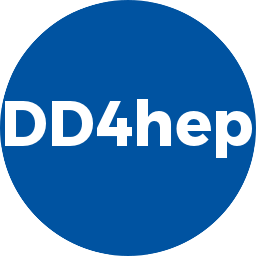 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_DETECTOR_H
14 #define DD4HEP_DETECTOR_H
16 #include <DD4hep/Version.h>
93 typedef std::map<std::string, Handle<NamedObject> >
HandleMap;
95 typedef std::map<std::string, PropertyValues>
Properties;
196 virtual const std::vector<DetElement>&
detectors(
const std::string& type,
197 bool throw_exc=
false)
const = 0;
200 virtual std::vector<DetElement>
detectors(
const std::string& type1,
201 const std::string& type2,
202 const std::string& type3=
"",
203 const std::string& type4=
"",
204 const std::string& type5=
"" ) = 0;
213 virtual std::vector<DetElement>
detectors(
unsigned int includeFlag,
214 unsigned int excludeFlag=0 )
const = 0 ;
245 template <
class T> T
constant(
const std::string& name)
const;
308 virtual void fromXML (
const std::string& fname,
311 virtual void fromXML (
const std::string& fname,
318 virtual long apply(
const char* factory,
int argc,
char** argv)
const = 0;
331 template <
typename IFACE,
typename CONCRETE> IFACE*
addExtension(CONCRETE* c) {
342 template <
class IFACE> IFACE*
extension(
bool alert=
true)
const {
343 return (IFACE*)
userExtension(detail::typeHash64<IFACE>(),alert);
351 static std::unique_ptr<Detector>
make_unique(
const std::string& name);
361 template <>
inline short Detector::constant<short>(
const std::string& name)
const {
367 template <>
inline unsigned short Detector::constant<unsigned short>(
const std::string& name)
const {
372 template <>
inline int Detector::constant<int>(
const std::string& name)
const {
377 template <>
inline unsigned int Detector::constant<unsigned int>(
const std::string& name)
const {
382 template <>
inline long Detector::constant<long>(
const std::string& name)
const {
387 template <>
inline unsigned long Detector::constant<unsigned long>(
const std::string& name)
const {
392 template <>
inline float Detector::constant<float>(
const std::string& name)
const {
397 template <>
inline double Detector::constant<double>(
const std::string& name)
const {
402 template <>
inline std::string Detector::constant<std::string>(
const std::string& name)
const {
403 return constantAsString(name);
407 #endif // DD4HEP_DETECTOR_H
virtual TGeoManager & manager() const =0
Access the geometry manager of this instance.
IFACE * addExtension(CONCRETE *c)
Extend the sensitive detector element with an arbitrary structure accessible by the type.
State
The detector description states.
virtual void setHeader(Header h)=0
Accessor to the header entry.
@ LOADING
The geometry is being created and loaded. (parsing ongoing)
Definition of the extension entry interface class.
virtual void dump() const =0
Stupid legacy method.
virtual DetElement world() const =0
Return reference to the top-most (world) detector element.
virtual Detector & addIDSpecification(const Handle< NamedObject > &element)=0
Add a new id descriptor by named reference to the detector description.
virtual DetElement detector(const std::string &name) const =0
Retrieve a subdetector element by its name from the detector description.
virtual const std::vector< DetElement > & detectors(const std::string &type, bool throw_exc=false) const =0
Access a set of subdetectors according to the sensitive type.
Handle class to hold the information of a sensitive detector.
std::map< std::string, PropertyValues > Properties
virtual Material vacuum() const =0
Return handle to material describing vacuum.
Handle class describing visualization attributes.
virtual const HandleMap & detectors() const =0
Accessor to the map of sub-detectors.
