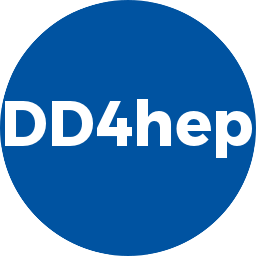 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
83 std::pair<std::string, PlacedVolume>
resolve_path(
const char* vol_path)
const;
84 void printG4(
const std::string& prefix,
const G4VPhysicalVolume* g4pv)
const;
113 #include <TGeoScaledShape.h>
116 #include <G4LogicalVolume.hh>
117 #include <G4PVPlacement.hh>
118 #include <G4Material.hh>
119 #include <G4Version.hh>
120 #include <G4VSolid.hh>
121 #include <CLHEP/Units/SystemOfUnits.h>
123 #ifndef GEANT4_NO_GDML
124 #include <G4GDMLParser.hh>
191 else if ( ::getenv(
"DUMP_GDML") )
writeGDML(::getenv(
"DUMP_GDML"));
195 std::pair<std::string, dd4hep::PlacedVolume>
197 std::string p = vol_path;
208 return make_pair(p,pv);
215 for (
auto it = g4map.begin(); it != g4map.end(); ++it ) {
216 const auto* mat = (*it).second;
217 if ( mat->GetName() == mat_name ) {
218 std::stringstream output;
219 const auto* ion = mat->GetIonisation();
220 printP2(
"+++ Dump of GEANT4 material: %s", mat_name);
224 output << std::setprecision(12);
225 output << ion->GetMeanExcitationEnergy()/CLHEP::eV;
229 output <<
" MEE: UNKNOWN";
230 always(
"+++ printMaterial: \n%s\n", output.str().c_str());
234 warning(
"+++ printMaterial: FAILED to find the material %s", mat_name);
236 warning(
"+++ printMaterial: Property materialName not set!");
243 const G4LogicalVolume* vol = 0;
246 auto vit = g4map.g4Volumes.find(pv.
volume());
247 warning(
"+++ printVolume: %s", vol_path);
248 if ( vit != g4map.g4Volumes.end() ) {
250 auto* sol = vol->GetSolid();
251 const auto* mat = vol->GetMaterial();
252 const auto* ion = mat->GetIonisation();
255 printP2(
"++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++");
256 printP2(
"+++ Dump of GEANT4 solid: %s", vol_path);
258 std::stringstream output;
263 output << std::setprecision(12);
264 output << ion->GetMeanExcitationEnergy()/CLHEP::eV;
268 output <<
" MEE: UNKNOWN";
271 output << std::endl << *sol;
272 printP2(
"%s", output.str().c_str());
273 printP2(
"+++ Dump of ROOT solid: %s", vol_path);
275 if ( sh->IsA() == TGeoScaledShape::Class() ) {
276 TGeoScaledShape* scaled = (TGeoScaledShape*)sh.
ptr();
277 const Double_t* scale = scaled->GetScale()->GetScale();
278 double dot = scale[0]*scale[1]*scale[2];
279 printP2(
"+++ TGeoScaledShape: %8.3g %8.3g %8.3g [%s]", scale[0], scale[1], scale[2],
280 dot > 0e0 ?
"RIGHT handed" :
"LEFT handed");
282 else if ( pit != g4map.g4Placements.end() ) {
283 const G4VPhysicalVolume* pl = (*pit).second;
284 const G4RotationMatrix* rot = pl->GetRotation();
285 const G4ThreeVector& tr = pl->GetTranslation();
286 G4Transform3D transform(rot ? *rot : G4RotationMatrix(), tr);
288 HepGeom::Rotate3D rr;
290 transform.getDecomposition(sc,rr,tt);
291 double dot = sc(0,0)*sc(1,1)*sc(2,2);
292 printP2(
"+++ TGeoShape: %8.3g %8.3g %8.3g [%s]", sc(0,0), sc(1,1), sc(2,2),
293 dot > 0e0 ?
