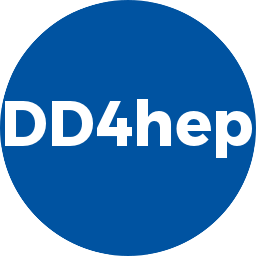 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
22 #include <TTimeStamp.h>
35 load_nominal_alignments(c.second);
48 TFile* f = TFile::Open(fname,
"RECREATE");
49 if ( f && !f->IsZombie()) {
52 DetectorData::patchRootStreamer(TGeoVolume::Class());
53 DetectorData::patchRootStreamer(TGeoNode::Class());
54 load_nominal_alignments(description.
world());
68 for(
const auto& vol : mgr.second->volumes ) {
69 persist->
nominals[vol.second->element] = vol.second->element.nominal();
72 printout(ALWAYS,
"DD4hepRootPersistency",
"+++ Saving %ld nominals....",persist->
nominals.size());
76 "DD4hepRootPersistency",
"+++ No valid Volume manager. No nominals saved.",
81 int nBytes = persist->Write(instance);
84 printout(ALWAYS,
"DD4hepRootPersistency",
85 "+++ Wrote %d Bytes of geometry data '%s' to '%s' [%8.3f seconds].",
86 nBytes, instance, fname, stop.AsDouble()-start.AsDouble());
88 printout(ALWAYS,
"DD4hepRootPersistency",
89 "+++ Successfully saved geometry data to file.");
93 DetectorData::unpatchRootStreamer(TGeoVolume::Class());
94 DetectorData::unpatchRootStreamer(TGeoNode::Class());
98 DetectorData::unpatchRootStreamer(TGeoVolume::Class());
99 DetectorData::unpatchRootStreamer(TGeoNode::Class());
100 except(
"DD4hepRootPersistency",
"Exception %s while saving file %s",e.what(), fname);
103 DetectorData::unpatchRootStreamer(TGeoVolume::Class());
104 DetectorData::unpatchRootStreamer(TGeoNode::Class());
105 except(
"DD4hepRootPersistency",
"UNKNOWN exception while saving file %s", fname);
109 printout(ERROR,
"DD4hepRootPersistency",
"+++ Cannot open file '%s'.",fname);
114 DetectorData::patchRootStreamer(TGeoVolume::Class());
115 DetectorData::patchRootStreamer(TGeoNode::Class());
116 TFile* f = TFile::Open(fname);
117 if ( f && !f->IsZombie()) {
121 if ( persist.get() ) {
125 for(
const auto& idd : iddesc ) {
129 printout(ALWAYS,
"DD4hepRootPersistency",
130 "+++ Fixed %ld IDDescriptor objects.",iddesc.size());
132 for(
const auto& sg : persist->m_segments ) {
139 printout(ALWAYS,
"DD4hepRootPersistency",
140 "+++ Fixed %ld segmentation objects.",persist->m_segments.size());
141 persist->m_segments.clear();
142 if ( persist->volumeManager().isValid() ) {
143 const auto& sdets = persist->volumeManager()->subdetectors;
144 size_t num[3] = {0,0,0};
145 for(
const auto& vm : sdets ) {
146 VolumeManager::Object* obj = vm.second.ptr();
147 obj->system = obj->id.field(
"system");
148 if ( 0 != obj->system ) {
149 printout(ALWAYS,
"DD4hepRootPersistency",
150 "+++ Fixed VolumeManager.system for %-24s %6ld volumes %4ld sdets %4ld mgrs.",
151 obj->detector.path().c_str(), obj->volumes.size(),
152 obj->subdetectors.size(), obj->managers.size());
153 num[0] += obj->volumes.size();
154 num[1] += obj->subdetectors.size();
155 num[2] += obj->managers.size();
158 printout(ALWAYS,
"DD4hepRootPersistency",
159 "+++ FAILED to fix VolumeManager.system for '%s: %s'.",
