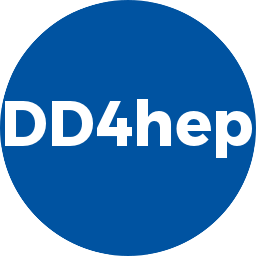 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
19 #include <DD4hep/detail/Handle.inl>
40 except(
"ConditionsManager",
"+++ Failed to access installed manager from Detector.");
64 listeners.insert(callee);
67 Listeners::iterator i=listeners.find(callee);
68 if ( i != listeners.end() ) listeners.erase(i);
84 listener.first->onRegisterCondition(condition, listener.second);
90 listener.first->onRegisterCondition(condition, listener.second);
95 std::vector<const IOVType*> result;
96 const auto& types = this->
iovTypes();
97 for (
const auto& i : types ) {
105 size_t id1 = data.find(
',');
106 size_t id2 = data.find(
'#');
107 if ( id2 == std::string::npos ) {
108 except(
"ConditionsManager",
"+++ Unknown IOV string representation: %s",data.c_str());
110 std::string iov_name = data.substr(id2+1);
115 if ( id1 != std::string::npos ) {
116 nents = ::sscanf(data.c_str(),
"%ld,%ld#",&k1,&k2) == 2 ? 2 : 0;
121 nents = ::sscanf(data.c_str(),
"%ld#",&k1) == 1 ? 1 : 0;
122 key.second =
key.first = k1;
125 except(
"ConditionsManager",
126 "+++ Failed to read keys from IOV string representation: %s",data.c_str());
134 except(
"ConditionsManager",
"+++ Unknown IOV type requested from data: %s. [%s]",
156 except(
"ConditionsManagerInstaller",
"Failed to create manager object of type %s",
159 assign(obj,
"ConditionsManager",factory);
193 std::pair<bool, const dd4hep::IOVType*>
211 std::vector<const IOVType*> result;
212 const auto& types = obj->
iovTypes();
213 result.reserve(types.size());
214 for(
const auto& i : types )
226 except(
"ConditionsManager",
"Invalid IOV type registration requested by IOV:%s",iov_rep.c_str());
234 except(
"ConditionsManager",
"+++ Attempt to register invalid IOV [FAILED]");
const IOVType * iovType(const std::string &iov_name) const
Access IOV by its name.
ConditionsDataLoader & loader() const
Access the conditions loader.
ConditionsPool * registerIOV(const IOV &iov) const
Register IOV with type and key.
void adoptCleanup(ConditionsCleanup *cleaner) const
Adopt cleanup handler. If a handler is registered, it is invoked at every "prepare" step.
AlignmentCondition::Object * cond
void declareProperty(const std::string &nam, T &val)
Declare property.
virtual ConditionsIOVPool * iovPool(const IOVType &type) const =0
Access conditions multi IOV pool by iov type.
The property class to assign options to actions.
virtual bool registerUnlocked(ConditionsPool &pool, Condition cond)=0
Register new condition with the conditions store. Unlocked version, not multi-threaded.
virtual Result load(const IOV &required_validity, ConditionsSlice &slice, ConditionUpdateUserContext *ctxt=0)=0
Load all updates to the clients with the defined IOV (1rst step of prepare)
virtual ~ConditionsManagerObject()
Default destructor.
virtual std::unique_ptr< UserPool > createUserPool(const IOVType *iovT) const =0
Create empty user pool object.
void clear() const
Full cleanup of all managed conditions.
Detector & detectorDescription() const
Access the detector description.
virtual const std::vector< IOVType > & iovTypes() const =0
Access IOV by its type.
static constexpr unsigned int UNKNOWN_IOV
virtual const IOVType * iovType(size_t iov_type) const =0
Access IOV by its type.
Property & operator[](const std::string &property_name) const
Access to properties.
std::pair< Key_value_type, Key_value_type > Key
static void increment(T *)
Increment count according to type information.
virtual std::pair< bool, const IOVType * > registerIOVType(size_t iov_type, const std::string &iov_name)=0
Register new IOV type if it does not (yet) exist.
ConditionUpdateUserContext class used by the derived conditions calculation mechanism.
Listeners m_onRegister
Conditions listeners on registration of new conditions.
Class describing the interval of validty type.
virtual void adoptCleanup(ConditionsCleanup *cleaner)=0
Adopt cleanup handler. If a handler is registered, it is invoked at every "prepare" step.
const std::vector< const IOVType * > iovTypesUsed() const
Access the used/registered IOV types.
DD4HEP_INSTANTIATE_HANDLE_NAMED(ConditionsManagerObject)
void fromString(const std::string &iov_str, IOV &iov)
Create IOV from string.
std::string invalidArg()
System error string for EINVAL. Sets errno accordingly.
Basic conditions manager implementation.
ConditionsIOVPool * iovPool(const IOVType &type) const
Access conditions multi IOV pool by iov type.
