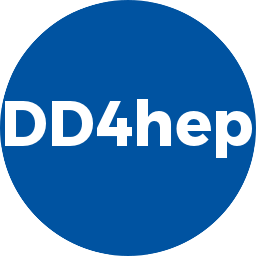 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDCOND_CONDITIONSSLICE_H
14 #define DDCOND_CONDITIONSSLICE_H
36 class ConditionsSlice;
86 std::for_each(std::begin(data), std::end(data), *
this);
92 void operator()(
const std::pair<Condition::key_type,Condition>& e)
const {
95 template <
typename T>
void process(
const T& data)
const {
96 std::for_each(std::begin(data), std::end(data), *
this);
107 std::unique_ptr<UserPool>
pool;
132 template <
typename T>
168 if ( !conds.empty() ) {
170 for(
const auto& e : conds )
204 #endif // DDCOND_CONDITIONSSLICE_H
unsigned long flags
Flag to steer conditions management.
std::vector< Condition > get(DetElement detector) const
Access all conditions from a given detector element.
ConditionsPool * registerIOV(const IOV &iov) const
Register IOV with type and key.
void manage(const T &conds, ManageFlag flg)
Insert a set of conditions to the slice AND register them to the conditions manager.
ContainedPools used_pools
If requested refence the used pools with a shared pointer to inhibit cleanup.
AlignmentCondition::Object * cond
static Condition select_cond(Condition c)
void derefPools()
Set flag to not reference the used pools during prepare (and drop possibly pending)
void process(const T &data) const
std::map< Condition::key_type, ConditionDependency * > Dependencies
virtual bool manage(ConditionsPool *pool, Condition condition, ManageFlag flg)
Local optimization: Insert a set of conditions to the slice AND register them to the conditions manag...
Helper to simplify the registration of new condtitions from arbitrary containers.
Condition::key_type key_type
ConditionsManager manager
Reference to the conditions manager.
void reset()
Clear the conditions content and the user pool.
std::shared_ptr< ConditionsContent > content
Container of conditions required by this slice.
Class describing the interval of validty type.
size_t size() const
Total size of all conditions contained in the slice.
Main condition object handle.
Class describing the interval of validty.
Handle class describing a detector element.
void refPools()
Set flag to reference the used pools during prepare.
ConditionsSlice()=delete
Default constructor.
ConditionsSlice & operator=(const ConditionsSlice ©)=delete
Default assignment operator.
Class implementing the conditions collection for a given IOV type.
bool registerUnlocked(ConditionsPool &pool, Condition cond) const
Register new condition with the conditions store. Unlocked version, not multi-threaded.
ConditionsContent::Dependencies & missingDerivations()
Access the map of missing computational conditions (only valid after preparation)
Inserter(ConditionsSlice &s)
unsigned int itemkey_type
Low part of the key identifies the item identifier.
ConditionsManager::Result status
Store the result of the prepare step.
Key key() const
Get the local key of the IOV.
ConditionsContent::Dependencies m_missingDerivations
If flag conditonsManager["OutputUnloadedConditions"]=true: will contain conditions not computed.
unsigned long long int key_type
Forward definition of the key type.
virtual void scan(const Condition::Processor &processor) const override
ConditionsMap overload: Interface to scan data content of the conditions mapping.
const IOV & iov() const
Access the combined IOV of the slice from the pool.
Manager class for condition handles.
std::unique_ptr< UserPool > pool
Reference to the user pool managing all conditions of this slice.
ConditionsContent::Conditions & missingConditions()
Access the map of missing conditions (only valid after preparation)
Result of a prepare call to the conditions manager.
const ConditionsContent::Dependencies & derived() const
Access the map of computational conditions from the desired content.
void operator()(const std::pair< Condition::key_type, Condition > &e) const
const ConditionsContent::Conditions & conditions() const
Access the map of conditions from the desired content.
const IOVType * iovType
Reference to IOV type.
Namespace for the AIDA detector description toolkit.
ConditionsContent::Conditions m_missingConditions
If flag conditonsManager["OutputUnloadedConditions"]=true: will contain conditions not loaded.
std::map< Condition::key_type, ConditionsLoadInfo * > Conditions
ConditionsPool * iov_pool
void fill_content(ConditionsManager mgr, ConditionsContent &content, const IOVType &typ)
Populate the conditions slice from the conditions manager (convenience)
Inserter(ConditionsSlice &s, const T &data)
std::vector< std::shared_ptr< ConditionsPool > > ContainedPools
virtual ~ConditionsSlice()
Default destructor.
static Condition select_cond(const std::pair< T, Condition > &c)
void operator()(Condition c) const
virtual bool insert(DetElement detector, Condition::itemkey_type key, Condition condition) override
ConditionsMap overload: Add a condition directly to the slice.
Abstract base for processing callbacks to conditions objects.
Conditions slice object. Defines which conditions should be loaded by the ConditionsManager.