160 obj->detector.path().c_str(),
"[No IDDescriptor field 'system']");
162 printout(ALWAYS,
"DD4hepRootPersistency",
163 "+++ Fixed VolumeManager TOTALS %-24s %6ld volumes %4ld sdets %4ld mgrs.",
"",num[0],num[1],num[2]);
164 printout(ALWAYS,
"DD4hepRootPersistency",
"+++ loaded %ld nominals....",persist->nominals.size());
167 printout(ALWAYS,
"DD4hepRootPersistency",
"+++ Volume manager NOT restored. [Was it ever up when saved?]");
171 if( tar_data !=
nullptr && src_data !=
nullptr ) {
174 printout(ALWAYS,
"DD4hepRootPersistency",
175 "+++ Successfully loaded detector description from file:%s [%8.3f seconds]",
176 fname, stop.AsDouble()-start.AsDouble());
177 DetectorData::unpatchRootStreamer(TGeoVolume::Class());
178 DetectorData::unpatchRootStreamer(TGeoNode::Class());
181 DetectorData::unpatchRootStreamer(TGeoVolume::Class());
182 DetectorData::unpatchRootStreamer(TGeoNode::Class());
185 printout(ERROR,
"DD4hepRootPersistency",
186 "+++ Cannot Cannot load instance '%s' from file '%s'.",
192 DetectorData::unpatchRootStreamer(TGeoVolume::Class());
193 DetectorData::unpatchRootStreamer(TGeoNode::Class());
194 except(
"DD4hepRootPersistency",
"Exception %s while loading file %s",e.what(), fname);
197 DetectorData::unpatchRootStreamer(TGeoVolume::Class());
198 DetectorData::unpatchRootStreamer(TGeoNode::Class());
199 except(
"DD4hepRootPersistency",
"UNKNOWN exception while loading %s", fname);
203 DetectorData::unpatchRootStreamer(TGeoVolume::Class());
204 DetectorData::unpatchRootStreamer(TGeoNode::Class());
205 printout(ERROR,
"DD4hepRootPersistency",
"+++ Cannot open file '%s'.",fname);
215 class PersistencyChecks {
220 PersistencyChecks() =
default;
222 size_t checkConstant(
const std::pair<std::string,Constant>& obj) {
223 if ( obj.first.empty() || obj.second->name.empty() ) {
224 printout(ERROR,
"chkConstant",
"+++ Invalid constant: key error %s <> %s [%s]",
225 obj.first.c_str(), obj.second->GetName(), obj.second->GetTitle());
229 if ( obj.first != obj.second->GetName() ) {
230 printout(ERROR,
"chkConstant",
"+++ Invalid constant: key error %s <> %s [%s]",
231 obj.first.c_str(), obj.second->GetName(), obj.second->GetTitle());
238 size_t checkProperty(
const std::pair<std::string, std::map<std::string,std::string> >& obj) {
239 if ( obj.first.empty() || obj.second.empty() ) {
240 printout(ERROR,
"chkProperty",
"+++ Empty property set: %s",obj.first.c_str());
250 printout(INFO,
"chkDetector",
"+++ %-40s Level:%3d Key:%08X VolID:%016llX",
255 printout(INFO,
"chkDetector",
"+++ Ideal: %s Delta:%s--%s--%s",
264 printout(INFO,
"chkDetector",
"+++ Ideal: %s",yes_no(ideal.
isValid()));
266 for(
const auto& c : children )
267 count += printDetElement(c.second);
272 printout(INFO,
"chkDetector",
"+++ Checking Sub-Detector: %-40s Key:%08X VolID:%016llX",
274 return printDetElement(d);
279 printout(INFO,
"chkDetector",
"+++ Checking SensitiveDetector: %-30s %-16s CombineHits:%s ecut:%g Collection:%s",
280 d.
name(), (
"["+d.
