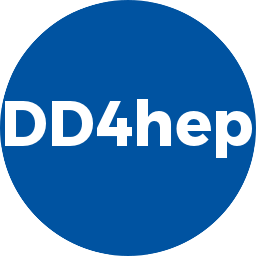 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
26 #if __cplusplus == 201402
34 if ( &
copy !=
this ) {
45 ::snprintf(text,
sizeof(text),
"%s(%d)",
name.c_str(),
int(
type));
56 : iovType(t), keyData(iov_value,iov_value)
63 : iovType(t), keyData(k)
74 void IOV::set(Key::first_type value) {
79 void IOV::set(Key::first_type val_1, Key::second_type val_2) {
93 Key::first_type tmp =
keyData.first;
109 if ( validity.first >
keyData.first )
110 keyData.first = validity.first;
111 if ( validity.second <
keyData.second )
112 keyData.second = validity.second;
125 if ( validity.first <
keyData.first )
126 keyData.first = validity.first;
127 if ( validity.second >
keyData.second )
128 keyData.second = validity.second;
146 ::snprintf(text,
sizeof(text),
"%s(%u):[%ld-%ld]",
151 char c_since[64], c_until[64];
153 static const Key_value_type max_time = detail::makeTime(2099,12,31,24,59,59);
154 std::time_t since = std::min(std::max(
keyData.first, nil), max_time);
155 std::time_t until = std::min(std::max(
keyData.second,nil), max_time);
156 struct tm* tm_since = ::gmtime_r(&since,&time_buff);
157 struct tm* tm_until = ::gmtime_r(&until,&time_buff);
158 if (
nullptr == tm_since ||
nullptr == tm_until ) {
159 except(
"IOV::str",
" Invalid epoch time stamp: %d:[%ld-%ld]",
162 ::strftime(c_since,
sizeof(c_since),
"%d-%m-%Y %H:%M:%S", tm_since);
163 ::strftime(c_until,
sizeof(c_until),
"%d-%m-%Y %H:%M:%S", tm_until);
164 ::snprintf(text,
sizeof(text),
"%s(%u):[%s - %s]",
169 ::snprintf(text,
sizeof(text),
"%s(%u):[%ld-%ld]",
174 ::snprintf(text,
sizeof(text),
"%u:[%ld-%ld]",
type,
long(
keyData.first),
long(
keyData.second));
void iov_intersection(const IOV &comparator)
Set the intersection of this IOV with the argument IOV.
bool contains(const IOV &iov) const
Check for validity containment.
std::string name
String name.
int optData
Optional user data.
IOV & invert()
Invert the key values (first=second and second=first)
std::int64_t Key_value_type
Key definition. Use fixed width type, though not portable!
static constexpr unsigned int UNKNOWN_IOV
std::string str() const
Conversion to string.
std::string str() const
Create string representation of the IOV.
std::pair< Key_value_type, Key_value_type > Key
Class describing the interval of validty type.
IOV()=delete
Initializing constructor: Does not set reference to IOVType !
Class describing the interval of validty.
void move(IOV &from)
Move the data content: 'from' will be reset to NULL.
IOVType & operator=(const IOVType ©)=default
Assignment operator.
void set(const Key &value)
Set discrete IOV value.
Key keyData
IOV key (if second==first, discrete, otherwise range)
static bool key_is_contained(const Key &key, const Key &test)
Check if IOV 'test' is fully contained in IOV 'key'.
unsigned int type
IOV buffer type: Must be a bitmap!
static constexpr Key_value_type MIN_KEY
void iov_union(const IOV &comparator)
Set the union of this IOV with the argument IOV.
IOV & reset()
Set keys to unphysical values (LONG_MAX, LONG_MIN)
const IOVType * iovType
Reference to IOV type.
Namespace for the AIDA detector description toolkit.
static constexpr Key_value_type MAX_KEY
unsigned int type
integer identifier used internally