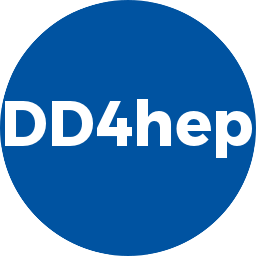 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
15 #ifdef DD4HEP_USE_TINYXML
18 #include <xercesc/util/Xerces_autoconf_config.hpp>
19 #include <xercesc/util/XMLString.hpp>
20 #include <xercesc/dom/DOMElement.hpp>
21 #include <xercesc/dom/DOMDocument.hpp>
22 #include <xercesc/dom/DOMNodeList.hpp>
23 #include <xercesc/dom/DOM.hpp>
41 static const size_t INVALID_NODE = ~0U;
42 static int s_float_precision = -1;
46 std::pair<int, double>
_toInteger(
const std::string& value);
48 void _toDictionary(
const std::string& name,
const std::string& value,
const std::string& typ);
54 bool s_resolve_environment =
true;
55 std::string _checkEnviron(
const std::string& env) {
56 if ( s_resolve_environment ) {
58 return r.empty() ? env : r;
65 #define _D(x) Xml(x).d
66 #define _E(x) Xml(x).e
67 #define _N(x) Xml(x).n
68 #define _L(x) Xml(x).l
69 #define _XE(x) Xml(x).xe
71 #ifdef DD4HEP_USE_TINYXML
72 #define ELEMENT_NODE_TYPE TiXmlNode::ELEMENT
73 #define getTagName Value
74 #define getTextContent GetText
76 #define getValue Value
77 #define getNodeValue Value
78 #define getNodeType Type
79 #define setNodeValue SetValue
80 #define getParentNode Parent()->ToElement
81 #define getAttributeNode(x) AttributeNode(x)
82 #define appendChild LinkEndChild
83 #define getOwnerDocument GetDocument
84 #define getDocumentElement RootElement
85 #define getDocumentURI Value
89 Xml(
const void* ptr) : p(ptr) {}
99 XmlElement* node_first(XmlElement* e,
const Tag_t& t) {
100 if ( t.
str()==
"*" )
return e ? (XmlElement*)
_E(e)->FirstChildElement() : 0;
101 return e ? (XmlElement*)
_E(e)->FirstChildElement(t.
str()) : 0;
103 size_t node_count(XmlElement* elt,
const Tag_t& t) {
114 return c ? ::strdup(c) : 0;
119 if(p && *p) {::free(*p); *p=0;}
122 return p ? ::strlen(p) : 0;
126 #define ELEMENT_NODE_TYPE xercesc::DOMNode::ELEMENT_NODE
136 xercesc::DOMElement*
e;
137 xercesc::DOMDocument*
d;
138 xercesc::DOMNodeList*
l;
143 return xercesc::XMLString::replicate(c);
146 return xercesc::XMLString::transcode(c);
149 return xercesc::XMLString::transcode(c);
152 return xercesc::XMLString::release(p);
155 return xercesc::XMLString::release(p);
158 return p ? xercesc::XMLString::stringLen(p) : 0;
161 return p ? xercesc::XMLString::stringLen(p) : 0;
165 size_t node_count(XmlElement* e,
const Tag_t& t) {
168 const std::string& tag = t;
169 xercesc::DOMElement *ee =
Xml(e).
e;
171 for(xercesc::DOMElement* elt=ee->getFirstElementChild(); elt; elt=elt->getNextElementSibling()) {
172 if ( elt->getParentNode() == ee ) {
173 std::string child_tag =
_toString(elt->getTagName());
174 if ( tag ==
"*" || child_tag == tag ) ++cnt;
181 XmlElement* node_first(XmlElement* e,
const Tag_t& t) {
183 const std::string& tag = t;
184 xercesc::DOMElement* ee =
Xml(e).
e;
186 for(xercesc::DOMElement* elt=ee->getFirstElementChild(); elt; elt=elt->getNextElementSibling()) {
187 if ( elt->getParentNode() == ee ) {
188 if ( tag ==
"*" )
return _XE(elt);
189 std::string child_tag =
_toString(elt->getTagName());
190 if ( child_tag == tag )
return _XE(elt);
202 std::string tmp(buff == 0 ?