type()+
"]").c_str(),
284 printout(ERROR,
"chkDetector",
285 "+++ FAILED SensitiveDetector:%s No Readout Stricture attached.",
291 if ( !checkReadout(ro) ) {
298 size_t checkReadout(
Readout ro) {
300 printout(INFO,
"chkReadOut",
"+++ Checking Readout: %s Collection No:%ld IDspec:%s",
303 if (
id.isValid() ) {
304 if ( !checkIDDescriptor(
id) ) ret = 0;
307 printout(ERROR,
"chkReadOut",
308 "+++ FAILED Segmentation: %s Found readout without ID descriptor!",
316 if ( !checkSegmentation(sg) ) {
319 if (
id.isValid() && seg &&
id.decoder() != sg.
decoder() ) {
320 printout(ERROR,
"chkReadOut",
"+++ FAILED Decoder ERROR:%p != %p",
321 (
void*)seg->
decoder(), (
void*)
id.decoder());
333 printout(INFO,
"chkSegment",
"+++ Checking Segmentation: %-24s [%s] DDSegmentation:%p Decoder:%p",
334 sg.
name(), seg->
type().c_str(),(
void*)seg, seg->decoder());
336 for (
const auto& par : pars ) {
337 printout(INFO,
"chkSegment",
"+++ Param:%-24s -> %-16s [%s] opt:%s",
338 par->name().c_str(), par->value().c_str(), par->type().c_str(),
339 yes_no(par->isOptional()));
342 if ( pars.empty() ) {
343 printout(ERROR,
"chkSegment",
344 "+++ FAILED Segmentation: %s Found readout with invalid [EMPTY] ID descriptor!",
350 printout(ERROR,
"chkSegment",
"+++ FAILED Segmentation: %s NO Decoder!!!",
357 printout(ERROR,
"chkSegment",
"+++ FAILED Segmentation: %s NO DDSegmentation",
365 printout(INFO,
"chkIDDescript",
"+++ Checking IDDesc: %s decoder:%p",
366 id->GetName(), (
void*)
id.
decoder());
367 printout(INFO,
"chkIDDescript",
"+++ Specs:%s",
id.fieldDescription().c_str());
368 for(
const auto& fld : fields )
369 printout(INFO,
"chkIDDescript",
"+++ Field:%-24s -> %016llX %3d %3d [%d,%d]",
370 fld.first.c_str(), fld.second->mask(), fld.second->offset(), fld.second->width(),
371 fld.second->minValue(), fld.second->maxValue());
372 if ( fields.empty() ) {
373 printout(ERROR,
"chkIDDescript",
374 "+++ FAILED IDDescriptor: %s Found invalid [EMPTY] ID descriptor!",
383 printout(INFO,
"chkLimitSet",
"+++ Checking Limits: %s Num.Limiits:%ld",
388 size_t checkRegion(
Region ls) {
389 printout(INFO,
"chkRegion",
"+++ Checking Limits: %s Num.Limiits:%ld",
395 printout(INFO,
"chkField",
"+++ Checking Field: %s",
400 AlignmentCondition::Object* align =
det->nominal.ptr();
402 printout(ERROR,
"chkNominals",
403 "+++ ERROR +++ Detector element with invalid nominal:%s",
407 else if ( 0 == align->alignment_data ) {
408 printout(ERROR,
"chkNominals",
409 "+++ ERROR +++ Detector element with invalid nominal data:%s",
413 else if (
Condition(align).data().ptr() != align->alignment_data ) {
414 printout(ERROR,
"chkNominals",
415 "+++ ERROR +++ Detector element with inconsisten nominal data:%s [%p != %p]",
416 det.path().c_str(),
Condition(align).data().ptr(),align->alignment_data);
428 const auto& sdets = mgr->subdetectors;
429 for(
const auto& vm : sdets ) {
430 VolumeManager::Object* obj = vm.second.
ptr();
431 for(
const auto& iv : obj->volumes ) {
433 count += checkAlignment(ctx->
element);
441 if (
v.isSensitive() ) {
442 count += checkAlignment(d);
444 for(
const auto& child : d.
children() )
445 count += checkDetectorNominals(child.second);
454 PersistencyChecks checks;
456 count += checks.checkConstant(obj);
457 printout(ALWAYS,
"chkProperty",
"+++ %s Checked %ld Constant objects. Num.Errors: %ld",
458 checks.errors==0 ?
