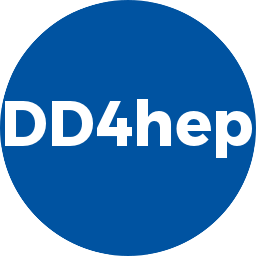 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_FACTORIES_H
14 #define DD4HEP_FACTORIES_H
30 class SensitiveDetector;
31 class SegmentationObject;
45 namespace DDSegmentation {
63 static void*
create(
const char* arg);
201 template <
typename P,
typename S>
class Factory;
255 #define DECLARE_DETELEMENT_FACTORY(x) namespace dd4hep \
256 { DD4HEP_PLUGINSVC_FACTORY(x,x,dd4hep::NamedObject*(dd4hep::Detector*,xml::Handle_t*,Ref_t*),__LINE__) }
257 #define DECLARE_NAMESPACE_DETELEMENT_FACTORY(n,x) namespace dd4hep \
258 { DD4HEP_PLUGINSVC_FACTORY(n::x,x,NamedObject*(dd4hep::Detector*,xml::Handle_t*,Ref_t*),__LINE__) }
259 #define DECLARE_NAMED_APPLY_FACTORY(n,x) namespace dd4hep \
260 { DD4HEP_PLUGINSVC_FACTORY(n::x,x,long(dd4hep::Detector*,int, char**),__LINE__) }
261 #define DECLARE_NAMED_TRANSLATION_FACTORY(n,x) namespace dd4hep \
262 { DD4HEP_PLUGINSVC_FACTORY(n::x,x,dd4hep::NamedObject*(dd4hep::Detector*),__LINE__) }
263 #define DECLARE_NAMED_XMLELEMENT_FACTORY(n,x) namespace dd4hep \
264 { DD4HEP_PLUGINSVC_FACTORY(n::x,x,dd4hep::NamedObject*(dd4hep::Detector*,xml::Handle_t*),__LINE__) }
265 #define DECLARE_NAMED_DETELEMENT_FACTORY(n,x) namespace dd4hep \
266 { DD4HEP_PLUGINSVC_FACTORY(n::x,x,dd4hep::*(),__LINE__) }
269 #define DECLARE_SEGMENTATION(name,func) DD4HEP_OPEN_PLUGIN(dd4hep,name) { \
270 template <> SegmentationObject* \
271 SegmentationFactory<name>::create(const DDSegmentation::BitFieldCoder* d) { return func(d); } \
272 DD4HEP_PLUGINSVC_FACTORY(name,segmentation_constructor__##name, \
273 SegmentationObject*(const DDSegmentation::BitFieldCoder*),__LINE__)}
276 #define DECLARE_CREATE(name,func) DD4HEP_OPEN_PLUGIN(dd4hep,name) { \
277 template <> void* SimpleConstructionFactory<name>::create() { return func(); } \
278 DD4HEP_PLUGINSVC_FACTORY(name,name,void*(),__LINE__)}
281 #define DECLARE_APPLY(name,func) DD4HEP_OPEN_PLUGIN(dd4hep,name) { \
282 template <> long ApplyFactory<name>::create(dd4hep::Detector& l,int n,char** a) {return func(l,n,a);} \
283 DD4HEP_PLUGINSVC_FACTORY(name,name,long(dd4hep::Detector*,int,char**),__LINE__)}
286 #define DECLARE_CONSTRUCTOR(name,func) DD4HEP_OPEN_PLUGIN(dd4hep,name) { \
287 template <> void* ConstructionFactory<name>::create(const char* n) { return func(n);} \
288 DD4HEP_PLUGINSVC_FACTORY(name,name,void*(const char*),__LINE__) }
291 #define DECLARE_DD4HEP_CONSTRUCTOR(name,func) DD4HEP_OPEN_PLUGIN(dd4hep,name) { \
292 template <> void* DetectorConstructionFactory<name>::create(dd4hep::Detector& l, int n,char** a) { return func(l,n,a);} \
293 DD4HEP_PLUGINSVC_FACTORY(name,name,void*(dd4hep::Detector*,int,char**),__LINE__) }
296 #define DECLARE_TRANSLATION(name,func) DD4HEP_OPEN_PLUGIN(dd4hep,name) { \
297 template <> Ref_t TranslationFactory<name>::create(dd4hep::Detector& l) {return func(l);} \
298 DECLARE_NAMED_TRANSLATION_FACTORY(Geometry,name) }
301 #define DECLARE_XMLELEMENT(name,func) DD4HEP_OPEN_PLUGIN(dd4hep,xml_element_##name) {\
302 template <> Ref_t XMLElementFactory<xml_element_##name>::create(dd4hep::Detector& l,ns::xml_h e) {return func(l,e);} \
303 DD4HEP_PLUGINSVC_FACTORY(xml_element_##name,name,NamedObject*(dd4hep::Detector*,ns::xml_h*),__LINE__) }
306 #define DECLARE_XML_SHAPE(name,func) DD4HEP_OPEN_PLUGIN(dd4hep,xml_element_##name) {\
307 template <> Handle<TObject> XMLObjectFactory<xml_element_##name>::create(dd4hep::Detector& l,ns::xml_h e) {return func(l,e);}\
308 DD4HEP_PLUGINSVC_FACTORY(xml_element_##name,name,TObject*(dd4hep::Detector*,ns::xml_h*),__LINE__) }
311 #define DECLARE_XML_VOLUME(name,func) DD4HEP_OPEN_PLUGIN(dd4hep,xml_element_##name) {\
312 template <> Handle<TObject> XMLObjectFactory<xml_element_##name>::create(dd4hep::Detector& l,ns::xml_h e) {return func(l,e);}\
313 DD4HEP_PLUGINSVC_FACTORY(xml_element_##name,name,TObject*(dd4hep::Detector*,ns::xml_h*),__LINE__) }
316 #define DECLARE_XML_DOC_READER(name,func) DD4HEP_OPEN_PLUGIN(dd4hep,xml_document_##name) { \
317 template <> long XMLDocumentReaderFactory<xml_document_##name>::create(dd4hep::Detector& l,ns::xml_h e) {return func(l,e);} \
318 DD4HEP_PLUGINSVC_FACTORY(xml_document_##name,name##_XML_reader,long(dd4hep::Detector*,ns::xml_h*),__LINE__) }
