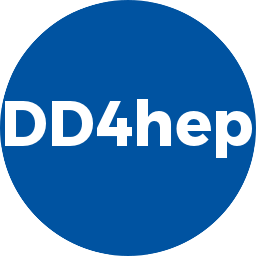 |
DD4hep
1.28.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
38 static Delta identity_delta;
55 Calculator() =
default;
57 ~Calculator() =
default;
59 Result compute(Context& context,
Entry& entry)
const;
61 void resolve(Context& context,
DetElement child)
const;
73 class Calculator::Context {
75 typedef std::map<DetElement,std::size_t,AlignmentsCalculator::PathOrdering> DetectorMap;
76 typedef std::map<unsigned int,std::size_t>
Keys;
77 typedef std::vector<Entry> Entries;
90 if (
det.isValid() ) {
97 except(
"AlignContext",
"Failed to add entry: invalid detector handle!");
106 static PrintLevel s_PRINT = WARNING;
124 Result Calculator::compute(Context& context,
Entry& e)
const {
128 if ( e.valid == 1 ) {
129 printout(DEBUG,
"ComputeAlignment",
"================ IGNORE %s (already valid)",
det.path().c_str());
136 TGeoHMatrix transform_for_delta;
138 printout(DEBUG,
"ComputeAlignment",
139 "============================== Compute transformation of %s",
det.path().c_str());
148 TGeoHMatrix parent_transform;
153 else if (
det.parent().isValid() ) {
160 align.
detectorTrafo =
det.nominal().detectorTransformation() * transform_for_delta;
172 if ( s_PRINT <= INFO ) {
173 printout(INFO,
"ComputeAlignment",
"Level:%d Path:%s DetKey:%08X: Cond:%s key:%16llX",
174 det.level(),
det.path().c_str(),
det.key(),
175 yes_no(e.
delta != 0), (
long long int)
cond.key());
176 if ( s_PRINT <= DEBUG ) {
177 ::printf(
"Nominal: '%s' ",
det.path().c_str());
178 det.nominal().worldTransformation().Print();
179 ::printf(
"Parent: '%s' -> '%s' ",
det.path().c_str(), parent_det.
path().c_str());
180 parent_transform.Print();
181 ::printf(
"DetectorTrafo: '%s' -> '%s' ",
det.path().c_str(),
det.parent().path().c_str());
182 det.nominal().detectorTransformation().Print();
183 ::printf(
"Delta: '%s' ",
det.path().c_str());
184 transform_for_delta.Print();
185 ::printf(
"Result: '%s' ",
det.path().c_str());
193 void Calculator::resolve(Context& context,
DetElement detector)
const {
194 auto children = detector.
children();
195 auto item = context.detectors.find(detector);
196 if ( item == context.detectors.end() ) context.insert(detector,0);
197 for(
const auto& c : children )
198 resolve(context, c.second);
207 Calculator::Context context(alignments);
208 for(
const auto& i : deltas )
209 context.insert(i.first, i.second);
210 for(
const auto& i : deltas )
211 obj.resolve(context,i.first);
212 for(
auto& i : context.entries )
213 result += obj.compute(context, i);
221 Calculator::Context context(alignments);
232 for(
const auto& i : deltas )
233 ordered_deltas.emplace(i.first, &i.second);
234 return compute(ordered_deltas, alignments);
241 Calculator::Context context(alignments);
252 for(
const auto& i : deltas )
253 ordered_deltas.insert(i);
254 return compute(ordered_deltas, alignments);
261 IOV* effective_iov)
const
271 IOV* effective_iov)
const
273 if ( !extract_context.empty() ) {
277 IOV* effective_iov = 0;
280 : delta_conditions(d), extract_context(e), effective_iov(eff_iov) {}
282 virtual int process(
Condition c)
const override {
286 if ( idd != extract_context.end() ) {
289 delta_conditions.emplace(de,&d);
299 DeltaScanner scanner(deltas,extract_context,effective_iov);
301 return deltas.size();
304 for(
const auto& d : deltas ) extract_context.emplace(d.first.key(), d.first);
305 return deltas.size();
311 IOV* effective_iov)
const
321 IOV* effective_iov)
const
324 return deltas.size();
328 static auto s_registry = GrammarRegistry::pre_note<AlignmentsCalculator::OrderedDeltas>(1);
void iov_intersection(const IOV &comparator)
Set the intersection of this IOV with the argument IOV.
const Children & children() const
Access to the list of children.
const std::string & path() const
Path of the detector element (not necessarily identical to placement path!)
