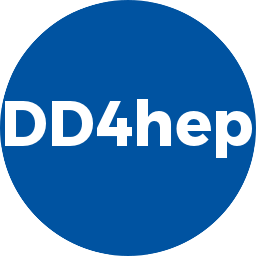 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDG4_FACTORIES_H
14 #define DDG4_FACTORIES_H
31 class G4LogicalVolume;
32 class G4ParticleDefinition;
34 class G4MagIntegratorStepper;
35 class G4EquationOfMotion;
39 class G4VUserPhysicsList;
40 class G4VPrimaryGenerator;
60 class Geant4Converter;
61 class Geant4Sensitive;
62 class Geant4UserPhysics;
63 class Geant4EventReader;
64 class Geant4PhysicsListActionSequence;
100 typedef std::map<std::string,std::string> STRM;
101 typedef G4MagIntegratorStepper Stepper;
125 {
return new P(a0, a1, *a2, *a3); }
129 {
return new P(a0,a1); }
133 {
return new P(a0); }
137 {
return new P(a0); }
141 {
return new P(a0); }
145 {
return (G4VProcess*)
new P(); }
153 {
return P::Definition(); }
161 P::ConstructParticle();
169 dd4hep::printout(dd4hep::INFO,
"PhysicsList",
"+++ Create physics list of type:%s",
170 dd4hep::typeName(
typeid(P)).c_str());
176 {
return new P(a0); }
179 #define __IMPLEMENT_GEANT4SENSDET(name,func) DD4HEP_OPEN_PLUGIN(dd4hep,geant4_sd_##name) { \
180 template <> G4VSensitiveDetector* Geant4SensitiveDetectorFactory< geant4_sd_##name >:: \
181 create(const str_t& n,dd4hep::Detector& l) { return func (n,l); } \
182 DD4HEP_PLUGINSVC_FACTORY(geant4_sd_##name,name,G4VSensitiveDetector*(std::string,dd4hep::Detector*),__LINE__) }
184 #define DECLARE_EXTERNAL_GEANT4SENSITIVEDETECTOR(id,func) __IMPLEMENT_GEANT4SENSDET(id,func)
185 #define DECLARE_GEANT4SENSITIVEDETECTOR(id) __IMPLEMENT_GEANT4SENSDET(id,new id)
186 #define DECLARE_GEANT4SENSITIVEDETECTOR_NS(ns,id) __IMPLEMENT_GEANT4SENSDET(id,new ns::id)
188 #define DECLARE_GEANT4EXTENDEDMATERIAL(name) \
189 DD4HEP_PLUGINSVC_FACTORY(name,name,G4Material*(dd4hep::Detector*,dd4hep::Material,G4Material*),__LINE__)
191 #define DECLARE_GEANT4EXTENDEDMATERIAL_NS(ns,name) using name_space::name; \
192 DD4HEP_PLUGINSVC_FACTORY(name,name,G4Material*(dd4hep::Detector*,dd4hep::Material,G4Material*),__LINE__)
194 #define DECLARE_GEANT4LOGICALVOLUME(name) \
195 DD4HEP_PLUGINSVC_FACTORY(name,name,G4LogicalVolume*(dd4hep::Detector*,dd4hep::Volume,G4VSolid*,G4Material*),__LINE__)
197 #define DECLARE_GEANT4LOGICALVOLUME_NS(ns,name) using name_space::name; \
198 DD4HEP_PLUGINSVC_FACTORY(name,name,G4LogicalVolume*(dd4hep::Detector*,dd4hep::Volume,G4VSolid*,G4Material*),__LINE__)
201 #define DECLARE_GEANT4SENSITIVE_NS(name_space,name) using name_space::name; \
202 DD4HEP_PLUGINSVC_FACTORY(name,name,DS::Geant4Sensitive*(_ns::CT*,std::string,dd4hep::DetElement*,dd4hep::Detector*),__LINE__)
204 #define DECLARE_GEANT4SENSITIVE(name) DECLARE_GEANT4SENSITIVE_NS(dd4hep::sim,name)
207 #define DECLARE_GEANT4ACTION_NS(name_space,name) using name_space::name; \
208 DD4HEP_PLUGINSVC_FACTORY(name,name,dd4hep::sim::Geant4Action*(_ns::CT*,std::string),__LINE__)
209 #define DECLARE_GEANT4ACTION(name) DECLARE_GEANT4ACTION_NS(dd4hep::sim,name)
213 #define DECLARE_GEANT4_STEPPER(name) DD4HEP_PLUGINSVC_FACTORY(G4##name,name,_ns::Stepper*(G4EquationOfMotion*),__LINE__)
214 #define DECLARE_GEANT4_MAGSTEPPER(name) DD4HEP_PLUGINSVC_FACTORY(G4##name,name,_ns::Stepper*(G4Mag_EqRhs*),__LINE__)
215 #define DECLARE_GEANT4_MAGMOTION(name) DD4HEP_PLUGINSVC_FACTORY(G4##name,name,G4Mag_EqRhs*(G4MagneticField*),__LINE__)
217 #define DECLARE_GEANT4_PROCESS(name) DD4HEP_PLUGINSVC_FACTORY(name,name,G4VProcess*(),__LINE__)
