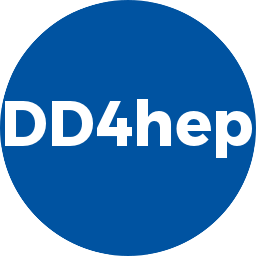 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_SEGMENTATIONS_H
14 #define DD4HEP_SEGMENTATIONS_H
26 class DetElementObject;
27 class SegmentationObject;
28 class SensitiveDetectorObject;
29 template <
typename T>
class SegmentationWrapper;
66 const char*
name()
const;
68 std::string
type()
const;
78 const BitFieldCoder*
decoder()
const;
106 #endif // DD4HEP_SEGMENTATIONS_H
Position position(const CellID &cellID) const
determine the local position based on the cell ID
SegmentationObject Object
Extern accessible definition of the contained element type.
bool useForHitPosition() const
Access flag for hit positioning.
Handle< SensitiveDetectorObject > sensitive() const
Access the sensitive detector using this segmentation object.
Handle: a templated class like a shared pointer, which allows specialized access to tgeometry objects...
Handle< DetElementObject > detector() const
Access the main detector element using this segmentation object.
Implementation class supporting generic Segmentation of sensitive detectors.
Class to hold a segmentation parameter with its description.
bool cellsSpanVolumes() const
std::vector< double > cellDimensions(const CellID &cellID) const
Returns a vector<double> of the cellDimensions of the given cell ID in natural order of dimensions,...
void setDecoder(const BitFieldCoder *decoder) const
Set the underlying decoder.
std::vector< Parameter > Parameters
void neighbours(const CellID &cellID, std::set< CellID > &neighbours) const
Calculates the neighbours of the given cell ID and adds them to the list of neighbours.
Segmentation(const Handle< Object > &e)
Copy Constructor from handle.
CellID cellID(const Position &localPosition, const Position &globalPosition, const VolumeID &volumeID) const
determine the cell ID based on the local position
VolumeID volumeID(const CellID &cellID) const
Determine the volume ID from the full cell ID by removing all local fields.
Segmentation(const Segmentation &e)=default
Copy Constructor.
Segmentation(Segmentation &&e)=default
Move Constructor.
const char * name() const
Accessor: Segmentation type.
ROOT::Math::XYZVector Position
std::string type() const
Accessor: Segmentation type.
dd4hep::DDSegmentation::VolumeID VolumeID
DDSegmentation::Segmentation * segmentation() const
Access to the base DDSegmentation object. WARNING: Deprecated call!
Segmentation & operator=(const Segmentation &seg)=default
Copy Assignment operator.
const BitFieldCoder * decoder() const
Access the underlying decoder.
DDSegmentation::Parameters parameters() const
Access to the parameters.
Namespace for the AIDA detector description toolkit.
Segmentation & operator=(Segmentation &&seg)=default
Move Assignment operator.
Segmentation()=default
Default constructor.
Handle class supporting generic Segmentations of sensitive detectors.
DDSegmentation::Parameter parameter(const std::string ¶meterName) const
Access to parameter by name.
Base class for all segmentations.
dd4hep::DDSegmentation::CellID CellID