AlignmentsCalculator::Result Result
AlignmentCondition::Object * cond
Helper to run DetElement scans.
unsigned int key() const
Access hash key of this detector element (Only valid once geometry is closed!)
virtual ConditionsMap & conditionsMap() const =0
Accessor for the current conditons mapping.
DetElementObject Object
Extern accessible definition of the contained element type.
virtual void scan(const Condition::Processor &processor) const =0
Interface to scan data content of the conditions mapping.
ConditionResolver * resolver
Internal reference to the resolver to access other conditions (Be careful)
Main handle class to hold an alignment conditions object.
std::map< DetElement, const Delta *, PathOrdering > OrderedDeltas
size_t extract_deltas(cond::ConditionUpdateContext &context, OrderedDeltas &deltas, IOV *effective_iov=0) const
Helper: Extract all Delta-conditions from the conditions map.
Condition::key_type hash
Hashed key representation.
GlobalAlignmentStack::StackEntry Entry
cond::ConditionUpdateContext & context
Reference to the user pool taking into account IOV intersections.
DetElement world() const
Access to the top level detector element.
bool isValid() const
Check the validity of the object held by the handle.
TGeoHMatrix * _transform(const Transform3D &trans)
Convert a Transform3D object to a newly created TGeoHMatrix.
Alignment and Delta item key.
static void increment(T *)
Increment count according to type information.
Key definition to optimize ans simplyfy the access to conditions entities.
Helper union to interprete conditions keys.
Scanner to find all alignment deltas in the detector hierarchy.
Class describing an condition to re-adjust an alignment.
struct dd4hep::ConditionKey::KeyMaker::@3 values
Main condition object handle.
Class describing the interval of validty.
Condition condition(const ConditionKey &key_value) const
Access to condition object by dependency key.
Handle class describing a detector element.
int scan(Q &p, DetElement start, int level=0, bool recursive=true) const
Detector element tree scanner using wrapped DetectorProcessor objects.
static const Condition::itemkey_type alignmentKey
Key value of an alignment condition object "alignment".
AlignmentData & data()
Data accessor for the use of decorators.
static const Condition::itemkey_type deltaKey
Key value of a delta condition "alignment_delta".
const TGeoHMatrix & worldTransformation() const
Create cached matrix to transform to world coordinates.
Transform3D trToWorld
Transformation from volume to the world.
Delta delta
Alignment changes.
static void decrement(T *)
Decrement count according to type information.
void computeMatrix(TGeoHMatrix &tr_delta) const
Compute the alignment delta for one detector element and its alignment condition.
Delta delta
Delta transformation to be applied.
Object encapsulating the result of a computation call to the alignments calculator.
Derived condition data-object definition.
Alignment nominal() const
Access to the constant ideal (nominal) alignment information.
int operator()(DetElement de, int) const
Callback to output alignments information.
TGeoHMatrix detectorTrafo
Intermediate buffer to store the transformation to the parent detector element.
T & get()
Generic getter. Specify the exact type, not a polymorph type.
std::map< Condition::key_type, DetElement > ExtractContext
Key key() const
Get the local key of the IOV.
unsigned long long int key_type
Forward definition of the key type.
TGeoHMatrix worldTrafo
Intermediate buffer to store the transformation to the world coordination system.
OrderedDeltas & deltas
Collection container.
Condition::detkey_type det_key
Namespace for the AIDA detector description toolkit.
Result compute(const std::map< DetElement, Delta > &deltas, ConditionsMap &alignments) const
Compute all alignment conditions of the internal dependency list.
Abstract base for processing callbacks to conditions objects.
const IOV & iov() const
Access the IOV block.
ConditionUpdateContext class used by the derived conditions calculation mechanism.
Namespace for implementation details of the AIDA detector description toolkit.
Condition::itemkey_type item_key