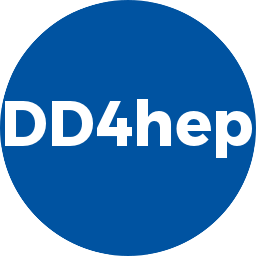 |
DD4hep
1.28.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
14 #include <DD4hep/detail/Handle.inl>
17 #include <Evaluator/Evaluator.h>
26 #if !defined(WIN32) && !defined(__ICC)
43 static bool s_allow_variable_redefine =
true;
46 void check_evaluation(
const std::string& value, std::pair<int,double> res, std::stringstream& err) {
47 if ( res.first != tools::Evaluator::OK) {
48 throw std::runtime_error(
"dd4hep: "+err.str()+
" : value="+value+
" [Evaluation error]");
57 bool tmp = s_allow_variable_redefine;
58 s_allow_variable_redefine = value;
63 std::stringstream err;
64 auto result =
eval.evaluate(value, err);
65 check_evaluation(value, result, err);
69 std::pair<int, double>
_toInteger(
const std::string& value) {
71 size_t idx = s.find(
"(int)");
72 if (idx != std::string::npos)
74 idx = s.find(
"(long)");
75 if (idx != std::string::npos)
86 unsigned short _toUShort(
const std::string& value) {
87 return (
unsigned short)
_toInteger(value).second;
90 int _toInt(
const std::string& value) {
94 unsigned int _toUInt(
const std::string& value) {
95 return (
unsigned int)
_toInteger(value).second;
103 return (
unsigned long)
_toInteger(value).second;
107 return value ==
"true" || value ==
"yes" || value ==
"True";
121 template <
typename T> T
_toType(
const std::string& value) {
122 notImplemented(
"Value "+value+
" cannot be converted to type "+typeName(
typeid(T)));
138 return (
unsigned short)
_toShort(value);
148 return (
unsigned int)
_toInt(value);
158 return (
unsigned long)
_toLong(value);
172 template <> std::string _toType<std::string>(
const std::string& value) {
177 double val =
_toDouble(left +
"*" + right);
178 if ( val >=
double(SCHAR_MIN) && val <=
double(SCHAR_MAX) )
179 return (
char) (int)val;
180 except(
"_multiply<char>",
181 "Multiplication %e = %s * %s out of bounds for conversion to char.",
182 val, left.c_str(), right.c_str());
187 double val =
_toDouble(left +
"*" + right);
188 if ( val >= 0 && val <=
double(UCHAR_MAX) )
189 return (
unsigned char) (int)val;
190 except(
"_multiply<unsigned char>",
191 "Multiplication %e = %s * %s out of bounds for conversion to unsigned char.",
192 val, left.c_str(), right.c_str());
197 double val =
_toDouble(left +
"*" + right);
198 if ( val >=
double(SHRT_MIN) && val <=
double(SHRT_MAX) )
200 except(
"_multiply<short>",
201 "Multiplication %e = %s * %s out of bounds for conversion to short.",
202 val, left.c_str(), right.c_str());
207 double val =
_toDouble(left +
"*" + right);
208 if ( val >= 0 && val <=
double(USHRT_MAX) )
209 return (
unsigned short)val;
210 except(
"_multiply<unsigned short>",
211 "Multiplication %e = %s * %s out of bounds for conversion to unsigned short.",
212 val, left.c_str(), right.c_str());
216 template <>
int _multiply<int>(
const std::string& left,
const std::string& right) {
217 return (
int)
_toDouble(left +
"*" + right);
221 return (
unsigned int)
_toDouble(left +
"*" + right);
225 return (
long)
_toDouble(left +
"*" + right);
229 return (
unsigned long)
_toDouble(left +
"*" + right);
233 return _toFloat(left +
"*" + right);
245 void _toDictionary(
const std::string& name,
const std::string& value,
const std::string& typ) {
246 if ( typ ==
"string" ) {
247 eval.setEnviron(name.c_str(),value.c_str());
252 std::stringstream err;
253 std::string n = name,
v = value;
254 size_t idx =
v.find(
"(int)");
255 if (idx != std::string::npos)
257 idx =
v.find(
"(float)");
258 if (idx != std::string::npos)
262 auto result =
eval.evaluate(
v, err);
263 check_evaluation(
v, result, err);
265 status =
eval.setVariable(n, result.second, err);
266 if ( status != tools::Evaluator::OK ) {
267 std::stringstream err_msg;
268 err_msg <<
"name=" << name <<
" value=" << value
269 <<
" " << err.str() <<
" [setVariable error]";
270 if ( status == tools::Evaluator::WARNING_EXISTING_VARIABLE ) {
271 if ( s_allow_variable_redefine )
272 printout(WARNING,
"Evaluator",
