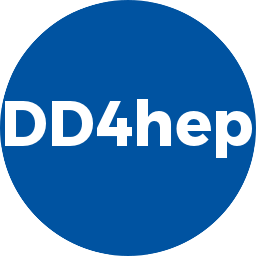 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
19 #ifndef DDSEGMENTATION_SEGMENTATION_H
20 #define DDSEGMENTATION_SEGMENTATION_H
33 namespace DDSegmentation {
50 Vector3D(
double x_val = 0.,
double y_val = 0.,
double z_val = 0.) :
51 X(x_val),
Y(y_val),
Z(z_val) {
96 virtual const std::string&
name()
const {
100 virtual void setName(
const std::string& value) {
104 virtual const std::string&
type()
const {
148 bool isOpt =
false) {
152 void registerIdentifier(
const std::string& nam,
const std::string& desc, std::string& ident,
153 const std::string& defaultVal);
161 static double binToPosition(
FieldID bin, std::vector<double>
const& cellBoundaries,
double offset = 0.);
163 static int positionToBin(
double position, std::vector<double>
const& cellBoundaries,
double offset = 0.);
186 #endif // DDSEGMENTATION_SEGMENTATION_H
std::string _name
The segmentation name.
std::map< std::string, Parameter > _parameters
The parameters for this segmentation.
const BitFieldCoder * _decoder
The cell ID encoder and decoder.
SegmentationParameter * Parameter
Simple container for a physics vector.
Helper class for decoding and encoding a bit field of 64bits for convenient declaration.
virtual ~Segmentation()
Destructor.
TypedSegmentationParameter< std::string > * StringParameter
TypedSegmentationParameter< std::vector< int > > * IntVecParameter
TypedSegmentationParameter< std::vector< std::string > > * StringVecParameter
TypedSegmentationParameter< double > * DoubleParameter
Class to hold a segmentation parameter with its description.
virtual bool cellsSpanVolumes() const
bool _ownsDecoder
Keeps track of the decoder ownership.
virtual const std::string & name() const
Access the segmentation name.
Vector3D(double x_val=0., double y_val=0., double z_val=0.)
Default constructor.
TypedSegmentationParameter< float > * FloatParameter
virtual Parameters parameters() const
Access to all parameters.
Vector3D(const T &v)
Constructor using a foreign vector class. Requires methods x(), y() and z()
void registerParameter(const std::string &nam, const std::string &desc, TYPE ¶m, const TYPE &defaultVal, UnitType unitTyp=SegmentationParameter::NoUnit, bool isOpt=false)
Add a parameter to this segmentation. Used by derived classes to define their parameters.
virtual Parameter parameter(const std::string ¶meterName) const
Access to parameter by name.
std::vector< Parameter > Parameters
virtual const BitFieldCoder * decoder() const
Access the underlying decoder.
TypedSegmentationParameter< std::vector< float > > * FloatVecParameter
TypedSegmentationParameter< std::vector< double > > * DoubleVecParameter
void registerIdentifier(const std::string &nam, const std::string &desc, std::string &ident, const std::string &defaultVal)
Add a cell identifier to this segmentation. Used by derived classes to define their required identifi...
virtual void setDecoder(const BitFieldCoder *decoder)
Set the underlying decoder.
std::string _description
The description of the segmentation.
virtual const std::string & description() const
Access the description of the segmentation.
virtual VolumeID volumeID(const CellID &cellID) const
Determine the volume ID from the full cell ID by removing all local fields.
static int positionToBin(double position, double cellSize, double offset=0.)
Helper method to convert a 1D position to a cell ID.
Segmentation(const std::string &cellEncoding="")
Default constructor used by derived classes passing the encoding string.
virtual CellID cellID(const Vector3D &localPosition, const Vector3D &globalPosition, const VolumeID &volumeID) const =0
Determine the cell ID based on the position.
TypedSegmentationParameter< int > * IntParameter
SegmentationParameter::UnitType UnitType
static double binToPosition(FieldID bin, double cellSize, double offset=0.)
Helper method to convert a bin number to a 1D position.
double z() const
Access to z value (required for use with ROOT GenVector)
Namespace for the AIDA detector description toolkit.
Concrete class to hold a segmentation parameter of a given type with its description.
virtual std::vector< double > cellDimensions(const CellID &cellID) const
Returns a vector<double> of the cellDimensions of the given cell ID in natural order of dimensions,...
std::string fieldDescription() const
std::string _type
The segmentation type.
virtual void neighbours(const CellID &cellID, std::set< CellID > &neighbours) const
Calculates the neighbours of the given cell ID and adds them to the list of neighbours.
virtual std::string fieldDescription() const
Access the encoding string.
virtual void setName(const std::string &value)
Set the segmentation name.
double y() const
Access to y value (required for use with ROOT GenVector)
Segmentation(const Segmentation &)
No copy constructor allowed.
std::map< std::string, StringParameter > _indexIdentifiers
The indices used for the encoding.
virtual void addSubsegmentation(long key_min, long key_max, Segmentation *entry)
Add subsegmentation. Call only valid for Multi-segmentations. Default implementation throws an except...
virtual void setParameters(const Parameters ¶meters)
Set all parameters from an existing set of parameters.
UnitType
Defines the parameter unit type (useful to convert to default set of units)
Base class for all segmentations.
double x() const
Access to x value (required for use with ROOT GenVector)
virtual Vector3D position(const CellID &cellID) const =0
Determine the local position based on the cell ID.
virtual const std::string & type() const
Access the segmentation type.