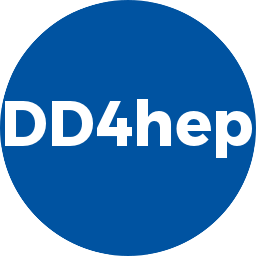 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_HANDLE_H
14 #define DD4HEP_HANDLE_H
24 #define RAD_2_DEGREE 57.295779513082320876798154814105
27 #define DEGREE_2_RAD 0.0174532925199432957692369076848
31 #define M_PI 3.14159265358979323846
52 return 0xFEEDAFFEDEADFACEULL;
103 :
m_element(element ? detail::safe_cast<T>::cast(element) : 0)
143 operator T&()
const {
155 template <
typename Q> Q*
_ptr()
const {
159 template <
typename Q> Q*
data()
const {
163 template <
typename Q> Q&
object()
const {
173 void assign(
Object* n,
const std::string& nam,
const std::string& title);
177 static void bad_assignment(
const std::type_info& from,
const std::type_info& to);
184 deletePtr(
handle.m_element);
199 void operator()(
const std::pair<typename M::key_type, typename M::mapped_type>& arg)
const
210 releasePtr(
handle.m_element);
225 void operator()(
const std::pair<typename M::key_type, typename M::mapped_type>& arg)
const
241 std::string
_toString(
short value,
const char* fmt =
"%d");
243 std::string
_toString(
int value,
const char* fmt =
"%d");
245 std::string
_toString(
unsigned long value,
const char* fmt =
"%ld");
247 std::string
_toString(
float value,
const char* fmt =
"%.17e");
249 std::string
_toString(
double value,
const char* fmt =
"%.17e");
251 std::string
_ptrToString(
const void* p,
const char* fmt =
"%p");
253 template <
typename T> std::string
_toString(
const T* p,
const char* fmt =
"%p")
257 template <
typename T> T
_toType(
const std::string& value);
260 bool _toBool(
const std::string& value);
262 short _toShort(
const std::string& value);
264 int _toInt(
const std::string& value);
266 long _toLong(
const std::string& value);
268 unsigned short _toUShort(
const std::string& value);
270 unsigned int _toUInt(
const std::string& value);
272 unsigned long _toULong(
const std::string& value);
274 float _toFloat(
const std::string& value);
276 double _toDouble(
const std::string& value);
299 inline unsigned int _toUInt(
unsigned int value) {
303 inline unsigned long _toULong(
unsigned long value) {
316 template <
class T> T
_multiply(
const std::string& left, T right);
318 template <
class T> T
_multiply(T left,
const std::string& right);
320 template <
class T> T
_multiply(
const std::string& left,
const std::string& right);
323 template <>
char _multiply<char>(
const std::string& left,
const std::string& right);
327 return left *
_toInt(right);
331 return _toInt(left) * right;
339 return left *
_toInt(right);
343 return _toInt(left) * right;
347 template <>
short _multiply<short>(
const std::string& left,
const std::string& right);
350 return left *
_toInt(right);
354 return _toInt(left) * right;
362 return left *
_toInt(right);
366 return _toInt(left) * right;
370 template <>
int _multiply<int>(
const std::string& left,
const std::string& right);
374 return left *
_toInt(right);
378 return _toInt(left) * right;
386 return left *
_toInt(right);
390 return _toInt(left) * right;
394 template <>
long _multiply<long>(
const std::string& left,
const std::string& right);
418 template <>
float _multiply<float>(
const std::string& left,
const std::string& right);
430 template <>
double _multiply<double>(
const std::string& left,
const std::string& right);
442 void _toDictionary(
const std::string& name,
const std::string& value);
444 void _toDictionary(
const std::string& name,
const std::string& value,
const std::string& typ);
455 #endif // DD4HEP_HANDLE_H
bool set_allow_variable_redefine(bool value)
Steer redefinition of variable re-definition during expression evaluation. returns old value.
void releaseHandles(M &arg)
Functional created of map destruction functors.
short _toShort(const std::string &value)
String conversions: string to short value.
bool _toBool(const std::string &value)
String conversions: string to boolean value.
void warning_deprecated_xml_factory(const char *name)
Function tp print warning about deprecated factory usage. Used by Plugin mechanism.
DestroyHandles(M &obj)
Initializing constructor.
void increment_object_validations()
void destroyHandle(T &handle)
Helper to delete objects from heap and reset the handle.
M & object
Container reference.
map Functor to release handles
T Object
Extern accessible definition of the contained element type.
bool operator>(const Handle< T > &element) const
Boolean operator > used for RB tree insertions.
Q & object() const
Access to an unrelated object type.
void _toDictionary(const std::string &name, const std::string &value)
Enter name value pair to the dictionary. "value" must be a numerical expression, which is evaluated.
bool operator!() const
Check the validity of the object held by the handle.
unsigned int _multiply< unsigned int >(const std::string &left, const std::string &right)
Generic multiplication using the evaluator: result = left * right.
