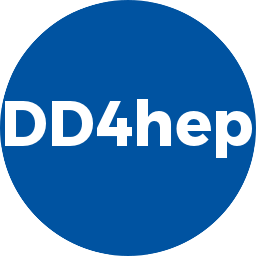 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDG4_GEANT4KERNEL_H
14 #define DDG4_GEANT4KERNEL_H
27 class G4VPhysicalVolume;
33 class Geant4Interrupts;
39 class Geant4Interrupts;
40 class Geant4ActionPhase;
46 virtual const char*
what() const noexcept
override {
return "Reached end of input file"; }
53 virtual const char*
what() const noexcept
override {
return "Event loop STOP signalled. Processing stops"; }
66 typedef std::map<unsigned long, Geant4Kernel*>
Workers;
67 typedef std::map<std::string, Geant4ActionPhase*>
Phases;
234 G4VPhysicalVolume*
world()
const;
236 void setWorld(G4VPhysicalVolume* volume);
258 void setOutputLevel(
const std::string
object, PrintLevel new_level);
289 Geant4Action*
globalAction(
const std::string& action_name,
bool throw_if_not_present =
true);
297 Geant4Action*
globalFilter(
const std::string& filter_name,
bool throw_if_not_present =
true);
300 Geant4ActionPhase*
getPhase(
const std::string& name);
303 virtual Geant4ActionPhase*
addSimplePhase(
const std::string& name,
bool throw_on_exist);
306 virtual Geant4ActionPhase*
addPhase(
const std::string& name,
const std::type_info& arg1,
const std::type_info& arg2,
307 const std::type_info& arg3,
bool throw_on_exist);
310 return addPhase(name,
typeid(A0),
typeid(
void),
typeid(
void), throw_on_exist);
313 template <
typename A0,
typename A1>
315 return addPhase(name,
typeid(A0),
typeid(A1),
typeid(
void), throw_on_exist);
318 template <
typename A0,
typename A1,
typename A2>
320 return addPhase(name,
typeid(A0),
typeid(A1),
typeid(A2), throw_on_exist);
328 virtual bool executePhase(
const std::string& name,
const void** args)
const;
331 virtual void loadGeometry(
const std::string& compact_file);
333 virtual void loadXML(
const char* fname);
381 #endif // DDG4_GEANT4KERNEL_H
std::map< unsigned long, Geant4Kernel * > Workers
Geant4ActionPhase * addPhase(const std::string &name, bool throw_on_exist=true)
Add a new phase to the phase.
UserFramework m_userFramework
Reference to the user framework.
void triggerStop()
Trigger smooth end-of-event-loop with finishing currently processing event.
const std::string & directoryName() const
Access the command directory.
virtual int configure()
Run the simulation: Configure Geant4.
G4UIdirectory * m_control
Top level control directory.
class dd4hep::sim::Geant4Kernel::PhaseSelector phase
std::pair< void *, const std::type_info * > UserFramework
Geant4Kernel * m_kernel
Reference to embedding object.
virtual void loadXML(const char *fname)
Load XML file.
void defineSensitiveDetectorType(const std::string &type, const std::string &factory)
Add new sensitive type to factory list.
void setWorld(G4VPhysicalVolume *volume)
Set the geometry world.
virtual Geant4ActionPhase * addSimplePhase(const std::string &name, bool throw_on_exist)
Add a new phase to the phase.
PrintLevel outputLevel() const
Access the output level.
The property class to assign options to actions.
Interruptsback interface class with argument.
static int configure(Geant4Kernel &kernel)
Configure the application.
int m_outputLevel
Property: Output level.
Embedded helper class to facilitate map access to the phases.
virtual void destroyPhases()
Destroy all phases. To be called only at shutdown.
unsigned long id() const
Access worker identifier.
static int run(Geant4Kernel &kernel)
Run the application and simulate events.
Geant4Kernel & declareProperty(const std::string &nam, T &val)
Declare property.
void exception(const std::string &src, const std::string &msg)
std::string m_dfltSensitiveDetectorType
Property: Name of the default factory to create G4VSensitiveDetector instances.
Workers m_workers
Worker threads.
virtual Geant4ActionPhase * addPhase(const std::string &name, const std::type_info &arg1, const std::type_info &arg2, const std::type_info &arg3, bool throw_on_exist)
Add a new phase to the phase.
Geant4Kernel(Geant4Kernel *m, unsigned long identifier)
Standard constructor for workers.
Geant4Kernel * m_master
Parent reference.
Geant4Kernel & registerGlobalAction(Geant4Action *action)
Register action by name to be retrieved when setting up and connecting action objects.
int m_processEvents
Master property: Flag if event loop is enabled.
Property & property(const std::string &name)
Access single property.
Class, which allows all Geant4Action derivatives to access the DDG4 kernel structures.
virtual bool executePhase(const std::string &name, const void **args) const
Execute phase action if it exists.
Action phase definition. Client callback at various stage of the simulation processing.
Geant4Interrupts * m_interrupts
Interrupt/signal handler: only on master instance.
PrintLevel setOutputLevel(PrintLevel new_level)
Set the global output level of the kernel object; returns previous value.
virtual bool removePhase(const std::string &name)
Remove an existing phase from the phase. If not existing returns false.
bool processEvents() const
Check if event processing should be continued.
PhaseSelector(Geant4Kernel *kernel)
Standard constructor.
void register_initialize(const std::function< void()> &callback)
Register initialize callback. Signature: (function)()
Geant4Context * m_threadContext
Thread context reference.