"PASSED" :
"FAILED", count, checks.errors);
459 return checks.errors;
465 PersistencyChecks checks;
467 count += checks.checkProperty(obj);
468 printout(ALWAYS,
"chkProperty",
"+++ %s Checked %ld Property objects. Num.Errors: %ld",
469 checks.errors==0 ?
"PASSED" :
"FAILED", count, checks.errors);
470 return checks.errors;
476 printout(ALWAYS,
"chkMaterials",
"+++ Check not implemented. Hence OK.");
483 PersistencyChecks checks;
485 count += checks.checkReadout(obj.second);
488 printout(ERROR,
"chkNominals",
489 "+++ Number of sensitive detectors does NOT match number of readouts: %ld != %ld",
493 printout(ALWAYS,
"chkNominals",
"+++ %s Checked %ld readout objects. Num.Errors: %ld",
494 checks.errors==0 ?
"PASSED" :
"FAILED", count, checks.errors);
495 return { count, checks.errors };
501 PersistencyChecks checks;
503 count += checks.checkField(obj.second);
504 printout(ALWAYS,
"chkFields",
"+++ %s Checked %ld Field objects. Num.Errors: %ld",
505 checks.errors==0 ?
"PASSED" :
"FAILED", count, checks.errors);
506 return checks.errors;
512 PersistencyChecks checks;
514 count += checks.checkRegion(obj.second);
515 printout(ALWAYS,
"chkRegions",
"+++ %s Checked %ld Region objects. Num.Errors: %ld",
516 checks.errors==0 ?
"PASSED" :
"FAILED", count, checks.errors);
517 return { count, checks.errors };
523 PersistencyChecks checks;
525 count += checks.checkIDDescriptor(obj.second);
526 printout(ALWAYS,
"chkReadouts",
"+++ %s Checked %ld Readout objects. Num.Errors: %ld",
527 checks.errors==0 ?
"PASSED" :
"FAILED", count, checks.errors);
528 return { count, checks.errors };
534 PersistencyChecks checks;
536 count += checks.checkDetector(obj.second);
537 printout(ALWAYS,
"chkDetectors",
"+++ %s Checked %ld DetElement objects. Num.Errors: %ld",
538 checks.errors==0 ?
"PASSED" :
"FAILED", count, checks.errors);
539 return { count, checks.errors };
545 PersistencyChecks checks;
547 count += checks.checkSensitive(obj.second);
548 printout(ALWAYS,
"chkSensitives",
"+++ %s Checked %ld SensitiveDetector objects. Num.Errors: %ld",
549 checks.errors==0 ?
"PASSED" :
"FAILED", count, checks.errors);
550 return { count, checks.errors };
555 PersistencyChecks checks;
558 count += checks.checkLimitset(obj.second);
559 printout(ALWAYS,
"chkSensitives",
"+++ %s Checked %ld SensitiveDetector objects. Num.Errors: %ld",
560 checks.errors==0 ?
"PASSED" :
"FAILED", count, checks.errors);
561 return { count, checks.errors };
566 PersistencyChecks checks;
568 printout(ALWAYS,
"chkNominals",
"+++ %s Checked %ld VolumeManager contexts. Num.Errors: %ld",
569 checks.errors==0 ?
"PASSED" :
"FAILED", count, checks.errors);
570 return { count , checks.errors };
576 PersistencyChecks checks;
577 const auto& dets =
object->sensitiveDetectors();
578 for(
const auto& d : dets )
580 printout(ALWAYS,
"chkNominals",
"+++ %s Checked %ld DetElements. Num.Errors: %ld",
581 checks.errors==0 ?
