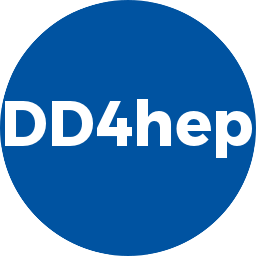 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_COMPONENTPROPERTIES_H
14 #define DD4HEP_COMPONENTPROPERTIES_H
58 template <
typename TYPE>
Property(TYPE& val);
62 static std::string
type(
const std::type_info& proptery);
66 std::string
type()
const;
70 std::string
str()
const;
74 const Property&
str(
const std::string& input)
const;
84 template <
typename TYPE> TYPE
value()
const;
86 template <
typename TYPE>
void value(TYPE&
value)
const;
88 template <
typename TYPE>
void set(
const TYPE&
value);
93 : m_par(&val), m_hdl(0)
101 if (grm.type() ==
typeid(TYPE))
102 *(TYPE*)
m_par = val;
103 else if (!grm.fromString(
m_par, BasicGrammar::instance< TYPE >().str(&val)))
116 if (grm.type() ==
typeid(TYPE))
117 val = *(TYPE*)
m_par;
118 else if (!BasicGrammar::instance< TYPE >().fromString(&val, this->
str()))
155 template <
typename T> T
value()
const {
return this->Property::value<T>();}
157 template <
typename T>
184 Properties::const_iterator
verifyExistence(
const std::string& name)
const;
194 bool exists(
const std::string& name)
const;
210 template <
typename T>
void add(
const std::string& name, T& value) {
214 template <
typename FUNCTOR>
void for_each(FUNCTOR& func) {
218 template <
typename FUNCTOR>
void for_each(
const FUNCTOR& func) {
274 virtual bool hasProperty(
const std::string& name)
const override;
278 template <
typename T>
void declareProperty(
const std::string& nam, T& val);
284 template <
typename T>
290 template <
typename T>
296 #endif // DD4HEP_COMPONENTPROPERTIES_H
void verifyNonExistence(const std::string &name) const
Verify that this property does not exist (throw exception if the name was found)
Property & operator=(const Property &p)=default
Assignment operator.
void for_each(FUNCTOR &func)
Apply functor on properties.
void declareProperty(const std::string &nam, T &val)
Declare property.
PropertyValue & operator=(const TYPE &val)
Assignment operator.
virtual Property & property(const std::string &name) override
Access single property.
The property class to assign options to actions.
std::string type() const
Property type name.
PropertyConfigurable()
Standard constructor.
T value() const
Retrieve value with data conversion.
virtual PropertyManager & properties()=0
Access to the properties of the object.
Property object as base class for all objects supporting properties.
std::string str() const
Conversion to string value.
virtual bool hasProperty(const std::string &name) const override
Check property for existence.
const BasicGrammar & grammar() const
Access grammar object.
std::map< std::string, Property > Properties
Property array definition.
bool exists(const std::string &name) const
Check for existence.
Concrete template instantiation of a combined property value pair.
virtual ~PropertyManager()
Default destructor.
void set(const T &val)
Set value of this property with data conversion.
void add(const std::string &name, T &value)
Add a new property.
Property()=default
Default constructor.
bool operator==(const TYPE &val) const
Equality operator.
void * m_par
Pointer to the data location.
PropertyValue & operator=(const PropertyValue &c)=default
Assignment operator.
Base class describing string evaluation to C++ objects using boost::spirit.
void adopt(const PropertyManager ©)
Import properties of another instance.
const Property & property(const std::string &name) const
Access property by name (CONST)
void * ptr() const
Access void data pointer.
const BasicGrammar * m_hdl
Reference to the grammar of this property (extended type description)
Property object as base class for all objects supporting properties.
void dump() const
Dump string values.
static const BasicGrammar & get(const std::type_info &info)
Access grammar by type info.
virtual const PropertyManager & properties() const override
Access to the properties of the object.
virtual Property & property(const std::string &name)=0
Access single property.
void for_each(const FUNCTOR &func)
Apply functor on properties.
Property & operator[](const std::string &name)
Access property by name.
Property(const Property &p)=default
Copy constructor.
virtual ~PropertyConfigurable()
Default destructor.
PropertyManager m_properties
Property pool.
std::string str() const
Conversion to string value.
PropertyValue()
Default constructor.
void value(TYPE &val) const
Retrieve value from stack with data conversion (large values e.g. vectors etc.)
void add(const std::string &name, const Property &property)
Add a new property.
TYPE value() const
Retrieve value.
void set(const TYPE &value)
Set value of this property.
virtual PropertyManager & properties() override
Access to the properties of the object.
const BasicGrammar & grammar() const
Access grammar object.
Manager to ease the handling of groups of properties.
size_t size() const
Access total number of properties.
Namespace for the AIDA detector description toolkit.
Properties::iterator verifyExistence(const std::string &name)
Verify that this property exists (throw exception if the name was not found)
virtual const PropertyManager & properties() const =0
Access to the properties of the object.
Properties m_properties
Property array/map.
Properties & properties()
Access to the property container.
virtual ~PropertyInterface()=default
Default destructor.
static void invalidConversion(const std::type_info &from, const std::type_info &to)
Error callback on invalid conversion.
PropertyValue(const PropertyValue &c)=default
Copy constructor.
const Properties & properties() const
Access to the property container.
PropertyManager()
Default constructor.
virtual bool hasProperty(const std::string &name) const =0
Check property for existence.