Class implementing the ID encoding of the detector response.
virtual Detector & add(IDDescriptor spec)=0
Add a new id descriptor to the detector description.
virtual void * removeUserExtension(unsigned long long int key, bool destroy)=0
Remove an existing extension object from the Detector instance.
virtual Detector & add(CartesianField entry)=0
Add a field component to the detector description.
std::string versionString()
return a string with the current dd4hep version in the form vXX-YY.
std::map< std::string, std::string > PropertyValues
virtual Region region(const std::string &name) const =0
Retrieve a region object by its name from the detector description.
virtual Detector & add(LimitSet limitset)=0
Add a new limit set to the detector description.
virtual const HandleMap & readouts() const =0
Accessor to the map of readout structures.
virtual double constantAsDouble(const std::string &name) const =0
Typed access to constants: double values.
virtual Volume pickMotherVolume(const DetElement &sd) const =0
Access mother volume by detector element.
virtual const HandleMap & fields() const =0
Accessor to the map of field entries, which together form the global field.
virtual Detector & add(DetElement detector)=0
Add a new subdetector to the detector description.
virtual Volume worldVolume() const =0
Return handle to the world volume containing everything.
virtual void * addUserExtension(unsigned long long int key, ExtensionEntry *entry)=0
Add an extension object to the detector element (low level member function)
virtual void fromXML(const std::string &fname, xml::UriReader *entity_resolver, DetectorBuildType type=BUILD_DEFAULT)=0
Read any geometry description or alignment file with external XML entity resolution.
virtual VolumeManager volumeManager() const =0
Return handle to the VolumeManager.
virtual IDDescriptor idSpecification(const std::string &name) const =0
Retrieve a id descriptor by its name from the detector description.
virtual void * userExtension(unsigned long long int key, bool alert=true) const =0
Access an existing extension object from the detector element (low level member function)
virtual Detector & addVisAttribute(const Handle< NamedObject > &element)=0
Add a new visualisation attribute by named reference to the detector description.
Class describing a field overlay with several sources.
virtual Detector & addField(const Handle< NamedObject > &field)=0
Add a field component by named reference to the detector description.
virtual Detector & addLimitSet(const Handle< NamedObject > &limset)=0
Add a new limit set by named reference to the detector description.
Handle class describing a material.
virtual Detector & addConstant(const Handle< NamedObject > &element)=0
Add a new constant by named reference to the detector description.
virtual Material material(const std::string &name) const =0
Retrieve a matrial by its name from the detector description.
virtual DetElement trackers() const =0
Return reference to detector element with all tracker devices.
virtual void setTrackingVolume(Volume vol)=0
Set the tracking volume of the detector.
static Detector & getInstance(const std::string &name="default")
—Factory method----—
virtual const HandleMap & constants() const =0
Accessor to the map of constants.
Helper class to access default temperature and pressure.
Handle class describing a detector element.
virtual Detector & add(Readout readout)=0
Add a new detector readout to the detector description.
virtual Detector & add(Region region)=0
Add a new detector region to the detector description.
virtual Material air() const =0
Return handle to material describing air.
Handle class describing a constant (define) object in description.
Handle class holding a placed volume (also called physical volume)
virtual Detector & addSensitiveDetector(const Handle< NamedObject > &element)=0
Add a new sensitive detector by named reference to the detector description.
Class to support the retrieval of detector elements and volumes given a valid identifier.
virtual long apply(const char *factory, int argc, char **argv) const =0
Manipulate geometry using factory converter.
virtual const HandleMap & regions() const =0
Accessor to the map of region settings.
virtual OverlayedField field() const =0
Return handle to the combined electromagentic field description.
virtual void init()=0
Initialize geometry.
Implementation class for the object extension mechanism.
Handle class describing a set of limits as they are used for simulation.
virtual SensitiveDetector sensitiveDetector(const std::string &name) const =0
Retrieve a sensitive detector by its name from the detector description.
Namespace for the AIDA detector description toolkit supporting XML utilities.
virtual std::string constantAsString(const std::string &name) const =0
Typed access to constants: access string values.
static void destroyInstance(const std::string &name="default")
Destroy the singleton instance.
virtual OpticalSurfaceManager surfaceManager() const =0
Access the optical surface manager.
virtual void endDocument(bool close_geometry=true)=0
Finalize the geometry.
virtual const STD_Conditions & stdConditions() const =0
Access default conditions (temperature and pressure.
virtual const HandleMap & limitsets() const =0
Accessor to the map of limit settings.
