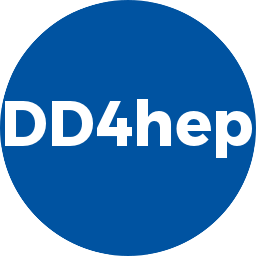 |
DD4hep
1.32.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
22 #include <TGeoManager.h>
23 #include <TClassStreamer.h>
24 #include <TDataMember.h>
25 #include <TDataType.h>
35 union FundamentalData {
49 void read(TBuffer& buff,
int dtyp) {
51 case 1: buff >> c;
break;
52 case 2: buff >> s;
break;
53 case 3: buff >> i;
break;
54 case 4: buff >> l;
break;
55 case 5: buff >> f;
break;
56 case 6: buff >> i;
break;
58 case 8: buff >> d;
break;
59 case 9: buff >> d;
break;
60 case 11: buff >> uc;
break;
61 case 12: buff >> us;
break;
62 case 13: buff >> ui;
break;
63 case 14: buff >> ul;
break;
64 case 15: buff >> ui;
break;
65 case 16: buff >> ll;
break;
66 case 17: buff >> ull;
break;
67 case 18: buff >> b;
break;
68 case 19: buff >> f;
break;
70 printout(ERROR,
"OpaqueData",
"Unknown fundamental data type: [%X]",dtyp);
74 void write(TBuffer& buff,
int dtyp)
const {
76 case 1: buff << c;
break;
77 case 2: buff << s;
break;
78 case 3: buff << i;
break;
79 case 4: buff << l;
break;
80 case 5: buff << f;
break;
81 case 6: buff << i;
break;
83 case 8: buff << d;
break;
84 case 9: buff << d;
break;
85 case 11: buff << uc;
break;
86 case 12: buff << us;
break;
87 case 13: buff << ui;
break;
88 case 14: buff << ul;
break;
89 case 15: buff << ui;
break;
90 case 16: buff << ll;
break;
91 case 17: buff << ull;
break;
92 case 18: buff << b;
break;
93 case 19: buff << f;
break;
95 printout(ERROR,
"OpaqueData",
"Unknown fundamental data type: [%X]",dtyp);
101 void stream_opaque_datablock(TBuffer& b,
void* obj) {
104 TClass* cl = BasicGrammar::instance<OpaqueDataBlock>().clazz();
108 if ( b.IsReading() ) {
109 b.ReadVersion(&R__s, &R__c, cl);
114 void* ptr = block->
ptr();
117 except(
"stream_opaque_datablock",
"Object cannot be handled by ROOT persistency. "
118 "Grammar %s does not allow for ROOT persistency.",gr.
type_name().c_str());
120 ptr = block->
bind(&gr);
124 if ( gr.
type() ==
typeid(std::string) )
125 b.ReadStdString(*(std::string*)ptr);
126 else if ( gr.
clazz() )
127 b.ReadClassBuffer(gr.
clazz(),ptr);
129 ((FundamentalData*)ptr)->read(b,gr.
data_type());
130 b.CheckByteCount(R__s, R__c, cl);
132 else if ( 0 == block->
grammar ) {
133 printout(ERROR,
"OpaqueData",
"+++ ERROR +++ Opaque data block has no grammar attached. Cannot be saved!");
136 const std::type_info& typ_info =
typeid(*block);
140 printout(DEBUG,
"OpaqueData",
"Saving object %p type: %s [%s] [%s]",
141 obj, typ_info.name(), typ.c_str(), block->
grammar->
name.c_str());
142 R__c = b.WriteVersion(cl,kTRUE);
146 if ( gr.
type() ==
typeid(std::string) )
147 b.WriteStdString(*(std::string*)block->
ptr());
148 else if ( gr.
clazz() )
149 b.WriteClassBuffer(gr.
clazz(),block->
ptr());
151 ((
const FundamentalData*)block->
ptr())->write(b,gr.
