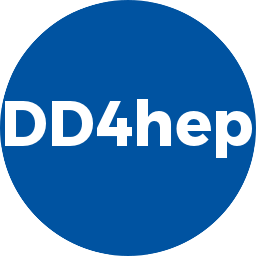 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
51 : m_kernel(kernel_pointer), m_run(0), m_event(0) {
74 invalidHandleError<Geant4Run>();
86 invalidHandleError<Geant4Event>();
102 std::string err = dd4hep::format(
"Geant4Kernel",
"createTrajectory: Purely virtual method. requires overloading!");
103 dd4hep::printout(dd4hep::FATAL,
"Geant4Kernel",
"createTrajectory: Purely virtual method. requires overloading!");
104 throw std::runtime_error(err);
Geant4RunActionSequence & runAction() const
Access to the main run action sequence from the kernel object.
void setEvent(Geant4Event *new_event)
Set the geant4 event reference.
User run context for DDG4.
Detector & detectorDescription() const
Access to detector description.
Concrete implementation of the Geant4 stacking action sequence.
Implementation of an object supporting arbitrary user extensions.
Geant4RunActionSequence * runAction(bool create)
Access run action sequence.
Geant4Kernel * m_kernel
Reference to the kernel object.
Geant4Run & run() const
Access the geant4 run – valid only between BeginRun() and EndRun()!
void setRun(Geant4Run *new_run)
Set the geant4 run reference.
Geant4StackingActionSequence * stackingAction(bool create)
Access stacking action sequence.
Class, which allows all Geant4Action derivatives to access the DDG4 kernel structures.
Geant4TrackingActionSequence * trackingAction(bool create)
Access tracking action sequence.
Geant4Run * m_run
Transient context variable - depending on the thread context: run reference.
static void increment(T *)
Increment count according to type information.
Geant4Event & event() const
Access the geant4 event – valid only between BeginEvent() and EndEvent()!
G4VPhysicalVolume * world() const
Access to geometry world.
Geant4SensDetSequences & sensitiveActions() const
Access to the sensitive detector sequences from the kernel object.
Geant4Context(Geant4Kernel *kernel)
Default constructor.
Concrete implementation of the Geant4 tracking action sequence.
Detector & detectorDescription() const
Access to detector description.
Concrete implementation of the Geant4 generator action sequence.
virtual ~Geant4Event()
Default destructor.
Geant4EventActionSequence * eventAction(bool create)
Access run action sequence.
static void decrement(T *)
Decrement count according to type information.
Concrete basic implementation of the Geant4 run action sequencer.
G4TrackingManager * trackMgr() const
Access the tracking manager.
Geant4SensDetSequences: class to access groups of sensitive actions.
G4VPhysicalVolume * world() const
Access to geometry world.
Geant4Event * m_event
Transient context variable - depending on the thread context: event reference.
Mini interface to THE random generator of the application.
Geant4Run(const G4Run *run)
Intializing constructor.
Geant4Event(const G4Event *run, Geant4Random *rndm)
Intializing constructor.
virtual ~Geant4Run()
Default destructor.
virtual G4VTrajectory * createTrajectory(const G4Track *track) const
Create a user trajectory.
UserFramework & userFramework()
Generic framework access.
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
UserFramework & userFramework() const
Generic framework access.
Geant4SteppingActionSequence * steppingAction(bool create)
Access stepping action sequence.
Geant4StackingActionSequence & stackingAction() const
Access to the main stacking action sequence from the kernel object.
virtual ~Geant4Context()
Default destructor.
The main interface to the dd4hep detector description package.
G4TrackingManager * trackMgr() const
Access the tracking manager.
Concrete implementation of the Geant4 event action sequence.
Geant4SteppingActionSequence & steppingAction() const
Access to the main stepping action sequence from the kernel object.
Geant4SensDetSequences & sensitiveActions() const
Access to the sensitive detector sequences from the actioncontainer object.
Geant4GeneratorActionSequence & generatorAction() const
Access to the main generator action sequence from the kernel object.
Geant4TrackingActionSequence & trackingAction() const
Access to the main tracking action sequence from the kernel object.
Concrete implementation of the Geant4 stepping action sequence.
User event context for DDG4.
Geant4EventActionSequence & eventAction() const
Access to the main event action sequence from the kernel object.
Geant4GeneratorActionSequence * generatorAction(bool create)
Access generator action sequence.
std::pair< void *, const std::type_info * > UserFramework