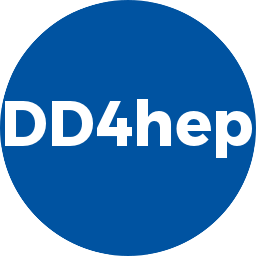 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_VOLUMES_H
14 #define DD4HEP_VOLUMES_H
28 #include <TGeoPatternFinder.h>
29 #include <TGeoExtension.h>
40 class SensitiveDetector;
81 typedef std::pair<std::string, int>
VolID;
88 class VolIDs:
public std::vector<VolID> {
90 typedef std::vector<VolID>
Base;
102 std::vector<VolID>::const_iterator
find(
const std::string& name)
const;
104 std::pair<std::vector<VolID>::iterator,
bool>
insert(
const std::string& name,
int value);
106 template<
class InputIt>
107 iterator
insert(InputIt first, InputIt last)
108 {
return this->Base::insert(this->Base::end(), first, last); }
109 #if !defined __GNUCC__ || (defined __GNUCC__ && __GNUC__ > 5)
110 template<
class InputIt>
112 iterator
insert(std::vector<VolID>::const_iterator pos, InputIt first, InputIt last)
113 {
return this->Base::insert(pos, first, last); }
115 std::string
str()
const;
118 class Parameterisation;
145 virtual void Release()
const override;
212 const char*
type()
const;
226 const TGeoMatrix&
matrix()
const;
342 virtual void Release()
const override;
408 Volume(
const std::string&
name,
const std::string& title);
437 const char*
type()
const;
451 Volume divide(
const std::string& divname,
int iaxis,
int ndiv,
double start,
double step,
int numed = 0,
const char*
option =
"");
616 size_t count_1,
const Position& inc_1,
617 size_t count_2,
const Position& inc_2,
618 size_t count_3,
const Position& inc_3);
632 size_t count_1,
const Position& inc_1,
633 size_t count_2,
const Position& inc_2,
634 size_t count_3,
const Position& inc_3);
655 std::string
option()
const;
659 const std::string& vis)
const;
705 void addProperty(
const std::string& nam,
const std::string& val)
const;
708 std::string
getProperty(
const std::string& nam,
const std::string& default_val=
"")
const;
711 operator TGeoVolume*()
const {
778 #endif // DD4HEP_VOLUMES_H
Implementation class extending the ROOT mulit-volumes (TGeoVolumeMulti)
virtual void Release() const override
TGeoExtension overload: Method called always when the pointer to the extension is not needed anymore.
const Volume & setLimitSet(const Detector &description, const std::string &name) const
Set the limits to the volume. Note: If the name string is empty, the action is ignored.
bool isAssembly() const
Test if this volume is an assembly structure.
Implementation class extending the ROOT volume (TGeoVolume)
unsigned char setSmartlessValue(unsigned char value)
Set the smartless option for G4 voxelization. Returns previous value.
Assembly & operator=(const Assembly &a)=default
Assignment operator (must match copy constructor)
Volume(Volume &&v)=default
Move from handle.
Helper class for BitFieldCoder that corresponds to one field value.
static void enableCopyNumberCheck(bool value)
Set flag to enable copy number checks when inserting new nodes.
@ NO_SMARTLESS_OPTIMIZATION
bool hasProperties() const
Check for existence of properties.
Assembly()=default
Default constructor.
Region region
Region reference.
Dimension trafo3D
Reference to the parameterised transformation for dimension 3.
unsigned long refCount
Number of entries for the parameterisation in dimension 2.
Handle class to hold the information of a sensitive detector.
Implementation class extending the ROOT placed volume.
virtual ~PlacedVolumeExtension()
Default destructor.
VolumeMulti()=default
Default constructor.
~ReflectionBuilder()=default
Default descructor.
virtual void Release() const override
TGeoExtension overload: Method called always when the pointer to the extension is not needed anymore.
Assembly(const Assembly &v)=default
Copy from handle.
virtual ~Parameterisation()=default
Default destructor.
Handle< TGeoVolume > reflected
Reference to the reflected volume (or to the original volume for reflections)
Handle class holding a placed volume (also called physical volume)
Handle class describing visualization attributes.
Abstract base for processing callbacks to PlacedVolume objects.
VolIDs & operator=(VolIDs &©)=default
Move assignment.
PlacedVolume paramVolume2D(Volume entity, size_t count_1, const Transform3D &inc_1, size_t count_2, const Transform3D &inc_2)
2D Parameterised volume implementation
PlacedVolume & addPhysVolID(const std::string &name, int value)
Add identifier.
PlacedVolume & operator=(PlacedVolume &&v)=default
Assignment operator (must match copy constructor)
const Volume & setOption(const std::string &opt) const
Set the volume's option value.