Detector & detectorDescription() const
Access to detector description.
Geant4ActionPhase * addPhase(const std::string &name, bool throw_on_exist=true)
Add a new phase to the phase.
Geant4Kernel & worker(unsigned long thread_identifier, bool create_if=false)
Access worker instance by its identifier.
ClientOutputLevels m_clientLevels
Property: Client output levels.
Geant4ActionPhase * addPhase(const std::string &name, bool throw_on_exist=true)
Add a new phase to the phase.
Geant4Kernel & registerGlobalFilter(Geant4Action *filter)
Register filter by name to be retrieved when setting up and connecting filter objects.
PrintLevel getOutputLevel(const std::string object) const
Retrieve the global output level of a named object.
void register_terminate(const std::function< void()> &callback)
Register terminate callback. Signature: (function)()
GlobalActions m_globalActions
Globally registered actions.
UserCallbacks m_actionInitialize
Registered action callbacks on initialize.
const std::map< std::string, std::string > & sensitiveDetectorTypes() const
Property access: Names with specialized factories to create G4VSensitiveDetector instances.
Geant4Interrupts & interruptHandler() const
Access interrupt handler. Will be created on the first call.
Geant4ActionPhase & operator[](const std::string &name) const
Phase access to the map.
G4RunManager * m_runManager
Reference to the run manager.
Geant4ActionPhase * getPhase(const std::string &name)
Access phase by name.
std::string m_runManagerType
Property: Name of the G4 run manager factory to be used. Default: Geant4RunManager.
bool hasProperty(const std::string &name) const
Check property for existence.
UserCallbacks m_actionConfigure
Registered action callbacks on configure.
int m_haveScoringMgr
Master property: Instantiate the Geant4 scoring manager object.
const std::string defaultSensitiveDetectorType() const
Property access: Name of the default factory to create G4VSensitiveDetector instances.
G4TrackingManager * trackMgr() const
Access the tracking manager.
std::vector< std::function< void()> > UserCallbacks
G4VPhysicalVolume * world() const
Access to geometry world.
virtual const char * what() const noexcept override
static int terminate(Geant4Kernel &kernel)
Terminate the application.
std::map< std::string, int > ClientOutputLevels
bool registerInterruptHandler(int sig_num)
Install DDG4 default handler for a given signal. If no handler: return false.
static unsigned long int thread_self()
Access thread identifier.
const Phases & phases() const
Access phase phases.
PropertyManager & properties()
Access to the properties of the object.
Phases m_phases
Action phases.
Geant4Action * globalFilter(const std::string &filter_name, bool throw_if_not_present=true)
Retrieve filter from repository.
int m_numThreads
Master property: Number of execution threads in multi threaded mode.
void register_configure(const std::function< void()> &callback)
Register configure callback. Signature: (function)()
static int initialize(Geant4Kernel &kernel)
Initialize the application.
std::string m_uiName
Property: Name of the UI action. Must be member of the global actions.
GlobalActions m_globalFilters
Globally registered filters of sensitive detectors.
void add(const std::string &name, const Property &property)
Add a new property.
G4VPhysicalVolume * m_world
Access to geometry world.
bool isMultiThreaded() const
long m_numEvent
Property: Number of events to be executed in batch mode.
Geant4Action * globalAction(const std::string &action_name, bool throw_if_not_present=true)
Retrieve action from repository.
std::map< std::string, Geant4Action * > GlobalActions
virtual int initialize()
Run the simulation: Initialize Geant4.
UserFramework & userFramework()
Generic framework access.
static Geant4Kernel & instance(Detector &description)
Instance accessor.
virtual Geant4Kernel & createWorker()
Create identified worker instance.
virtual int run()
Run the simulation: Simulate the number of events given by the property "NumEvents".
virtual void loadGeometry(const std::string &compact_file)
Construct detector geometry using description plugin.
unsigned long m_id
Flag: Master instance (id<0) or worker (id >= 0)
Main executor steering the Geant4 execution.
void setUserFramework(T *object)
Set the framework context to the kernel object.
Manager to ease the handling of groups of properties.
std::string m_controlName
Property: Name of the G4UI command tree.
Class, which allows all Geant4Action to be stored.
Helper class to indicate the end of the input file.
Namespace for the AIDA detector description toolkit.
void setTrackMgr(G4TrackingManager *mgr)
Access the tracking manager.
virtual const char * what() const noexcept override
virtual int runEvents(int num_events)
Run the simulation: Simulate the number of events "num_events" and modify the property "NumEvents".
The main interface to the dd4hep detector description package.
Detector * m_detDesc
Detector description object.
std::map< std::string, Geant4ActionPhase * > Phases
PropertyManager m_properties
Property pool.
G4RunManager & runManager()
Access to the Geant4 run manager.
G4TrackingManager * m_trackMgr
Reference to Geant4 track manager.
virtual ~Geant4Kernel()
Default destructor.
Geant4Kernel & master() const
Thread's master context.
PhaseSelector & operator=(const PhaseSelector &c)
Assignment operator.
Helper class to indicate the stop of processing.
UserCallbacks m_actionTerminate
Registered action callbacks on terminate.
std::map< std::string, std::string > m_sensitiveDetectorTypes
Property: Names with specialized factories to create G4VSensitiveDetector instances.
void applyInterruptHandlers()
(Re-)apply registered interrupt handlers to override potentially later registrations by other librari...
void printProperties() const
Print the property values.
int numWorkers() const
Access number of workers.
Generic context to extend user, run and event information.
virtual int terminate() override
Run the simulation: Terminate Geant4.