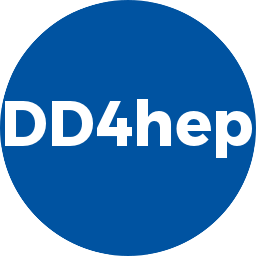 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_DETELEMENT_H
14 #define DD4HEP_DETELEMENT_H
34 class DetElementObject;
35 class SensitiveDetectorObject;
90 std::string
type()
const;
147 void*
extension(
unsigned long long int key,
bool alert)
const;
150 template <
typename IFACE,
typename CONCRETE> IFACE*
addExtension(CONCRETE* c)
const {
151 return (IFACE*) this->
addExtension(detail::typeHash64<IFACE>(),
157 return (IFACE*) this->
extension(detail::typeHash64<IFACE>());
205 typedef std::map<std::string, DetElement>
Children;
227 void check(
bool condition,
const std::string& msg)
const;
267 virtual unsigned long long int hash64()
const override
268 {
return detail::typeHash64<Q>(); }
317 return object<Object>();
343 std::pair<DetElement,Volume>
reflect(
const std::string& new_name)
const;
346 std::pair<DetElement,Volume>
reflect(
const std::string& new_name,
int new_id)
const;
354 void*
extension(
unsigned long long int key,
bool alert)
const;
357 template <
typename IFACE,
typename CONCRETE> IFACE*
addExtension(CONCRETE* c)
const {
363 return (IFACE*) this->
extension(detail::typeHash64<IFACE>(),
true);
366 template <
typename IFACE> IFACE*
extension(
bool alert)
const {
367 return (IFACE*) this->
extension(detail::typeHash64<IFACE>(),alert);
370 template <
typename Q,
typename T>
372 void (T::*pmf)(
unsigned long typ,
DetElement&
det,
void* opt_par))
const
390 std::string
type()
const;
401 unsigned int key()
const;
405 const std::string&
path()
const;
412 const std::string& region,
413 const std::string& limits,
414 const std::string& vis);
452 DetElement child(
const std::string& child_name,
bool throw_if_not_found)
const;
471 #endif // DD4HEP_DETELEMENT_H
const Children & children() const
Access to the list of children.
const std::string & path() const
Path of the detector element (not necessarily identical to placement path!)
IDDescriptor idSpec() const
Access IDDescription structure.
Definition of the extension entry interface class.
void callAtUpdate(unsigned int typ, Q *pointer, void(T::*pmf)(unsigned long typ, DetElement &det, void *opt_par)) const
Extend the detector element with an arbitrary callback.
SensitiveDetector & setEnergyCutoff(double value)
Set energy cut off.
DetElement(NamedObject *obj)
Constructor to hold handled object.
DetElement & setRegion(const Detector &description, const std::string &name, const Volume &volume)
Set the regional attributes to the detector element.
unsigned int key() const
Access hash key of this detector element (Only valid once geometry is closed!)
SensitiveDetector(Object *obj_pointer)
Constructor to copy handled object.
virtual ~DetElementExtension()=default
Default destructor.
Handle class to hold the information of a sensitive detector.
DetElement & setLimitSet(const Detector &description, const std::string &name, const Volume &volume)
Set the limits to the detector element.
DetElement parent() const
Access to the detector elements's parent.
DetElementObject Object
Extern accessible definition of the contained element type.
DetElement & operator=(const DetElement &e)=default
Assignment copy operator.
std::string type() const
Access detector type (structure, tracker, calorimeter, etc.).
Handle class holding a placed volume (also called physical volume)
DetElement clone(int flag) const
Clone (Deep copy) the DetElement structure.
DetElementExtension(T *p)
Typed objects constructor.
SensitiveDetector & setHitsCollection(const std::string &spec)
Assign the name of the hits collection.
PlacedVolume placement() const
Access to the physical volume of this detector element.
SensitiveDetector & setVerbose(bool value)
Set flag to handle hits collection.
virtual ~Processor()
Default destructor.
SensitiveDetector(const Handle< Q > &e)
Templated constructor for handle conversions.
Class implementing the ID encoding of the detector response.
static void checkTypes(const std::type_info &typ1, const std::type_info &typ2, void *test)
Check the compatibility of two typed objects. The test is the result of a dynamic_cast.
DetElement(const Handle< Q > &e)
Templated constructor for handle conversions.
SensitiveDetector(SensitiveDetector &&sd)=default
Move from handle.
Handle: a templated class like a shared pointer, which allows specialized access to tgeometry objects...
DetElementExtension()=delete
Inhibit default constructor.
virtual void destruct() const override
Wrapper for the object destruction.
Base class for Solid (shape) objects.
DetElement & add(DetElement sub_element)
Add new child to the detector structure.
DetElement & setCombineHits(bool value, SensitiveDetector &sens)
Setter: Combine hits attribute.
DetElement()=default
Default constructor.
VolumeID volumeID() const
The cached VolumeID of this subdetector element.
DetElement & setType(const std::string &typ)
Set detector type (structure, tracker, calorimeter, etc.).
const char * name() const
Access the object name (or "" if not supported by the object)
bool operator<(const DetElement e) const
Operator less to insert into a map.
SensitiveDetector(const SensitiveDetector &sd)=default
Copy from handle.
DetElement(Object *obj)
Constructor to hold handled object.
Data class with properties of a detector element.
std::pair< DetElement, Volume > reflect(const std::string &new_name) const
Reflect (Deep copy) the DetElement structure with a new name.
void check(bool condition, const std::string &msg) const
Internal assert function to check conditions.
bool operator==(const Handle< T > &e) const
Equality operator.
