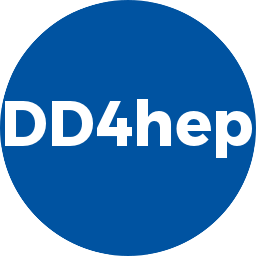 |
DD4hep
1.30.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
28 #include <G4RunManager.hh>
29 #include <G4ScoringManager.hh>
30 #include <G4UIdirectory.hh>
31 #include <G4Threading.hh>
32 #include <G4AutoLock.hh>
44 G4Mutex kernel_mutex = G4MUTEX_INITIALIZER;
45 std::unique_ptr<Geant4Kernel> s_main_instance;
46 void description_unexpected() {
52 <<
"**************************************************** \n"
53 <<
"* A runtime error has occured : \n"
54 <<
"* " << e.what() << std::endl
55 <<
"* the program will have to be terminated - sorry. \n"
56 <<
"**************************************************** \n"
71 : m_kernel(c.m_kernel) {
86 throw except(
"Geant4Kernel",
"Attempt to access the nonexisting phase '%s'", nam.c_str());
107 m_control->SetGuidance(
"Control for named Geant4 actions");
131 m_control->SetGuidance(
"Control for thread specific Geant4 actions");
138 if (
this == s_main_instance.get() ) {
139 s_main_instance.release();
164 if (
nullptr == s_main_instance.get() ) {
165 G4AutoLock protection_lock(&kernel_mutex); {
166 if (
nullptr == s_main_instance.get() ) {
168 std::set_terminate(description_unexpected);
173 return *(s_main_instance.get());
185 printout(INFO,
"Geant4Kernel",
186 "+++ Stop signal seen. Will finish after current event(s) have been processed.");
187 printout(INFO,
"Geant4Kernel",
188 "+++ Depending on the complexity of the simulation, this may take some time ...");
199 if ( sig_num == SIGINT ) {
212 unsigned long int thr_id = (
unsigned long int)::pthread_self();
222 printout(INFO,
"Geant4Kernel",
"+++ Created worker instance id=%ul",identifier);
225 except(
"Geant4Kernel",
"DDG4: Only the master instance may create workers.");
226 throw std::runtime_error(
"Geant4Kernel::createWorker");
232 return *((*i).second);
234 else if ( identifier ==
m_id ) {
239 if ( identifier ==
self ) {
243 else if( create_if ) {
246 except(
"Geant4Kernel",
"DDG4: The Kernel object 0x%p does not exists!",(
void*)identifier);
247 throw std::runtime_error(
"Geant4Kernel::worker");
271 printout(INFO,
"Geant4Kernel",
"+++ Define sensitive type: %s -> %s", type.c_str(), factory.c_str());
275 else if( iter->first == type && iter->second == factory ) {
278 except(
"Geant4Kernel",
279 "+++ The sensitive type %s is already defined and used %s. Cannot overwrite with %s",
280 type.c_str(), iter->second.c_str(), factory.c_str());
284 printout(ALWAYS,
"Geant4Kernel",
"OutputLevel: %d",
m_outputLevel);
285 printout(ALWAYS,
"Geant4Kernel",
"UI: %s",
m_uiName.c_str());
286 printout(ALWAYS,
"Geant4Kernel",
"NumEvents: %ld",
m_numEvent);
287 printout(ALWAYS,
"Geant4Kernel",
"NumThreads: %d",
m_numThreads);
289 printout(ALWAYS,
"Geant4Kernel",
"OutputLevel[%s]: %d", name.c_str(), level);
310 return (PrintLevel)(*i).second;
311 return dd4hep::PrintLevel(dd4hep::printLevel()-1);
318 return (PrintLevel)old;
330 std::string(
"Geant4RunManager"));
332 except(
"Geant4Kernel",
333 "+++ Invalid Geant4RunManager class: %s. Aborting.",
339 if (
nullptr == G4ScoringManager::GetScoringManager() ) {
340 except(
"Geant4Kernel",
"+++ FAILED to create the G4ScoringManager instance.");
347 except(
"Geant4Kernel",
348 "+++ Invalid Geant4RunManager action: %s. Invalid inheritance.",
351 except(
"Geant4Kernel",
352 "+++ Only the master thread may instantiate a G4RunManager object!");
353 throw std::runtime_error(
"Is never called -- just to satisfy compiler!");
358 char* arg = (
char*) compact_file.c_str();
365 const char* args[] = { fname, 0 };
391 except(
"Geant4Kernel",
"++ FAILED to configure DDG4 executive");
402 except(
"Geant4Kernel",
"++ FAILED to initialize DDG4 executive");
415 printout(FATAL,
"Geant4Kernel",
"+++ Exception while simulating:%s",e.what());
418 printout(FATAL,
"Geant4Kernel",
"+++ UNKNOWN exception while simulating.");
430 printout(INFO,
"Geant4Kernel",
"++ Terminate Geant4 and delete associated actions.");
433 for(
auto& call : calls) call();
459 const std::string& nam = action->
name();
463 printout(INFO,
"Geant4Kernel",
"++ Registered global action %s of type %s",
464 nam.c_str(),typeName(
typeid(*action)).c_str());
467 except(
"Geant4Kernel",
"DDG4: The action '%s' is already globally "
468 "registered. [Action-Already-Registered]", nam.c_str());
470 except(
