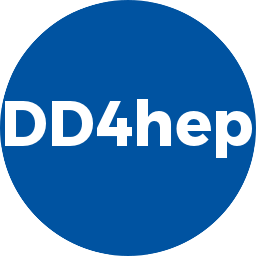 |
DD4hep
1.28.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
24 #include <TGeoMatrix.h>
31 void reset_matrix(TGeoHMatrix* m) {
32 double tr[3] = {0e0,0e0,0e0};
33 double rot[9] = {1e0,0e0,0e0,
36 m->SetTranslation(tr);
62 ReferenceBitMask<AlignmentData::BitMask> mask(a.
flag);
69 for (
size_t i = 0, n=path.size(); n>0 && i < n-1; ++i) {
72 a.
nodes.emplace_back(p);
81 mask.set(AlignmentData::HAVE_PARENT_TRAFO);
82 mask.set(AlignmentData::HAVE_WORLD_TRAFO);
83 mask.set(AlignmentData::IDEAL);
92 const Alignment::NodeList& node_list)
95 ReferenceBitMask<AlignmentData::BitMask> mask(a.
flag);
97 for (
size_t i = a.
nodes.size(); i > 1; --i) {
98 TGeoMatrix* m = a.
nodes[i - 1]->GetMatrix();
102 if ( !a.
nodes.empty() ) {
112 mask.set(AlignmentData::IDEAL);
121 ReferenceBitMask<AlignmentData::BitMask> mask(a.
flag);
129 for (
size_t i = 0, n=path.size(); n>0 && i < n-1; ++i) {
138 mask.set(AlignmentData::SURVEY);
std::vector< PlacedVolume > nodes
The list of TGeoNodes (physical placements)
DetElement parent() const
Access to the detector elements's parent.
Handle class holding a placed volume (also called physical volume)
PlacedVolume placement
The subdetector placement corresponding to the actual detector element's volume.
PlacedVolume placement() const
Access to the physical volume of this detector element.
DetElement detector
Reference to the next hosting detector element.
bool isValid() const
Check the validity of the object held by the handle.
TGeoHMatrix * _transform(const Transform3D &trans)
Convert a Transform3D object to a newly created TGeoHMatrix.
AlignmentData & values()
Accessor to the alignment data. Cannot be NULL. Initialized in the constructor(s)
Data class with properties of a detector element.
Handle< T > & clear()
Release the object held by the handle.
Handle class describing a detector element.
unsigned int magic
Magic word to verify object if necessary.
const TGeoHMatrix & worldTransformation() const
Create cached matrix to transform to world coordinates.
Transform3D trToWorld
Transformation from volume to the world.
Delta delta
Alignment changes.
BitMask flag
Flag to remember internally calculated quatities.
Main handle class to hold an alignment object.
Derived condition data-object definition.
Alignment nominal() const
Access to the constant ideal (nominal) alignment information.
TGeoHMatrix detectorTrafo
Intermediate buffer to store the transformation to the parent detector element.
TGeoHMatrix worldTrafo
Intermediate buffer to store the transformation to the world coordination system.
T * ptr() const
Access to the held object.
Alignment survey() const
Access to the constant survey alignment information.