"" : buff);
204 if ( tmp.length()<3 )
return tmp;
205 if ( !(tmp[0] ==
'$' && tmp[1] ==
'{') )
return tmp;
206 tmp = _checkEnviron(tmp);
216 return Xml(a).
a->getValue();
219 int node_type(XmlNode* n) {
return Xml(n).
n->getNodeType();}
226 int tmp = s_float_precision;
227 s_float_precision = precision;
233 return s_float_precision;
243 template <
typename T>
static inline std::string __to_string(T value,
const char* fmt) {
245 ::snprintf(text,
sizeof(text), fmt, value);
251 if ( !s || *s == 0 )
return "";
252 else if ( !(*s ==
'$' && *(s+1) ==
'{') )
return s;
253 return _checkEnviron(s);
258 if ( s.length() < 3 || s[0] !=
'$' )
return s;
259 else if ( !(s[0] ==
'$' && s[1] ==
'{') )
return s;
260 return _checkEnviron(s);
265 return __to_string(
v, fmt);
270 return __to_string(
v, fmt);
275 return __to_string(
v, fmt);
280 return __to_string(
v, fmt);
285 if ( s_float_precision >= 0 ) {
287 ::snprintf(format,
sizeof(format),
"%%.%de", s_float_precision);
288 return __to_string(
v, format);
290 return __to_string(
v, fmt);
295 if ( s_float_precision >= 0 ) {
297 ::snprintf(format,
sizeof(format),
"%%.%de", s_float_precision);
298 return __to_string(
v, format);
300 return __to_string(
v, fmt);
315 return __to_string(
v, fmt);
328 if ( val >= 0 )
return (
unsigned long) val;
330 throw std::runtime_error(
"dd4hep: Severe error during expression evaluation of " + s);
338 return (
unsigned int)
_toULong(value);
344 char c = ::toupper(s[0]);
345 if ( c ==
'T' || c ==
'1' )
return true;
346 if ( c ==
'F' || c ==
'0' )
return false;
347 return _toInt(value) != 0;
383 template <
typename T>
386 const XmlChar* item_value = item;
390 #ifndef DD4HEP_USE_TINYXML
412 bool tmp = s_resolve_environment;
413 s_resolve_environment = new_value;
417 template <
typename B>
418 static inline std::string i_add(
const std::string& a, B b) {
445 std::string res = a.
str() + b;
450 std::string res = a + b.
str();
460 std::string res = a.
str() + b;
464 #ifndef DD4HEP_USE_TINYXML
568 : m_tag(
copy.m_tag), m_node(
copy.m_node), m_ptr(0)
575 : m_tag(tag_value), m_node(node), m_ptr(0)
591 #ifdef DD4HEP_USE_TINYXML
597 for(elt=elt->getNextElementSibling(); elt; elt=elt->getNextElementSibling()) {
599 std::string child_tag =
_toString(elt->getTagName());
608 #ifdef DD4HEP_USE_TINYXML
614 for(elt=elt->getPreviousElementSibling(); elt; elt=elt->getPreviousElementSibling()) {
616 std::string child_tag =
_toString(elt->getTagName());
640 return _E(
m_node)->getTextContent();
651 #ifdef DD4HEP_USE_TINYXML
654 XmlElement* e =
_XE(
_N(
m_node)->Clone()->ToElement());
657 throw std::runtime_error(
"TiXml: Handle_t::clone: Invalid source handle type [No element type].");
662 throw std::runtime_error(
"Xml: Handle_t::clone: Invalid source handle.");
672 return attribute_node(
m_node, tag_value);
682 std::vector < Attribute > attrs;
684 #ifdef DD4HEP_USE_TINYXML
688 xercesc::DOMNamedNodeMap* l =
_E(
m_node)->getAttributes();
689 for (
XmlSize_t i = 0, n = l->getLength(); i < n; ++i) {
690 xercesc::DOMNode* attr_node = l->item(i);
691 attrs.emplace_back(
Attribute(attr_node));
699 size_t n = node_count(
m_node, t);
700 if (n == INVALID_NODE && !throw_exception)
702 else if (n != INVALID_NODE)
704 std::string msg =
"Handle_t::numChildren: ";
706 msg +=
"Element [" +
tag() +
"] has no children of type '" +
_toString(t) +
"'";
708 msg +=
"Element [INVALID] has no children of type '" +
_toString(t) +
"'";
709 throw std::runtime_error(msg);
715 if (e || !throw_exception)
717 std::string msg =
"Handle_t::child: ";
719 msg +=
"Element [" +
tag() +
"] has no child of type '" +
_toString(t) +
"'";
721 msg +=
"Element [INVALID]. Cannot remove child of type: '" +
_toString(t) +
"'";
722 throw std::runtime_error(msg);
736 #ifdef DD4HEP_USE_TINYXML
743 std::string msg =
"Handle_t::remove: ";
745 msg +=
"Element [" +
tag() +
"] has no child of type '" + node.