"+++ Overwriting variable: "+err_msg.str());
274 except(
"Evaluator",
"+++ Overwriting variable: "+err_msg.str());
283 size_t current_index = 0;
284 std::stringstream processed_variable;
288 size_t id1 = env.find(
"${", current_index);
290 if (id1 == std::string::npos) {
293 processed_variable << env.substr(current_index);
296 size_t id2 = env.find(
"}", id1);
297 if (id2 == std::string::npos) {
298 std::runtime_error(
"dd4hep: Syntax error, bad variable syntax: " + env);
300 processed_variable << env.substr(current_index, id1 -current_index );
301 std::string
v = env.substr(id1, id2-id1+1);
302 std::stringstream err;
303 auto ret =
eval.getEnviron(
v, err);
305 if ( ret.first != tools::Evaluator::OK) {
306 std::cerr <<
v <<
": " << err.str() << std::endl;
307 throw std::runtime_error(
"dd4hep: Severe error during environment lookup of " +
v +
" " + err.str());
310 processed_variable << ret.second;
311 current_index = id2 + 1;
313 return processed_variable.str();
319 value.reserve(
v.length()+1);
320 for(
const char* p =
v.c_str(); *p; ++p) {
321 if ( !::isspace(*p) ) value += *p;
326 template <
typename T>
static inline std::string __to_string(T value,
const char* fmt) {
328 ::snprintf(text,
sizeof(text), fmt, value);
333 return value ?
"true" :
"false";
337 return __to_string((
int)value, fmt);
341 return __to_string(value, fmt);
344 std::string
_toString(
unsigned long value,
const char* fmt) {
345 return __to_string(value, fmt);
349 return __to_string(value, fmt);
353 return __to_string(value, fmt);
357 return __to_string(value, fmt);
361 static long s_numVerifies = 0;
364 return s_numVerifies;
370 const char* edge =
"++++++++++++++++++++++++++++++++++++++++++";
371 size_t len = std::strlen(name);
372 std::cerr << edge << edge << edge << std::endl;
373 std::cerr <<
"++ The usage of the factory: \"" << name <<
"\" is DEPRECATED due to naming conventions."
374 << std::setw(53-len) << std::right <<
"++" << std::endl;
375 std::cerr <<
"++ Please use \"DD4hep_" << name <<
"\" instead." << std::setw(93-len) << std::right <<
"++" << std::endl;
376 std::cerr << edge << edge << edge << std::endl;
388 return this->m_element ? this->m_element->name().c_str() :
"";
404 #include <TGeoMedium.h>
405 #include <TGeoMaterial.h>
406 #include <TGeoElement.h>
411 #include <TGeoMatrix.h>
419 #include <TGeoNode.h>
428 #include <TGeoBBox.h>
429 #include <TGeoPcon.h>
430 #include <TGeoPgon.h>
431 #include <TGeoTube.h>
432 #include <TGeoCone.h>
433 #include <TGeoArb8.h>
434 #include <TGeoTrd1.h>
435 #include <TGeoTrd2.h>
436 #include <TGeoParaboloid.h>
437 #include <TGeoSphere.h>
438 #include <TGeoTorus.h>
439 #include <TGeoTessellated.h>
440 #include <TGeoBoolNode.h>
441 #include <TGeoVolume.h>
442 #include <TGeoScaledShape.h>
443 #include <TGeoCompositeShape.h>
444 #include <TGeoShapeAssembly.h>
483 #include <TGeoPhysicalNode.h>
486 #include <TGeoBoolNode.h>
492 #include <TGeoPatternFinder.h>
bool _toType< bool >(const std::string &value)
Generic type conversion from string to primitive value.
bool set_allow_variable_redefine(bool value)
Steer redefinition of variable re-definition during expression evaluation. returns old value.
short _toShort(const std::string &value)
String conversions: string to short value.
bool _toBool(const std::string &value)
String conversions: string to boolean value.
long _toType< long >(const std::string &value)
Generic type conversion from string to primitive value.
void warning_deprecated_xml_factory(const char *name)
Function tp print warning about deprecated factory usage. Used by Plugin mechanism.
void increment_object_validations()
void _toDictionary(const std::string &name, const std::string &value)
Enter name value pair to the dictionary. "value" must be a numerical expression, which is evaluated.
unsigned int _multiply< unsigned int >(const std::string &left, const std::string &right)
Generic multiplication using the evaluator: result = left * right.
