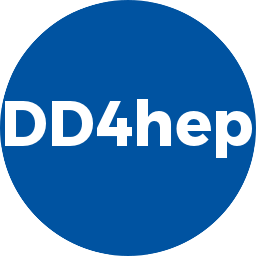 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
40 assign(o, nam,
"alignment");
48 assign(o, nam,
"alignment");
std::vector< PlacedVolume > nodes
The list of TGeoNodes (physical placements)
const TGeoHMatrix & detectorTransformation() const
Access the alignment/placement matrix with respect to the world.
Condition::key_type hash
Hash value of the name.
detail::AlignmentObject Object
Extern accessible definition of the contained element type.
AlignmentData & data()
Data accessor for the use of decorators.
void localToDetector(const Position &local, Position &detector) const
Transformation from local coordinates of the placed volume to the detector system.
const std::vector< PlacedVolume > & nodes() const
Access to the node list.
bool isValid() const
Check the validity of the object held by the handle.
Processor()
Default constructor.
bool is_bound() const
Check if object is already bound....
bool is_bound() const
Check if object is already bound....
const Delta & delta() const
Access the delta value of the object.
AlignmentData & values()
Accessor to the alignment data. Cannot be NULL. Initialized in the constructor(s)
Class describing the interval of validty type.
void localToWorld(const Position &local, Position &global) const
Transformation from local coordinates of the placed volume to the world system.
Class describing an condition to re-adjust an alignment.
void worldToLocal(const Position &global, Position &local) const
Transformation from world coordinates of the local placed volume coordinates.
Class describing the interval of validty.
void assign(Object *n, const std::string &nam, const std::string &title)
Assign a new named object. Note: object references must be managed by the user.
static const Condition::itemkey_type alignmentKey
Key value of an alignment condition object "alignment".
AlignmentData & data()
Data accessor for the use of decorators.
static const Condition::itemkey_type deltaKey
Key value of a delta condition "alignment_delta".
const TGeoHMatrix & detectorTransformation() const
Access the alignment/placement matrix with respect to the world.
static const std::string deltaName
Key name of a delta condition "alignment_delta".
const TGeoHMatrix & worldTransformation() const
Create cached matrix to transform to world coordinates.
const IOVType * iovType() const
Access safely the IOV-type.
static Condition::itemkey_type itemCode(const char *value)
32 bit hashcode of the item
Delta delta
Alignment changes.
static const std::string alignmentName
Key name of an alignment condition object "alignment".
Derived condition data-object definition.
void detectorToLocal(const Position &detector, Position &local) const
Transformation from detector element coordinates to the local placed volume coordinates.
const Transform3D & localToWorld() const
Access the currently applied alignment/placement matrix.
void localToDetector(const Position &local, Position &detector) const
Transformation from local coordinates of the placed volume to the detector system.
unsigned long long int key_type
Forward definition of the key type.
Alignment()=default
Default constructor.
unsigned int itemkey_type
Low part of the key identifies the item identifier.
AlignmentData * alignment_data
Cached pointer to the bound conditions data, since these may be accessed very frequently.
const Delta & delta() const
Access the delta value of the object.
const TGeoHMatrix & worldTransformation() const
Create cached matrix to transform to world coordinates.
const IOV * iovData() const
Access safely the IOV.
ROOT::Math::XYZVector Position
void detectorToLocal(const Position &detector, Position &local) const
Transformation from detector element coordinates to the local placed volume coordinates.
detail::AlignmentObject * access() const
Checked object access. Throws invalid handle runtime exception if invalid handle.
detail::AlignmentObject * ptr() const
Access to the held object.
Namespace for the AIDA detector description toolkit.
OpaqueDataBlock data
Data block.
const TGeoHMatrix & worldTransformation() const
Create cached matrix to transform to world coordinates.
void worldToLocal(const Position &global, Position &local) const
Transformation from world coordinates of the local placed volume coordinates.
const IOV & iov() const
Access the IOV block.
const IOVType & iovType() const
Access the IOV type.
AlignmentCondition()
Default constructor.
const TGeoHMatrix & detectorTransformation() const
Access the alignment/placement matrix with respect to the world.
The data class behind an alignments handle.
key_type key() const
Access the hash identifier.