tag() +
"'";
747 msg +=
"Element [INVALID]. Cannot remove child of type: '" + node.
tag() +
"'";
749 msg +=
"Element [INVALID]. Cannot remove [INVALID] child. Big Shit!!!!";
751 throw std::runtime_error(msg);
756 #ifdef DD4HEP_USE_TINYXML
760 xercesc::DOMElement* e =
_E(
m_node);
761 xercesc::DOMNodeList* l = e->getElementsByTagName(tag_value);
762 for (
XmlSize_t i = 0, n = l->getLength(); i < n; ++i)
763 e->removeChild(l->item(i));
768 return node_first(
m_node, tag_value) != 0;
773 _N(
m_node)->setNodeValue(text_value);
778 #ifdef DD4HEP_USE_TINYXML
779 _N(
m_node)->setNodeValue(text_value.c_str());
787 #ifdef DD4HEP_USE_TINYXML
790 _N(
m_node)->setTextContent(text_value);
796 #ifdef DD4HEP_USE_TINYXML
805 #ifdef DD4HEP_USE_TINYXML
808 xercesc::DOMElement* e =
_E(
m_node);
809 xercesc::DOMNamedNodeMap* l = e->getAttributes();
810 for (
XmlSize_t i = 0, n = l->getLength(); i < n; ++i) {
811 xercesc::DOMAttr* a = (xercesc::DOMAttr*) l->item(i);
812 e->removeAttributeNode(a);
818 #ifdef DD4HEP_USE_TINYXML
828 xercesc::DOMElement* e =
_E(
m_node);
829 xercesc::DOMNamedNodeMap* l = e->getAttributes();
830 for (
XmlSize_t i = 0, len = l->getLength(); i < len; ++i) {
831 xercesc::DOMNode* n = l->item(i);
832 if (n->getNodeType() == xercesc::DOMNode::ATTRIBUTE_NODE) {
833 xercesc::DOMAttr* a = (xercesc::DOMAttr*) n;
834 e->setAttribute(a->getName(), a->getValue());
845 std::string msg =
"Handle_t::attr_ptr: ";
847 msg +=
"Element [" +
tag() +
"] has no attribute of type '" +
_toString(t) +
"'";
849 msg +=
"Element [INVALID] has no attribute of type '" +
_toString(t) +
"'";
850 throw std::runtime_error(msg);
856 return Xml(a).
a->getName();
858 throw std::runtime_error(
"Attempt to access invalid XML attribute object!");
863 return attribute_value(
attr_ptr(attr_tag));
868 return attribute_value(attr_val);
874 return a ? attribute_value(a) : 0;
880 ::snprintf(txt,
sizeof(txt),
"%d", val);
887 ::snprintf(txt,
sizeof(txt),
"%s", val ?
"true" :
"false");
894 ::snprintf(txt,
sizeof(txt),
"%.8e", val);
901 ::snprintf(txt,
sizeof(txt),
"%.8e", val);
910 #ifndef DD4HEP_USE_TINYXML
918 return v ?
setAttr(nam, attribute_value(
v)) : 0;
923 #ifdef DD4HEP_USE_TINYXML
928 xercesc::DOMElement* e =
_E(
m_node);
929 xercesc::DOMAttr* a = e->getAttributeNode(nam);
931 a = e->getOwnerDocument()->createAttribute(nam);
932 e->setAttributeNode(a);
943 ref.
