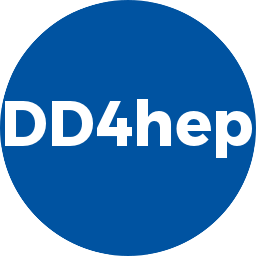 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
14 #ifndef DD4HEP_VOLUMEMANAGER_H
15 #define DD4HEP_VOLUMEMANAGER_H
25 #include <TGeoMatrix.h>
32 class VolumeManagerObject;
100 void worldToLocal(
const double world[3],
double local[3])
const;
164 template <
typename Q>
172 const std::string&
name,
221 #endif // DD4HEP_VOLUMEMANAGER_H
Position worldToElement(const Position &world) const
Transform world coordinates to the DetElement coordinates.
DetElement lookupDetElement(VolumeID volume_id) const
Convenience routine: Lookup the closest subdetector detector element in the hierarchy according to a ...
VolumeManagerContext * lookupContext(VolumeID volume_id) const
Lookup the context, which belongs to a registered physical volume.
VolumeManagerContext & operator=(const VolumeManagerContext ©)=delete
Inhibit assignment.
bool adoptPlacement(VolumeManagerContext *context)
Register physical volume with the manager (normally: section manager)
Handle class holding a placed volume (also called physical volume)
VolumeManagerContext(VolumeManagerContext &©)=delete
Inhibit move constructor.
Class implementing the ID encoding of the detector response.
DetElement element
Handle to the closest Detector element.
const TGeoMatrix & worldTransformation(const ConditionsMap &map, VolumeID volume_id) const
Convenience routine: Access the transformation of a physical volume to the world coordinate system.
Handle: a templated class like a shared pointer, which allows specialized access to tgeometry objects...
DetElement detector() const
Access the top level detector element.
PlacedVolume volumePlacement() const
Acces the sensitive volume placement.
const char * name() const
Access the object name (or "" if not supported by the object)
This structure describes the internal data of the volume manager object.
std::ostream & operator<<(std::ostream &os, const DetType &t)
VolumeManager()=default
Default constructor.
VolumeID mask
Ignore mask of the placement identifier.
virtual ~VolumeManagerContext()
Default destructor.
PlacedVolume lookupVolumePlacement(VolumeID volume_id) const
Lookup a physical (placed) volume identified by its 64 bit hit ID.
Handle class describing a detector element.
Class to support the retrieval of detector elements and volumes given a valid identifier.
DetElement lookupDetector(VolumeID volume_id) const
Convenience routine: Lookup a top level subdetector detector element according to a contained 64 bit ...
static VolumeManager getVolumeManager(const Detector &description)
static accessor calling DD4hepVolumeManagerPlugin if necessary
VolumeManager subdetector(VolumeID id) const
Access the volume manager by cell id.
VolumeManager(const Handle< Q > &e)
Constructor to be used when reading the already parsed object.
Position localToElement(const double local[3]) const
Transform local coordinates to the DetElement coordinates.
const TGeoHMatrix & toElement() const
Access the transformation to the closest detector element.
VolumeManager addSubdetector(DetElement detector, Readout ro)
Add a new Volume manager section according to a new subdetector.
PlacedVolume lookupDetElementPlacement(VolumeID volume_id) const
Lookup a physical (placed) volume of the detector element containing a volume identified by its 64 bi...
VolumeManagerContext & operator=(VolumeManagerContext &©)=delete
Inhibit move assignment.
long flag
Flag to indicate optional information.
ROOT::Math::XYZVector Position
VolumeManager & operator=(const VolumeManager &m)=default
Assignment operator.
dd4hep::DDSegmentation::VolumeID VolumeID
IDDescriptor idSpec() const
Access IDDescription structure.
detail::VolumeManagerObject * ptr() const
Access to the held object.
Namespace for the AIDA detector description toolkit.
VolumeID identifier
Placement identifier.
Object & _data() const
Additional data accessor.
This structure describes the cached data for one placement held by the volume manager.
The main interface to the dd4hep detector description package.
Handle to the implementation of the readout structure of a subdetector.
Position worldToLocal(const Position &world) const
Transform world coordinates to the local coordinates.
VolumeManagerContext(const VolumeManagerContext ©)=delete
Inhibit copy constructor.
VolumeManagerContext()=default
Default constructor.
VolumeManager(const VolumeManager &e)=default
Constructor to be used when reading the already parsed object.
Position localToWorld(const double local[3]) const
Transform local coordinates to the world coordinates.
PlacedVolume elementPlacement() const
Acces the detector element volume placement.