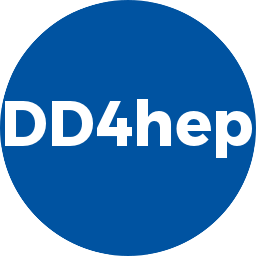 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
19 #include <DD4hep/detail/Handle.inl>
33 std::string seg_type =
"segmentation_constructor__"+typ;
37 if ( !nam.empty() ) obj->
setName(nam);
41 except(
"Segmentation",
"FAILED to create segmentation: %s. [Missing factory]",typ.c_str());
DDSegmentation::Parameters parameters() const
Access to all parameters.
Position position(const CellID &cellID) const
determine the local position based on the cell ID
A segmentation for arbitrary sizes in R and R-dependent sizes in Phi.
Segmentation base class describing cartesian grid segmentation in the X-Y plane.
bool useForHitPosition() const
Access flag for hit positioning.
DDSegmentation::Segmentation * segmentation
Reference to base segmentation.
Handle< SensitiveDetectorObject > sensitive
Reference to hosting top level sensitve detector structure.
Handle< SensitiveDetectorObject > sensitive() const
Access the sensitive detector using this segmentation object.
Handle: a templated class like a shared pointer, which allows specialized access to tgeometry objects...
Handle< DetElementObject > detector() const
Access the main detector element using this segmentation object.
Implementation class supporting generic Segmentation of sensitive detectors.
Class to hold a segmentation parameter with its description.
bool cellsSpanVolumes() const
Segmentation base class describing cartesian grid segmentation in the X-Z plane.
virtual bool cellsSpanVolumes() const
Wrapper to support multiple segmentations.
unsigned char useForHitPosition
Flag to use segmentation for hit positioning.
Segmentation base class describing cartesian strip segmentation in Z.
std::vector< double > cellDimensions(const CellID &cellID) const
Returns a vector<double> of the cellDimensions of the given cell ID in natural order of dimensions,...
void setDecoder(const BitFieldCoder *decoder) const
Set the underlying decoder.
void assign(Object *n, const std::string &nam, const std::string &title)
Assign a new named object. Note: object references must be managed by the user.
DD4HEP_INSTANTIATE_SEGMENTATION_HANDLE(DDSegmentation::NoSegmentation)
std::vector< Parameter > Parameters
void neighbours(const CellID &cellID, std::set< CellID > &neighbours) const
Calculates the neighbours of the given cell ID and adds them to the list of neighbours.
A segmentation for arbitrary sizes in R and R-dependent sizes in Phi.
a megatile is a rectangule in x-y, split into a grid along x and y, with an exactly integer number of...
virtual const BitFieldCoder * decoder() const
Access the underlying decoder.
Segmentation base class describing cylindrical grid segmentation in the Phi-Z cylinder.
Segmentation base class describing cartesian grid segmentation in space.
A segmentation class to describe projective cylinders.
Handle< DetElementObject > detector
Reference to hosting top level DetElement structure.
const std::string & type() const
Access the segmentation type.
CellID cellID(const Position &localPosition, const Position &globalPosition, const VolumeID &volumeID) const
determine the cell ID based on the local position
const std::string & name() const
Access the segmentation name.
Segmentation base class describing cartesian strip segmentation in Y.
VolumeID volumeID(const CellID &cellID) const
Determine the volume ID from the full cell ID by removing all local fields.
DD4HEP_INSTANTIATE_HANDLE_UNNAMED(SegmentationObject)
virtual void setDecoder(const BitFieldCoder *decoder)
Set the underlying decoder.
const char * name() const
Accessor: Segmentation type.
ROOT::Math::XYZVector Position
std::string type() const
Accessor: Segmentation type.
virtual VolumeID volumeID(const CellID &cellID) const
Determine the volume ID from the full cell ID by removing all local fields.
Segmentation base class describing cartesian grid segmentation in along U,V rotated some angle from l...
This class exists to provide a segmenation when it is need but doesn't exist.
dd4hep::DDSegmentation::VolumeID VolumeID
virtual CellID cellID(const Vector3D &localPosition, const Vector3D &globalPosition, const VolumeID &volumeID) const =0
Determine the cell ID based on the position.
DDSegmentation::Segmentation * segmentation() const
Access to the base DDSegmentation object. WARNING: Deprecated call!
SegmentationObject * access() const
Checked object access. Throws invalid handle runtime exception if invalid handle.
Segmentation base class describing cartesian grid segmentation in the Y-Z plane.
const BitFieldCoder * decoder() const
Access the underlying decoder.
DDSegmentation::Parameters parameters() const
Access to the parameters.
Namespace for the AIDA detector description toolkit.
virtual std::vector< double > cellDimensions(const CellID &cellID) const
Returns a vector<double> of the cellDimensions of the given cell ID in natural order of dimensions,...
Segmentation class describing segmentation in R-Phi-Eta.
virtual void neighbours(const CellID &cellID, std::set< CellID > &neighbours) const
Calculates the neighbours of the given cell ID and adds them to the list of neighbours.
Segmentation()=default
Default constructor.
Segmentation class describing segmentation in Phi-Eta.
Segmentation base class describing cartesian strip segmentation in X.
DDSegmentation::Parameter parameter(const std::string ¶meterName) const
Access to parameter by name.
A segmentation class to describe tiled layers.
Base class for all segmentations.
A segmentation class to describe wafer grids in X-Y.
DDSegmentation::Parameter parameter(const std::string ¶meterName) const
Access to parameter by name.
Segmentation base class describing cartesian grid segmentation.
virtual Vector3D position(const CellID &cellID) const =0
Determine the local position based on the cell ID.
void setName(const std::string &value)
Set the segmentation name.
dd4hep::DDSegmentation::CellID CellID