219 #define DECLARE_GEANT4_PHYSICS(name) DD4HEP_PLUGINSVC_FACTORY(name,name,G4VPhysicsConstructor*(),__LINE__)
221 #define DECLARE_GEANT4_GENERATOR(name) DD4HEP_PLUGINSVC_FACTORY(name,name,G4VPrimaryGenerator*(),__LINE__)
223 #define DECLARE_GEANT4_PARTICLE(name) DD4HEP_PLUGINSVC_FACTORY(name,name,G4ParticleDefinition*(),__LINE__)
225 #define DECLARE_GEANT4_PARTICLEGROUP(name) DD4HEP_PLUGINSVC_FACTORY(name,name,long(),__LINE__)
227 #define DECLARE_GEANT4_PHYSICS_LIST(name) typedef DS::Geant4UserPhysicsList< name > G4_physics_list_##name; \
229 DD4HEP_PLUGINSVC_FACTORY(G4_physics_list_##name,name,G4VUserPhysicsList*(DS::Geant4PhysicsListActionSequence*,int),__LINE__)
230 #define DECLARE_GEANT4_SETUP(name,func) DD4HEP_OPEN_PLUGIN(dd4hep,xml_g4_setup_##name) { \
232 template <> long Geant4SetupAction< xml_g4_setup_##name >:: \
233 create(dd4hep::Detector& l,const _ns::GH& e, const _ns::STRM& a) {return func(l,e,a);} \
234 DD4HEP_PLUGINSVC_FACTORY(xml_g4_setup_##name,name##_Geant4_action,long(dd4hep::Detector*,const _ns::GH*,const _ns::STRM*),__LINE__) }
237 #define DECLARE_GEANT4_EVENT_READER(name) DD4HEP_PLUGINSVC_FACTORY(name,name,_ns::RDR*(std::string),__LINE__)
240 #define DECLARE_GEANT4_EVENT_READER_NS(name_space,name) typedef name_space::name __##name##__; \
241 DD4HEP_PLUGINSVC_FACTORY(__##name##__,name,_ns::RDR*(std::string),__LINE__)
243 #endif // DDG4_FACTORIES_H
Templated factory method to G4ExtendedMaterial objects.
#define DD4HEP_PLUGIN_FACTORY_ARGS_3(R, A0, A1, A2)
#define DD4HEP_PLUGIN_FACTORY_ARGS_4(R, A0, A1, A2, A3)
static G4Material * create(dd4hep::Detector &description, dd4hep::Material mat, G4Material *base_material)
#define DD4HEP_PLUGIN_FACTORY_ARGS_1(R, A0)
#define DD4HEP_PLUGIN_FACTORY_ARGS_0(R)
Deprecated: Templated factory method to create sensitive detector.
#define DD4HEP_PLUGIN_FACTORY_ARGS_2(R, A0, A1)
Handle class describing a material.
Templated factory method to G4ExtendedMaterial objects.
Handle class describing a detector element.
Handle class holding a placed volume (also called physical volume)
The base class for all dd4hep geometry crawlers.
static G4LogicalVolume * create(dd4hep::Detector &description, dd4hep::Volume vol, G4VSolid *sol, G4Material *mat)
Class of the Geant4 toolkit. See http://www-geant4.kek.jp/Reference.
The implementation of the single Geant4 physics list action sequence.
Factory base class implementing some utilities.
Default base class for all Geant 4 actions and derivates thereof.
Basic geant4 event reader class. This interface/base-class must be implemented by concrete readers.
Class of the Geant4 toolkit. See http://www-geant4.kek.jp/Reference.
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
Templated factory method to invoke setup action.
Namespace for the AIDA detector description toolkit.
The main interface to the dd4hep detector description package.
The base class for Geant4 sensitive detector actions implemented by users.
static G4VSensitiveDetector * create(const str_t &name, dd4hep::Detector &description)
static long create(dd4hep::Detector &description, const dd4hep::detail::GeoHandler &cnv, const std::map< str_t, str_t > &attrs)
Generic context to extend user, run and event information.
Class of the Geant4 toolkit. See http://www-geant4.kek.jp/Reference.