DetElement & operator=(DetElement &&sd)=default
Assignment move operator.
SensitiveDetector(Handle< SensitiveDetectorObject > &&sd)
Move from named handle.
DetElement & setTypeFlag(unsigned int types)
Set the flag word encoding detector types ( ideally use dd4hep::DetType for encoding )
unsigned int typeFlag() const
Access the type of the sensitive detector.
Handle class describing a detector element.
bool operator!=(const Handle< T > &e) const
Non-Equality operator.
Handle class holding a placed volume (also called physical volume)
DetElement(const DetElement &e)=default
Constructor to copy handle.
Volume volume() const
Access to the logical volume of the detector element's placement.
IFACE * extension() const
Access extension element by the type.
virtual unsigned long long int hash64() const override
Hash value.
Implementation class for the object extension mechanism.
Handle class describing a set of limits as they are used for simulation.
const std::string & hitsCollection() const
Access the hits collection name.
IFACE * extension(bool alert) const
Access extension element by the type.
DetElement & setAttributes(const Detector &description, const Volume &volume, const std::string ®ion, const std::string &limits, const std::string &vis)
Set all attributes in one go.
Definition of the generic callback structure for member functions.
Object & _data() const
Additional data accessor.
virtual int processElement(DetElement detector)=0
Container callback for object processing.
void i_addUpdateCall(unsigned int callback_type, const Callback &callback) const
Internal call to extend the detector element with an arbitrary structure accessible by the type.
bool operator!=(const Handle< T > &e) const
Non-Equality operator.
bool combineHits() const
Access flag to combine hist.
DetElementExtension(const DetElementExtension ©)=default
Copy constructor.
const std::string & placementPath() const
Access to the full path to the placed object.
void removeAtUpdate(unsigned int type, void *pointer) const
Remove callback from object.
Handle class describing a region as used in simulation.
Main handle class to hold an alignment object.
Alignment nominal() const
Access to the constant ideal (nominal) alignment information.
virtual void * object() const override
Wrapper to access the desired interface.
DetElement(DetElement &&e)=default
Constructor to move handle.
SensitiveDetector & setReadout(Readout readout)
Assign the IDDescriptor reference.
DetElementExtension & operator=(const DetElementExtension ©)=default
Assignment operator.
Abstract base for processing callbacks to DetElement objects.
int level() const
Access the hierarchical level of the detector element (Only valid once geometry is closed!...
IFACE * addExtension(CONCRETE *c) const
Extend the detector element with an arbitrary structure accessible by the type.
bool combineHits() const
Getter: Combine hits attribute.
SensitiveDetector()
Default constructor.
DetElement & setPlacement(const PlacedVolume &volume)
Set the physical volumes of the detector element.
SensitiveDetector(const Handle< SensitiveDetectorObject > &sd)
Copy from named handle.
IFACE * addExtension(CONCRETE *c) const
Extend the sensitive detector element with an arbitrary structure accessible by the type.
LimitSet limits() const
Access to the limit set of the sensitive detector (not mandatory).
void * addExtension(unsigned long long int key, ExtensionEntry *entry) const
Add an extension object to the detector element.
std::string type() const
Access the type of the sensitive detector.
Region region() const
Access to the region setting of the sensitive detector (not mandatory)
bool verbose() const
Access flag to combine hist.
Solid solid() const
Access to the shape of the detector element's placement.
dd4hep::DDSegmentation::VolumeID VolumeID
std::map< std::string, DetElement > Children
Wrapper class for detector element extension objects.
SensitiveDetectorObject * ptr() const
Access to the held object.
virtual ExtensionEntry * clone(void *det) const override
Copy/clone the object.
PlacedVolume idealPlacement() const
Access to the ideal physical volume of this detector element.
void * addExtension(ExtensionEntry *entry) const
Add an extension object to the detector element.
DetElement & setVisAttributes(const Detector &description, const std::string &name, const Volume &volume)
Set Visualization attributes to the detector element.
Data class with properties of sensitive detectors.
double energyCutoff() const
Access energy cut off.
Namespace for the AIDA detector description toolkit.
Implementation of a named object.
SensitiveDetector & operator=(const SensitiveDetector &sd)=default
Assignment copy operator.
DetElement child(const std::string &name) const
Access to individual children by name.
Readout readout() const
Access readout structure of the sensitive detector.
SensitiveDetector & operator=(SensitiveDetector &&sd)=default
Assignment move operator.
The main interface to the dd4hep detector description package.
Handle to the implementation of the readout structure of a subdetector.
bool operator==(const Handle< T > &e) const
Equality operator.
int id() const
Get the detector identifier.
SensitiveDetector & setRegion(Region reg)
Set the regional attributes to the sensitive detector.
SensitiveDetector & setCombineHits(bool value)
Set flag to handle hits collection.
SensitiveDetector & setLimitSet(LimitSet limits)
Set the limits to the sensitive detector.
DetElement world() const
Access to the world object. Only possible once the geometry is closed.
Alignment survey() const
Access to the constant survey alignment information.
IFACE * extension() const
Access extension element by the type.
Processor()
Default constructor.
virtual void * copy(void *det) const override
Copy/clone the object.
SensitiveDetector & setType(const std::string &typ)
Set detector type (structure, tracker, calorimeter, etc.).
T * copy(DetElement de) const
This one ensures we have the correct signatures.