Handle class describing a region as used in simulation.
virtual std::vector< std::string > detectorTypes() const =0
Access the availible detector types.
virtual const HandleMap & sensitiveDetectors() const =0
Accessor to the map of sub-detectors.
static std::unique_ptr< Detector > make_unique(const std::string &name)
Unique creation without internal registration.
virtual void fromCompact(const std::string &fname, DetectorBuildType type=BUILD_DEFAULT)=0
Deprecated call (use fromXML): Read compact geometry description or alignment file.
virtual LimitSet limitSet(const std::string &name) const =0
Retrieve a limitset by its name from the detector description.
virtual Detector & add(VisAttr attr)=0
Add a new visualisation attribute to the detector description.
virtual Detector & add(Constant constant)=0
Add a new constant to the detector description.
virtual Volume trackingVolume() const =0
Return handle to the volume containing the tracking devices.
virtual VisAttr visAttributes(const std::string &name) const =0
Retrieve a visualization attribute by its name from the detector description.
virtual VisAttr invisible() const =0
Return handle to "invisible" visualization attributes.
virtual const HandleMap & visAttributes() const =0
Accessor to the map of visualisation attributes.
virtual State state() const =0
Access the state of the geometry.
Class supporting to read data given a URI.
std::map< std::string, Handle< NamedObject > > HandleMap
Type definition of a map of named handles.
@ NOT_READY
The detector description object is freshly created. No geometry nothing.
virtual Detector & add(SensitiveDetector entry)=0
Add a new sensitive detector to the detector description.
virtual DetectorBuildType buildType() const =0
Access flag to steer the detail of building of the geometry/detector description.
virtual ~Detector()=default
Destructor.
virtual Constant constant(const std::string &name) const =0
Retrieve a constant by its name from the detector description.
virtual long constantAsLong(const std::string &name) const =0
Typed access to constants: long values.
IFACE * extension(bool alert=true) const
Access extension element by the type.
DetectorBuildType
Detector description build types.
Namespace for the AIDA detector description toolkit.
T constant(const std::string &name) const
Typed access to constants: access any type values.
virtual void fromXML(const std::string &fname, DetectorBuildType type=BUILD_DEFAULT)=0
Read any geometry description or alignment file.
virtual Detector & addDetector(const Handle< NamedObject > &detector)=0
Add a new subdetector by named reference to the detector description.
The main interface to the dd4hep detector description package.
bool is_user_defined() const
Handle to the implementation of the readout structure of a subdetector.
virtual void setStdConditions(double temp, double pressure)=0
Set the STD temperature and pressure.
virtual CartesianField field(const std::string &name) const =0
Retrieve a field component by its name from the detector description.
virtual Readout readout(const std::string &name) const =0
Retrieve a readout object by its name from the detector description.
virtual const HandleMap & idSpecifications() const =0
Accessor to the map of ID specifications.
virtual void setStdConditions(const std::string &type)=0
Set the STD conditions according to defined types (STP or NTP)
virtual void declareParent(const std::string &detector_name, const DetElement &det)=0
Register new parent detector using the detector name.
virtual Detector & addReadout(const Handle< NamedObject > &readout)=0
Add a new detector readout by named reference to the detector description.
virtual Volume parallelWorldVolume() const =0
Return handle to the parallel world volume.
virtual std::vector< DetElement > detectors(unsigned int includeFlag, unsigned int excludeFlag=0) const =0
virtual Header header() const =0
Accessor to the map of header entries.
IFACE * removeExtension(bool destroy=true)
Remove an existing extension object from the Detector instance. If not destroyed, the instance is ret...
@ READY
The geometry is loaded.
Class to support the handling of optical surfaces.
virtual Detector & addRegion(const Handle< NamedObject > ®ion)=0
Add a new detector region by named reference to the detector description.
virtual Properties & properties() const =0
Access to properties map.
Base class describing any field with 3D cartesian vectors for the field strength.
virtual std::vector< DetElement > detectors(const std::string &type1, const std::string &type2, const std::string &type3="", const std::string &type4="", const std::string &type5="")=0
Access a set of subdetectors according to several sensitive types.