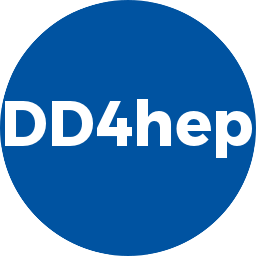 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_OBJECTS_H
14 #define DD4HEP_OBJECTS_H
27 class TGeoTranslation;
28 class TGeoPhysicalNode;
32 #pragma GCC diagnostic push
33 #pragma GCC diagnostic ignored "-Wdeprecated" // Code that causes warning goes here
36 #include <Math/Vector3D.h>
37 #include <Math/Transform3D.h>
38 #include <Math/Translation3D.h>
39 #include <Math/RotationX.h>
40 #include <Math/RotationY.h>
41 #include <Math/RotationZ.h>
42 #include <Math/Rotation3D.h>
43 #include <Math/RotationZYX.h>
44 #include <Math/EulerAngles.h>
45 #include <Math/VectorUtil.h>
46 #include <TGeoElement.h>
47 #include <TGeoMaterial.h>
48 #include <TGeoMedium.h>
49 #include <TGeoPhysicalNode.h>
50 #include <TGDMLMatrix.h>
53 #pragma GCC diagnostic pop
61 #define _USE_MATH_DEFINES
63 #define M_PI 3.14159265358979323846
84 template <
class V> V
RotateX(
const V&
v,
double a) {
87 template <
class V> V
RotateY(
const V&
v,
double a) {
90 template <
class V> V
RotateZ(
const V&
v,
double a) {
170 const std::string
name()
const;
172 void setName(
const std::string& new_name);
174 const std::string
title()
const;
176 void setTitle(
const std::string& new_title);
178 const std::string&
author()
const;
180 void setAuthor(
const std::string& new_author);
182 const std::string&
url()
const;
184 void setUrl(
const std::string& new_url);
186 const std::string&
status()
const;
188 void setStatus(
const std::string& new_status);
190 const std::string&
version()
const;
192 void setVersion(
const std::string& new_version);
194 const std::string&
comment()
const;
196 void setComment(
const std::string& new_comment);
219 Constant(
const std::string&
name,
const std::string& val,
const std::string& typ=
"number");
256 Atom(
const std::string&
name,
const std::string& formula,
int Z,
int N,
double density);
309 std::string
propertyRef(
const std::string&
name,
const std::string& default_value=
"");
371 void setColor(
float alpha,
float red,
float green,
float blue);
374 bool rgb(
float& red,
float& green,
float& blue)
const;
376 bool argb(
float&
alpha,
float& red,
float& green,
float& blue)
const;
427 typedef std::set<Limit>
Set;
448 const std::set<Limit>&
limits()
const;
452 const std::set<Limit>&
cuts()
const;
488 std::vector<std::string>&
limits()
const;
504 #include <Math/Vector4D.h>
505 #include <Math/Point3D.h>
511 return std::sqrt(l.X() * r.X() + l.Y() * r.Y() + l.Z() * r.Z());
515 return 0.5 * (p1.R() + p2.R()) / 2.0;
519 return 0.5 * (p1 + p2);
527 bool operator<(
const XYZPoint& a,
const XYZPoint& b);
529 bool operator<(
const XYZVector& a,
const XYZVector& b);
531 bool operator<(
const PxPyPzEVector& a,
const PxPyPzEVector& b);
534 #endif // DD4HEP_OBJECTS_H
Material & operator=(const Material ©)=default
Assignment operator.
void setAuthorEmail(const std::string &addr)
Set the author's email address.
Author(const Author &e)=default
Constructorto be used for assignment from a handle.
Limit & operator=(const Limit &c)=default
Assignment operator.
Constant & operator=(const Constant ©)=default
Copy assignment.
bool operator==(const Limit &c) const
Equality operator.
Material()=default
Default constructor.
int color() const
Get object color.
const std::set< Limit > & cuts() const
Accessor to limits container.
std::set< Limit >::const_iterator const_iterator
Handle class describing an element in the periodic table.
std::string toString() const
String representation of this object.
bool argb(float &alpha, float &red, float &green, float &blue) const
Get alpha and RGB values of the color (if valid)
Constant(const Constant ©)=default
Constructor to be used for assignment from a handle.
bool wasThresholdSet() const
Access was_threshold_set flag.
Constant(const Handle< Q > &e)
Constructor to be used when reading the already parsed DOM tree.
Author(const Handle< Q > &e)
Constructor to be used when assigning already valid handle.
TGeoElement Object
Extern accessible definition of the contained element type.
std::string particles
Particle the limit should be applied to.
ROOT::Math::XYZVector XYZAngles
Property property(const char *name) const
Access to tabular properties of the material.
Author()=default
Default constructor.
const TGDMLMatrix * Property
std::string toString() const
String representation of this object.
Region & setStoreSecondaries(bool value)
Set flag to store secondaries.
Handle class describing visualization attributes.
bool storeSecondaries() const
Access secondaries flag.
Region & setCut(double value)
Set default production cut.
Region()=default
Default constructor.
const std::set< Limit > & limits() const
Accessor to limits container.
