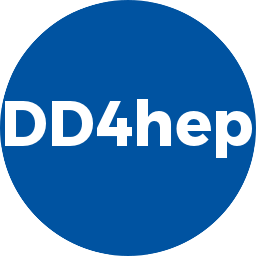 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
14 #ifndef DDG4_GEANT4CONTEXT_H
15 #define DDG4_GEANT4CONTEXT_H
26 class G4VPhysicalVolume;
27 class G4TrackingManager;
44 class Geant4RunActionSequence;
45 class Geant4EventActionSequence;
46 class Geant4SteppingActionSequence;
47 class Geant4TrackingActionSequence;
48 class Geant4StackingActionSequence;
49 class Geant4GeneratorActionSequence;
50 class Geant4SensDetSequences;
80 operator const G4Run&()
const {
return *
m_run; }
88 template <
typename T> T*
addExtension(T* ptr,
bool take_ownership=
true) {
95 template <
typename T> T*
extension(
bool alert=
true) {
133 operator const G4Event&()
const {
return *
m_event; }
144 template <
typename T> T*
addExtension(T* ptr,
bool take_ownership=
true) {
235 G4VPhysicalVolume*
world()
const;
244 G4TrackingManager*
trackMgr()
const;
266 #endif // DDG4_GEANT4CONTEXT_H
Geant4RunActionSequence & runAction() const
Access to the main run action sequence from the kernel object.
Geant4Random * m_random
Reference to the main random number generator.
Definition of the extension entry interface class.
void setEvent(Geant4Event *new_event)
Set the geant4 event reference.
User run context for DDG4.
Detector & detectorDescription() const
Access to detector description.
Concrete implementation of the Geant4 stacking action sequence.
Implementation of an object supporting arbitrary user extensions.
T * addExtension(T *ptr, bool take_ownership=true)
Add user extension object. Ownership is transferred!
Geant4Kernel * m_kernel
Reference to the kernel object.
Geant4Run & run() const
Access the geant4 run – valid only between BeginRun() and EndRun()!
void setRun(Geant4Run *new_run)
Set the geant4 run reference.
T * addExtension(T *ptr, bool take_ownership=true)
Add user extension object. Ownership is transferred and object deleted at the end of the event.
const G4Run & run() const
Access the G4Event directly: Explicit G4Run accessor.
Class, which allows all Geant4Action derivatives to access the DDG4 kernel structures.
Geant4Run * m_run
Transient context variable - depending on the thread context: run reference.
Geant4Event & event() const
Access the geant4 event – valid only between BeginEvent() and EndEvent()!
Implementation class for the object extension mechanism.
Geant4Random & random() const
Access the random number generator.
G4VPhysicalVolume * world() const
Access to geometry world.
Geant4SensDetSequences & sensitiveActions() const
Access to the sensitive detector sequences from the kernel object.
Geant4Context(Geant4Kernel *kernel)
Default constructor.
Concrete implementation of the Geant4 tracking action sequence.
const G4Event * m_event
Reference to the original Geant4 event object.
T * extension(bool alert=true)
Access to type safe extension object. Exception is thrown if the object is invalid.
void * addExtension(unsigned long long int k, ExtensionEntry *e)
Add an extension object to the detector element.
Concrete implementation of the Geant4 generator action sequence.
virtual ~Geant4Event()
Default destructor.
Implementation class for the object extension mechanism.
Concrete basic implementation of the Geant4 run action sequencer.
Geant4SensDetSequences: class to access groups of sensitive actions.
Geant4Event * m_event
Transient context variable - depending on the thread context: event reference.
Mini interface to THE random generator of the application.
Geant4Run(const G4Run *run)
Intializing constructor.
Geant4Event(const G4Event *run, Geant4Random *rndm)
Intializing constructor.
T * extension(bool alert=true)
Access to type safe extension object. Exception is thrown if the object is invalid.
const G4Run * m_run
Reference to the original Geant4 run object.
virtual ~Geant4Run()
Default destructor.
virtual G4VTrajectory * createTrajectory(const G4Track *track) const
Create a user trajectory.
void * addExtension(unsigned long long int k, ExtensionEntry *e)
Add an extension object to the detector element.
Geant4Run * runPtr() const
Access the geant4 run by ptr. Must be checked by clients!
Geant4Kernel & kernel() const
Access to the kernel object.
UserFramework & userFramework() const
Generic framework access.
Geant4StackingActionSequence & stackingAction() const
Access to the main stacking action sequence from the kernel object.
Namespace for the AIDA detector description toolkit.
const G4Event & event() const
Access the G4Event directly: Explicit G4Event accessor.
virtual ~Geant4Context()
Default destructor.
Geant4Event * eventPtr() const
Access the geant4 event by ptr. Must be checked by clients!
The main interface to the dd4hep detector description package.
G4TrackingManager * trackMgr() const
Access the tracking manager.
Concrete implementation of the Geant4 event action sequence.
Geant4SteppingActionSequence & steppingAction() const
Access to the main stepping action sequence from the kernel object.
Geant4GeneratorActionSequence & generatorAction() const
Access to the main generator action sequence from the kernel object.
Geant4TrackingActionSequence & trackingAction() const
Access to the main tracking action sequence from the kernel object.
T & framework() const
Access to the user framework. Specialized function to be implemented by the client.
Concrete implementation of the Geant4 stepping action sequence.
User event context for DDG4.
void * addExtension(unsigned long long int key, ExtensionEntry *entry)
Add an extension object to the detector element.
Geant4EventActionSequence & eventAction() const
Access to the main event action sequence from the kernel object.
Generic context to extend user, run and event information.
void * extension(unsigned long long int key, bool alert) const
Access an existing extension object from the detector element.
std::pair< void *, const std::type_info * > UserFramework