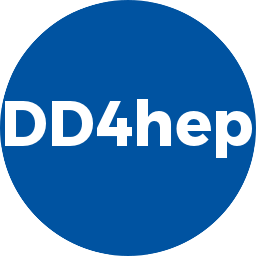 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
13 #ifndef DD4HEP_CALLBACK_H
14 #define DD4HEP_CALLBACK_H
40 typedef unsigned long (*
func_t)(
void* obj,
const void* fun,
const void* args[]);
72 operator bool()
const {
76 unsigned long execute(
const void* user_param[])
const {
80 template <
typename T>
static T*
cast(
void* p) {
84 template <
typename T>
static const T*
c_cast(
const void* p) {
118 template <
typename T>
const Callback&
_make(
ulong (*fptr)(
void* o,
const void* f,
const void* u[]), T pmf) {
120 typename Wrapper<T>::Functor f(pmf);
126 template <
typename R,
typename T>
const Callback&
make(R (T::*pmf)()) {
127 typedef R (T::*pfunc_t)();
128 struct _Wrapper :
public Wrapper<pfunc_t> {
129 static ulong call(
void* o,
const void* f,
const void*[]) {
133 return _make(_Wrapper::call, pmf);
136 template <
typename R,
typename T>
const Callback&
make(R (T::*pmf)()
const) {
137 typedef R (T::*pfunc_t)()
const;
138 struct _Wrapper :
public Wrapper<pfunc_t> {
139 static ulong call(
void* o,
const void* f,
const void*[]) {
143 return _make(_Wrapper::call, pmf);
147 typedef void (T::*pfunc_t)()
const;
148 struct _Wrapper :
public Wrapper<pfunc_t> {
149 static ulong call(
void* o,
const void* f,
const void*[]) {
154 return _make(_Wrapper::call, pmf);
158 typedef void (T::*pfunc_t)()
const;
159 struct _Wrapper :
public Wrapper<pfunc_t> {
160 static ulong call(
void* o,
const void* f,
const void*[]) {
165 return _make(_Wrapper::call, pmf);
169 template <
typename R,
typename T,
typename A>
const Callback&
make(R (T::*pmf)(A)) {
171 typedef R (T::*pfunc_t)(A);
172 struct _Wrapper :
public Wrapper<pfunc_t> {
173 static ulong call(
void* o,
const void* f,
const void* u[]) {
174 return (
ulong) (cast<T>(o)->*(
typename Wrapper<pfunc_t>::Functor(f).pmf))((A) u[0]);
177 return _make(_Wrapper::call, pmf);
180 template <
typename R,
typename T,
typename A>
const Callback&
make(R (T::*pmf)(A)
const) {
181 typedef R (T::*pfunc_t)(A)
const;
182 struct _Wrapper :
public Wrapper<pfunc_t> {
183 static ulong call(
void* o,
const void* f,
const void* u[]) {
187 return _make(_Wrapper::call, pmf);
190 template <
typename T,
typename A>
const Callback&
make(
void (T::*pmf)(A)) {
191 typedef void (T::*pfunc_t)(
const A);
192 struct _Wrapper :
public Wrapper<pfunc_t> {
193 static ulong call(
void* o,
const void* f,
const void* u[]) {
198 return _make(_Wrapper::call, pmf);
201 template <
typename T,
typename A>
const Callback&
make(
void (T::*pmf)(A)
const) {
202 typedef void (T::*pfunc_t)(
const A)
const;
203 struct _Wrapper :
public Wrapper<pfunc_t> {
204 static ulong call(
void* o,
const void* f,
const void* u[]) {
209 return _make(_Wrapper::call, pmf);
214 template <
typename R,
typename T,
typename A0,
typename A1>
const Callback&
make(R (T::*pmf)(A0, A1)) {
216 typedef R (T::*pfunc_t)(A0, A1);
217 typedef Wrapper<pfunc_t> _W;
218 struct _Wrapper :
public _W {
219 static ulong call(
void* o,
const void* f,
const void* u[]) {
220 return (
ulong) (cast<T>(o)->*(
typename _W::Functor(f).pmf))((A0) u[0], (A1) u[1]);
223 return _make(_Wrapper::call, pmf);
226 template <
typename R,
typename T,
typename A0,
typename A1>
