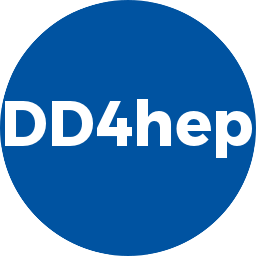 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
14 #ifndef DD4HEP_DETECTORDATA_H
15 #define DD4HEP_DETECTORDATA_H
49 :
std::runtime_error(
"dd4hep: " + msg) {
71 std::string n = e.
name();
72 std::pair<iterator, bool> r = this->emplace(n, e.
ptr());
73 if (!throw_on_doubles || r.second) {
75 printout(WARNING,
"Detector",
76 "+++ Object '%s' is already defined. New value will be ignored",
87 T* obj =
dynamic_cast<T*
>(e.
ptr());
89 this->
append(e, throw_on_doubles);
222 #endif // DD4HEP_DETECTORDATA_H
State
The detector description states.
ObjectHandleMap m_sensitive
The map of top level sub-detector sensitive detector objects indexed by the detector name.
std::map< std::string, DetElement > m_detectorParents
ObjectHandleMap m_display
The map of display attributes in use.
dd4hep::VolumeManager volumeManager() const
Return handle to the VolumeManager.
void clearData()
Clear data content: DOES NOT RELEASEW ALLOCATED RESOURCES!
TGeoManager & manager() const
Access the geometry manager of this instance.
Implementation of an object supporting arbitrary user extensions.
void append(const Handle< NamedObject > &e, bool throw_on_doubles=true)
Append entry to map.
std::map< std::string, PropertyValues > Properties
Handle class describing visualization attributes.
dd4hep::Volume trackingVolume() const
Return handle to the world volume containing the volume with the tracking devices.
bool isValid() const
Check the validity of the object held by the handle.
dd4hep::Material vacuum() const
Return handle to material describing vacuum.
const char * name() const
Access the object name (or "" if not supported by the object)
ObjectHandleMap m_idDict
Map of readout IDDescriptors indexed by hit collection name.
DetectorData()
Default constructor.
static void patchRootStreamer(TClass *cl)
Assignment operator.
bool m_inhibitConstants
Flag to inhibit the access to global constants. Value set by constants section 'Detector_InhibitConst...
dd4hep::Material air() const
Return handle to material describing air.
Specialized exception class.
Class describing a field overlay with several sources.
const Detector::HandleMap & fields() const
Accessor to the map of field entries, which together form the global field.
Handle class describing a material.
ObjectHandleMap m_regions
Map of regions settings for the simulation.
Handle class describing a detector element.
const Detector::HandleMap & visAttributes() const
Accessor to the map of visualisation attributes.
const Detector::HandleMap & idSpecifications() const
Accessor to the map of ID specifications.
Handle class holding a placed volume (also called physical volume)
DetectorData(const DetectorData ©)=delete
Copy constructor.
Class to support the retrieval of detector elements and volumes given a valid identifier.
const Detector::HandleMap & constants() const
Accessor to the map of constants.
DetectorBuildType m_buildType
Detector::State m_state
Detector description state.
VolumeManager m_volManager
Volume manager reference.
ObjectHandleMap m_readouts
Map of readout descriptors indexed by subdetector name.
dd4hep::DetElement trackers() const
Return reference to detector element with all tracker devices.
ObjectHandleMap m_detectors
The map of top level sub-detector objects indexed by name.
dd4hep::OverlayedField field() const
Return handle to the combined electromagentic field description.
void destroyData(bool destroy_mgr=true)
Clear data content: releases all allocated resources.
DetectorData(DetectorData &©)=delete
Move constructor.
ObjectHandleMap()=default
Default constructor.
Detector::Properties m_properties
ObjectHandleMap m_fields
The map of electro magnet field components for the global overlay field.
virtual ~DetectorData()
Default destructor.
dd4hep::Volume worldVolume() const
Return handle to the world volume containing everything.
std::map< std::string, Handle< NamedObject > > HandleMap
Type definition of a map of named handles.
@ NOT_READY
The detector description object is freshly created. No geometry nothing.
Implementation of a map of named dd4hep Handles.
InvalidObjectError(const std::string &msg)
Volume m_parallelWorldVol
T * ptr() const
Access to the held object.
Material m_materialVacuum
void adoptData(DetectorData &source, bool CLR=true)
Adopt all data from source structure.
ObjectExtensions m_extensions
Definition of the extension type.
DetectorBuildType
Detector description build types.
Namespace for the AIDA detector description toolkit.
Data implementation class of the Detector interface.
dd4hep::VisAttr invisible() const
Return handle to "invisible" visualization attributes.
dd4hep::Header header() const
Accessor to the header entry.
TGeoManager * m_manager
Reference to the geometry manager object from ROOT.
dd4hep::Volume parallelWorldVolume() const
Return handle to the world volume containing the volume with the tracking devices.
const Detector::HandleMap & limitsets() const
Accessor to the map of limit settings.
std::string name
Name the map.
static void unpatchRootStreamer(TClass *cl)
UNPatch the ROOT streamers to adapt for DD4hep (set fUserExtension transient)
ObjectHandleMap m_limits
Map of limit sets.
const Detector::HandleMap & regions() const
Accessor to the map of region settings.
const Detector::HandleMap & detectors() const
Accessor to the map of sub-detectors.
void append(const Handle< NamedObject > &e, bool throw_on_doubles=true)
Append entry to map.
dd4hep::DetElement world() const
Return reference to the top-most (world) detector element.
const Detector::HandleMap & sensitiveDetectors() const
Retrieve a sensitive detector by its name from the detector description.
const Detector::HandleMap & readouts() const
Accessor to the map of readout structures.