"Geant4Kernel",
471 "DDG4: Attempt to globally register an invalid action. [Action-Invalid]");
479 if( throw_if_not_present ) {
480 except(
"Geant4Kernel",
"DDG4: The action '%s' is not globally "
481 "registered. [Action-Missing]", nam.c_str());
493 const std::string& nam = filter->
name();
499 except(
"Geant4Kernel",
"DDG4: The filter '%s' is already globally "
500 "registered. [Filter-Already-Registered]", nam.c_str());
502 except(
"Geant4Kernel",
503 "DDG4: Attempt to globally register an invalid filter. [Filter-Invalid]");
511 if (throw_if_not_present) {
512 except(
"Geant4Kernel",
"DDG4: The filter '%s' is not already globally "
513 "registered. [Filter-Missing]", filter_name.c_str());
521 (*i).second->execute(arguments);
531 except(
"Geant4Kernel",
"DDG4: The Geant4 action phase '%s' does not exist. [No-Entry]", nam.c_str());
537 return addPhase(name,
typeid(
void),
typeid(
void),
typeid(
void),throw_on_exist);
542 const std::type_info& arg0,
543 const std::type_info& arg1,
544 const std::type_info& arg2,
552 else if (throw_on_exist) {
553 except(
"Geant4Kernel",
"DDG4: The Geant4 action phase %s already exists. [Already-Exists]", nam.c_str());
void triggerStop()
Trigger smooth end-of-event-loop with finishing currently processing event.
virtual int configure()
Run the simulation: Configure Geant4.
G4UIdirectory * m_control
Top level control directory.
class dd4hep::sim::Geant4Kernel::PhaseSelector phase
Geant4Kernel * m_kernel
Reference to embedding object.
virtual void loadXML(const char *fname)
Load XML file.
void applyHandlers()
(Re-)apply registered interrupt handlers to override potentially later registrations by other librari...
void defineSensitiveDetectorType(const std::string &type, const std::string &factory)
Add new sensitive type to factory list.
void setWorld(G4VPhysicalVolume *volume)
Set the geometry world.
virtual Geant4ActionPhase * addSimplePhase(const std::string &name, bool throw_on_exist)
Add a new phase to the phase.
The property class to assign options to actions.
Interruptsback interface class with argument.
static int configure(Geant4Kernel &kernel)
Configure the application.
int m_outputLevel
Property: Output level.
Embedded helper class to facilitate map access to the phases.
virtual void destroyPhases()
Destroy all phases. To be called only at shutdown.
Geant4Context * m_context
Geant4 worker context (thread specific)
static int run(Geant4Kernel &kernel)
Run the application and simulate events.
Geant4Kernel & declareProperty(const std::string &nam, T &val)
Declare property.
void exception(const std::string &src, const std::string &msg)
std::string m_dfltSensitiveDetectorType
Property: Name of the default factory to create G4VSensitiveDetector instances.
Workers m_workers
Worker threads.
virtual Geant4ActionPhase * addPhase(const std::string &name, const std::type_info &arg1, const std::type_info &arg2, const std::type_info &arg3, bool throw_on_exist)
Add a new phase to the phase.
Geant4Kernel(Geant4Kernel *m, unsigned long identifier)
Standard constructor for workers.
Geant4Kernel * m_master
Parent reference.
Geant4Kernel & registerGlobalAction(Geant4Action *action)
Register action by name to be retrieved when setting up and connecting action objects.
int m_processEvents
Master property: Flag if event loop is enabled.
Property & property(const std::string &name)
Access single property.
Class, which allows all Geant4Action derivatives to access the DDG4 kernel structures.
virtual bool executePhase(const std::string &name, const void **args) const
Execute phase action if it exists.
Action phase definition. Client callback at various stage of the simulation processing.
Property & property(const std::string &name)
Access single property.
Geant4Interrupts * m_interrupts
Interrupt/signal handler: only on master instance.
static void increment(T *)
Increment count according to type information.
PrintLevel setOutputLevel(PrintLevel new_level)
Set the global output level of the kernel object; returns previous value.
virtual bool removePhase(const std::string &name)
Remove an existing phase from the phase. If not existing returns false.
bool processEvents() const
Check if event processing should be continued.
Geant4Context * workerContext()
Thread's Geant4 execution context.