"PASSED" :
"FAILED", count, checks.errors);
582 return { count, checks.errors };
588 PersistencyChecks checks;
589 const auto& dets =
object->sensitiveDetectors();
590 for(
const auto& d : dets ) {
592 if ( seg.
isValid() ) count += checks.checkSegmentation(seg);
594 printout(ALWAYS,
"chkNominals",
"+++ %s Checked %ld readout segmentations. Num.Errors: %ld",
595 checks.errors==0 ?
"PASSED" :
"FAILED", count, checks.errors);
596 return { count, checks.errors };
601 PersistencyChecks checks;
605 count += checks.checkProperty(obj);
607 count += checks.checkConstant(obj);
609 count += checks.checkLimitset(obj.second);
611 count += checks.checkField(obj.second);
613 count += checks.checkRegion(obj.second);
615 count += checks.checkIDDescriptor(obj.second);
617 count += checks.checkDetector(obj.second);
619 count += checks.checkSensitive(obj.second);
622 printout(ALWAYS,
"chkAll",
"+++ Checked %ld objects. Num.Errors:%ld",
623 count, checks.errors);
const Children & children() const
Access to the list of children.
dd4hep::VolumeManager volumeManager() const
Return handle to the VolumeManager.
ObjectHandleMap m_sensitive
The map of top level sub-detector sensitive detector objects indexed by the detector name.
virtual DetElement world() const =0
Return reference to the top-most (world) detector element.
std::pair< std::size_t, std::size_t > checkSegmentations() const
Call to check segmentations starting from the top level Detector element.
std::vector< std::pair< std::string, const Field * > > FieldMap
unsigned int key() const
Access hash key of this detector element (Only valid once geometry is closed!)
std::pair< std::size_t, std::size_t > checkLimitSets() const
Call to check a limit-set object.
virtual DetElement detector(const std::string &name) const =0
Retrieve a subdetector element by its name from the detector description.
Handle class to hold the information of a sensitive detector.
void exception(const std::string &src, const std::string &msg)
virtual const HandleMap & detectors() const =0
Accessor to the map of sub-detectors.
PlacedVolume placement() const
Access to the physical volume of this detector element.
static int load(dd4hep::Detector &description, const char *fname, const char *instance="Geometry")
Load an detector description from a ROOT file to memory.
const std::set< Limit > & limits() const
Accessor to limits container.
void setSegmentation(const Segmentation &segment) const
Assign segmentation structure to readout.
std::pair< std::size_t, std::size_t > checkRegions() const
Call to check a Region object.
Class implementing the ID encoding of the detector response.
DetElement element
Handle to the closest Detector element.
bool isValid() const
Check the validity of the object held by the handle.
virtual const HandleMap & readouts() const =0
Accessor to the map of readout structures.
std::pair< std::size_t, std::size_t > checkReadouts() const
Call to check a Readout object.
std::pair< std::size_t, std::size_t > checkDetectors() const
Call to check a top level Detector element (subdetector)
Helper class to support ROOT persistency of Detector objects.
static int save(dd4hep::Detector &description, const char *fname, const char *instance="Geometry")
Save an existing detector description in memory to a ROOT file.
DD4hepRootPersistency()
Default constructor.
virtual const HandleMap & fields() const =0
Accessor to the map of field entries, which together form the global field.
bool hasRotation() const
Access flags: Check if the delta operation contains a rotation.
VolumeID volumeID() const
The cached VolumeID of this subdetector element.
const char * name() const
Access the object name (or "" if not supported by the object)
const Delta & delta() const
Access the delta value of the object.
std::vector< std::string > & limits() const
Access references to user limits.
virtual VolumeManager volumeManager() const =0
Return handle to the VolumeManager.
Class describing an condition to re-adjust an alignment.
std::pair< std::size_t, std::size_t > checkIdSpecs() const
Call to check an ID specification.
bool hasPivot() const
Access flags: Check if the delta operation contains a pivot.
virtual const std::string & name() const
Access the segmentation name.
size_t checkFields() const
Call to theck the DD4hep fields.
