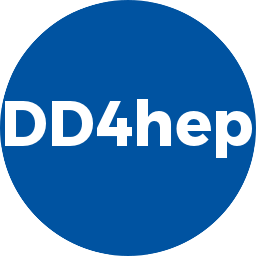 |
DD4hep
1.32.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
17 #include <Parsers/Parsers.h>
44 throw std::runtime_error(
"Attempt to access property grammar from invalid object.");
52 throw std::runtime_error(
"Attempt to access property grammar from invalid object.");
61 throw std::runtime_error(
"Attempt to access property grammar from invalid object.");
70 throw std::runtime_error(
"Attempt to access property grammar from invalid object.");
76 this->set < std::string > (val);
79 throw std::runtime_error(
"Attempt to set invalid string to property!");
112 throw std::runtime_error(
"The property:" + name +
" already exists for this component.");
116 PropertyManager::Properties::const_iterator
121 throw std::runtime_error(
"PropertyManager: Unknown property:" + name);
125 PropertyManager::Properties::iterator
130 throw std::runtime_error(
"PropertyManager: Unknown property:" + name);
162 printout(ALWAYS,
"PropertyManager",
"Property %s = %s",
163 i.first.c_str(), i.second.str().c_str());
192 return os << result.
str();
197 static auto s_registry = GrammarRegistry::pre_note<Property>(1);
void verifyNonExistence(const std::string &name) const
Verify that this property does not exist (throw exception if the name was found)
Property & operator=(const Property &p)=default
Assignment operator.
virtual Property & property(const std::string &name) override
Access single property.
The property class to assign options to actions.
std::string type() const
Property type name.
PropertyConfigurable()
Standard constructor.
std::string str() const
Conversion to string value.
virtual bool hasProperty(const std::string &name) const override
Check property for existence.
Concrete type dependent grammar definition.
virtual std::string str(const void *ptr) const
Serialize an opaque value to a string.
virtual bool fromString(void *ptr, const std::string &value) const
Set value from serialized string. On successful data conversion TRUE is returned.
bool exists(const std::string &name) const
Check for existence.
virtual ~PropertyManager()
Default destructor.
void * m_par
Pointer to the data location.
Base class describing string evaluation to C++ objects using boost::spirit.
void adopt(const PropertyManager ©)
Import properties of another instance.
const Property & property(const std::string &name) const
Access property by name (CONST)
const BasicGrammar * m_hdl
Reference to the grammar of this property (extended type description)
void dump() const
Dump string values.
std::ostream & toStream(const Property &result, std::ostream &os)
int parse(Property &result, const std::string &input)
Property & operator[](const std::string &name)
Access property by name.
virtual ~PropertyConfigurable()
Default destructor.
PropertyManager m_properties
Property pool.
void add(const std::string &name, const Property &property)
Add a new property.
virtual const std::type_info & type() const =0
Access to the type information.
virtual PropertyManager & properties() override
Access to the properties of the object.
const BasicGrammar & grammar() const
Access grammar object.
Manager to ease the handling of groups of properties.
size_t size() const
Access total number of properties.
Namespace for the AIDA detector description toolkit.
Properties::iterator verifyExistence(const std::string &name)
Verify that this property exists (throw exception if the name was not found)
Properties m_properties
Property array/map.
PropertyManager()
Default constructor.