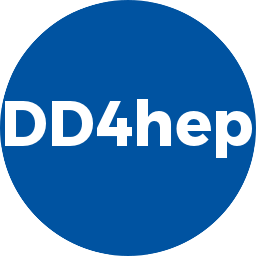 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DDG4_GEANT4GEOMETRYINFO_H
14 #define DDG4_GEANT4GEOMETRYINFO_H
41 class G4LogicalVolume;
44 class G4VisAttributes;
45 class G4VPhysicalVolume;
46 class G4OpticalSurface;
47 class G4LogicalSkinSurface;
48 class G4LogicalBorderSurface;
51 class G4PhysicsOrderedFreeVector;
61 class Geant4AssemblyVolume;
69 namespace Geant4GeometryMaps {
71 typedef std::map<const TGeoIsotope*, G4Isotope*>
IsotopeMap;
74 typedef std::map<Volume, G4LogicalVolume*>
VolumeMap;
75 typedef std::map<PlacedVolume, Geant4AssemblyVolume*>
AssemblyMap;
78 typedef std::pair<PlacedVolumeChain,const G4VPhysicalVolume*>
ImprintEntry;
81 typedef std::map<const TGeoShape*, G4VSolid*>
SolidMap;
132 std::map<VisAttr, G4VisAttributes*>
g4Vis;
135 std::map<SensitiveDetector,std::set<const TGeoVolume*> >
sensitives;
136 std::map<Region, std::set<const TGeoVolume*> >
regions;
137 std::map<LimitSet, std::set<const TGeoVolume*> >
limits;
157 G4VPhysicalVolume*
world()
const;
159 void setWorld(
const TGeoNode* node);
163 #endif // DDG4_GEANT4GEOMETRYINFO_H
~PropertyVector()=default
std::map< SensitiveDetector, std::set< const TGeoVolume * > > sensitives
std::map< OpticalSurface, G4OpticalSurface * > g4OpticalSurfaces
Geant4GeometryMaps::AssemblyMap g4AssemblyVolumes
std::pair< PlacedVolumeChain, const G4VPhysicalVolume * > ImprintEntry
std::map< Region, G4Region * > g4Regions
std::map< PlacedVolume, Geant4AssemblyVolume * > AssemblyMap
static std::string placementPath(const Geant4TouchableHandler::Geant4PlacementPath &path, bool reverse=true)
Assemble Geant4 volume path.
Data container to store information obtained during the geometry scan.
struct dd4hep::sim::Geant4GeometryInfo::PlacementFlags::_flags flags
Geant4GeometryMaps::MaterialMap g4Materials
std::vector< const G4VPhysicalVolume * > Geant4PlacementPath
std::map< VisAttr, G4VisAttributes * > g4Vis
std::map< const TGeoIsotope *, G4Isotope * > IsotopeMap
std::vector< double > bins
std::map< uint64_t, Placement > g4Paths
Geant4GeometryInfo()
Default constructor.
void setWorld(const TGeoNode *node)
Set the world volume.
Class of the Geant4 toolkit. See http://www-geant4.kek.jp/Reference.
virtual ~Geant4GeometryInfo()
Default destructor.
Geant4GeometryMaps::G4PlacementMap g4Parameterised
std::map< LimitSet, std::set< const TGeoVolume * > > limits
Geometry mapping from dd4hep to Geant 4.
std::vector< double > values
Class of the Geant4 toolkit. See http://www-geant4.kek.jp/Reference.
Geant4GeometryMaps::PlacementMap g4Placements
Class of the ROOT toolkit. See http://root.cern.ch/root/htmldoc/ClassIndex.html.
Geant4GeometryMaps::IsotopeMap g4Isotopes
std::map< BorderSurface, G4LogicalBorderSurface * > g4BorderSurfaces
Geant4GeometryMaps::VolumeImprintMap g4VolumeImprints
std::map< const G4VPhysicalVolume *, PlacedVolume > G4PlacementMap
G4VPhysicalVolume * m_world
Geant4GeometryMaps::G4PlacementMap g4Replicated
std::map< Material, G4Material * > MaterialMap
std::map< Atom, G4Element * > ElementMap
std::map< LimitSet, G4UserLimits * > g4Limits
Geant4GeometryMaps::SolidMap g4Solids
Class of the Geant4 toolkit. See http://www-geant4.kek.jp/Reference.
dd4hep::DDSegmentation::VolumeID VolumeID
static std::string placementPath(const Geant4PlacementPath &path, bool reverse=true)
Assemble Geant4 volume path.
std::vector< ImprintEntry > Imprints
Concreate class holding the relation information between geant4 objects and dd4hep objects.
std::map< Volume, Imprints > VolumeImprintMap
Namespace for the AIDA detector description toolkit.
std::map< Region, std::set< const TGeoVolume * > > regions
Geant4GeometryMaps::ElementMap g4Elements
G4VPhysicalVolume * world() const
The world placement.
std::map< SkinSurface, G4LogicalSkinSurface * > g4SkinSurfaces
std::vector< const TGeoNode * > PlacedVolumeChain
std::map< Volume, G4LogicalVolume * > VolumeMap
std::map< const TGeoShape *, G4VSolid * > SolidMap
std::map< PropertyTable, PropertyVector * > g4OpticalProperties
Geant4GeometryMaps::VolumeMap g4Volumes
std::map< PlacedVolume, G4VPhysicalVolume * > PlacementMap