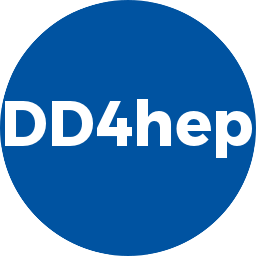 |
DD4hep
1.32.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_ALIGNMENTDATA_H
14 #define DD4HEP_ALIGNMENTDATA_H
22 #include <TGeoMatrix.h>
29 class AlignmentCondition;
158 void localToWorld(
const Double_t local[3], Double_t global[3])
const;
169 void worldToLocal(
const Double_t global[3], Double_t local[3])
const;
174 {
return worldToLocal({global[0],global[1],global[2]}); }
201 #endif // DD4HEP_ALIGNMENTDATA_H
std::vector< PlacedVolume > nodes
The list of TGeoNodes (physical placements)
Alignment nominal() const
Access the ideal/nominal alignment/placement matrix.
Delta(const RotationZYX &rot)
Initializing constructor.
void clear()
Reset information to identity.
Handle class holding a placed volume (also called physical volume)
AlignmentData()
Standard constructor.
PlacedVolume placement
The subdetector placement corresponding to the actual detector element's volume.
DetElement detector
Reference to the next hosting detector element.
Delta(const Position &tr)
Initializing constructor.
void localToDetector(const Position &local, Position &detector) const
Transformation from local coordinates of the placed volume to the detector system.
Position worldToLocal(const Double_t global[3]) const
Transformation from local coordinates of the placed volume to the world system.
bool hasRotation() const
Access flags: Check if the delta operation contains a rotation.
Class describing an condition to re-adjust an alignment.
bool hasPivot() const
Access flags: Check if the delta operation contains a pivot.
Delta()=default
Default constructor.
ROOT::Math::Translation3D Translation3D
void setFlag(unsigned int mask)
Check a given flag.
Handle class describing a detector element.
unsigned int magic
Magic word to verify object if necessary.
Transform3D trToWorld
Transformation from volume to the world.
Delta delta
Alignment changes.
void computeMatrix(TGeoHMatrix &tr_delta) const
Compute the alignment delta for one detector element and its alignment condition.
Delta(const Position &tr, const RotationZYX &rot)
Initializing constructor.
BitMask flag
Flag to remember internally calculated quatities.
Main handle class to hold an alignment object.
Derived condition data-object definition.
const Transform3D & localToWorld() const
Access the currently applied alignment/placement matrix.
TGeoHMatrix detectorTrafo
Intermediate buffer to store the transformation to the parent detector element.
unsigned int BitMask
Forward declaration of the utility bit mask.
ROOT::Math::Transform3D Transform3D
bool hasTranslation() const
Access flags: Check if the delta operation contains a translation.
TGeoHMatrix worldTrafo
Intermediate buffer to store the transformation to the world coordination system.
AlignmentData & operator=(const AlignmentData ©)
Assignment operator necessary due to copy constructor.
AlignmentData & data()
Data accessor for decorator.
ROOT::Math::XYZVector Position
Position localToDetector(const Double_t local[3]) const
Transformation from local coordinates of the placed volume to the world system.
void detectorToLocal(const Position &detector, Position &local) const
Transformation from detector element coordinates to the local placed volume coordinates.
Position detectorToLocal(const Double_t det[3]) const
Transformation from detector element coordinates to the local placed volume coordinates.
Namespace for the AIDA detector description toolkit.
~Delta()
Default destructor.
Position localToWorld(const Double_t local[3]) const
Transformation from local coordinates of the placed volume to the world system.
const TGeoHMatrix & worldTransformation() const
Create cached matrix to transform to world coordinates.
Delta(const Position &tr, const Translation3D &piv, const RotationZYX &rot)
Initializing constructor.
ROOT::Math::RotationZYX RotationZYX
void worldToLocal(const Position &global, Position &local) const
Transformation from world coordinates of the local placed volume coordinates.
Delta(const Translation3D &piv, const RotationZYX &rot)
Initializing constructor.
const TGeoHMatrix & detectorTransformation() const
Access the alignment/placement matrix with respect to the world.
bool checkFlag(unsigned int mask) const
Check a given flag.
virtual ~AlignmentData()
Default destructor.
Delta & operator=(const Delta &c)
Assignment operator.
std::ostream & operator<<(std::ostream &s, const dd4hep::Delta &data)
print alignment delta object