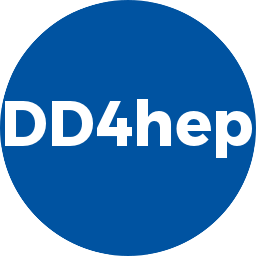 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
24 #include <TGeoMatrix.h>
25 #include <TGeoManager.h>
26 #include <TGeoElement.h>
27 #include <TGeoMaterial.h>
62 Header::Header(
const std::string& author_name,
const std::string& descr_url) {
64 assign(obj_ptr, author_name, descr_url);
89 return data<Object>()->url;
94 data<Object>()->url = new_url;
99 return data<Object>()->author;
104 data<Object>()->author = new_author;
109 return data<Object>()->status;
114 data<Object>()->status = new_status;
119 return data<Object>()->version;
124 data<Object>()->version = new_version;
129 return data<Object>()->comment;
134 data<Object>()->comment = new_comment;
152 throw std::runtime_error(
"dd4hep: Attempt to access internals from invalid Constant handle!");
157 std::stringstream os;
165 Atom::Atom(
const std::string& nam,
const std::string& formula,
int Z,
int N,
double density) {
166 TGeoElementTable* t = TGeoElement::GetElementTable();
167 TGeoElement* e = t->FindElement(nam.c_str());
169 t->AddElement(nam.c_str(), formula.c_str(), Z, N, density);
170 e = t->FindElement(nam.c_str());
179 TGeoMaterial* mat = val->GetMaterial();
182 throw std::runtime_error(
"dd4hep: The medium " + std::string(val->GetName()) +
" has an invalid material reference!");
184 throw std::runtime_error(
"dd4hep: Attempt to access proton number from invalid material handle!");
190 TGeoMaterial* mat =
ptr()->GetMaterial();
193 throw std::runtime_error(
"dd4hep: The medium " + std::string(
ptr()->
GetName()) +
" has an invalid material reference!");
195 throw std::runtime_error(
"dd4hep: Attempt to access atomic number from invalid material handle!");
201 TGeoMaterial* mat =
ptr()->GetMaterial();
203 return mat->GetDensity();
204 throw std::runtime_error(
"dd4hep: The medium " + std::string(
ptr()->
GetName()) +
" has an invalid material reference!");
206 throw std::runtime_error(
"dd4hep: Attempt to access density from invalid material handle!");
212 TGeoMaterial* mat =
ptr()->GetMaterial();
214 return mat->GetRadLen();
215 throw std::runtime_error(
"dd4hep: The medium " + std::string(
ptr()->
GetName()) +
" has an invalid material reference!");
217 throw std::runtime_error(
"dd4hep: Attempt to access radiation length from invalid material handle!");
223 TGeoMaterial* mat =
ptr()->GetMaterial();
225 return mat->GetIntLen();
226 throw std::runtime_error(
"The medium " + std::string(
ptr()->
GetName()) +
" has an invalid material reference!");
228 throw std::runtime_error(
"Attempt to access interaction length from invalid material handle!");
233 double frac = 0e0, tot = 0e0;
234 TGeoElement* elt = atom.
access();
235 TGeoMaterial* mat =
access()->GetMaterial();
236 for (
int i=0, n=mat->GetNelements(); i<n; ++i ) {
237 TGeoElement* e = mat->GetElement(i);
238 if ( mat->IsMixture() ) {
239 TGeoMixture*
mix = (TGeoMixture*)mat;
240 tot +=
mix->GetWmixt()[i];
246 if ( mat->IsMixture() ) {
247 TGeoMixture*
mix = (TGeoMixture*)mat;
248 frac +=
mix->GetWmixt()[i];
255 return tot>1e-20 ? frac/tot : 0.0;
260 return access()->GetMaterial()->GetProperty(nam);
265 return access()->GetMaterial()->GetProperty(nam.c_str());
270 auto* o =
access()->GetMaterial();
271 const char* p = o->GetPropertyRef(
name.c_str());
273 return default_value;
279 auto* o =
access()->GetMaterial();
280 double value = o->GetConstProperty(nam.c_str(), &err);
281 if ( err != kTRUE )
return value;
282 throw std::runtime_error(
"Attempt to access non existing material const property: "+nam);
287 auto* o =
access()->GetMaterial();
288 const char* p = o->GetConstPropertyRef(
name.c_str());
290 return default_value;
296 TGeoMedium* val =
ptr();
297 std::stringstream out;
298 out << val->GetName() <<
" " << val->GetTitle()
299 <<
" id:" << std::hex << val->GetId()
300 <<
" Pointer:" << val->GetPointerName();
303 throw std::runtime_error(
"Attempt to convert invalid material handle to string!");
310 obj->
color = gROOT->GetColor(kWhite);
322 obj->
color = gROOT->GetColor(kWhite);
332 return object<Object>().showDaughters;
337 object<Object>().showDaughters = value;
342 return object<Object>().visible;
347 object<Object>().visible = value;
352 return object<Object>().lineStyle;
357 object<Object>().lineStyle = value;
362 return object<Object>().drawingStyle;
367 object<Object>().drawingStyle = value;
372 return object<Object>().alpha;
377 return object<Object>().color->GetNumber();
382 Object& o = object<Object>();
383 Int_t col = TColor::GetColor(red, green, blue);
385 o.