PhaseSelector(Geant4Kernel *kernel)
Standard constructor.
void register_initialize(const std::function< void()> &callback)
Register initialize callback. Signature: (function)()
bool exists(const std::string &name) const
Check for existence.
Geant4Kernel & worker(unsigned long thread_identifier, bool create_if=false)
Access worker instance by its identifier.
ClientOutputLevels m_clientLevels
Property: Client output levels.
Geant4Kernel & registerGlobalFilter(Geant4Action *filter)
Register filter by name to be retrieved when setting up and connecting filter objects.
PrintLevel getOutputLevel(const std::string object) const
Retrieve the global output level of a named object.
void register_terminate(const std::function< void()> &callback)
Register terminate callback. Signature: (function)()
GlobalActions m_globalActions
Globally registered actions.
UserCallbacks m_actionInitialize
Registered action callbacks on initialize.
Geant4Interrupts & interruptHandler() const
Access interrupt handler. Will be created on the first call.
Geant4ActionPhase & operator[](const std::string &name) const
Phase access to the map.
G4RunManager * m_runManager
Reference to the run manager.
Geant4ActionPhase * getPhase(const std::string &name)
Access phase by name.
void setContext(Geant4Context *ctxt)
Set the thread's context.
std::string m_runManagerType
Property: Name of the G4 run manager factory to be used. Default: Geant4RunManager.
bool hasProperty(const std::string &name) const
Check property for existence.
UserCallbacks m_actionConfigure
Registered action callbacks on configure.
virtual long apply(const char *factory, int argc, char **argv) const =0
Manipulate geometry using factory converter.
static void decrement(T *)
Decrement count according to type information.
int m_haveScoringMgr
Master property: Instantiate the Geant4 scoring manager object.
static void destroyInstance(const std::string &name="default")
Destroy the singleton instance.
G4VPhysicalVolume * world() const
Access to geometry world.
static int terminate(Geant4Kernel &kernel)
Terminate the application.
Default base class for all Geant 4 actions and derivates thereof.
bool registerInterruptHandler(int sig_num)
Install DDG4 default handler for a given signal. If no handler: return false.
static unsigned long int thread_self()
Access thread identifier.
PropertyManager & properties()
Access to the properties of the object.
const std::string & name() const
Access name of the action.
Phases m_phases
Action phases.
Geant4Action * globalFilter(const std::string &filter_name, bool throw_if_not_present=true)
Retrieve filter from repository.
int m_numThreads
Master property: Number of execution threads in multi threaded mode.
void register_configure(const std::function< void()> &callback)
Register configure callback. Signature: (function)()
static int initialize(Geant4Kernel &kernel)
Initialize the application.
std::string m_uiName
Property: Name of the UI action. Must be member of the global actions.
GlobalActions m_globalFilters
Globally registered filters of sensitive detectors.
G4VPhysicalVolume * m_world
Access to geometry world.
bool isMultiThreaded() const
long m_numEvent
Property: Number of events to be executed in batch mode.
Geant4Action * globalAction(const std::string &action_name, bool throw_if_not_present=true)
Retrieve action from repository.
virtual int initialize()
Run the simulation: Initialize Geant4.
static Geant4Kernel & instance(Detector &description)
Instance accessor.
virtual int terminate()
Terminate all associated action instances.
virtual Geant4Kernel & createWorker()
Create identified worker instance.
long addRef()
Increase reference count.
virtual int run()
Run the simulation: Simulate the number of events given by the property "NumEvents".
virtual void loadGeometry(const std::string &compact_file)
Construct detector geometry using description plugin.
void set(const TYPE &value)
Set value of this property.
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
unsigned long m_id
Flag: Master instance (id<0) or worker (id >= 0)
std::string m_controlName
Property: Name of the G4UI command tree.
Class, which allows all Geant4Action to be stored.
virtual int runEvents(int num_events)
Run the simulation: Simulate the number of events "num_events" and modify the property "NumEvents".
The main interface to the dd4hep detector description package.
Detector * m_detDesc
Detector description object.
PropertyManager m_properties
Property pool.
virtual void enableUI()
Enable and install UI messenger.
G4RunManager & runManager()
Access to the Geant4 run manager.
virtual ~Geant4Kernel()
Default destructor.
bool registerHandler_SIGINT()
Specialized handler for SIGINT.
PhaseSelector & operator=(const PhaseSelector &c)
Assignment operator.
UserCallbacks m_actionTerminate
Registered action callbacks on terminate.
std::map< std::string, std::string > m_sensitiveDetectorTypes
Property: Names with specialized factories to create G4VSensitiveDetector instances.
void applyInterruptHandlers()
(Re-)apply registered interrupt handlers to override potentially later registrations by other librari...
void printProperties() const
Print the property values.
int numWorkers() const
Access number of workers.
Generic context to extend user, run and event information.
virtual int terminate() override
Run the simulation: Terminate Geant4.