Main condition object handle.
virtual Parameters parameters() const
Access to all parameters.
std::pair< std::size_t, std::size_t > checkVolManager() const
Call to check the volume manager hierarchy.
virtual const HandleMap & constants() const =0
Accessor to the map of constants.
Handle class describing a detector element.
const Detector::HandleMap & idSpecifications() const
Accessor to the map of ID specifications.
Handle class holding a placed volume (also called physical volume)
Class to support the retrieval of detector elements and volumes given a valid identifier.
virtual ~DD4hepRootPersistency()
Default destructor.
virtual const HandleMap & regions() const =0
Accessor to the map of region settings.
VolumeManager m_volManager
Volume manager reference.
dd4hep::DetectorData * m_data
The main data block.
Handle class describing a set of limits as they are used for simulation.
const std::string & hitsCollection() const
Access the hits collection name.
size_t checkAll() const
Check all of the above.
bool combineHits() const
Access flag to combine hist.
Class of the ROOT toolkit. See http://root.cern.ch/root/htmldoc/ClassIndex.html.
std::map< dd4hep::DetElement, dd4hep::AlignmentCondition > nominals
Helper to save alignment conditions from the DetElement nominals.
virtual const BitFieldCoder * decoder() const
Access the underlying decoder.
virtual const HandleMap & limitsets() const =0
Accessor to the map of limit settings.
Handle class describing a region as used in simulation.
Main handle class to hold an alignment object.
virtual const HandleMap & sensitiveDetectors() const =0
Accessor to the map of sub-detectors.
Alignment nominal() const
Access to the constant ideal (nominal) alignment information.
std::pair< std::size_t, std::size_t > checkSensitives() const
Call to check a sensitive detector.
std::pair< std::size_t, std::size_t > checkNominals() const
Call to check the nominal alignments in the DetElement hierarchy.
Segmentation segmentation() const
Access segmentation structure.
int level() const
Access the hierarchical level of the detector element (Only valid once geometry is closed!...
size_t checkMaterials() const
Call to check a Material object.
std::map< dd4hep::Readout, std::pair< dd4hep::IDDescriptor, dd4hep::DDSegmentation::Segmentation * > > m_segments
Helper since plain segmentations cannot be saved.
bool hasTranslation() const
Access flags: Check if the delta operation contains a translation.
std::string type() const
Access the type of the sensitive detector.
const char * name() const
Accessor: Segmentation type.
DDSegmentation::Segmentation * segmentation() const
Access to the base DDSegmentation object. WARNING: Deprecated call!
std::map< std::string, DetElement > Children
T * ptr() const
Access to the held object.
void adoptData(DetectorData &source, bool CLR=true)
Adopt all data from source structure.
const BitFieldCoder * decoder() const
Access the underlying decoder.
double energyCutoff() const
Access energy cut off.
void rebuild(const std::string &description)
Re-build object in place.
Namespace for the AIDA detector description toolkit.
Readout readout() const
Access readout structure of the sensitive detector.
Data implementation class of the Detector interface.
Volume volume() const
Logical volume of this placement.
ClassImp(DD4hepRootPersistency) using namespace dd4hep
size_t numCollections() const
Access number of hit collections.
This structure describes the cached data for one placement held by the volume manager.
The main interface to the dd4hep detector description package.
Handle to the implementation of the readout structure of a subdetector.
Handle class supporting generic Segmentations of sensitive detectors.
BitFieldCoder * decoder() const
Access the BitFieldCoder object.
IDDescriptor idSpec() const
Access IDDescription structure.
virtual const HandleMap & idSpecifications() const =0
Accessor to the map of ID specifications.
size_t checkProperties() const
Check detector description properties (string defines)
Base class for all segmentations.
Alignment survey() const
Access to the constant survey alignment information.
size_t checkConstants() const
Check the collection of define statements.
dd4hep::Detector * object
Reference to the detector model object.
virtual Properties & properties() const =0
Access to properties map.
Base class describing any field with 3D cartesian vectors for the field strength.
virtual const std::string & type() const
Access the segmentation type.