Solid solid() const
Access to Solid (Shape)
Handle: a templated class like a shared pointer, which allows specialized access to tgeometry objects...
const Volume & setRegion(const Detector &description, const std::string &name) const
Set the regional attributes to the volume. Note: If the name string is empty, the action is ignored.
PlacedVolumeExtension Object
ROOT::Math::Rotation3D Rotation3D
VolIDs volIDs
ID container.
Dimension trafo2D
Reference to the parameterised transformation for dimension 2.
Parameterisation & operator=(const Parameterisation ©)=default
Assignment operator.
PlacedVolume(PlacedVolume &&e)=default
Move constructor.
Base class for Solid (shape) objects.
PlacedVolume paramVolume1D(const Transform3D &start, Volume entity, size_t count, const Transform3D &inc)
1D Parameterised volume implementation
iterator insert(std::vector< VolID >::const_iterator pos, InputIt first, InputIt last)
Insert bunch of entries.
bool isSensitive() const
Accessor if volume is sensitive (ie. is attached to a sensitive detector)
bool operator!=(const Handle< T > &e) const
Non-Equality operator.
unsigned long magic
Magic word to detect memory corruptions.
const char * name() const
Access the object name (or "" if not supported by the object)
VisAttr vis
Reference to visualization attributes.
Implementation class extending the ROOT assembly volumes (TGeoVolumeAssembly)
virtual int processPlacement(PlacedVolume pv)=0
Container callback for object processing.
PlacedVolume placeVolume(const Volume &volume) const
Place daughter volume. The position and rotation are the identity.
Parameterisation * addref()
Increase ref count.
Volume(const TGeoVolume *v)
Copy from handle.
Volume(const Volume &v)=default
Copy from handle.
Volume & operator=(const Volume &a)=default
Assignment operator (must match copy constructor)
long refCount
Reference count on object (used to implement Grab/Release)
VolumeMulti(const VolumeMulti &v)=default
Copy from handle.
VolumeExtension(VolumeExtension &©)=delete
No move.
Material material() const
Access to the Volume material.
ClassDef(Parameterisation, 200)
Enable ROOT persistency.
void release()
Decrease ref count.
Handle class describing a material.
VolIDs & operator=(const VolIDs &c)=default
Assignment operator.
std::string option() const
Access the volume's option value.
PlacedVolume daughter(std::size_t which) const
Access the daughter by index.
Volume(const Handle< T > &v)
Copy from arbitrary Element.
Volume & operator=(Volume &&a)=default
Assignment operator (must match move constructor)
PlacedVolume(const PlacedVolume &e)=default
Copy assignment.
Processor()
Default constructor.
Handle class holding a placed volume (also called physical volume)
const Volume & setSensitiveDetector(const SensitiveDetector &obj) const
Assign the sensitive detector structure.
PlacedVolume(const TGeoNode *e)
Constructor taking implementation object pointer.
bool operator==(const Handle< T > &e) const
Equality operator.
bool operator==(const Handle< T > &e) const
Equality operator.
Parameterisation & operator=(Parameterisation &©)=default
Move assignment.
const Volume & setSolid(const Solid &s) const
Set the volume's solid shape.
void copy(const VolumeExtension &c)
Copy the object.
Handle class describing a set of limits as they are used for simulation.
Material material() const
Volume material.
LimitSet limitSet() const
Access to the limit set.
PlacedVolume replicate(const Volume entity, ReplicationAxis axis, size_t count, double inc, double start=0e0)
1D volume replication implementation
Region region() const
Access to the handle to the region structure.
Handle< NamedObject > sens_det
Reference to the sensitive detector.
int flags
Bit field to determine the usage. Bit 0...15 reserverd for system usage. 16...31 user space.
Assembly(const Handle< T > &v)
Copy from arbitrary Element.
void addProperty(const std::string &nam, const std::string &val) const
Add Volume property (name-value pair)
const Volume & setMaterial(const Material &m) const
Set the volume's material.
PlacedVolume paramVolume3D(Volume entity, size_t count_1, const Transform3D &inc_1, size_t count_2, const Transform3D &inc_2, size_t count_3, const Transform3D &inc_3)
3D Parameterised volume implementation
bool testFlagBit(unsigned int bit) const
Test the user flag bit.
iterator insert(InputIt first, InputIt last)
Insert bunch of entries.
VolIDs(const VolIDs ©)=default
Copy constructor.
unsigned long flags
Number of entries for the parameterisation in dimension 2.
Handle class describing a region as used in simulation.
bool operator!=(const Handle< T > &e) const
Non-Equality operator.
Parameterisation(const Parameterisation &c)=default
Copy constructor.
TGeoVolume * m_element
Single and only data member: Reference to the actual element.
unsigned char smartlessValue() const
access the smartless option for G4 voxelization
PlacedVolume()=default
Default constructor.
ClassDefOverride(VolumeExtension, 200)
Enable ROOT persistency.
const TGeoMatrix & matrix() const
Access the full transformation matrix to the parent volume.