color = gROOT->GetColor(col);
387 except(
"VisAttr",
"+++ %s Failed to allocate Color: r:%02X g:%02X b:%02X",
388 this->
name(),
int(red*255.),
int(green*255.),
int(blue*255));
390 o.
colortr =
new TColor(gROOT->GetListOfColors()->GetLast()+1,
397 Object& o = object<Object>();
399 o.
color->GetRGB(red, green, blue);
406 bool VisAttr::argb(
float& alpha,
float& red,
float& green,
float& blue)
const {
407 Object& o = object<Object>();
410 o.
color->GetRGB(red, green, blue);
419 TColor* c = obj->
color;
421 std::snprintf(text,
sizeof(text),
"%-20s RGB:%-8s [%d] %7.2f Style:%d %d ShowDaughters:%3s Visible:%3s",
ptr()->
GetName(),
459 std::pair<Object::iterator, bool> ret = data<Object>()->limits.insert(limit);
465 const Object* o = data<Object>();
471 std::pair<Object::iterator, bool> ret = data<Object>()->cuts.insert(cut_obj);
477 return data<Object>()->cuts;
493 object<Object>().store_secondaries = value;
499 object<Object>().was_threshold_set =
true;
504 object<Object>().
cut = value;
505 object<Object>().use_default_cut =
false;
511 return object<Object>().user_limits;
516 return object<Object>().cut;
521 return object<Object>().threshold;
526 return object<Object>().store_secondaries;
530 return object<Object>().use_default_cut;
534 return object<Object>().was_threshold_set;
546 struct IDSpec :
public Ref_t {
548 template <
typename Q>
552 void addField(
const std::string& name,
const std::pair<int,int>& field);
556 : RefElement(doc,Tag_idspec,name)
560 object<Object>().Attr_length = dsc.
maxBit();
561 for(
const auto& i : f ) {
562 const std::string& nam = i.second;
563 const pair<int,int>& fld = m.find(nam)->second;
568 void IDSpec::addField(
const std::string& name,
const pair<int,int>& field) {
569 addField(Strng_t(name),field);
572 void IDSpec::addField(
const std::string& name,
const pair<int,int>& field) {
573 Element e(document(),Tag_idfield);
574 e.object<Object>().Attr_signed = field.second<0;
575 e.object<Object>().Attr_label = name;
576 e.object<Object>().Attr_start = field.first;
577 e.object<Object>().Attr_length = abs(field.second);
void setAuthorEmail(const std::string &addr)
Set the author's email address.
bool operator==(const Limit &c) const
Equality operator.
int color() const
Get object color.
const std::set< Limit > & cuts() const
Accessor to limits container.
Handle class describing an element in the periodic table.
std::string toString() const
String representation of this object.
bool argb(float &alpha, float &red, float &green, float &blue) const
Get alpha and RGB values of the color (if valid)
bool wasThresholdSet() const
Access was_threshold_set flag.
std::vector< std::pair< std::string, const Field * > > FieldMap
HeaderObject Object
Extern accessible definition of the contained element type.
std::string particles
Particle the limit should be applied to.
Property property(const char *name) const
Access to tabular properties of the material.
