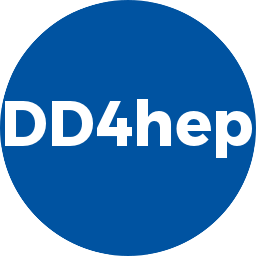 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
18 #include <DD4hep/detail/Handle.inl>
28 : name(c.name),
key(c.
key), key_min(c.key_min), key_max(c.key_max)
34 : name(n),
key(k), key_min(k_min), key_max(k_max)
57 Object& ro = object<Object>();
58 return ro.
hits.size();
60 except(
"dd4hep::Readout",
"numCollections: Cannot access object data [Invalid Handle]");
61 throw std::runtime_error(
"dd4hep: Readout");
66 std::vector<std::string> colls;
68 Object& ro = object<Object>();
69 if ( !ro.
hits.empty() ) {
70 for(
const auto& hit : ro.
hits )
71 colls.emplace_back(hit.name);
75 except(
"dd4hep::Readout",
"collectionsNames: Cannot access object data [Invalid Handle]");
76 throw std::runtime_error(
"dd4hep: Readout");
81 std::vector<const HitCollection*> colls;
83 Object& ro = object<Object>();
84 if ( !ro.
hits.empty() ) {
85 for(
const auto& hit : ro.
hits )
86 colls.emplace_back(&hit);
90 except(
"dd4hep::Readout",
"collections: Cannot access object data [Invalid Handle]");
91 throw std::runtime_error(
"dd4hep: Readout");
98 data<Object>()->id = new_descriptor;
107 except(
"dd4hep::Readout",
"setIDDescriptor: Cannot assign ID descriptor [Invalid Handle]");
108 throw std::runtime_error(
"dd4hep: Readout");
113 return object<Object>().id;
119 Object& ro = object<Object>();
121 if ( e && e != seg.
ptr() ) {
129 except(
"dd4hep::Readout",
"setSegmentation: Cannot assign segmentation [Invalid Handle]");
130 throw std::runtime_error(
"dd4hep: Readout");
std::string name
Hit collection name.
long key_min
Range values of the key is not empty.
Segmentation segmentation
Handle to the readout segmentation.
Definition of the HitCollection parameters used by the Readout.
Concrete object implementation of the Readout Handle.
void setSegmentation(const Segmentation &segment) const
Assign segmentation structure to readout.
std::string key
Discriminator key name from the <id> string.
Class implementing the ID encoding of the detector response.
bool isValid() const
Check the validity of the object held by the handle.
Implementation class supporting generic Segmentation of sensitive detectors.
std::vector< const HitCollection * > collections() const
Access hit collections if present.
std::vector< std::string > collectionNames() const
Access explicit names of hit collections if present.
HitCollection()
Default constructor.
void setDecoder(const BitFieldCoder *decoder) const
Set the underlying decoder.
void assign(Object *n, const std::string &nam, const std::string &title)
Assign a new named object. Note: object references must be managed by the user.
Segmentation segmentation() const
Access segmentation structure.
void setIDDescriptor(const Ref_t &spec) const
Assign IDDescription to readout structure.
DDSegmentation::Segmentation * segmentation() const
Access to the base DDSegmentation object. WARNING: Deprecated call!
T * ptr() const
Access to the held object.
Namespace for the AIDA detector description toolkit.
HitCollection & operator=(const HitCollection &c)
Assignment operator.
size_t numCollections() const
Access number of hit collections.
Handle class supporting generic Segmentations of sensitive detectors.
IDDescriptor idSpec() const
Access IDDescription structure.
Readout()=default
Default constructor.
std::vector< HitCollection > hits
Hit collection container (if defined)