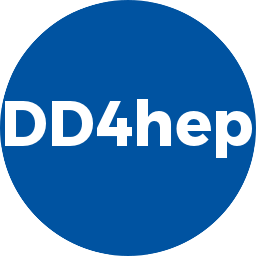 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_CONDITIONS_H
14 #define DD4HEP_CONDITIONS_H
34 class ConditionObject;
131 virtual int operator()(
const std::pair<Condition::key_type,Condition>& e)
const
132 {
return this->
process(e.second); }
178 #if defined(DD4HEP_CONDITIONS_DEBUG) || !defined(DD4HEP_MINIMAL_CONDITIONS)
179 const std::string&
type()
const;
182 const std::string&
value()
const;
184 const std::string&
comment()
const;
186 const std::string&
address()
const;
201 const std::type_info&
typeInfo()
const;
211 template <
typename T,
typename... Args> T&
construct(Args... args);
217 template <
typename T> T&
bind();
223 template <
typename T> T&
bind(
const std::string& val);
225 template <
typename T> T&
get();
227 template <
typename T>
const T&
get()
const;
229 template <
typename T> T&
as();
231 template <
typename T>
const T&
as()
const;
282 #if defined(DD4HEP_CONDITIONS_HAVE_NAME)
313 this->
values.item_key = item;
397 {
return hash == compare; }
405 {
return hash < compare; }
439 {
return (*
this)(
cond.second); }
443 {
return (*
this)(
cond.second.ptr()); }
446 virtual size_t size()
const {
return 0; }
540 #endif // DD4HEP_CONDITIONS_H
int dataType() const
Access the data type.
std::vector< Condition > RangeConditions
const std::string & value() const
Access the value field of the condition as a string.
static Condition::key_type hashCode(DetElement detector, const char *value)
Hash code generation from input string.
virtual ~ConditionsSelect()
Default destructor.
ConditionsSelect()=default
Default constructor.
int have_condition_item_inventory(int value=-1)
Setup conditions item name inventory for debugging.
AlignmentCondition::Object * cond
bool operator==(const ConditionsSelectWrapper &compare)=delete
Default assignment operator.
const std::type_info & typeInfo() const
Access to the type information.
mask_type flags() const
Flag operations: Get condition flags.
ConditionKey & operator=(const ConditionKey &key)=default
Assignment operator from object copy.
detail::ConditionObject Object
Extern accessible definition of the contained element type.
bool testFlag(mask_type option) const
Flag operations: Test for a given a conditons flag.
ConditionsSelect & operator=(const ConditionsSelect ©)=default
Default assignment operator.
const std::string & comment() const
Access the comment field of the condition.
Processor & operator=(const Processor ©)=delete
Assignment operator.
Processor()=default
Default constructor.
T & construct(Args... args)
Construct conditions object and bind the data.
Condition::key_type hash
Hashed key representation.
Condition(Object *p)
Initializing constructor.
ConditionKey()=default
Default constructor.
Class describing an opaque conditions data block.
bool isValid() const
Check the validity of the object held by the handle.
Handle: a templated class like a shared pointer, which allows specialized access to tgeometry objects...
key_type key() const
Hash identifier.
OBJECT & object
Reference to the infomation collector.
KeyMaker(Condition::detkey_type det, Condition::itemkey_type item)
std::string toString() const
Conversion to string.
Key definition to optimize ans simplyfy the access to conditions entities.
bool operator()(std::pair< Condition::key_type, Condition::Object * > cond) const
Selection callback: return true if the condition should be selected.
const char * name() const
Access the object name (or "" if not supported by the object)
Helper union to interprete conditions keys.
bool is_bound() const
Check if object is already bound....
Conditions selector functor. Default implementation selects everything evaluated.
Class describing the interval of validty type.
virtual ~ConditionsSelectWrapper()=default
Default destructor.
ConditionsSelectWrapper()=delete
Default constructor.
detkey_type detector_key() const
DetElement part of the identifier.
ConditionsSelectWrapper(OBJECT &o)
Default constructor.
void * bind(const BasicGrammar *grammar)
Bind data value.
unsigned int detkey_type
High part of the key identifies the detector element.
Main condition object handle.
ConditionsSelectWrapper(const ConditionsSelectWrapper ©)=default
Copy constructor.
ConditionsSelectWrapper & operator=(const ConditionsSelectWrapper ©)=default
Default assignment operator.