Small object describing a limit structure acting on a particle type.
std::string toString() const
Conversion to a string representation.
Handle class describing an author entity.
void setShowDaughters(bool value)
Set Flag to show/hide daughter elements.
std::string constPropertyRef(const std::string &name, const std::string &default_value="")
Access string property value from the material table.
Atom & operator=(const Atom ©)=default
Assignment operator.
ROOT::Math::Rotation3D Rotation3D
void setLineStyle(int style)
Set line style.
std::string propertyRef(const std::string &name, const std::string &default_value="")
Access string property value from the material table.
Region & operator=(const Region &c)=default
Assignment operator.
ROOT::Math::Polar3DVector PositionPolar
V RotateZ(const V &v, double a)
const char * name() const
Access the object name (or "" if not supported by the object)
std::vector< std::string > & limits() const
Access references to user limits.
LimitSet(const LimitSet &e)=default
Copy constructor for handle.
double intLength() const
Access the interaction length of the underlying material.
double A() const
atomic number of the underlying material
double fraction(Atom atom) const
Access the fraction of an element within the material.
V RotateY(const V &v, double a)
float alpha() const
Get alpha value.
VisAttr(const VisAttr &e)=default
Copy constructor for handle.
Region(const Region &e)=default
Copy Constructor.
double operator*(const dd4hep::Position &l, const dd4hep::Position &r)
Dot product of 3-vectors.
Region & setCut(double value, const std::string &particle)
Set particle type specific cut (Geant4 accepts e+, e-, gamma, and proton)
double density() const
density of the underlying material
std::string dataType() const
Access the constant.
int lineStyle() const
Get line style.
Handle class describing a material.
int drawingStyle() const
Get drawing style.
bool operator==(const Constant &rhs) const
Equality operator.
ROOT::Math::Translation3D Translation3D
bool showDaughters() const
Get Flag to show/hide daughter elements.
void setDrawingStyle(int style)
Set drawing style.
double threshold() const
Access production threshold.
LimitSet & operator=(const LimitSet &c)=default
Assignment operator.
Handle()=default
Default constructor.
Handle class describing a constant (define) object in description.
Author & operator=(const Author &e)=default
Assignment operator.
void setVisible(bool value)
Set visibility flag.
Handle class describing a set of limits as they are used for simulation.
std::string name
Limit name.
void setAuthorName(const std::string &nam)
Set the author's name.
Concrete object implementation of the Region Handle.
double constProperty(const std::string &name) const
Access to tabular properties of the material.
ROOT::Math::RotationZ RotationZ
double Z() const
proton number of the underlying material
std::set< Limit > Set
Iterator definitions.
Handle class describing a region as used in simulation.
bool rgb(float &red, float &green, float &blue) const
Get RGB values of the color (if valid)
Concrete object implementation for the Constant handle.
ConstantObject * m_element
Single and only data member: Reference to the actual element.
std::string content
Content.
Atom(const Atom ©)=default
Copy constructor.
V RotateX(const V &v, double a)
ROOT::Math::RhoZPhiVector PositionRhoZPhi
Material(const Material ©)=default
Copy constructor.
std::string authorName() const
Access the auhor's name.
std::set< Limit >::iterator iterator
IDDescriptor IDDescriptor
double value
Double value.
Atom()=default
Default constructor.
double cut() const
Access cut value.
bool addCut(const Limit &limit)
Add new limit. Returns true if the new limit was added, false if it already existed.
ROOT::Math::Transform3D Transform3D
std::string toString() const
String representation of this object.
Limit(const Limit &c)=default
Copy constructor.
ROOT::Math::XYZVector Position
ROOT::Math::EulerAngles EulerAngles
ROOT::Math::RotationY RotationY
bool operator<(const XYZPoint &a, const XYZPoint &b)
Allow point insertion of a point in maps.
bool visible() const
Get visibility flag.
bool operator<(const Limit &c) const
operator less
bool useDefaultCut() const
Access use_default_cut flag.
Namespace for the AIDA detector description toolkit.
double mean_length(const dd4hep::Position &p1, const dd4hep::Position &p2)
Calculate the mean length of two vectors.
dd4hep::Position mean_direction(const dd4hep::Position &p1, const dd4hep::Position &p2)
Calculate the mean direction of two vectors.
The main interface to the dd4hep detector description package.
Limit()=default
Default constructor.
ROOT::Math::RotationX RotationX
Concrete object implementation of the LimitSet Handle.
double radLength() const
Access the radiation length of the underlying material.
ROOT::Math::RotationZYX RotationZYX
void setColor(float alpha, float red, float green, float blue)
Set object color.
Constant()=default
Default constructor.
Material(Object *e)
Initialization from pointer.
bool addLimit(const Limit &limit)
Add new limit. Returns true if the new limit was added, false if it already existed.
VisAttr & operator=(const VisAttr &attr)=default
Assignment operator.
VisAttr()=default
Default constructor.
Atom(Object *e)
Initialization from pointer.
std::string authorEmail() const
Access the auhor's email address.
LimitSet()=default
Constructor to be used when reading the already parsed DOM tree.
Region & setThreshold(double value)
Set threshold in MeV.