const Callback&
make(R (T::*pmf)(A0, A1)
const) {
227 typedef R (T::*pfunc_t)(A0, A1);
229 struct _Wrapper :
public _W {
230 static ulong call(
void* o,
const void* f,
const void* u[]) {
231 return (
ulong) (cast<T>(o)->*(
typename _W::Functor(f).pmf))((A0) u[0], (A1) u[1]);
234 return _make(_Wrapper::call, pmf);
237 template <
typename T,
typename A0,
typename A1>
const Callback&
make(
void (T::*pmf)(A0, A1)) {
238 typedef void (T::*pfunc_t)(A0, A1);
240 struct _Wrapper :
public _W {
241 static ulong call(
void* o,
const void* f,
const void* u[]) {
242 (cast<T>(o)->*(
typename _W::Functor(f).pmf))((A0) u[0], (A1) u[1]);
246 return _make(_Wrapper::call, pmf);
249 template <
typename T,
typename A0,
typename A1>
const Callback&
make(
void (T::*pmf)(A0, A1)
const) {
250 typedef void (T::*pfunc_t)(A0, A1);
252 struct _Wrapper :
public _W {
253 static ulong call(
void* o,
const void* f,
const void* u[]) {
254 (cast<T>(o)->*(
typename _W::Functor(f).pmf))((A0) u[0], (A1) u[1]);
258 return _make(_Wrapper::call, pmf);
263 template <
typename R,
typename T,
typename A0,
typename A1,
typename A2>
const Callback&
make(R (T::*pmf)(A0, A1, A2)) {
265 typedef R (T::*pfunc_t)(A0, A1, A2);
266 typedef Wrapper<pfunc_t> _W;
267 struct _Wrapper :
public _W {
268 static ulong call(
void* o,
const void* f,
const void* u[]) {
269 return (
ulong) (cast<T>(o)->*(
typename _W::Functor(f).pmf))((A0) u[0], (A1) u[1], (A2) u[2]);
272 return _make(_Wrapper::call, pmf);
275 template <
typename R,
typename T,
typename A0,
typename A1,
typename A2>
const Callback&
make(R (T::*pmf)(A0, A1, A2)
const) {
276 typedef R (T::*pfunc_t)(A0, A1, A2);
278 struct _Wrapper :
public _W {
279 static ulong call(
void* o,
const void* f,
const void* u[]) {
280 return (
ulong) (cast<T>(o)->*(
typename _W::Functor(f).pmf))((A0) u[0], (A1) u[1], (A2) u[2]);
283 return _make(_Wrapper::call, pmf);
286 template <
typename T,
typename A0,
typename A1,
typename A2>
const Callback&
make(
void (T::*pmf)(A0, A1, A2)) {
287 typedef void (T::*pfunc_t)(A0, A1, A2);
289 struct _Wrapper :
public _W {
290 static ulong call(
void* o,
const void* f,
const void* u[]) {
291 (cast<T>(o)->*(
typename _W::Functor(f).pmf))((A0) u[0], (A1) u[1], (A2) u[2]);
295 return _make(_Wrapper::call, pmf);
298 template <
typename T,
typename A0,
typename A1,
typename A2>
const Callback&
make(
void (T::*pmf)(A0, A1, A2)
const) {
299 typedef void (T::*pfunc_t)(A0, A1, A2);
301 struct _Wrapper :
public _W {
302 static ulong call(
void* o,
const void* f,
const void* u[]) {
303 (cast<T>(o)->*(
typename _W::Functor(f).pmf))((A0) u[0], (A1) u[1], (A2) u[2]);
307 return _make(_Wrapper::call, pmf);
314 template <
typename P,
typename R,
typename T>
static T*
dyn_cast(P* p, R (T::*)()) {
315 return dynamic_cast<T*
>(p);
317 template <
typename P,
typename R,
typename T>
static const T*
dyn_cast(
const P* p, R (T::*)()
const) {
318 return dynamic_cast<const T*
>(p);
321 template <
typename P,
typename R,
typename T,
typename A>
static T*
dyn_cast(P* p, R (T::*)(A)) {
322 return dynamic_cast<T*
>(p);
324 template <
typename P,
typename R,
typename T,
typename A>
static const T*
dyn_cast(
const P* p, R (T::*)(A)
const) {
325 return dynamic_cast<const T*