PlacedVolumeExtension::VolIDs VolIDs
virtual TGeoExtension * Grab() override
TGeoExtension overload: Method called whenever requiring a pointer to the extension.
void execute() const
Perform scan.
Volume motherVol() const
Parent volume (envelope)
Scan geometry and create reflected volumes.
std::string toString() const
String dump.
Dimension trafo1D
Reference to the parameterised transformation for dimension 1.
PlacedVolumeExtension()
Default constructor.
Parameterisation()=default
Default constructor.
ReflectionBuilder(Detector &desc)
Initializing constructor.
Volume()=default
Default constructor.
std::pair< Transform3D, size_t > Dimension
ROOT::Math::Transform3D Transform3D
VolumeMulti(const Handle< T > &v)
Copy from arbitrary Element.
PlacedVolume(const Handle< T > &e)
Copy assignment from other handle type.
std::size_t num_daughters() const
Number of daughters placed in this volume.
Volume divide(const std::string &divname, int iaxis, int ndiv, double start, double step, int numed=0, const char *option="")
Divide volume into subsections (See the ROOT manual for details)
unsigned char smartLess
Geant4 optimization flag: Smartless.
ROOT::Math::XYZVector Position
std::pair< std::string, int > VolID
VolumeExtension()
Default constructor.
unsigned long magic
Magic word to detect memory corruptions.
bool isReflected() const
Test if this volume was reflected.
const char * type() const
Access the object type from the class information.
Class describing a box shape.
std::vector< VolID > Base
TGeoNode * ptr() const
Access to the held object.
virtual TGeoExtension * Grab() override
TGeoExtension overload: Method called whenever requiring a pointer to the extension.
const char * type() const
Access the object type from the class information.
Box boundingBox() const
Access the bounding box of the volume (if available)
VolumeExtension & operator=(VolumeExtension &©)=delete
No move assignment.
void verifyVolumeMulti()
Import volume from pointer as a result of Solid->Divide()
const Volume & setVisAttributes(const VisAttr &obj) const
Set Visualization attributes to the volume.
Namespace for the AIDA detector description toolkit.
std::string str() const
String representation for debugging.
VolIDs()=default
Default constructor.
VolumeMulti(TGeoVolume *v)
Copy from pointer as a result of Solid->Divide()
int copyNumber() const
Access the copy number of this placement within its mother.
virtual ~Processor()
Default destructor.
std::vector< PlacedVolume > placements
Reference to the placements of this volume.
Volume volume() const
Logical volume of this placement.
std::pair< std::vector< VolID >::iterator, bool > insert(const std::string &name, int value)
Insert new entry.
Object * data() const
Check if placement is properly instrumented.
The main interface to the dd4hep detector description package.
Parameterisation(Parameterisation &©)=default
Default move.
std::string getProperty(const std::string &nam, const std::string &default_val="") const
Access property value. Returns default_value if the property is not present.
const detail::BitFieldElement * field
Bitfield from sensitive detector to encode the volume ID on the fly.
Volume reflect() const
Create a reflected volume tree. The reflected volume has left-handed coordinates.
virtual ~VolumeExtension()
Default destructor.
void setFlagBit(unsigned int bit)
Set user flags in bit-field.
Class of the ROOT toolkit. See http://root.cern.ch/root/htmldoc/ClassIndex.html.
long refCount
Reference count on object (used to implement Grab/Release)
ClassDefOverride(PlacedVolumeExtension, 200)
Enable ROOT persistency.
const PlacedVolumeExtension::VolIDs & volIDs() const
Access to the volume IDs.
ROOT::Math::RotationZYX RotationZYX
VolumeMulti & operator=(const VolumeMulti &a)=default
Assignment operator (must match copy constructor)
const Volume & setAttributes(const Detector &description, const std::string ®ion, const std::string &limits, const std::string &vis) const
Attach attributes to the volume.
TList * properties
Reference to properties.
Transform3D start
Reference to the starting point of the parameterisation.
VolIDs(VolIDs &©)=default
Move constructor.
VisAttr visAttributes() const
Access the visualisation attributes.
Parameterisation * params
Reference to the parameterised transformation.
Position position() const
Access the translation vector to the parent volume.
Optional parameters to implement special features such as parametrization.
std::vector< VolID >::const_iterator find(const std::string &name) const
Find entry.
std::string toStringMesh(const TGeoShape *shape, int precision=2)
Output mesh vertices to string.
Object * data() const
Check if placement is properly instrumented.
PlacedVolumeExtension & operator=(PlacedVolumeExtension &©)
Move assignment.
PlacedVolume & operator=(const PlacedVolume &v)=default
Assignment operator (must match copy constructor)
Handle< NamedObject > sensitiveDetector() const
Access to the handle to the sensitive detector.
LimitSet limits
Limit sets used for simulation.