ConditionKey(const ConditionKey &c)=default
Copy constructor.
Class describing the interval of validty.
static int haveInventory(int value=-1)
Allow to trace condition names from keys for debugging.
Handle class describing a detector element.
T & as()
Generic getter. Resolves polymorph types. It is mandatory that the datatype is polymorph!
Processor(const Processor ©)=default
Copy constructor.
virtual size_t size() const
Overloadable entry: Return number of conditions selected. Default does nothing....
Condition(const Condition &c)=default
Copy constructor.
Base class describing string evaluation to C++ objects using boost::spirit.
static Condition::itemkey_type itemCode(const char *value)
32 bit hashcode of the item
bool operator==(const ConditionKey &k) const
T & as()
Generic getter. Resolves polymorph types. It is mandatory that the datatype is polymorph!
itemkey_type item_key() const
Item part of the identifier.
virtual ~Processor()=default
Default destructor.
HashCompare(Condition::key_type k)
static constexpr unsigned long long int FIRST_KEY
Flags to indicate global conditions ranges.
T & get()
Generic getter. Specify the exact type, not a polymorph type.
T & bind()
Bind the data of the conditions object to a given format.
std::string str(int with_data=WITH_IOV|WITH_ADDRESS|WITH_DATATYPE) const
Output method.
T & get()
Generic getter. Specify the exact type, not a polymorph type.
virtual int operator()(Condition c) const
Conditions callback for object processing.
bool operator()(Condition cond) const
Selection callback: return true if the condition should be selected.
unsigned int itemkey_type
Low part of the key identifies the item identifier.
void unFlag(mask_type option)
Flag operations: UN-Set a conditons flag.
unsigned long long int key_type
Forward definition of the key type.
unsigned int mask_type
Forward definition of the object properties.
const std::string & type() const
Access the type field of the condition.
ConditionState
Flags to indicate the conditions type ans state.
ConditionDetectorRangeKeys
Flags to indicate conditions detector ranges (high word of the conditions key)
The data class behind a conditions handle.
void setFlag(mask_type option)
Flag operations: Set a conditons flag.
Processor(Processor &©)=default
Move constructor.
KeyMaker(Condition::key_type k)
ConditionsSelect(const ConditionsSelect ©)=default
Copy constructor.
bool operator<(const ConditionKey &compare) const
Equality operator using the string representation.
Condition & operator=(const Condition &c)=default
Assignment copy operator.
Condition::detkey_type det_key
bool operator()(std::pair< Condition::key_type, Condition > cond) const
Selection callback: return true if the condition should be selected.
std::string get_condition_item_name(Condition::itemkey_type key)
Resolve key from conditions item name inventory for debugging.
Namespace for the AIDA detector description toolkit.
Condition::itemkey_type item_key() const
Access the detector element part of the key.
Compare keys by the hash value.
Condition & operator=(Condition &&c)=default
Assignment move operator.
Condition()=default
Default constructor.
const IOVType & iovType() const
Access the IOV type.
virtual int operator()(const std::pair< Condition::key_type, Condition > &e) const
Conditions callback for object processing in maps.
T & construct(Args... args)
Construct conditions object and bind the data.
Condition(Condition &&c)=default
Move constructor.
Conditions selector functor. Wraps a user defined object by reference.
static constexpr unsigned long long int LAST_KEY
struct dd4hep::ConditionKey::KeyMaker::@2 values
ConditionItemRangeKeys
Flags to indicate conditions item ranges (low word of the conditions key)
ConditionKey(Condition::key_type key)
Constructor from fully qualified key.
const BasicGrammar & descriptor() const
Access to the grammar type.
virtual bool operator()(Condition::Object *cond) const =0
Overloadable entry: Selection callback: return true if the condition should be selected.
Abstract base for processing callbacks to conditions objects.
const std::string & address() const
Access the address string [e.g. database identifier].
bool operator==(const ConditionKey &compare) const
Equality operator using key object.
const IOV & iov() const
Access the IOV block.
StringFlags
Flags to steer the conditions conversion to string.
Condition::detkey_type detector_key() const
Access the detector element part of the key.
bool is_bound() const
Check if object is already bound....
OpaqueDataBlock & data() const
Access the opaque data block.
Condition::itemkey_type item_key
virtual int process(Condition c) const =0
Processing callback.