>(p);
380 template <
typename A0>
void operator()(A0 a0)
const;
382 template <
typename A0,
typename A1>
void operator()(A0 a0, A1 a1)
const;
384 template <
typename A0,
typename A1,
typename A2>
void operator()(A0 a0, A1 a1, A2 a2)
const;
386 static void checkTypes(
const std::type_info& typ1,
const std::type_info& typ2,
void* test);
389 template <
typename TYPE,
typename R,
typename OBJECT>
392 checkTypes(
typeid(TYPE),
typeid(OBJECT),
dynamic_cast<OBJECT*
>(pointer));
396 template <
typename TYPE,
typename R,
typename OBJECT>
398 checkTypes(
typeid(TYPE),
typeid(OBJECT),
dynamic_cast<OBJECT*
>(pointer));
402 template <
typename TYPE,
typename OBJECT>
404 checkTypes(
typeid(TYPE),
typeid(OBJECT),
dynamic_cast<OBJECT*
>(pointer));
408 template <
typename TYPE,
typename OBJECT>
410 checkTypes(
typeid(TYPE),
typeid(OBJECT),
dynamic_cast<OBJECT*
>(pointer));
415 template <
typename TYPE,
typename R,
typename OBJECT,
typename A>
418 checkTypes(
typeid(TYPE),
typeid(OBJECT),
dynamic_cast<OBJECT*
>(pointer));
422 template <
typename TYPE,
typename OBJECT,
typename A>
424 checkTypes(
typeid(TYPE),
typeid(OBJECT),
dynamic_cast<OBJECT*
>(pointer));
428 template <
typename TYPE,
typename R,
typename OBJECT,
typename A>
430 checkTypes(
typeid(TYPE),
typeid(OBJECT),
dynamic_cast<OBJECT*
>(pointer));
434 template <
typename TYPE,
typename OBJECT,
typename A>
436 checkTypes(
typeid(TYPE),
typeid(OBJECT),
dynamic_cast<OBJECT*
>(pointer));
442 template <
typename TYPE,
typename R,
typename OBJECT,
typename A1,
typename A2>
445 checkTypes(
typeid(TYPE),
typeid(OBJECT),
dynamic_cast<OBJECT*
>(pointer));
449 template <
typename TYPE,
typename R,
typename OBJECT,
typename A1,
typename A2>
451 checkTypes(
typeid(TYPE),
typeid(OBJECT),
dynamic_cast<OBJECT*
>(pointer));
455 template <
typename TYPE,
typename OBJECT,
typename A1,
typename A2>
457 checkTypes(
typeid(TYPE),
typeid(OBJECT),
dynamic_cast<OBJECT*
>(pointer));
461 template <
typename TYPE,
typename OBJECT,
typename A1,
typename A2>
463 checkTypes(
typeid(TYPE),
typeid(OBJECT),
dynamic_cast<OBJECT*
>(pointer));
471 const void* args[1] = { 0 };
477 template <
typename A0>
inline
480 const void* args[1] = { a0 };
486 template <
typename A0,
typename A1>
inline
489 const void* args[2] = { a0, a1 };
495 template <
typename A0,
typename A1,
typename A2>
inline
498 const void* args[3] = { a0, a1, a2 };
505 #endif // DD4HEP_CALLBACK_H
void add(TYPE *pointer, void(OBJECT::*pmf)(A) const, Location where=CallbackSequence::END)
Add a new callback to a const void member function and 1 argument.
void add(TYPE *pointer, void(OBJECT::*pmf)(), Location where=CallbackSequence::END)
Add a new callback to a void member function with no arguments.
static const T * dyn_cast(const P *p, R(T::*)() const)
Structure definition to store callback related data.
Callback(const void *p)
Constructor with object initialization.
void add(TYPE *pointer, void(OBJECT::*pmf)(A), Location where=CallbackSequence::END)
Add a new callback to a void member function and 1 argument.
const Callback & _make(ulong(*fptr)(void *o, const void *f, const void *u[]), T pmf)
Callback setup function for Callbacks with member functions taking no arguments.