Main condition object handle.
std::pair< bool, const IOVType * > registerIOVType(size_t iov_type, const std::string &iov_name) const
Register new IOV type if it does not (yet) exist.
static ConditionsManager from(T &host)
Static accessor if installed as an extension.
Namespace for implementation details of the AIDA detector description toolkit.
Class describing the interval of validty.
Detector & detectorDescription() const
Access to the detector description instance.
Result prepare(const IOV &required_validity, ConditionsSlice &slice, ConditionUpdateUserContext *ctxt=0) const
Prepare all updates to the clients with the defined IOV.
void pushUpdates() const
Push all pending updates to the conditions store.
virtual size_t blockRegister(ConditionsPool &pool, const std::vector< Condition > &cond) const =0
Register a whole block of conditions with identical IOV.
Listeners m_onRemove
Conditions listeners on de-registration of new conditions.
void set(const Key &value)
Set discrete IOV value.
const std::vector< const IOVType * > iovTypesUsed() const
Access the used/registered IOV types.
virtual void initialize()=0
Initialize the object after having set the properties.
void assign(Object *n, const std::string &nam, const std::string &title)
Assign a new named object. Note: object references must be managed by the user.
void registerCallee(Listeners &listeners, const Listener &callee, bool add)
Register callback listener object.
const Property & property(const std::string &name) const
Access property by name (CONST)
Class implementing the conditions collection for a given IOV type.
bool registerUnlocked(ConditionsPool &pool, Condition cond) const
Register new condition with the conditions store. Unlocked version, not multi-threaded.
void onRegister(Condition condition)
Listener invocation when a condition is registered to the cache.
static void decrement(T *)
Decrement count according to type information.
PropertyManager & properties() const
Access to the property manager.
Key keyData
IOV key (if second==first, discrete, otherwise range)
Result load(const IOV &required_validity, ConditionsSlice &slice, ConditionUpdateUserContext *ctxt=0) const
Load all updates to the clients with the defined IOV (1rst step of prepare)
virtual Result prepare(const IOV &req_iov, ConditionsSlice &slice, ConditionUpdateUserContext *ctx=0)=0
Prepare all updates to the clients with the defined IOV.
std::pair< ConditionsListener *, void * > Listener
virtual Result compute(const IOV &required_validity, ConditionsSlice &slice, ConditionUpdateUserContext *ctxt=0)=0
Compute all derived conditions with the defined IOV (2nd step of prepare)
Key key() const
Get the local key of the IOV.
ConditionsPool * registerIOV(const std::string &data)
Register IOV using new string data.
Result compute(const IOV &required_validity, ConditionsSlice &slice, ConditionUpdateUserContext *ctxt=0) const
Compute all derived conditions with the defined IOV (2nd step of prepare)
Manager class for condition handles.
void callOnRegister(const Listener &callee, bool add)
(Un)Registration of conditions listeners with callback when a new condition is registered
unsigned int type
IOV buffer type: Must be a bitmap!
Result of a prepare call to the conditions manager.
virtual PropertyManager & properties() override
Access to the properties of the object.
Interface for a generic conditions loader.
ConditionsManagerObject * access() const
Checked object access. Throws invalid handle runtime exception if invalid handle.
Base class to handle conditions cleanups.
Manager to ease the handling of groups of properties.
ConditionsManagerObject * ptr() const
Access to the held object.
ConditionsManager & initialize()
Initialize the object after having set the properties.
ConditionsManagerObject(Detector &description)
Default constructor.
const IOVType * iovType
Reference to IOV type.
void clean(const ConditionsCleanup &cleaner) const
Invoke cache cleanup with user defined policy.
IFACE * extension(bool alert=true) const
Access extension element by the type.
Namespace for the AIDA detector description toolkit.
Implementation of a named object.
void onRemove(Condition condition)
Listener invocation when a condition is deregistered from the cache.
std::set< Listener > Listeners
ConditionsDataLoader * loader() const
Access to the data loader.
ConditionsManager()=default
Default constructor.
The main interface to the dd4hep detector description package.
std::unique_ptr< UserPool > createUserPool(const IOVType *iovT) const
Create empty user pool object.
size_t blockRegister(ConditionsPool &pool, const std::vector< Condition > &cond) const
Register a whole block of conditions with identical IOV.
void fromString(const std::string &iov_str, IOV &iov) const
Create IOV from string.
void callOnRemove(const Listener &callee, bool add)
(Un)Registration of conditions listeners with callback when a condition is unregistered
unsigned int type
integer identifier used internally
Pool of conditions satisfying one IOV type (epoch, run, fill, etc)
virtual int clean(const IOVType *typ, int max_age)=0
Clean conditions, which are above the age limit.
bool m_doLoad
Property: Flag to indicate if items should be loaded (or not)
Conditions slice object. Defines which conditions should be loaded by the ConditionsManager.
virtual std::pair< int, int > clear()=0
Full cleanup of all managed conditions.
bool m_doOutputUnloaded
Property: Flag to indicate if unloaded items should be saved to the slice (or not)