"RIGHT handed" :
"LEFT handed");
295 const TGeoMatrix* matrix = pv->GetMatrix();
297 matrix->TestBit(TGeoMatrix::kGeoReflection) ?
"LEFT handed" :
"RIGHT handed");
298 printP2(
"+++ Shape: %s cubic volume: %8.3g mm^3 area: %8.3g mm^2",
299 sol->GetName().c_str(), sol->GetCubicVolume(), sol->GetSurfaceArea());
304 auto ai = g4map.g4AssemblyVolumes.find(pv.
ptr());
305 if ( ai != g4map.g4AssemblyVolumes.end() ) {
307 warning(
"+++ printVolume: volume %s is an assembly...need to resolve imprint",vol_path);
308 for(Int_t i=0; i <
v->GetNdaughters(); ++i) {
309 TGeoNode* dau_nod =
v->GetNode(i);
310 std::string p = vol_path + std::string(
"/") + dau_nod->GetName();
316 warning(
"+++ printVolume: FAILED to find the volume %s in geant4 mapping...",vol_path);
319 warning(
"+++ printVolume: FAILED to dump invalid volume",vol_path);
327 if ( physVol.second.isValid() ) {
331 warning(
"+++ printVolume: Property VolumePath not set. [Ignored]");
339 if ( pv.isValid() ) {
341 TGeoVolume* vol = pv->GetVolume();
342 for(Int_t i=0; i < vol->GetNdaughters(); ++i) {
344 std::string path = (p +
"/") + dau_pv.
name();
352 warning(
"+++ printVolume: Could not access Volume/DetElement '%s'", vol_path ? vol_path :
"UNKNOWN");
359 if ( pv.isValid() ) {
361 TGeoVolume* vol = pv->GetVolume();
362 for(Int_t i=0; i < vol->GetNdaughters(); ++i) {
364 std::string path = (p +
"/") + dau_pv.
name();
372 warning(
"+++ printVolume: Could not access Volume/DetElement '%s'", vol_path ? vol_path :
"UNKNOWN");
380 auto pv = physVol.second;
381 if ( pv.isValid() ) {
383 auto it = g4map.find(pv.volume());
384 if ( it != g4map.end() ) {
385 const G4LogicalVolume* vol = (*it).second;
386 auto* g4_sol = vol->GetSolid();
387 Box rt_sol = pv.volume().solid();
388 printP2(
"Geant4 Shape: %s cubic volume: %8.3g mm^3 area: %8.3g mm^2",
389 g4_sol->GetName().c_str(), g4_sol->GetCubicVolume(), g4_sol->GetSurfaceArea());
390 #if G4VERSION_NUMBER>=1040
391 G4ThreeVector pMin, pMax;
392 double conv = (dd4hep::centimeter/CLHEP::centimeter)/2.0;
393 g4_sol->BoundingLimits(pMin,pMax);
394 printP2(
"Geant4 Bounding box extends: %8.3g %8.3g %8.3g",
395 (pMax.x()-pMin.x())*conv, (pMax.y()-pMin.y())*conv, (pMax.z()-pMin.z())*conv);
397 printP2(
"ROOT Bounding box dimensions: %8.3g %8.3g %8.3g",
398 rt_sol->GetDX(), rt_sol->GetDY(), rt_sol->GetDZ());
403 warning(
"+++ checkVolume: FAILED to find the volume %s from the top volume",vol_path);
405 warning(
"+++ checkVolume: Property VolumePath not set. [Ignored]");
411 #ifdef GEANT4_NO_GDML
412 warning(
"+++ writeGDML: GDML not found in the present Geant4 build! Output: %s not written", output);
415 if ( output && ::strlen(output) > 0 && output !=
m_dumpGDML.c_str() )
425 const char* gdml_dmp = ::getenv(
"DUMP_GDML");
428 parser.Write(gdml_dmp, w);
429 info(
"+++ writeGDML: Wrote GDML file: %s", gdml_dmp);
433 warning(
"+++ writeGDML: Neither property DumpGDML nor environment DUMP_GDML set. No file written!");
439 std::string path = prefix +
"/";
440 printP2(
"+++ GEANT4 volume: %s", prefix.c_str());
441 auto* g4v = g4pv->GetLogicalVolume();
442 for(
size_t i=0, n=g4v->GetNoDaughters(); i<n; ++i) {
443 auto* dau = g4v->GetDaughter(i);
444 printG4(path + dau->GetName(), dau);
451 if ( pv.isValid() ) {
453 auto it = g4map.find(pv);
454 if ( it != g4map.end() ) {
460 warning(