DDSegmentation::Segmentation _Segmentation
Handle: a templated class like a shared pointer, which allows specialized access to tgeometry objects...
std::string _toString(bool value)
String conversions: boolean value to string.
short _multiply< short >(const std::string &left, const std::string &right)
Generic multiplication using the evaluator: result = left * right.
int _toInt(const std::string &value)
String conversions: string to integer value.
unsigned long _multiply< unsigned long >(const std::string &left, const std::string &right)
Generic multiplication using the evaluator: result = left * right.
const char * name() const
Access the object name (or "" if not supported by the object)
int _multiply< int >(const std::string &left, const std::string &right)
Generic multiplication using the evaluator: result = left * right.
unsigned long _toULong(const std::string &value)
String conversions: string to long integer value.
long _toLong(const std::string &value)
String conversions: string to long integer value.
unsigned short _toUShort(const std::string &value)
String conversions: string to unsigned short value.
unsigned short _toType< unsigned short >(const std::string &value)
Generic type conversion from string to primitive value.
Concrete object implementation for the Header handle.
float _multiply< float >(const std::string &left, const std::string &right)
Generic multiplication using the evaluator: result = left * right.
std::string remove_whitespace(const std::string &v)
String manipulations: Remove unconditionally all white spaces.
const dd4hep::tools::Evaluator & evaluator()
DD4HEP_INSTANTIATE_SHAPE_HANDLE(TGeoCone)
void assign(Object *n, const std::string &nam, const std::string &title)
Assign a new named object. Note: object references must be managed by the user.
std::pair< int, double > _toInteger(const std::string &value)
unsigned short _multiply< unsigned short >(const std::string &left, const std::string &right)
Generic multiplication using the evaluator: result = left * right.
unsigned char _multiply< unsigned char >(const std::string &left, const std::string &right)
Generic multiplication using the evaluator: result = left * right.
long _multiply< long >(const std::string &left, const std::string &right)
Generic multiplication using the evaluator: result = left * right.
int _toType< int >(const std::string &value)
Generic type conversion from string to primitive value.
Class of the ROOT toolkit. See http://root.cern.ch/root/htmldoc/ClassIndex.html.
std::string _ptrToString(const void *p, const char *fmt="%p")
Pointer to text conversion.
DD4HEP_INSTANTIATE_HANDLE_CODE(RAW, TObject, NamedObject)
short _toType< short >(const std::string &value)
Generic type conversion from string to primitive value.
std::pair< int, double > _toFloatingPoint(const std::string &value)
std::string _getEnviron(const std::string &env)
Evaluate string constant using environment stored in the evaluator.
long num_object_validations()
char _multiply< char >(const std::string &left, const std::string &right)
Generic multiplication using the evaluator: result = left * right.
DD4HEP_INSTANTIATE_HANDLE(TGeoElement)
float _toType< float >(const std::string &value)
Generic type conversion from string to primitive value.
float _toFloat(const std::string &value)
String conversions: string to float value.
double _toDouble(const std::string &value)
String conversions: string to double value.
double _multiply< double >(const std::string &left, const std::string &right)
Generic multiplication using the evaluator: result = left * right.
Class of the ROOT toolkit. See http://root.cern.ch/root/htmldoc/ClassIndex.html.
Namespace for the AIDA detector description toolkit.
Implementation of a named object.
T _toType(const std::string &value)
Generic type conversion from string to primitive value.
DD4HEP_INSTANTIATE_HANDLE_UNNAMED(Detector)
unsigned long _toType< unsigned long >(const std::string &value)
Generic type conversion from string to primitive value.
The main interface to the dd4hep detector description package.
Class of the ROOT toolkit. See http://root.cern.ch/root/htmldoc/ClassIndex.html.
virtual void setName(const std::string &value)
Set the segmentation name.
unsigned int _toType< unsigned int >(const std::string &value)
Generic type conversion from string to primitive value.
Base class for all segmentations.
unsigned int _toUInt(const std::string &value)
String conversions: string to unsigned integer value.
double _toType< double >(const std::string &value)
Generic type conversion from string to primitive value.