321 #define DECLARE_XML_PLUGIN(name,func) DD4HEP_OPEN_PLUGIN(dd4hep,xml_document_##name) { \
322 template <> long XMLDocumentReaderFactory<xml_document_##name>::create(dd4hep::Detector& l,ns::xml_h e) {return func(l,e);} \
323 DD4HEP_PLUGINSVC_FACTORY(xml_document_##name,name,long(dd4hep::Detector*,ns::xml_h*),__LINE__) }
326 #define DECLARE_XML_PROCESSOR_BASIC(name,func,deprecated) DD4HEP_OPEN_PLUGIN(dd4hep,det_element_##name) {\
327 template <> Ref_t XmlDetElementFactory< det_element_##name >::create(dd4hep::Detector& l,ns::xml_h e,ns::ref_t h) \
328 { if (deprecated) warning_deprecated_xml_factory(#name); return func(l,e,h);} \
329 DD4HEP_PLUGINSVC_FACTORY(det_element_##name,name,NamedObject*(dd4hep::Detector*,ns::xml_h*,Ref_t*),__LINE__) }
332 #define DECLARE_JSON_PROCESSOR_BASIC(name,func) DD4HEP_OPEN_PLUGIN(dd4hep,det_element_##name) { \
333 template <> Ref_t JsonDetElementFactory< det_element_##name >::create(dd4hep::Detector& l,ns::json_h e,ns::ref_t h) \
334 { return func(l,e,h);} \
335 DD4HEP_PLUGINSVC_FACTORY(det_element_##name,name,NamedObject*(dd4hep::Detector*,ns::json_h*,ns::ref_t*),__LINE__) }
337 #define DECLARE_XML_PROCESSOR(name,func) DECLARE_XML_PROCESSOR_BASIC(name,func,0)
338 #define DECLARE_SUBDETECTOR(name,func) DECLARE_XML_PROCESSOR_BASIC(name,func,0)
339 #define DECLARE_DETELEMENT(name,func) DECLARE_XML_PROCESSOR_BASIC(name,func,0)
340 #define DECLARE_DEPRECATED_DETELEMENT(name,func) DECLARE_XML_PROCESSOR_BASIC(name,func,1)
342 #define DECLARE_JSON_DETELEMENT(name,func) DECLARE_JSON_PROCESSOR_BASIC(name,func)
344 #endif // DD4HEP_FACTORIES_H
Template class for a generic dd4hep object constructor.
Helper structure to shortcut type definitions for the factories.
Helper class for decoding and encoding a bit field of 64bits for convenient declaration.
static Handle< TObject > create(Detector &description, xml::Handle_t e)
Standard factory to create Detector elements from an XML representation.
static void * create(const char *arg)
#define DD4HEP_PLUGIN_FACTORY_ARGS_3(R, A0, A1, A2)
Implementation class supporting generic Segmentation of sensitive detectors.
Class to easily access the properties of single XmlElements.
static SegmentationObject * create(const DDSegmentation::BitFieldCoder *decoder)
#define DD4HEP_PLUGIN_FACTORY_ARGS_1(R, A0)
#define DD4HEP_PLUGIN_FACTORY_ARGS_0(R)
dd4hep::json::Handle_t json_h
#define DD4HEP_PLUGIN_FACTORY_ARGS_2(R, A0, A1)
static Handle< NamedObject > create(Detector &description, json::Handle_t e, Handle< NamedObject > sens)
static long create(Detector &description, xml::RefElement &handle, xml::Handle_t element)
Template class with a generic constructor signature.
static Handle< NamedObject > create(Detector &description, xml::Handle_t e)
Namespace for the AIDA detector description toolkit supporting XML utilities.
Factory base class implementing some utilities.
Template class for a generic segmentation object constructor.
Read an arbitrary XML document and analyze its content.
static Handle< NamedObject > create(Detector &description, xml::Handle_t e, Handle< NamedObject > sens)
Standard factory to create ROOT objects from an XML representation.
Standard factory to create Detector elements from the compact XML representation.
Read an arbitrary XML document and analyze its content.
dd4hep::xml::Handle_t xml_h
User abstraction class to manipulate named XML elements (references) within a document.
T * ptr() const
Access to the held object.
Create an arbitrary object from its XML representation.
static void * create(Detector &description, int argc, char **argv)
static long create(Detector &description, int argc, char **argv)
Class of the ROOT toolkit. See http://root.cern.ch/root/htmldoc/ClassIndex.html.
Namespace for the AIDA detector description toolkit.
static long create(Detector &description, xml::Handle_t e)
Implementation of a named object.
Class to easily access the properties of single JsonElements.
The main interface to the dd4hep detector description package.
Template class with a generic signature to apply Detector plugins.
Specialized factory to translate objects, which can be retrieved from Detector.
void handle(const O *o, const C &c, F pmf)
static Handle< NamedObject > create(Detector &description)