setAttr(Unicode_ref, ref_name);
954 static unsigned int adler32(
unsigned int adler,
const XmlChar* xml_buff,
size_t len) {
955 #define DO1(buf,i) {s1 +=(unsigned char)buf[i]; s2 += s1;}
956 #define DO2(buf,i) DO1(buf,i); DO1(buf,i+1);
957 #define DO4(buf,i) DO2(buf,i); DO2(buf,i+2);
958 #define DO8(buf,i) DO4(buf,i); DO4(buf,i+4);
959 #define DO16(buf) DO8(buf,0); DO8(buf,8);
961 static const unsigned int BASE = 65521;
963 static const unsigned int NMAX = 5550;
964 unsigned int s1 = adler & 0xffff;
965 unsigned int s2 = (adler >> 16) & 0xffff;
966 const char* buf = (
const char*)xml_buff;
972 int k = len < NMAX ? (int) len : NMAX;
981 s1 += (
unsigned char) *buf++;
987 unsigned int result = (s2 << 16) | s1;
994 #ifdef DD4HEP_USE_TINYXML
995 typedef std::map<std::string, std::string> StringMap;
998 if ( 0 == fcn ) fcn = adler32;
1001 std::map<std::string,std::string> m;
1005 param = (*fcn)(param,e->
Value(),::strlen(e->
Value()));
1006 for(StringMap::const_iterator i=m.begin();i!=m.end();++i) {
1007 param = (*fcn)(param,(*i).first.c_str(),(*i).first.length());
1008 param = (*fcn)(param,(*i).second.c_str(),(*i).second.length());
1026 if ( 0 == fcn ) fcn = adler32;
1035 #ifdef DD4HEP_USE_TINYXML
1038 return _XE(
_D(
m_doc)->createElement(tag_value));
1046 throw std::runtime_error(
"Document::root: Invalid handle!");
1055 throw std::runtime_error(
"Document::uri: Invalid handle!");
1061 printout(DEBUG,
"DocumentHolder",
"+++ Release DOM document....");
1062 #ifdef DD4HEP_USE_TINYXML
1079 : m_element(
Xml(doc.createElt(type)).xe) {
1101 throw std::runtime_error(
"Element::clone: Invalid element pointer -- unable to clone node!");
1136 #ifndef DD4HEP_USE_TINYXML
1145 #ifdef DD4HEP_USE_TINYXML
1171 :
Element(e), m_name(e.m_name) {
1182 std::cout <<
"Error:tag=" <<
m_element.
tag() << std::endl;
1183 return attribute_value(
m_name);
1188 std::cout <<
"Error:tag=" <<
m_element.
tag() << std::endl;
1189 return attribute_value(
m_name);
1196 #ifndef DD4HEP_USE_TINYXML
1198 : m_children(element, tag_value) {
1204 : m_children(element,
Strng_t(tag_value)) {
1210 : m_children(node_list) {
1228 throw std::runtime_error(std::string(e.what()) +
"\n" +
"dd4hep: Error interpreting XML nodes of type <" +
tag() +
"/>");
1230 throw std::runtime_error(std::string(e.what()) +
"\n" +
"dd4hep: Error interpreting collections XML nodes.");
1264 #ifdef DD4HEP_USE_TINYXML
1271 #ifdef DD4HEP_USE_TINYXML
void throw_loop_exception(const std::exception &e) const
Helper function to throw an exception.
void setValue(const XmlChar *text) const
Set the element's value.
static XmlChar * replicate(const XmlChar *c)
Replicate string: internally allocates new string, which must be free'ed with release.
TinyXML class. See http://www.grinninglizard.com/tinyxml.
Document document() const
Access the hosting document handle of this DOM element.
Class to support the access to collections of XmlNodes (or XmlElements)
Handle_t setChild(const XmlChar *tag) const
Check if a child with the required tag exists - if not create it and add it to the current node.
std::string m_str
STL string buffer.
TinyXML class. See http://www.grinninglizard.com/tinyxml.
const XmlChar * getRef(const XmlChar *tag) const
Access the value of the reference attribute of the node (attribute ref="ref-name")
XmlElement * next() const
Advance to next element.
std::string tag() const
Text access to the element's tag.
std::string uri() const
Acces the document URI.
double _toDouble(const XmlChar *value)
Conversion function from raw unicode string to double.
void setName(const XmlChar *new_name)
Change/set the object's name.
const XmlChar * cpXmlChar
void append(Handle_t handle) const
Append a new element to the existing tree.
NodeList & operator=(const NodeList &l)
Assignment operator.
Helper structure to shortcut type definitions for the factories.
Class describing a list of XML nodes.
Handle_t clone(XmlDocument *new_doc) const
Clone the DOMelement - with the option of a deep copy.
void exception(const std::string &src, const std::string &msg)
unsigned int _toUInt(const XmlChar *value)
Conversion function from raw unicode string to unsigned int.
const XmlChar * attr_value_nothrow(const XmlChar *attr) const
Access attribute value by the attribute's unicode name (no exception thrown if not present)
Handle_t m_element
The underlying object holding the XmlElement pointer.
const XmlAttr * Attribute
XmlElement * previous() const
Go back to previous element.
void _toDictionary(const std::string &name, const std::string &value)
Enter name value pair to the dictionary. "value" must be a numerical expression, which is evaluated.