Author()=default
Default constructor.
const TGDMLMatrix * Property
std::string toString() const
String representation of this object.
Region & setStoreSecondaries(bool value)
Set flag to store secondaries.
bool storeSecondaries() const
Access secondaries flag.
unsigned maxBit() const
The total number of encoding bits for this descriptor.
Region & setCut(double value)
Set default production cut.
const char * GetName() const
Access name.
Region()=default
Default constructor.
const std::set< Limit > & limits() const
Accessor to limits container.
Small object describing a limit structure acting on a particle type.
Class implementing the ID encoding of the detector response.
std::string toString() const
Conversion to a string representation.
void setShowDaughters(bool value)
Set Flag to show/hide daughter elements.
std::string constPropertyRef(const std::string &name, const std::string &default_value="")
Access string property value from the material table.
bool isValid() const
Check the validity of the object held by the handle.
unsigned long long int magic_word()
Access to the magic word, which is protecting some objects against memory corruptions.
void setLineStyle(int style)
Set line style.
std::string propertyRef(const std::string &name, const std::string &default_value="")
Access string property value from the material table.
const char * name() const
Access the object name (or "" if not supported by the object)
std::vector< std::string > & limits() const
Access references to user limits.
double intLength() const
Access the interaction length of the underlying material.
double A() const
atomic number of the underlying material
double fraction(Atom atom) const
Access the fraction of an element within the material.
void SetName(const char *nam)
Set name (used by Handle)
float alpha() const
Get alpha value.
double density() const
density of the underlying material
std::string dataType() const
Access the constant.
int lineStyle() const
Get line style.
int drawingStyle() const
Get drawing style.
bool showDaughters() const
Get Flag to show/hide daughter elements.
void setDrawingStyle(int style)
Set drawing style.
double threshold() const
Access production threshold.
const char * GetName(T *p)
unsigned char showDaughters
void assign(Object *n, const std::string &nam, const std::string &title)
Assign a new named object. Note: object references must be managed by the user.
void setVisible(bool value)
Set visibility flag.
std::string name
Limit name.
void setAuthorName(const std::string &nam)
Set the author's name.
Concrete object implementation of the Region Handle.
double constProperty(const std::string &name) const
Access to tabular properties of the material.
double Z() const
proton number of the underlying material
std::vector< std::pair< size_t, std::string > > FieldIDs
unsigned char drawingStyle
void SetTitle(const char *tit)
Set Title (used by Handle)
std::string dataType
Constant type.
Handle class describing a region as used in simulation.
bool rgb(float &red, float &green, float &blue) const
Get RGB values of the color (if valid)
T * m_element
Single and only data member: Reference to the actual element.
std::string content
Content.
std::string authorName() const
Access the auhor's name.
double value
Double value.
Atom()=default
Default constructor.
const char * GetTitle() const
Get name (used by Handle)
double cut() const
Access cut value.
bool addCut(const Limit &limit)
Add new limit. Returns true if the new limit was added, false if it already existed.
std::string toString() const
String representation of this object.
const FieldMap & fields() const
Access the fieldmap container.
double _toDouble(const std::string &value)
String conversions: string to double value.
bool visible() const
Get visibility flag.
T * access() const
Checked object access. Throws invalid handle runtime exception if invalid handle.
bool operator<(const Limit &c) const
operator less
TGeoMedium * ptr() const
Access to the held object.
bool useDefaultCut() const
Access use_default_cut flag.
Namespace for the AIDA detector description toolkit.
Implementation of a named object.
The main interface to the dd4hep detector description package.
Concrete object implementation of the LimitSet Handle.
double radLength() const
Access the radiation length of the underlying material.
std::set< Limit > limits
Particle specific limits.
void setColor(float alpha, float red, float green, float blue)
Set object color.
Constant()=default
Default constructor.
bool addLimit(const Limit &limit)
Add new limit. Returns true if the new limit was added, false if it already existed.
VisAttr()=default
Default constructor.
std::string authorEmail() const
Access the auhor's email address.
const FieldIDs & ids() const
Access the field-id container.
Concrete object implementation of the VisAttr Handle.
LimitSet()=default
Constructor to be used when reading the already parsed DOM tree.
Region & setThreshold(double value)
Set threshold in MeV.