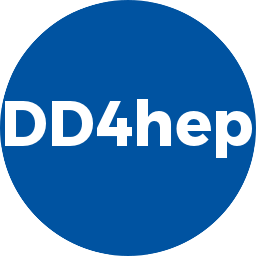 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
30 const std::shared_ptr<ConditionsContent>& cont)
31 : manager(mgr), content(cont)
38 : manager(
copy.manager), content(
copy.content)
57 if (
pool.get() )
return pool->validity();
58 except(
"ConditionsSlice",
59 "pool-iov: Failed to access validity of non-existing pool.");
60 return pool->validity();
75 except(
"ConditionsSlice",
76 "manage_condition: Cannot access conditions pool according to IOV:%s.",
77 pool->validity().str().c_str());
81 except(
"ConditionsSlice",
82 "manage_condition: Failed to register condition %016llx according to IOV:%s.",
83 condition->hash,
pool->validity().str().c_str());
87 ret =
pool->insert(condition);
89 except(
"ConditionsSlice",
90 "manage_condition: Failed to register condition %016llx to user pool with IOV:%s.",
91 condition->hash,
pool->validity().str().c_str());
96 except(
"ConditionsSlice",
97 "manage_condition: Cannot manage invalid condition!");
104 return manage(p, condition, flg);
116 return pool->get(detector, lower, upper);
124 except(
"ConditionsSlice",
125 "manage_condition: Cannot access conditions pool according to IOV:%s.",
126 pool->validity().str().c_str());
130 except(
"ConditionsSlice",
131 "manage_condition: Failed to register condition %016llx according to IOV:%s.",
132 condition->hash,
pool->validity().str().c_str());
134 return pool->insert(detector,
key, condition);
136 except(
"ConditionsSlice",
137 "insert_condition: Cannot insert invalid condition to the user pool!");
143 return pool->get(detector,
key);
148 pool->scan(processor);
156 pool->scan(detector, lower, upper, processor);
164 void operator()(
const ConditionsIOVPool::Elements::value_type&
v) {
165 v.second->select_all(*
this);
169 #if !defined(DD4HEP_MINIMAL_CONDITIONS)
177 virtual size_t size()
const {
return content.
conditions().size(); }
188 for_each(begin(pools),end(pools),SliceOper(content));
unsigned long flags
Flag to steer conditions management.
std::vector< Condition > get(DetElement detector) const
Access all conditions from a given detector element.
ConditionsPool * registerIOV(const IOV &iov) const
Register IOV with type and key.
ContainedPools used_pools
If requested refence the used pools with a shared pointer to inhibit cleanup.
void derefPools()
Set flag to not reference the used pools during prepare (and drop possibly pending)
Condition::key_type hash
Hash value of the name.
Conditions & conditions()
Access to the real condition entries to be loaded.
virtual bool manage(ConditionsPool *pool, Condition condition, ManageFlag flg)
Local optimization: Insert a set of conditions to the slice AND register them to the conditions manag...
std::map< IOV::Key, Element > Elements
Shortcut name for the actual conditions container.
Condition::mask_type flags
Flags.
ConditionsManager manager
Reference to the conditions manager.
bool isValid() const
Check the validity of the object held by the handle.
void reset()
Clear the conditions content and the user pool.
static void increment(T *)
Increment count according to type information.
std::string address
Condition address.
Elements elements
Container of IOV dependent conditions pools.
Conditions selector functor. Default implementation selects everything evaluated.
Class describing the interval of validty type.
ConditionsIOVPool * iovPool(const IOVType &type) const
Access conditions multi IOV pool by iov type.
Main condition object handle.
Namespace for implementation details of the AIDA detector description toolkit.
Conditions content object. Defines which conditions should be loaded by the ConditionsManager.
Class describing the interval of validty.
Handle class describing a detector element.
ConditionsSlice()=delete
Default constructor.
Class implementing the conditions collection for a given IOV type.
bool registerUnlocked(ConditionsPool &pool, Condition cond) const
Register new condition with the conditions store. Unlocked version, not multi-threaded.
static void decrement(T *)
Decrement count according to type information.
unsigned int itemkey_type
Low part of the key identifies the item identifier.
virtual void scan(const Condition::Processor &processor) const override
ConditionsMap overload: Interface to scan data content of the conditions mapping.
const IOV & iov() const
Access the combined IOV of the slice from the pool.
The data class behind a conditions handle.
Manager class for condition handles.
std::unique_ptr< UserPool > pool
Reference to the user pool managing all conditions of this slice.
void fill_content(ConditionsManager mgr, ConditionsContent &content, const IOVType &typ)
Populate the conditions slice from the conditions manager (convenience)
virtual ~ConditionsSlice()
Default destructor.
virtual bool insert(DetElement detector, Condition::itemkey_type key, Condition condition) override
ConditionsMap overload: Add a condition directly to the slice.
Pool of conditions satisfying one IOV type (epoch, run, fill, etc)
std::pair< Condition::key_type, ConditionsLoadInfo * > addLocation(Condition::key_type hash, const T &info)
Add a new conditions key. T must inherit from class ConditionsContent::Info.
Abstract base for processing callbacks to conditions objects.
Conditions slice object. Defines which conditions should be loaded by the ConditionsManager.