data_type());
152 b.SetByteCount(R__c, kTRUE);
160 : m_manager(0), m_world(), m_trackers(), m_worldVol(),
161 m_trackingVol(), m_field(),
163 m_inhibitConstants(false)
165 static bool first =
true;
168 TClass* cl = gROOT->GetClass(
"dd4hep::OpaqueDataBlock");
170 except(
"PersistencyIO",
"+++ Missing TClass for 'dd4hep::OpaqueDataBlock'.");
172 if ( 0 == cl->GetStreamer() ) {
173 cl->AdoptStreamer(
new TClassStreamer(stream_opaque_datablock));
174 printout(INFO,
"PersistencyIO",
"+++ Set Streamer to %s",cl->GetName());
189 printout(INFO,
"PersistencyIO",
190 "+++ Set data member %s.fUserExtension as PERSISTENT.",
192 dm = cl->GetDataMember(
"fUserExtension");
193 dm->SetTitle(dm->GetTitle()+2);
200 printout(INFO,
"PersistencyIO",
201 "+++ Set data member %s.fUserExtension as TRANSIENT.",
203 dm = cl->GetDataMember(
"fUserExtension");
204 dm->SetTitle((std::string(
"! ")+dm->GetTitle()).c_str());
205 dm->ResetBit(BIT(2));
227 std::cout <<
"Delete " << def.first << std::endl;
229 delete def.second.ptr();
300 w->description =
dynamic_cast<Detector*
>(
this);
317 if ( gGeoManager !=
m_manager )
delete gGeoManager;
Handle class to hold the information of the top DetElement object 'world'.
const std::string name
Instance type name.
unsigned long long int key_type
key_type hash() const
Access the hash value for this grammar type.
ObjectHandleMap m_sensitive
The map of top level sub-detector sensitive detector objects indexed by the detector name.
std::map< std::string, DetElement > m_detectorParents
ObjectHandleMap m_display
The map of display attributes in use.
void destroyHandle(T &handle)
Helper to delete objects from heap and reset the handle.
void clearData()
Clear data content: DOES NOT RELEASEW ALLOCATED RESOURCES!
Class describing an opaque conditions data block.
static void increment(T *)
Increment count according to type information.
ObjectHandleMap m_idDict
Map of readout IDDescriptors indexed by hit collection name.
DetectorData()
Default constructor.
static void patchRootStreamer(TClass *cl)
Assignment operator.
bool m_inhibitConstants
Flag to inhibit the access to global constants. Value set by constants section 'Detector_InhibitConst...
int data_type() const
Access ROOT data type for fundamentals.
Handle< T > & clear()
Release the object held by the handle.
const std::string & type_name() const
Access to the type information name.
void * bind(const BasicGrammar *grammar)
Bind data value.
ObjectHandleMap m_regions
Map of regions settings for the simulation.
Base class describing string evaluation to C++ objects using boost::spirit.
VolumeManager m_volManager
Volume manager reference.
ObjectHandleMap m_readouts
Map of readout descriptors indexed by subdetector name.
ObjectHandleMap m_detectors
The map of top level sub-detector objects indexed by name.
static void decrement(T *)
Decrement count according to type information.
void destroyHandles(M &arg)
Functional created of map destruction functors.
static const BasicGrammar & get(const std::type_info &info)
Access grammar by type info.
void destroyData(bool destroy_mgr=true)
Clear data content: releases all allocated resources.
void * ptr() const
Write access to the data buffer. Is only valid after call to bind<T>()
void(* bind)(void *pointer)=0
Bind opaque address to object.
void move(ObjectExtensions ©)
Move extensions to target object.
Detector::Properties m_properties
ObjectHandleMap m_fields
The map of electro magnet field components for the global overlay field.
virtual ~DetectorData()
Default destructor.
DetElement & setPlacement(const PlacedVolume &volume)
Set the physical volumes of the detector element.
struct dd4hep::BasicGrammar::specialization_t specialization
virtual const std::type_info & type() const =0
Access to the type information.
TClass * clazz() const
Access the ROOT class for complex objects.
Volume m_parallelWorldVol
Material m_materialVacuum
void adoptData(DetectorData &source, bool CLR=true)
Adopt all data from source structure.
ObjectExtensions m_extensions
Definition of the extension type.
void clear(bool destroy=true)
Clear all extensions.
Namespace for the AIDA detector description toolkit.
Data implementation class of the Detector interface.
The main interface to the dd4hep detector description package.
TGeoManager * m_manager
Reference to the geometry manager object from ROOT.
const BasicGrammar * grammar
Data type.
static void unpatchRootStreamer(TClass *cl)
UNPatch the ROOT streamers to adapt for DD4hep (set fUserExtension transient)
Concrete implementation class of the Detector interface.
ObjectHandleMap m_limits
Map of limit sets.