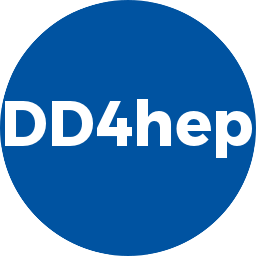 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
27 static std::string s_empty_string;
42 this->
assign(det_data, det_name, det_type);
61 det_parent.
add(*
this);
88 if (o->placementPath.empty()) {
91 return o->placementPath;
93 return s_empty_string;
129 o->
key = dd4hep::detail::hash32(o->
path);
161 if ( !o->path.empty() )
166 return s_empty_string;
187 if ( !o->nominal.isValid() ) {
189 o->nominal->values().detector = *
this;
200 if ( !o->survey.isValid() ) {
215 Children::const_iterator i = c.find(child_name);
216 if ( i != c.end() )
return (*i).second;
217 throw std::runtime_error(
"dd4hep: DetElement::child Unknown child with name: "+child_name);
219 throw std::runtime_error(
"dd4hep: DetElement::child: Self is not defined [Invalid Handle]");
226 Children::const_iterator i = c.find(child_name);
227 if ( i != c.end() )
return (*i).second;
228 if ( throw_if_not_found ) {
229 throw std::runtime_error(
"dd4hep: DetElement::child Unknown child with name: "+child_name);
232 if ( throw_if_not_found ) {
233 throw std::runtime_error(
"dd4hep: DetElement::child: Self is not defined [Invalid Handle]");
247 return (o) ? o->world() :
World();
253 throw std::runtime_error(
"dd4hep: " + msg);
260 auto r = object<Object>().
children.emplace(sdet.
name(), sdet);
266 "DetElement::add: Element %s is already present in path %s [Double-Insert]",
267 sdet.
name(), this->path().c_str());
269 except(
"dd4hep",
"DetElement::add: Self is not defined [Invalid Handle]");
270 throw std::runtime_error(
"dd4hep: DetElement::add");
278 n->SetTitle(o->GetTitle());
290 n->SetTitle(o->GetTitle());
306 except(
"DetElement",
"reflect: Only placed DetElement objects can be reflected: %s",
314 Object& o = object<Object>();
323 Object& o = object<Object>();
334 if ( !o->idealPlace.isValid() ) {
339 except(
"dd4hep",
"DetElement::setPlacement: Placement is not defined [Invalid Handle]");
340 throw std::runtime_error(
"dd4hep: DetElement::add");
346 return object<Object>().volumeID;
358 return volume()->GetShape();
382 const std::string& region,
383 const std::string& limits,
384 const std::string& vis)
398 object<Object>().ecut = 0e0;
399 object<Object>().verbose = 0;
453 int v = value ? 1 : 0;
465 int v = value ? 1 : 0;
Handle class to hold the information of the top DetElement object 'world'.
const Children & children() const
Access to the list of children.
const std::string & path() const
Path of the detector element (not necessarily identical to placement path!)
IDDescriptor idSpec() const
Access IDDescription structure.
Definition of the extension entry interface class.
DetElement::Children children
The array of children.
const Volume & setLimitSet(const Detector &description, const std::string &name) const
Set the limits to the volume. Note: If the name string is empty, the action is ignored.
AlignmentCondition::Object * cond
SensitiveDetector & setEnergyCutoff(double value)
Set energy cut off.
DetElement & setRegion(const Detector &description, const std::string &name, const Volume &volume)
Set the regional attributes to the detector element.
unsigned int key() const
Access hash key of this detector element (Only valid once geometry is closed!)
Handle class to hold the information of a sensitive detector.
DetElement & setLimitSet(const Detector &description, const std::string &name, const Volume &volume)
Set the limits to the detector element.
DetElement parent() const
Access to the detector elements's parent.
DetElementObject Object
Extern accessible definition of the contained element type.
unsigned int typeFlag
Flag to encode detector types.
Main handle class to hold an alignment conditions object.
std::string type() const
Access detector type (structure, tracker, calorimeter, etc.).
Handle class holding a placed volume (also called physical volume)
DetElement clone(int flag) const
Clone (Deep copy) the DetElement structure.
SensitiveDetector & setHitsCollection(const std::string &spec)
Assign the name of the hits collection.
PlacedVolume placement() const
Access to the physical volume of this detector element.
SensitiveDetector & setVerbose(bool value)
Set flag to handle hits collection.
virtual ~Processor()
Default destructor.
Class implementing the ID encoding of the detector response.
bool isValid() const
Check the validity of the object held by the handle.
Handle: a templated class like a shared pointer, which allows specialized access to tgeometry objects...
virtual Region region(const std::string &name) const =0
Retrieve a region object by its name from the detector description.
const Volume & setRegion(const Detector &description, const std::string &name) const
Set the regional attributes to the volume. Note: If the name string is empty, the action is ignored.
void removeAtUpdate(unsigned int type, void *pointer)
Remove callback from object.
DetElement & add(DetElement sub_element)
Add new child to the detector structure.
DetElement & setCombineHits(bool value, SensitiveDetector &sens)
Setter: Combine hits attribute.
DetElement()=default
Default constructor.
VolumeID volumeID() const
The cached VolumeID of this subdetector element.
