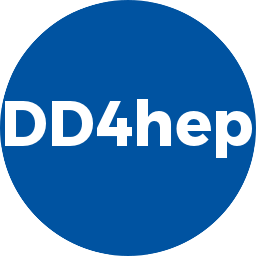 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDCOND_CONDITIONSCONTENT_H
14 #define DDCOND_CONDITIONSCONTENT_H
23 #include <unordered_map>
56 virtual const std::type_info&
type()
const = 0;
57 virtual const void*
ptr()
const = 0;
60 template<
typename T> T*
data()
const {
return (T*)
ptr(); }
90 virtual const std::type_info&
type()
const override
92 virtual const void*
ptr()
const override
100 typedef std::map<Condition::key_type,ConditionDependency* >
Dependencies;
101 typedef std::map<Condition::key_type,ConditionsLoadInfo* >
Conditions;
137 std::pair<Condition::key_type, ConditionsLoadInfo*>
140 std::pair<Condition::key_type, ConditionsLoadInfo*>
143 template <
typename T> std::pair<Condition::key_type, ConditionsLoadInfo*>
148 template <
typename T> std::pair<Condition::key_type, ConditionsLoadInfo*>
153 std::pair<Condition::key_type, ConditionDependency*>
156 std::pair<Condition::key_type, ConditionDependency*>
167 #endif // DDCOND_CONDITIONSCONTENT_H
LoadInfo & operator=(const LoadInfo ©)=delete
Dependencies m_derived
Container of derived conditions required by this content.
AlignmentCondition::Object * cond
virtual ~LoadInfo()=default
Conditions m_conditions
Container of conditions required by this content.
Conditions & conditions()
Access to the real condition entries to be loaded.
ConditionsLoadInfo * addRef()
std::map< Condition::key_type, ConditionDependency * > Dependencies
std::size_t info(const std::string &src, const std::string &msg)
ConditionsLoadInfo(ConditionsLoadInfo &©)=delete
Move constructor.
virtual std::string toString() const =0
const Conditions & conditions() const
Access to the real condition entries to be loaded (CONST)
virtual ~ConditionsContent()
Default destructor.
Key definition to optimize ans simplyfy the access to conditions entities.
ConditionsLoadInfo(const ConditionsLoadInfo ©)=delete
Copy constructor.
bool remove(Condition::key_type condition)
Remove a condition from the content.
virtual ~ConditionsLoadInfo()
Default destructor.
Conditions content object. Defines which conditions should be loaded by the ConditionsManager.
Condition dependency definition.
Dependencies & derived()
Access to the derived condition entries to be computed.
LoadInfo(const LoadInfo &c)=delete
Handle class describing a detector element.
std::pair< Condition::key_type, ConditionsLoadInfo * > addLocationInfo(Condition::key_type hash, ConditionsLoadInfo *info)
Add a new conditions key. T must inherit from class ConditionsContent::Info.
virtual const void * ptr() const =0
void merge(const ConditionsContent &to_add)
Merge the content of "to_add" into the this content.
virtual const void * ptr() const override
ConditionsContent()
Default constructor.
const Dependencies & derived() const
Access to the derived condition entries to be computed (CONST)
ConditionsContent & operator=(const ConditionsContent ©)=delete
Default assignment operator.
virtual std::string toString() const override
unsigned int itemkey_type
Low part of the key identifies the item identifier.
Base class for data loading information.
unsigned long long int key_type
Forward definition of the key type.
virtual const std::type_info & type() const override
ConditionsContent(const ConditionsContent ©)=delete
Copy constructor.
virtual ConditionsLoadInfo * clone() const override
virtual ConditionsLoadInfo * clone() const =0
Namespace for the AIDA detector description toolkit.
std::pair< Condition::key_type, ConditionDependency * > addDependency(ConditionDependency *dep)
Add a new shared conditions dependency.
std::map< Condition::key_type, ConditionsLoadInfo * > Conditions
unsigned int hash(unsigned int initialSeed, unsigned int eventNumber, unsigned int runNumber)
calculate hash from initialSeed, eventID and runID
Concrete class for data loading information.
ConditionsLoadInfo()
Default constructor.
void clear()
Clear the conditions content definitions.
std::pair< Condition::key_type, ConditionsLoadInfo * > addLocation(Condition::key_type hash, const T &info)
Add a new conditions key. T must inherit from class ConditionsContent::Info.
ConditionsLoadInfo & operator=(const ConditionsLoadInfo ©)=delete
Assignment operator.
std::pair< Condition::key_type, ConditionsLoadInfo * > addLocation(DetElement de, Condition::itemkey_type item, const T &info)
Add a new shared conditions dependency.
std::pair< Condition::key_type, ConditionsLoadInfo * > insertKey(Condition::key_type hash)
Add a new conditions key representing a real (not derived) condition.
virtual const std::type_info & type() const =0