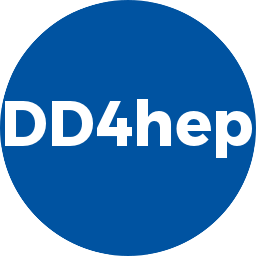 |
DD4hep
1.32.1
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
14 #ifndef DDG4_GEANT4DETECTORCONSTRUCTION_H
15 #define DDG4_GEANT4DETECTORCONSTRUCTION_H
24 class G4VPhysicalVolume;
25 class G4LogicalVolume;
43 class Geant4GeometryInfo;
44 class Geant4AssemblyVolume;
45 class Geant4DetectorConstruction;
46 class Geant4DetectorConstructionContext;
47 class Geant4DetectorConstructionSequence;
69 G4VPhysicalVolume*
world {
nullptr };
116 const std::string&
name);
158 #if !defined(__CINT__)
162 const std::map<Material, G4Material*>&
materials()
const;
164 const std::map<Atom, G4Element*>&
elements()
const;
166 const std::map<const TGeoShape*, G4VSolid*>&
shapes()
const;
168 const std::map<Volume, G4LogicalVolume*>&
volumes()
const;
170 const std::map<PlacedVolume, G4VPhysicalVolume*>&
placements()
const;
172 const std::map<PlacedVolume, Geant4AssemblyVolume*>&
assemblies()
const;
175 const std::map<LimitSet, G4UserLimits*>&
limits()
const;
177 const std::map<Region, G4Region*>&
regions()
const;
186 #endif // DDG4_GEANT4DETECTORCONSTRUCTION_H
Geant4DetectorConstructionContext(Detector &l, G4VUserDetectorConstruction *d)
Initializing Constructor.
virtual void constructField(Geant4DetectorConstructionContext *ctxt)
Electromagnetic field construction callback. Called at "ConstructSDandField()".
virtual void constructSensitives(Geant4DetectorConstructionContext *ctxt)
Sensitive detector construction callback. Called at "ConstructSDandField()".
virtual G4VSensitiveDetector * createSensitiveDetector(const std::string &type, const std::string &name)
Create Geant4 sensitive detector object using the factory mechanism.
virtual ~Geant4DetectorConstructionSequence()
Default destructor.
const std::map< const TGeoShape *, G4VSolid * > & shapes() const
Access to the converted shapes.
virtual void updateContext(Geant4Context *ctxt) override
Set or update client context.
Geant4DetectorConstruction(Geant4Context *context, const std::string &nam)
Standard Constructor.
Class of the Geant4 toolkit. See http://www-geant4.kek.jp/Reference.
~Geant4DetectorConstructionContext()
Default destructor.
G4VPhysicalVolume * world
Reference to the world after construction.
Geant4 detector construction context definition.
Detector & description
Reference to geometry object.
virtual void constructGeo(Geant4DetectorConstructionContext *ctxt)
Geometry construction callback. Called at "Construct()".
Class of the Geant4 toolkit. See http://www-geant4.kek.jp/Reference.
const std::map< Atom, G4Element * > & elements() const
Access to the converted elements.
void adopt(Geant4DetectorConstruction *action)
Add an actor responding to all callbacks. Sequence takes ownership.
virtual void constructSensitives(Geant4DetectorConstructionContext *ctxt)
Sensitive detector construction callback. Called at "ConstructSDandField()".
const std::map< LimitSet, G4UserLimits * > & limits() const
Access to the converted limit sets.
Default base class for all Geant 4 actions and derivates thereof.
const std::string & name() const
Access name of the action.
Concrete basic implementation of the Geant4 detector construction sequencer.
Actors< Geant4DetectorConstruction > m_actors
The list of action objects to be called.
G4VUserDetectorConstruction * detector
G4 User detector initializer.
virtual ~Geant4DetectorConstruction()
Default destructor.
Geant4DetectorConstruction * get(const std::string &nam) const
Access an actor by name.
Concreate class holding the relation information between geant4 objects and dd4hep objects.
virtual void constructGeo(Geant4DetectorConstructionContext *ctxt)
Geometry construction callback. Called at "Construct()".
void setSensitiveDetector(G4LogicalVolume *vol, G4VSensitiveDetector *sd)
Helper: Assign sensitive detector to logical volume.
Namespace for the AIDA detector description toolkit.
const std::map< Material, G4Material * > & materials() const
Access to the converted materials.
virtual void constructField(Geant4DetectorConstructionContext *ctxt)
Electromagnetic field construction callback. Called at "ConstructSDandField()".
Class of the Geant4 toolkit. See http://www-geant4.kek.jp/Reference.
const std::map< Volume, G4LogicalVolume * > & volumes() const
Access to the converted volumes.
The main interface to the dd4hep detector description package.
Actor class to manipulate action groups.
const std::map< PlacedVolume, G4VPhysicalVolume * > & placements() const
Access to the converted placements.
Geant4GeometryInfo * geometry
The cached geometry information.
Basic implementation of the Geant4 detector construction action.
Geant4DetectorConstructionSequence(Geant4Context *context, const std::string &nam)
Standard Constructor.
const std::map< Region, G4Region * > & regions() const
Access to the converted regions.
Generic context to extend user, run and event information.
Geant4Context * context() const
Access the context.
const std::map< PlacedVolume, Geant4AssemblyVolume * > & assemblies() const
Access to the converted assemblys.