DetElement & setType(const std::string &typ)
Set detector type (structure, tracker, calorimeter, etc.).
const char * name() const
Access the object name (or "" if not supported by the object)
Data class with properties of a detector element.
void SetName(const char *nam)
Set name (used by Handle)
std::pair< DetElement, Volume > reflect(const std::string &new_name) const
Reflect (Deep copy) the DetElement structure with a new name.
void check(bool condition, const std::string &msg) const
Internal assert function to check conditions.
DetElement & setTypeFlag(unsigned int types)
Set the flag word encoding detector types ( ideally use dd4hep::DetType for encoding )
DetElement parent
Reference to the parent element.
unsigned int typeFlag() const
Access the type of the sensitive detector.
Handle class describing a detector element.
Handle class holding a placed volume (also called physical volume)
void assign(Object *n, const std::string &nam, const std::string &title)
Assign a new named object. Note: object references must be managed by the user.
Volume volume() const
Access to the logical volume of the detector element's placement.
IFACE * extension() const
Access extension element by the type.
Handle class describing a set of limits as they are used for simulation.
const std::string & hitsCollection() const
Access the hits collection name.
DetElement & setAttributes(const Detector &description, const Volume &volume, const std::string ®ion, const std::string &limits, const std::string &vis)
Set all attributes in one go.
Definition of the generic callback structure for member functions.
int level
Hierarchical level within the detector description.
void i_addUpdateCall(unsigned int callback_type, const Callback &callback) const
Internal call to extend the detector element with an arbitrary structure accessible by the type.
bool combineHits() const
Access flag to combine hist.
void SetTitle(const char *tit)
Set Title (used by Handle)
const std::string & placementPath() const
Access to the full path to the placed object.
void removeAtUpdate(unsigned int type, void *pointer) const
Remove callback from object.
Handle class describing a region as used in simulation.
Main handle class to hold an alignment object.
Alignment nominal() const
Access to the constant ideal (nominal) alignment information.
DetElementObject * m_element
Single and only data member: Reference to the actual element.
SensitiveDetector & setReadout(Readout readout)
Assign the IDDescriptor reference.
virtual LimitSet limitSet(const std::string &name) const =0
Retrieve a limitset by its name from the detector description.
PlacedVolume placement
The subdetector placement corresponding to the actual detector element's volume.
int level() const
Access the hierarchical level of the detector element (Only valid once geometry is closed!...
bool combineHits() const
Getter: Combine hits attribute.
SensitiveDetector()
Default constructor.
DetElement & setPlacement(const PlacedVolume &volume)
Set the physical volumes of the detector element.
const char * GetTitle() const
Get name (used by Handle)
LimitSet limits() const
Access to the limit set of the sensitive detector (not mandatory).
void * addExtension(unsigned long long int key, ExtensionEntry *entry) const
Add an extension object to the detector element.
std::string type() const
Access the type of the sensitive detector.
int id
Unique integer identifier of the detector instance.
std::pair< DetElement, Volume > reflect(const std::string &new_name, int new_id, SensitiveDetector sd)
Reflect all volumes in a DetElement sub-tree and re-attach the placements.
Region region() const
Access to the region setting of the sensitive detector (not mandatory)
bool verbose() const
Access flag to combine hist.
Solid solid() const
Access to the shape of the detector element's placement.
UpdateCallbacks updateCalls
Placeholder for structure with update callbacks.
dd4hep::DDSegmentation::VolumeID VolumeID
std::map< std::string, DetElement > Children
DetElementObject * access() const
Checked object access. Throws invalid handle runtime exception if invalid handle.
DetElementObject * ptr() const
Access to the held object.
PlacedVolume idealPlacement() const
Access to the ideal physical volume of this detector element.
void * addExtension(ExtensionEntry *entry) const
Add an extension object to the detector element.
DetElement & setVisAttributes(const Detector &description, const std::string &name, const Volume &volume)
Set Visualization attributes to the detector element.
double energyCutoff() const
Access energy cut off.
const Volume & setVisAttributes(const VisAttr &obj) const
Set Visualization attributes to the volume.
Namespace for the AIDA detector description toolkit.
DetElement child(const std::string &name) const
Access to individual children by name.
Readout readout() const
Access readout structure of the sensitive detector.
Volume volume() const
Logical volume of this placement.
int combineHits
Flag to process hits.
The main interface to the dd4hep detector description package.
Handle to the implementation of the readout structure of a subdetector.
std::string name
The object name.
int id() const
Get the detector identifier.
IDDescriptor idSpec() const
Access IDDescription structure.
SensitiveDetector & setRegion(Region reg)
Set the regional attributes to the sensitive detector.
SensitiveDetector & setCombineHits(bool value)
Set flag to handle hits collection.
virtual DetElementObject * clone(int new_id, int flag) const
Deep object copy to replicate DetElement trees e.g. for reflection.
SensitiveDetector & setLimitSet(LimitSet limits)
Set the limits to the sensitive detector.
DetElement world() const
Access to the world object. Only possible once the geometry is closed.
Alignment survey() const
Access to the constant survey alignment information.
unsigned int key
Access hash key of this detector element (Only valid once geometry is closed!)
IFACE * extension() const
Access extension element by the type.
Processor()
Default constructor.
void * addExtension(unsigned long long int key, ExtensionEntry *entry)
Add an extension object to the detector element.
SensitiveDetector & setType(const std::string &typ)
Set detector type (structure, tracker, calorimeter, etc.).
void * extension(unsigned long long int key, bool alert) const
Access an existing extension object from the detector element.
virtual unsigned long long int hash64() const =0
Hash value.
std::string path
Full path to this detector element. May be invalid.
std::string hitsCollection