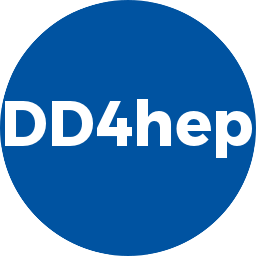 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_OPAQUEDATA_H
14 #define DD4HEP_OPAQUEDATA_H
63 std::string
str()
const;
65 const std::type_info&
typeInfo()
const;
73 template <
typename T> T&
get();
75 template <
typename T>
const T&
get()
const;
77 template <
typename T> T&
as();
79 template <
typename T>
const T&
as()
const;
141 template <
typename T,
typename... Args> T&
construct(Args... args);
143 template <
typename T> T&
bind();
145 template <
typename T> T&
bind(
void*
ptr,
size_t len);
147 template <
typename T> T&
bind(
const std::string& value);
149 template <
typename T> T&
bind(
void*
ptr,
size_t len,
const std::string& value);
151 template <
typename T> T&
bind(T&&
data);
172 if ( obj )
return *obj;
174 throw std::bad_cast();
180 const T* obj = (
const T*)(
grammar->
cast().apply_dynCast(Cast::instance<T>(), this->
pointer));
181 if ( obj )
return *obj;
183 throw std::bad_cast();
188 this->
bind(&BasicGrammar::instance<OBJECT>());
189 new(this->
pointer) OBJECT(std::move(obj));
194 this->
bind(&BasicGrammar::instance<T>());
195 return *(
new(this->
pointer) T(std::forward<Args>(args)...));
200 this->
bind(&BasicGrammar::instance<T>());
201 return *(
new(this->
pointer) T());
206 this->
bind(&BasicGrammar::instance<T>());
207 new(this->
pointer) T(std::move(obj));
212 this->
bind(ptr,len,&BasicGrammar::instance<T>());
213 return *(
new(this->
pointer) T());
218 T& ret = this->bind<T>();
219 if ( !value.empty() && !this->fromString(value) ) {
220 throw std::runtime_error(
"OpaqueDataBlock::set> Failed to bind type "+
221 typeName(
typeid(T))+
" to condition data block.");
228 T& ret = this->bind<T>(
ptr, len);
229 if ( !value.empty() && !this->fromString(value) ) {
230 throw std::runtime_error(
"OpaqueDataBlock::set> Failed to bind type "+
231 typeName(
typeid(T))+
" to condition data block.");
241 #endif // DD4HEP_OPAQUEDATA_H
virtual ~OpaqueData()=default
Standard Destructor.
virtual bool equals(const std::type_info &other_type) const =0
Access to the type information.
OpaqueData()=default
Standard initializing constructor.
constexpr static const size_t BUFFER_SIZE
Buffer size of the in-place data buffer.
T & construct(Args... args)
Construct conditions object and bind the data.
unsigned int type
Data buffer type: Must be a bitmap of enum _DataTypes!
OpaqueData(const OpaqueData ©)=default
Copy constructor.
unsigned char data[BUFFER_SIZE]
Data buffer: plain data are allocated directly on this buffer.
Class describing an opaque conditions data block.
bool fromString(const std::string &rep)
No ROOT persistency.
bool is_bound() const
Check if object is already bound....
OpaqueDataBlock()
Standard initializing constructor.
T & as()
Generic getter. Resolves polymorph types. It is mandatory that the datatype is polymorph!
Base class describing string evaluation to C++ objects using boost::spirit.
OpaqueData & operator=(const OpaqueData ©)=default
Assignment operator.
Class describing an opaque data block.
T & get()
Generic getter. Specify the exact type, not a polymorph type.
void * ptr() const
Write access to the data buffer. Is only valid after call to bind<T>()
T & bind()
Bind data value.
const void * ptr() const
Access to the data buffer (read only!). Is only valid after call to bind<T>()
const std::type_info & typeInfo() const
Access type id of the condition.
OpaqueDataBlock & operator=(const OpaqueDataBlock ©)
Assignment operator (Required by ROOT dictionaries)
Namespace for the AIDA detector description toolkit.
virtual const Cast & cast() const
Access ABI object cast.
void bindExtern(void *ptr, const BasicGrammar *grammar)
Bind external data value to the pointer.
const BasicGrammar * grammar
Data type.
const std::string & dataType() const
Access type name of the condition data block.
std::string str() const
Create string representation of the data block.
void * pointer
No ROOT persistency.
~OpaqueDataBlock()
Standard Destructor.