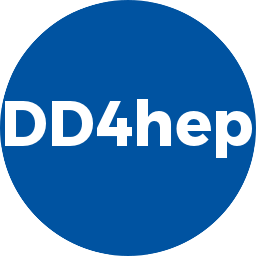 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_ALIGNMENTS_H
14 #define DD4HEP_ALIGNMENTS_H
153 const std::vector<PlacedVolume>&
nodes()
const;
159 void localToWorld(
const Double_t local[3], Double_t global[3])
const;
170 void worldToLocal(
const Double_t global[3], Double_t local[3])
const;
175 {
return worldToLocal({global[0],global[1],global[2]}); }
181 void localToDetector(
const Double_t local[3], Double_t detector[3])
const;
192 void detectorToLocal(
const Double_t detector[3], Double_t local[3])
const;
200 #endif // DD4HEP_ALIGNMENTS_H
Position localToWorld(const Double_t local[3]) const
Transformation from local coordinates of the placed volume to the world system.
const TGeoHMatrix & detectorTransformation() const
Access the alignment/placement matrix with respect to the world.
AlignmentCondition(const Handle< Q > &e)
Constructor to be used when reading the already parsed object.
detail::AlignmentObject Object
Extern accessible definition of the contained element type.
AlignmentCondition(detail::ConditionObject *p)
Assignment constructor from condition object.
Abstract base for processing callbacks to container objects.
AlignmentData & data()
Data accessor for the use of decorators.
Main handle class to hold an alignment conditions object.
virtual ~Processor()=default
Default destructor.
Alignment(const Handle< Q > &e)
Constructor to be used when reading the already parsed object.
const std::vector< PlacedVolume > & nodes() const
Access to the node list.
Handle: a templated class like a shared pointer, which allows specialized access to tgeometry objects...
Alignment and Delta item key.
Processor()
Default constructor.
const char * name() const
Access the object name (or "" if not supported by the object)
bool is_bound() const
Check if object is already bound....
const Delta & delta() const
Access the delta value of the object.
Class describing the interval of validty type.
Position detectorToLocal(const Double_t det[3]) const
Transformation from detector element coordinates to the local placed volume coordinates.
void localToWorld(const Position &local, Position &global) const
Transformation from local coordinates of the placed volume to the world system.
Class describing an condition to re-adjust an alignment.
void worldToLocal(const Position &global, Position &local) const
Transformation from world coordinates of the local placed volume coordinates.
Class describing the interval of validty.
static const Condition::itemkey_type alignmentKey
Key value of an alignment condition object "alignment".
AlignmentData & data()
Data accessor for the use of decorators.
static const Condition::itemkey_type deltaKey
Key value of a delta condition "alignment_delta".
const TGeoHMatrix & detectorTransformation() const
Access the alignment/placement matrix with respect to the world.
static const std::string deltaName
Key name of a delta condition "alignment_delta".
const TGeoHMatrix & worldTransformation() const
Create cached matrix to transform to world coordinates.
static const std::string alignmentName
Key name of an alignment condition object "alignment".
Position worldToLocal(const Double_t global[3]) const
Transformation from local coordinates of the placed volume to the world system.
Alignment(Object *p)
Default constructor.
Main handle class to hold an alignment object.
Derived condition data-object definition.
void detectorToLocal(const Position &detector, Position &local) const
Transformation from detector element coordinates to the local placed volume coordinates.
void localToDetector(const Position &local, Position &detector) const
Transformation from local coordinates of the placed volume to the detector system.
unsigned long long int key_type
Forward definition of the key type.
Alignment()=default
Default constructor.
unsigned int itemkey_type
Low part of the key identifies the item identifier.
AlignmentCondition(Object *p)
Default constructor.
const Delta & delta() const
Access the delta value of the object.
const TGeoHMatrix & worldTransformation() const
Create cached matrix to transform to world coordinates.
The data class behind a conditions handle.
ROOT::Math::XYZVector Position
virtual int operator()(Alignment alignment) const =0
Container callback for object processing.
Namespace for the AIDA detector description toolkit.
Position localToDetector(const Double_t local[3]) const
Transformation from local coordinates of the placed volume to the world system.
const IOV & iov() const
Access the IOV block.
const IOVType & iovType() const
Access the IOV type.
AlignmentCondition()
Default constructor.
The data class behind an alignments handle.
key_type key() const
Access the hash identifier.