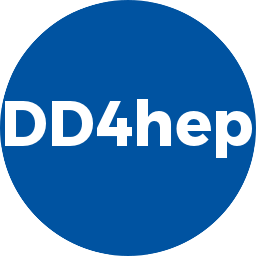 |
DD4hep
1.32.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
30 throw std::runtime_error(
"Opaque data block is unbound. Cannot parse string representation.");
38 throw std::runtime_error(
"Opaque data block is unbound. Cannot create string representation.");
46 throw std::runtime_error(
"Opaque data block is unbound. Cannot determine type information!");
54 throw std::runtime_error(
"Opaque data block is unbound. Cannot determine type information!");
72 except(
"OpaqueDataBlock",
"Grammar type %s does not support object copy. Operation not allowed.",
106 except(
"OpaqueDataBlock",
"Grammar type %s does not support object copy. Operation not allowed.",
118 except(
"OpaqueData",
"You may not bind opaque data multiple times!");
126 except(
"OpaqueData",
"Extern data may not be bound!");
139 except(
"OpaqueData",
"You may not bind opaque multiple times!");
141 typeinfoCheck(
grammar->
type(),g->
type(),
"Opaque data blocks may not be assigned.");
150 except(
"OpaqueData",
"You may not bind opaque data multiple times!");
160 except(
"OpaqueData",
"Extern data may not be bound!");
167 else if ( len <=
sizeof(
data) )
176 except(
"OpaqueData",
"You may not bind opaque data multiple times!");
178 typeinfoCheck(
grammar->
type(),g->
type(),
"Opaque data blocks may not be assigned.");
183 static auto s_registry = GrammarRegistry::pre_note<OpaqueDataBlock>(1);
virtual size_t sizeOf() const =0
Access the object size (sizeof operator)
unsigned int type
Data buffer type: Must be a bitmap of enum _DataTypes!
void(* copy)(void *to, const void *from)=0
Opaque copy constructor.
unsigned char data[BUFFER_SIZE]
Data buffer: plain data are allocated directly on this buffer.
virtual std::string str(const void *ptr) const
Serialize an opaque value to a string.
Class describing an opaque conditions data block.
static void increment(T *)
Increment count according to type information.
bool fromString(const std::string &rep)
No ROOT persistency.
virtual bool fromString(void *ptr, const std::string &value) const
Set value from serialized string. On successful data conversion TRUE is returned.
const std::string & type_name() const
Access to the type information name.
OpaqueDataBlock()
Standard initializing constructor.
Base class describing string evaluation to C++ objects using boost::spirit.
OpaqueData & operator=(const OpaqueData ©)=default
Assignment operator.
static void decrement(T *)
Decrement count according to type information.
Class describing an opaque data block.
void * ptr() const
Write access to the data buffer. Is only valid after call to bind<T>()
T & bind()
Bind data value.
struct dd4hep::BasicGrammar::specialization_t specialization
const std::type_info & typeInfo() const
Access type id of the condition.
OpaqueDataBlock & operator=(const OpaqueDataBlock ©)
Assignment operator (Required by ROOT dictionaries)
virtual const std::type_info & type() const =0
Access to the type information.
Namespace for the AIDA detector description toolkit.
void bindExtern(void *ptr, const BasicGrammar *grammar)
Bind external data value to the pointer.
const BasicGrammar * grammar
Data type.
const std::string & dataType() const
Access type name of the condition data block.
virtual void destruct(void *pointer) const =0
Opaque object destructor.
std::string str() const
Create string representation of the data block.
void * pointer
No ROOT persistency.
~OpaqueDataBlock()
Standard Destructor.