Handle< T > & operator=(Handle< T > &&element)=default
Assignment move operator.
bool operator<(const Handle< T > &element) const
Boolean operator < used for RB tree insertions.
Handle(const Handle< T > &element)=default
Copy constructor.
bool isValid() const
Check the validity of the object held by the handle.
Handle: a templated class like a shared pointer, which allows specialized access to tgeometry objects...
std::string _toString(bool value)
String conversions: boolean value to string.
short _multiply< short >(const std::string &left, const std::string &right)
Generic multiplication using the evaluator: result = left * right.
unsigned long long int magic_word()
Access to the magic word, which is protecting some objects against memory corruptions.
int _toInt(const std::string &value)
String conversions: string to integer value.
unsigned long _multiply< unsigned long >(const std::string &left, const std::string &right)
Generic multiplication using the evaluator: result = left * right.
void operator()(const std::pair< typename M::key_type, typename M::mapped_type > &arg) const
Action operator.
const char * name() const
Access the object name (or "" if not supported by the object)
int _multiply< int >(const std::string &left, const std::string &right)
Generic multiplication using the evaluator: result = left * right.
unsigned long _toULong(const std::string &value)
String conversions: string to long integer value.
long _toLong(const std::string &value)
String conversions: string to long integer value.
Handle(const Handle< Q > &element)
Initializing constructor from unrelated handle with type checking.
T & operator*() const
Access the held object using the * operator.
unsigned short _toUShort(const std::string &value)
String conversions: string to unsigned short value.
Handle< T > & clear()
Release the object held by the handle.
float _multiply< float >(const std::string &left, const std::string &right)
Generic multiplication using the evaluator: result = left * right.
std::string remove_whitespace(const std::string &v)
String manipulations: Remove unconditionally all white spaces.
T * operator->() const
Access the held object using the -> operator.
static void bad_assignment(const std::type_info &from, const std::type_info &to)
Helper routine called when unrelated types are assigned.
Handle()=default
Default constructor.
Handle(Q *element)
Initializing constructor from unrelated pointer with type checking.
void assign(Object *n, const std::string &nam, const std::string &title)
Assign a new named object. Note: object references must be managed by the user.
Q * data() const
Access to an unrelated object type.
unsigned short _multiply< unsigned short >(const std::string &left, const std::string &right)
Generic multiplication using the evaluator: result = left * right.
unsigned char _multiply< unsigned char >(const std::string &left, const std::string &right)
Generic multiplication using the evaluator: result = left * right.
long _multiply< long >(const std::string &left, const std::string &right)
Generic multiplication using the evaluator: result = left * right.
void destroyHandles(M &arg)
Functional created of map destruction functors.
void operator()(T handle) const
void releaseHandle(T &handle)
Helper to delete objects from heap and reset the handle.
std::string _ptrToString(const void *p, const char *fmt="%p")
Pointer to text conversion.
map Functor to destroy handles and delete the cached object
T * m_element
Single and only data member: Reference to the actual element.
Q * _ptr() const
Access to an unrelated object type.
bool operator==(const Handle< T > &element) const
Boolean operator == used for RB tree insertions.
long num_object_validations()
char _multiply< char >(const std::string &left, const std::string &right)
Generic multiplication using the evaluator: result = left * right.
void destroy()
Destroy the underlying object (be careful here: things are not reference counted)!
Handle(T *element)
Initializing constructor from pointer.
Handle< T > Base
Self type: used by sub-classes.
void operator()(T ptr) const
Functor to destroy handles and delete the cached object.
Handle< NamedObject > Ref_t
Default Ref_t definition describing named objects.
M & object
Container reference.
float _toFloat(const std::string &value)
String conversions: string to float value.
double _toDouble(const std::string &value)
String conversions: string to double value.
T _multiply(const std::string &left, T right)
Generic multiplication using the evaluator: result = left * right.
Handle< T > & operator=(const Handle< T > &element)=default
Assignment copy operator.
T * access() const
Checked object access. Throws invalid handle runtime exception if invalid handle.
T * ptr() const
Access to the held object.
double _multiply< double >(const std::string &left, const std::string &right)
Generic multiplication using the evaluator: result = left * right.
Namespace for the AIDA detector description toolkit.
T _toType(const std::string &value)
Generic type conversion from string to primitive value.
Functor to destroy handles and delete the cached object.
ReleaseHandles(M &obj)
Initializing constructor.
void operator()(const std::pair< typename M::key_type, typename M::mapped_type > &arg) const
Action operator.
void handle(const O *o, const C &c, F pmf)
unsigned int _toUInt(const std::string &value)
String conversions: string to unsigned integer value.
Handle(Handle< T > &&element)=default
Copy constructor.