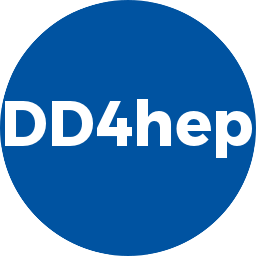 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
57 std::string
str()
const;
70 explicit IOV() =
delete;
74 using Key = std::pair<Key_value_type, Key_value_type>;
109 std::string
str()
const;
117 void set(
const Key& value);
154 {
return key.first >= test.first &&
key.second <= test.second; }
157 {
return key.first <= test.first &&
key.second >= test.second; }
160 {
return key.first <= test.second &&
key.first >= test.first; }
163 {
return key.second >= test.first &&
key.second <= test.second; }
168 (test.first <=
key.first &&
key.second >= test.second) ||
169 (test.first <=
key.first &&
key.first <= test.second) ||
170 (test.first <=
key.second &&
key.second <= test.second);
182 if (
type > test.
type )
return false;
189 #endif // DD4HEP_IOV_H
void iov_intersection(const IOV &comparator)
Set the intersection of this IOV with the argument IOV.
IOVType & operator=(IOVType &©)=default
Move assignment operator.
bool contains(const IOV &iov) const
Check for validity containment.
std::string name
String name.
static bool key_partially_contained(const Key &key, const Key &test)
Check if IOV 'test' has an overlap on the upper interval edge with IOV 'key'.
int optData
Optional user data.
IOV(IOV &©)=default
Move constructor.
~IOV()=default
Standard Destructor.
IOV & invert()
Invert the key values (first=second and second=first)
std::int64_t Key_value_type
Key definition. Use fixed width type, though not portable!
static constexpr unsigned int UNKNOWN_IOV
static IOV forever(const IOVType *typ)
Conditions key representing eternity.
std::string str() const
Conversion to string.
std::string str() const
Create string representation of the IOV.
std::pair< Key_value_type, Key_value_type > Key
IOVType(const IOVType ©)=default
Copy constructor.
static Key key_forever()
Conditions key representing eternity.
Class describing the interval of validty type.
IOV()=delete
Initializing constructor: Does not set reference to IOVType !
void set(Key_value_type val_1, Key_value_type val_2)
Set range IOV value.
Class describing the interval of validty.
void move(IOV &from)
Move the data content: 'from' will be reset to NULL.
IOVType & operator=(const IOVType ©)=default
Assignment operator.
void set(const Key &value)
Set discrete IOV value.
bool is_discrete() const
Check if the IOV corresponds to a range.
static bool key_overlaps_lower_end(const Key &key, const Key &test)
Check if IOV 'test' has an overlap on the lower interval edge with IOV 'key'.
Key keyData
IOV key (if second==first, discrete, otherwise range)
static bool key_overlaps_higher_end(const Key &key, const Key &test)
Check if IOV 'test' has an overlap on the upper interval edge with IOV 'key'.
bool has_range() const
Check if the IOV corresponds to a range.
static bool key_is_contained(const Key &key, const Key &test)
Check if IOV 'test' is fully contained in IOV 'key'.
static bool partial_match(const IOV &iov, const IOV &test)
Check if IOV 'test' is of same type and is at least partially contained in iov.
Key key() const
Get the local key of the IOV.
IOVType(IOVType &©)=default
Move constructor.
unsigned int type
IOV buffer type: Must be a bitmap!
IOVType()=default
Standard Constructor.
static constexpr Key_value_type MIN_KEY
~IOVType()=default
Standard Destructor.
void iov_union(const IOV &comparator)
Set the union of this IOV with the argument IOV.
IOV & operator=(const IOV &c)=default
Assignment operator.
bool operator<(const IOV &test) const
Allow for IOV sorting in maps.
static bool full_match(const IOV &iov, const IOV &test)
Check if IOV 'test' is of same type and is fully contained in iov.
IOV & reset()
Set keys to unphysical values (LONG_MAX, LONG_MIN)
const IOVType * iovType
Reference to IOV type.
Namespace for the AIDA detector description toolkit.
IOV(const IOV ©)=default
Copy constructor.
IOV & operator=(IOV &&c)=default
Move assignment operator.
static constexpr Key_value_type MAX_KEY
unsigned int type
integer identifier used internally
static bool key_contains_range(const Key &key, const Key &test)
Same as above, but reverse logic. Gives sometimes more understandable logic.
static bool same_type(const IOV &iov, const IOV &test)
Check if 2 IOV objects are of the same type.
void set(Key_value_type value)
Set discrete IOV value.