
Text access to the element's value.
bool _toBool(const XmlChar *value)
Conversion function from raw unicode string to bool.
T attr(const XmlAttr *att) const
Access attribute with implicit return type conversion.
Elt_t ptr() const
Direct access to the XmlElement by function.
Strng_t(const Strng_t &c)
Copy constructor.
void text(const std::string value) const
Set text attribute to the XML node.
void handle(const O *o, const C &c, F pmf)
void operator--() const
Operator to advance the collection (pre decrement)
void removeAttrs() const
Remove all attributes of this element.
Elt_t current() const
Access to current element.
std::string text() const
Text access to the element's text.
Elt_t operator->() const
Direct access to the XmlElement using the operator->
void addComment(const XmlChar *text) const
Add comment node to the element.
Attribute attr_nothrow(const XmlChar *tag) const
Access attribute pointer by the attribute's unicode name (no exception thrown if not present)
Handle_t root() const
Access the ROOT eleemnt of the DOM document.