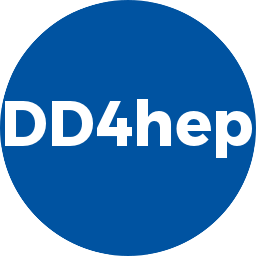 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
16 #include <DD4hep/detail/Handle.inl>
35 for (
size_t i = 0; i < bf.
size(); ++i) {
37 o->fieldIDs.emplace_back(i, f->
name());
38 o->fieldMap.emplace_back(f->
name(), f);
46 assign(obj, nam,
"iddescriptor");
47 _construct(obj, description);
53 std::string dsc = description;
74 return data<Object>()->decoder.highestBit();
80 return data<Object>()->fieldIDs;
82 except(
"IDDescriptor",
"dd4hep: Attempt to access an invalid IDDescriptor object.");
83 throw std::runtime_error(
"dd4hep");
89 return data<Object>()->fieldMap;
91 except(
"IDDescriptor",
"dd4hep: Attempt to access an invalid IDDescriptor object.");
92 throw std::runtime_error(
"dd4hep");
98 for (
const auto& i : fm )
99 if (i.first == field_name)
101 except(
"IDDescriptor",
"dd4hep: %s: This ID descriptor has no field with the name: %s",
102 name(),field_name.c_str());
103 throw std::runtime_error(
"dd4hep");
109 return fm[identifier].second;
115 for (
const auto& i : fm )
116 if (i.second == field_name)
118 except(
"IDDescriptor",
"dd4hep: %s: This ID descriptor has no field with the name: %s",
119 name(),field_name.c_str());
120 throw std::runtime_error(
"dd4hep");
126 for (
const auto& i : id_vector ) {
127 const auto* fld =
field(i.first);
138 for (
const auto& i : id_vector ) {
139 const BitFieldElement* fld =
field(i.first);
140 int off = fld->offset();
142 id |= ((fld->value(val << off) << off)&fld->mask());
150 int off = fld->offset();
151 return ((fld->value(value << off) << off)&fld->mask());
153 except(
"IDDescriptor",
"dd4hep: %s: Cannot encode value with void Field reference.");
160 return detail::reverseBits<VolumeID>(
encode(id_vector));
165 std::vector<std::pair<const BitFieldElement*, VolumeID> >& flds)
const
170 flds.emplace_back(&f, f.value(vid));
176 std::stringstream
str;
178 str << f.name() <<
":" << std::setw(4) << std::setfill(
'0')
179 << std::hex << std::right << f.value(vid)
180 << std::left << std::dec <<
" ";
181 return str.str().substr(0,
str.str().length()-1);
187 std::stringstream
str;
189 if ( 0 == (mask&f.mask()) )
continue;
190 str << f.name() <<
":" << std::setw(4) << std::setfill(
'0')
191 << std::hex << std::right << f.value(vid)
192 << std::left << std::dec <<
" ";
194 return str.str().substr(0,
str.str().length()-1);
199 return &(data<Object>()->decoder);
void decodeFields(VolumeID vid, std::vector< std::pair< const BitFieldElement *, VolumeID > > &fields) const
Decode volume IDs and return filled descriptor with all fields.
size_t fieldID(const std::string &field_name) const
Get the field identifier of one field by name.
Concrete object implementation of the IDDescriptorObject Handle.
Helper class for BitFieldCoder that corresponds to one field value.
BitFieldCoder decoder
Decoder object.
std::vector< std::pair< std::string, const Field * > > FieldMap
IDDescriptorObject Object
Extern accessible definition of the contained element type.
const BitFieldElement * field(const std::string &field_name) const
Get the field descriptor of one field by name.
Helper class for decoding and encoding a bit field of 64bits for convenient declaration.
~BitFieldCoder()=default
Default destructor.
unsigned maxBit() const
The total number of encoding bits for this descriptor.
const char * GetName() const
Access name.
bool isValid() const
Check the validity of the object held by the handle.
const std::vector< BitFieldElement > & fields() const
const char * name() const
Access the object name (or "" if not supported by the object)
IDDescriptor()=default
Default constructor.
const std::string & name() const
void assign(Object *n, const std::string &nam, const std::string &title)
Assign a new named object. Note: object references must be managed by the user.
static VolumeID encode(const Field *fld, VolumeID value)
Encode partial volume identifiers to a volumeID.
std::vector< std::pair< size_t, std::string > > FieldIDs
std::string str(VolumeID vid) const
Decode volume IDs and return string reprensentation for debugging purposes.
IDDescriptorObject * m_element
Single and only data member: Reference to the actual element.
VolumeID get_mask(const std::vector< std::pair< std::string, int > > &id_vector) const
Compute the submask for a given set of volume IDs.
const FieldMap & fields() const
Access the fieldmap container.
dd4hep::DDSegmentation::VolumeID VolumeID
IDDescriptorObject * access() const
Checked object access. Throws invalid handle runtime exception if invalid handle.
IDDescriptorObject * ptr() const
Access to the held object.
std::string fieldDescription() const
The string description of all fields from the BitField.
void rebuild(const std::string &description)
Re-build object in place.
Namespace for the AIDA detector description toolkit.
std::string fieldDescription() const
BitFieldCoder * decoder() const
Access the BitFieldCoder object.
VolumeID encode_reverse(const std::vector< std::pair< std::string, int > > &id_vector) const
Encode a set of volume identifiers to a volumeID with the system ID on the top bits.
std::string toString() const
Access string representation.
const FieldIDs & ids() const
Access the field-id container.