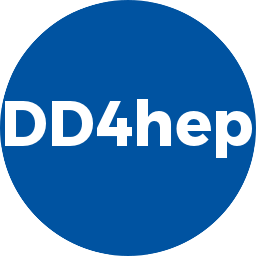 |
DD4hep
1.32.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
18 #include <G4PVPlacement.hh>
24 : m_detDesc(description_ref), m_dataPtr(0) {
44 except(
"Geant4Mapping",
"Attempt to access an invalid data block!");
76 except(
"Geant4Mapping",
"Cannot create volume manager without Geant4 geometry info [Invalid-Info]");
84 for(
const auto& entry : pm ) {
85 if ( entry.second == node )
PlacedVolume placement(const G4VPhysicalVolume *node) const
Accessor to resolve geometry placements.
Geant4GeometryInfo & init()
Create and attach new data block. Delete old data block if present.
Geant4GeometryInfo & data() const
Access to the data pointer.
Handle class holding a placed volume (also called physical volume)
virtual ~Geant4Mapping()
Standard destructor.
static Geant4Mapping & instance()
Possibility to define a singleton instance.
Geant4Mapping(const Detector &description)
Initializing Constructor.
static Detector & getInstance(const std::string &name="default")
—Factory method----—
Geometry mapping from dd4hep to Geant 4.
Geant4GeometryMaps::PlacementMap g4Placements
The Geant4VolumeManager to facilitate optimized lookups of cell IDs from touchables.
Geant4VolumeManager volumeManager() const
Access the volume manager.
const Detector & m_detDesc
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
Concreate class holding the relation information between geant4 objects and dd4hep objects.
void attach(Geant4GeometryInfo *data)
Set a new data block.
Geant4GeometryInfo * m_dataPtr
The main interface to the dd4hep detector description package.
void checkValidity() const
When resolving pointers, we must check for the validity of the data block.
Geant4GeometryInfo * detach()
Release data and pass over the ownership.
std::map< PlacedVolume, G4VPhysicalVolume * > PlacementMap