Handle_t setRef(const XmlChar *tag, const XmlChar *ref)
Add reference child as a new child node. The obj must have the "name" attribute!
void append(Handle_t e) const
Append a DOM element to the current node.
Tag_t & operator=(const char *s)
Assignment of a normal ASCII string.
Handle_t parent() const
Access the element's parent element.
long _toLong(const XmlChar *value)
Conversion function from raw unicode string to long.
Handle_t addChild(const XmlChar *tag) const
Add a new child to the DOM node.
const TiXmlNode * FirstChild() const
The first child of this node. Will be null if there are no children.
unsigned int() fcn_t(unsigned int, const XmlChar *, size_t)
Checksum (sub-)tree of a xml document/tree.
virtual const TiXmlElement * ToElement() const
Cast to a more defined type. Will return null if not of the requested type.
static size_t length(const char *s)
String length in native representation.
Handle_t createElt(const XmlChar *tag) const
Create DOM element.
Handle_t remove(Handle_t e) const
Remove a single child node identified by its handle from the tree of the element.
Class to easily access the properties of single XmlElements.
bool enableEnvironResolution(bool new_value)
Enable/disable environment resolution when parsing strings.
void setAttrs(Handle_t e) const
Set attributes as in argument handle.
Handle_t(Elt_t e=0)
Initializing constructor.
XmlElement * reset()
Reset the nodelist - e.g. restart iteration from beginning.
const char * Value() const
Return the value of this attribute.
void _toDictionary(const XmlChar *name, const XmlChar *value)
Helper function to populate the evaluator dictionary.
~NodeList()
Default destructor.
size_t numChildren(const XmlChar *tag, bool throw_exception) const
Access the number of children of this DOM element with a given tag name.
Handle_t::Elt_t Elt_t
Simplification type declarations.
const XmlChar * attr_name(const Attribute attr) const
Access attribute name (throws exception if not present)
NodeList(const NodeList &l)
Copy constructor.
const XmlChar * rawText() const
Unicode text access to the element's text.
Class to support both way translation between C++ and XML strings.
bool hasChild(const XmlChar *tag) const
Check the existence of a child with a given tag name.
int get_float_precision()
Access floating point precision on conversion to string.
const TiXmlAttribute * FirstAttribute() const
Access the first attribute in this element.
const XmlChar * refName() const
Access the object's reference name in unicode (same as name)
Collection_t & reset()
Reset the collection object to restart the iteration.
void removeChildren(const XmlChar *tag) const
Remove children with a given tag name from the DOM node.
XmlChar * m_xml
Pointer to unicode string.
TinyXML class. See http://www.grinninglizard.com/tinyxml.
const XmlChar * attr_value(const Attribute attr) const
Access attribute value by the attribute (throws exception if not present)
const TiXmlElement * NextSiblingElement() const
Handle_t child(const Strng_t &tag_value, bool except=true) const
Access child by tag name. Thow an exception if required in case the child is not present.
int set_float_precision(int precision)
Change floating point precision on conversion to string.
std::pair< int, double > _toInteger(const std::string &value)
int _toInt(const XmlChar *value)
Conversion function from raw unicode string to int.
virtual ~DocumentHolder()
Standard destructor - releases the document.
Namespace for the AIDA detector description toolkit supporting XML utilities.
Attribute setRef(const XmlChar *tag, const XmlChar *refname) const
Set the reference attribute to the node (adds attribute ref="ref-name")
void SetAttribute(const char *name, const char *_value)
Attribute m_name
Attribute holding thre name.
#define ELEMENT_NODE_TYPE
NodeList children(const XmlChar *tag) const
Access a group of children identified by the same tag name.
const XmlChar * name() const
Access the object's name in unicode.
static void release(char **p)
Release string.
Attribute attr_ptr(const XmlChar *attr) const
Access attribute pointer by the attribute's unicode name (throws exception if not present)
Elt_t ptr() const
Access to XmlElement pointer.
Element(const Handle_t &e)
Constructor from XmlElement handle.