"+++ printVolume: Could not access Volume/DetElement '%s'", vol_path ? vol_path :
"UNKNOWN");
468 "' [uses argument - or - property DumpGDML]",
470 m_control->
addCall(
"printVolume",
"Print Geant4 volume properties [uses argument]",
472 m_control->
addCall(
"printTree",
"Print volume tree WITHOUT properties [uses argument]",
474 m_control->
addCall(
"printG4Tree",
"Print Geant4 volume tree [uses argument]",
476 m_control->
addCall(
"printVolumeTree",
"Print volume tree with properties [uses argument]",
478 m_control->
addCall(
"checkVolume",
"Check Geant4 volume properties [uses argument]",
480 m_control->
addCall(
"printMaterial",
"Print Geant4 material properties [uses argument]",
Helper to dump Geant4 volume hierarchy.
bool debugRegions
Property: Flag to debug regions during conversion mechanism.
bool m_debugElements
Property: Flag to debug elements during conversion mechanism.
int printVolumeTree(const char *vol_path)
Print volume tree with attributes.
bool debugMaterials
Property: Flag to debug materials during conversion mechanism.
void printP2(const char *fmt,...) const
Support for messages with variable output level using output level+2.
virtual DetElement world() const =0
Return reference to the top-most (world) detector element.
unsigned long m_dumpHierarchy
Property: Dump geometry hierarchy if not NULL. Flags can steer actions.
int printVolTree(const char *vol_path)
Print volume tree WITHOUT attributes.
Geometry converter from dd4hep to Geant 4.
void setWorld(G4VPhysicalVolume *volume)
Set the geometry world.
bool debugLimits
Property: Flag to debug LimitSets during conversion mechanism.
bool debugElements
Property: Flag to debug elements during conversion mechanism.
virtual void installCommandMessenger() override
Install command control messenger to write GDML file from command prompt.
Geant4GeometryInfo & data() const
Access to the data pointer.
Handle class holding a placed volume (also called physical volume)
virtual ~Geant4DetectorGeometryConstruction()
Default destructor.
bool m_debugMaterials
Property: Flag to debug materials during conversion mechanism.
PlacedVolume placement() const
Access to the physical volume of this detector element.
void printG4(const std::string &prefix, const G4VPhysicalVolume *g4pv) const
Class to create Geant4 detector geometry from TGeo representation in memory.
Geant4GeometryMaps::MaterialMap g4Materials
bool debugSurfaces
Property: Flag to debug surfaces during conversion mechanism.
Solid solid() const
Access to Solid (Shape)
bool isValid() const
Check the validity of the object held by the handle.
bool m_printSensitives
Property: Flag to dump all sensitives after the conversion procedure.
bool debugShapes
Property: Flag to debug shapes during conversion mechanism.
static void increment(T *)
Increment count according to type information.
int printVolumeObj(const char *vol_path, PlacedVolume pv, int flg)
Print geant4 volume.
static Geant4Mapping & instance()
Possibility to define a singleton instance.
int printMaterial(const char *mat_name)
Print geant4 material.
const char * name() const
Access the object name (or "" if not supported by the object)
#define DECLARE_GEANT4ACTION(name)
Plugin defintion to create Geant4Action objects.
void warning(const char *fmt,...) const
Support of warning messages.