CallbackSequence & operator=(const CallbackSequence &c)
Assignment operator.
static mfunc_t pmf(pmf_t f)
const Callback & make(void(T::*pmf)(A0, A1))
Callback setup function for Callbacks with const void member functions taking 2 arguments.
static void checkTypes(const std::type_info &typ1, const std::type_info &typ2, void *test)
Check the compatibility of two typed objects. The test is the result of a dynamic_cast.
void add(const Callback &cb, Location where)
Generically Add a new callback to the sequence depending on the location arguments.
static T * dyn_cast(P *p, R(T::*)(A))
CallbackSequence(const CallbackSequence &c)
Copy constructor.
void add(TYPE *pointer, R(OBJECT::*pmf)() const, Location where=CallbackSequence::END)
Add a new callback to a const member function with explicit return type and no arguments.
void add(TYPE *pointer, void(OBJECT::*pmf)() const, Location where=CallbackSequence::END)
Add a new callback to a const void member function and no arguments.
const Callback & make(R(T::*pmf)(A0, A1, A2) const)
Callback setup function for Callbacks with const member functions with explicit return type taking 3 ...
void add(TYPE *pointer, R(OBJECT::*pmf)(A1, A2), Location where=CallbackSequence::END)
Add a new callback to a member function with explicit return type and 2 arguments.
const Callback & make(R(T::*pmf)())
Callback setup function for Callbacks with member functions with explicit return type taking no argum...
void add(TYPE *pointer, R(OBJECT::*pmf)(), Location where=CallbackSequence::END)
Add a new callback to a member function with explicit return type and no arguments.
Definition of an actor on sequences of callbacks.
CallbackSequence()
Default constructor.
static Callback make(void *p, T pmf)
Callback(void *p)
Constructor with object initialization.
void add(TYPE *pointer, void(OBJECT::*pmf)(A1, A2) const, Location where=CallbackSequence::END)
Add a new callback to a const void member function with 2 arguments.
Definition of the generic callback structure for member functions.
const Callback & make(void(T::*pmf)(A0, A1, A2))
Callback setup function for Callbacks with const void member functions taking 3 arguments.
void add(TYPE *pointer, R(OBJECT::*pmf)(A), Location where=CallbackSequence::END)
Add a new callback to a member function with explicit return type and 1 argument.
static T * dyn_cast(P *p, R(T::*)())
void add(const Callback &cb)
Generically Add a new callback to the sequence depending on the location arguments.
void operator()() const
Execution overload for callbacks with no arguments.
const Callback & make(void(T::*pmf)(A0, A1) const)
Callback setup function for Callbacks with const void member functions taking 2 arguments.
void clear()
Clear the sequence and remove all callbacks.
void add(TYPE *pointer, R(OBJECT::*pmf)(A) const, Location where=CallbackSequence::END)
Add a new callback to a const member function with explicit return type and 1 argument.
static const T * dyn_cast(const P *p, R(T::*)(A) const)
Callback(void *p, void *mf, func_t c)
Initializing constructor.
const Callback & make(void(T::*pmf)(A0, A1, A2) const)
Callback setup function for Callbacks with const void member functions taking 3 arguments.
Wrapper around a C++ member function pointer.
const Callback & make(void(T::*pmf)())
Callback setup function for Callbacks with void member functions taking no arguments.
const Callback & make(R(T::*pmf)(A) const)
Callback setup function for Callbacks with const member functions with explicit return type taking 1 ...
Union to store pointers to member functions in callback objects.
unsigned long execute(const void *user_param[]) const
Execute the callback with the required number of user parameters.
Namespace for the AIDA detector description toolkit.
Callback()
Default constructor.
void add(TYPE *pointer, R(OBJECT::*pmf)(A1, A2) const, Location where=CallbackSequence::END)
Add a new callback to a const member function with explicit return type and 2 arguments.
void add(TYPE *pointer, void(OBJECT::*pmf)(A1, A2), Location where=CallbackSequence::END)
Add a new callback to a void member function with 2 arguments.
static T * cast(void *p)
Template cast function used internally by the wrapper for type conversion to the object's type.
const Callback & make(void(T::*pmf)(A))
Callback setup function for Callbacks with void member functions taking 1 argument.
std::vector< Callback > Callbacks
const Callback & make(R(T::*pmf)(A0, A1) const)
Callback setup function for Callbacks with const member functions with explicit return type taking 2 ...
static const T * c_cast(const void *p)
Template const cast function used internally by the wrapper for type conversion to the object's type.
const Callback & make(R(T::*pmf)() const)
Callback setup function for Callbacks with const member functions with explicit return type taking no...
const Callback & make(void(T::*pmf)(A) const)
Callback setup function for Callbacks with const void member functions taking 1 argument.
const Callback & make(void(T::*pmf)() const)
Callback setup function for Callbacks with const void member functions taking no arguments.
unsigned long(* func_t)(void *obj, const void *fun, const void *args[])