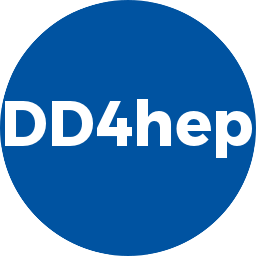 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
25 int s_have_inventory = 0;
27 std::map<Condition::itemkey_type,std::string> item_names;
30 if ( s_have_inventory > 0 ) {
31 std::lock_guard<std::mutex> protect(lock);
32 item_names.emplace(
key, item);
36 auto i = item_names.find(
key);
37 if( i != item_names.end() ) {
41 std::snprintf(text,
sizeof(text),
"%08X",
key);
50 s_have_inventory = value;
52 return s_have_inventory;
60 return s_key_tracer.get(
key);
66 if ( hash_key != 0 && hash_key != ~0x0ULL ) {
72 except(
"Condition",
"+++ Cannot create a condition with an invalid key: %016llX",hash_key);
87 void*
ptr = ::operator
new(
sizeof(
Object)+memory);
95 std::stringstream output;
97 #if defined(DD4HEP_CONDITIONS_HAVE_NAME)
99 output << std::setw(16) << std::left << o->name;
103 output <<
" " << (ptr_iov ? ptr_iov->
str().c_str() :
"IOV:[UNKNOWN]");
105 #if !defined(DD4HEP_MINIMAL_CONDITIONS)
107 output <<
" (" << o->type <<
")";
112 output <<
" Data:" << o->
data.
str();
113 #if !defined(DD4HEP_MINIMAL_CONDITIONS)
117 output <<
" \"" << o->
comment <<
"\"";
142 #if !defined(DD4HEP_MINIMAL_CONDITIONS)
208 invalidHandleError<Condition>();
211 throw std::runtime_error(
"Null pointer in Grammar object");
222 KeyMaker key_maker(detector.
key(), detail::hash32(value));
234 KeyMaker key_maker(det_key, detail::hash32(value));
244 #ifdef DD4HEP_CONDITIONS_HAVE_NAME
245 name = detector.
path()+
"#"+value;
254 #ifdef DD4HEP_CONDITIONS_HAVE_NAME
256 ::snprintf(text,
sizeof(text),
"%08X#",det_key);
264 #ifdef DD4HEP_CONDITIONS_HAVE_NAME
266 ::snprintf(text,
sizeof(text),
"#%08X",
item_key);
267 name = detector.
path()+text;
275 return key_maker.
hash;
282 return key_maker.
hash;
288 s_key_tracer.add(code, value);
295 s_key_tracer.add(code, value);
303 ::snprintf(text,
sizeof(text),
"%08X-%08X",
key.values.det_key,
key.values.item_key);
304 #if defined(DD4HEP_CONDITIONS_HAVE_NAME)
305 if ( !name.empty() ) {
306 std::stringstream str;
307 str <<
"(" << name <<
") " << text;
std::string value
Condition value (in string form)
int dataType() const
Access the data type.
const std::string & path() const
Path of the detector element (not necessarily identical to placement path!)
const std::string & value() const
Access the value field of the condition as a string.
static Condition::key_type hashCode(DetElement detector, const char *value)
Hash code generation from input string.
virtual ~ConditionsSelect()
Default destructor.
int have_condition_item_inventory(int value=-1)
Setup conditions item name inventory for debugging.
unsigned int key() const
Access hash key of this detector element (Only valid once geometry is closed!)
const std::type_info & typeInfo() const
Access to the type information.
mask_type flags() const
Flag operations: Get condition flags.
Condition::key_type hash
Hash value of the name.
detail::ConditionObject Object
Extern accessible definition of the contained element type.
bool testFlag(mask_type option) const
Flag operations: Test for a given a conditions flag.
const std::string & comment() const
Access the comment field of the condition.
unsigned int type
Data buffer type: Must be a bitmap of enum _DataTypes!
Condition::key_type hash
Hashed key representation.
ConditionKey()=default
Default constructor.
Condition::mask_type flags
Flags.
Class describing an opaque conditions data block.
Handle: a templated class like a shared pointer, which allows specialized access to tgeometry objects...
std::string str() const
Create string representation of the IOV.
key_type key() const
Hash identifier.
std::string address
Condition address.
std::string toString() const
Conversion to string.
Helper union to interprete conditions keys.
Class describing the interval of validty type.
detkey_type detector_key() const
DetElement part of the identifier.
void unFlag(Condition::mask_type option)
Flag operations: UN-Set a conditions flag.
unsigned int detkey_type
High part of the key identifies the detector element.
Class describing the interval of validty.
Handle class describing a detector element.
Base class describing string evaluation to C++ objects using boost::spirit.
void assign(Object *n, const std::string &nam, const std::string &title)
Assign a new named object. Note: object references must be managed by the user.
const IOVType * iovType() const
Access safely the IOV-type.
static Condition::itemkey_type itemCode(const char *value)
32 bit hashcode of the item
itemkey_type item_key() const
Item part of the identifier.
std::string str(int with_data=WITH_IOV|WITH_ADDRESS|WITH_DATATYPE) const
Output method.
std::string comment
Comment string.
unsigned int itemkey_type
Low part of the key identifies the item identifier.
void unFlag(mask_type option)
Flag operations: UN-Set a conditions flag.
void setFlag(Condition::mask_type option)
Flag operations: Set a conditions flag.
unsigned long long int key_type
Forward definition of the key type.
const IOV * iovData() const
Access safely the IOV.
unsigned int mask_type
Forward definition of the object properties.
const std::string & type() const
Access the type field of the condition.
The data class behind a conditions handle.
virtual const std::type_info & type() const =0
Access to the type information.
void setFlag(mask_type option)
Flag operations: Set a conditions flag.
detail::ConditionObject * access() const
Checked object access. Throws invalid handle runtime exception if invalid handle.
detail::ConditionObject * ptr() const
Access to the held object.
Condition::detkey_type det_key
std::string get_condition_item_name(Condition::itemkey_type key)
Resolve key from conditions item name inventory for debugging.
Namespace for the AIDA detector description toolkit.
Condition::itemkey_type item_key() const
Access the detector element part of the key.
unsigned int hash(unsigned int initialSeed, unsigned int eventNumber, unsigned int runNumber)
calculate hash from initialSeed, eventID and runID
Condition()=default
Default constructor.
OpaqueDataBlock data
Data block.
const BasicGrammar * grammar
Data type.
const IOVType & iovType() const
Access the IOV type.
const std::string & dataType() const
Access type name of the condition data block.
std::string str() const
Create string representation of the data block.
struct dd4hep::ConditionKey::KeyMaker::@2 values
const BasicGrammar & descriptor() const
Access to the grammar type.
bool testFlag(Condition::mask_type option) const
Flag operations: Test for a given a conditions flag.
const std::string & address() const
Access the address string [e.g. database identifier].
const IOV & iov() const
Access the IOV block.
OpaqueDataBlock & data() const
Access the opaque data block.
Condition::itemkey_type item_key