Attribute getAttr(const XmlChar *name) const
Access single attribute by its name.
std::pair< int, double > _toFloatingPoint(const std::string &value)
std::string _toString(const Attribute attr)
Convert xml attribute to STL string.
const TiXmlAttribute * Next() const
Get the next sibling attribute in the DOM. Returns null at end.
static XmlChar * transcode(const char *c)
Transcode string.
std::string _getEnviron(const std::string &env)
Evaluate string constant using environment stored in the evaluator.
Strng_t & operator=(const XmlChar *s)
Assignment opertor from unicode string.
TinyXML class. See http://www.grinninglizard.com/tinyxml.
Helper class to encapsulate a unicode string.
Class supporting the basic functionality of an XML document including ownership.
Handle_t child(const XmlChar *tag, bool throw_exception=true) const
Access a single child by its tag name (unicode)
Handle_t clone(const Document &new_doc) const
Clone the DOMelement.
void setText(const XmlChar *text) const
Set the element's text.
Handle_t clone(Handle_t source) const
Clone a DOM element / sub-tree.
DocumentHolder & assign(DOC d)
Assign new document. Old document is dropped.
Class supporting the basic functionality of an XML document.
const TiXmlElement * FirstChildElement() const
Convenience function to get through elements.
RefElement(const Handle_t &e)
Construction from existing object handle.
Strng_t operator+(const Strng_t &a, const char *b)
Unicode string concatenation of a normal ASCII string from right side.
Elt_t parentElement() const
Access the XmlElements parent.
RefElement & operator=(const RefElement &e)
Assignment operator.
size_t size() const
Access the collection size. Avoid this call – sloooow!
Elt_t m_node
The pointer to the XmlElement.
User abstraction class to manipulate named XML elements (references) within a document.
const XmlChar * ptr() const
Accessor to unicode string.
unsigned long _toULong(const XmlChar *value)
Conversion function from raw unicode string to unsigned long.
const char * Value() const
Collection_t(Handle_t node, const XmlChar *tag)
Constructor over XmlElements with a given tag name.
const XmlChar * rawTag() const
Unicode text access to the element's tag. Tis must be wrong ....
float _toFloat(const XmlChar *value)
Conversion function from raw unicode string to float.
XERCES_XMLCH_T XmlChar
Use the definition from the autoconf header of Xerces:
Union to ease castless object access when using XercesC.
User abstraction class to manipulate XML elements within a document.
Namespace for the AIDA detector description toolkit.
const std::string & str() const
Access writable STL string.
std::vector< Attribute > attributes() const
Retrieve a collection of all attributes of this DOM element.
Attribute setAttr(const XmlChar *nam, const T &val) const
Set single attribute.
std::string _ptrToString(const void *p, const char *fmt="%p")
Format void pointer (64 bits) to string with arbitrary format.
std::string getEnviron(const std::string &env)
Helper function to lookup environment from the expression evaluator.
NodeList m_children
Reference to the list of child nodes.
Attribute setAttr(const XmlChar *t, const XmlChar *v) const
Generic attribute setter with unicode value.
const TiXmlAttribute * AttributeNode(const char *name) const
const char * Name() const
Return the name of this attribute.
unsigned int checksum(unsigned int param, unsigned int(fcn)(unsigned int param, const XmlChar *, size_t)=0) const
Checksum (sub-)tree of a xml document/tree. Default will pick up the adler32 checksum.
bool hasAttr(const XmlChar *t) const
Check for the existence of a named attribute.
virtual const TiXmlText * ToText() const
Cast to a more defined type. Will return null if not of the requested type.
void operator++() const
Operator to advance the collection (pre increment)
const TiXmlNode * NextSibling(const std::string &_value) const
STL std::string form.
const XmlChar * rawValue() const
Unicode text access to the element's value.
TinyXML class. See http://www.grinninglizard.com/tinyxml.
T attr(const Attribute a) const
Access typed attribute value by the XmlAttr.
bool _toBool(const XmlChar *value)
Conversion function from raw unicode string to bool.
Elt_t ptr() const
Direct access to the XmlElement by function.
void operator--() const
Operator to advance the collection (pre decrement)
void removeAttrs() const
Remove all attributes of this element.
void addComment(const XmlChar *text) const
Add comment node to the element.
Attribute attr_nothrow(const XmlChar *tag) const
Access attribute pointer by the attribute's unicode name (no exception thrown if not present)
Handle_t root() const
Access the ROOT eleemnt of the DOM document.