G4VPhysicalVolume * world() const
Access to geometry world.
Detector & detectorDescription() const
Access to detector description.
G4VPhysicalVolume * world
Reference to the world after construction.
void info(const char *fmt,...) const
Support of info messages.
bool debugPlacements
Property: Flag to debug placements during conversion mechanism.
Geant4 detector construction context definition.
Geant4Converter & create(DetElement top)
Create geometry conversion.
Handle class describing a detector element.
bool m_debugShapes
Property: Flag to debug shapes during conversion mechanism.
Detector & description
Reference to geometry object.
Handle class holding a placed volume (also called physical volume)
bool m_printPlacements
Property: Flag to dump all placements after the conversion procedure.
bool m_debugReflections
Property: Flag to debug reflections during conversion mechanism.
Geometry mapping from dd4hep to Geant 4.
std::pair< std::string, PlacedVolume > resolve_path(const char *vol_path) const
Geant4GeometryMaps::PlacementMap g4Placements
Definition of the generic callback structure for member functions.
Geant4Action & declareProperty(const std::string &nam, T &val)
Declare property.
static void decrement(T *)
Decrement count according to type information.
bool m_debugPlacements
Property: Flag to debug placements during conversion mechanism.
int m_geoInfoPrintLevel
Property: Printout level of info object.
Geant4VolumeManager volumeManager() const
Access the volume manager.
bool m_debugRegions
Property: Flag to debug regions during conversion mechanism.
Geant4DetectorGeometryConstruction(Geant4Context *ctxt, const std::string &nam)
Initializing constructor for DDG4.
PrintLevel outputLevel() const
Access the output level.
bool debugVolumes
Property: Flag to debug volumes during conversion mechanism.
bool printSensitives
Property: Flag to dump all sensitives after the conversion procedure.
int checkVolume(const char *vol_path)
Check geant4 volume.
bool m_debugSurfaces
Property: Flag to debug regions during conversion mechanism.
int printVolume(const char *vol_path)
Print geant4 volume.
Geant4UIMessenger * m_control
Control directory of this action.
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
Geant4Kernel & kernel() const
Access to the kernel object.
bool m_debugLimits
Property: Flag to debug limit sets during conversion mechanism.
Class describing a box shape.
T * ptr() const
Access to the held object.
void attach(Geant4GeometryInfo *data)
Set a new data block.
virtual void installCommandMessenger()
Install command control messenger if wanted.
void constructGeo(Geant4DetectorConstructionContext *ctxt) override
Geometry construction callback. Called at "Construct()".
Namespace for the AIDA detector description toolkit.
bool debugReflections
Property: Flag to debug reflections during conversion mechanism.
void addCall(const std::string &name, const std::string &description, const Callback &cb, size_t npar=0)
Add a new callback structure.
Volume volume() const
Logical volume of this placement.
The main interface to the dd4hep detector description package.
void always(const char *fmt,...) const
Support of always printed messages.
std::string m_dumpGDML
Property: G4 GDML dump file name (default: empty. If non empty, dump)
int writeGDML(const char *gdml_output)
Write GDML file.
virtual void dump(const std::string &indent, const G4VPhysicalVolume *vol) const
Dump the volume hierarchy as it is known to geant 4.
Geant4GeometryInfo * detach()
Release data and pass over the ownership.
Geant4GeometryInfo * geometry
The cached geometry information.
virtual void enableUI()
Enable and install UI messenger.
G4VPhysicalVolume * world() const
The world placement.
Basic implementation of the Geant4 detector construction action.
bool printPlacements
Property: Flag to dump all placements after the conversion procedure.
bool m_debugVolumes
Property: Flag to debug volumes during conversion mechanism.
Generic context to extend user, run and event information.
int printG4Tree(const char *vol_path)
Print geant4 volume tree.
Geant4Context * context() const
Access the context.
Geant4GeometryMaps::VolumeMap g4Volumes