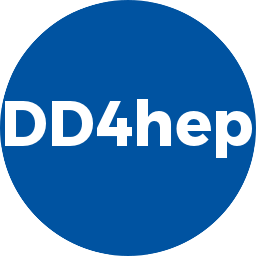 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
14 #ifndef DDG4_GEANT4ACTION_H
15 #define DDG4_GEANT4ACTION_H
29 class G4EventGenerator;
31 class G4TrackingManager;
38 #if defined(G__ROOT) || defined(__CLING__) || defined(__ROOTCLING__)
39 #define DDG4_DEFINE_ACTION_DEFAULT_CTOR(action) public: action() = default;
41 #define DDG4_DEFINE_ACTION_DEFAULT_CTOR(action) protected: action() = delete;
49 #define DDG4_DEFINE_ACTION_CONSTRUCTORS(action) \
50 DDG4_DEFINE_ACTION_DEFAULT_CTOR(action) \
52 action(action&& copy) = delete; \
53 action(const action& copy) = delete; \
54 action& operator=(action&& copy) = delete; \
55 action& operator=(const action& copy) = delete
65 class Geant4UIMessenger;
68 template <
typename TO,
typename FROM> TO
fast_cast(FROM from) {
70 return static_cast<TO
>(from);
72 return dynamic_cast<TO
>(from);
91 TypeName(
const std::pair<std::string, std::string>& c)
94 TypeName(
const std::string& typ,
const std::string& nam)
101 static TypeName split(
const std::string& type_name,
const std::string& delim);
170 typedef typename std::vector<T*>
_V;
175 void add(T* obj) {
m_v.emplace_back(obj); }
177 operator const _V&()
const {
return m_v; }
181 typename _V::iterator
begin() {
return m_v.begin(); }
182 typename _V::iterator
end() {
return m_v.end(); }
183 typename _V::const_iterator
begin()
const {
return m_v.begin(); }
184 typename _V::const_iterator
end()
const {
return m_v.end(); }
188 (*this)(&T::updateContext,ctxt);
191 template <
typename F>
typename _V::value_type
get(
const F& f)
const {
193 typename _V::const_iterator i=std::find_if(
m_v.begin(),
m_v.end(),f);
194 return i==
m_v.end() ? 0 : (*i);
199 template <
typename R,
typename Q>
void operator()(R (Q::*pmf)()) {
201 for (
const auto& o :
m_v)
204 template <
typename R,
typename Q,
typename A0>
void operator()(R (Q::*pmf)(A0), A0 a0) {
206 for (
const auto& o :
m_v)
209 template <
typename R,
typename Q,
typename A0,
typename A1>
void operator()(R (Q::*pmf)(A0, A1), A0 a0, A1 a1) {
211 for (
const auto& o :
m_v)
215 template <
typename R,
typename Q>
void operator()(R (Q::*pmf)()
const)
const {
217 for (
const auto& o :
m_v)
220 template <
typename R,
typename Q,
typename A0>
void operator()(R (Q::*pmf)(A0)
const, A0 a0)
const {
222 for (
const auto& o :
m_v)
225 template <
typename R,
typename Q,
typename A0,
typename A1>
void operator()(R (Q::*pmf)(A0, A1)
const, A0 a0, A1 a1)
const {
227 for (
const auto& o :
m_v)
231 template <
typename Q>
bool filter(
bool (Q::*pmf)()
const)
const {
233 for (
const auto& o :
m_v)
238 template <
typename Q,
typename A0>
bool filter(
bool (Q::*pmf)(A0)
const, A0 a0)
const {
240 for (
const auto& o :
m_v)
241 if ( !(o->*pmf)(a0) )
245 template <
typename Q,
typename A0,
typename A1>
bool filter(
bool (Q::*pmf)(A0, A1)
const, A0 a0, A1 a1)
const {
247 for (
const auto& o :
m_v)
248 if ( !(o->*pmf)(a0,a1) )
280 const std::string&
name()
const {
321 void print(
const char* fmt, ...)
const;
323 void printM1(
const char* fmt, ...)
const;
325 void printM2(
const char* fmt, ...)
const;
327 void printP1(
const char* fmt, ...)
const;
329 void printP2(
const char* fmt, ...)
const;
332 void always(
const char* fmt, ...)
const;
334 void debug(
const char* fmt, ...)
const;
336 void info(
const char* fmt, ...)
const;
338 void warning(
const char* fmt, ...)
const;
340 void error(
const char* fmt, ...)
const;
342 bool return_error(
bool return_value,
const char* fmt, ...)
const;
344 void fatal(
const char* fmt, ...)
const;
346 void except(
const char* fmt, ...)
const;
379 #endif // DDG4_GEANT4ACTION_H
_V::const_iterator end() const
virtual void updateContext(Geant4Context *ctxt)
Set or update client context.
void printP1(const char *fmt,...) const
Support for messages with variable output level using output level+1.
void printP2(const char *fmt,...) const
Support for messages with variable output level using output level+2.
void operator()(R(Q::*pmf)(A0, A1) const, A0 a0, A1 a1) const
bool m_needsControl
Default property: Flag to create control instance.
bool filter(bool(Q::*pmf)() const) const
CONST filters.
The property class to assign options to actions.
Geant4Action(Geant4Context *context, const std::string &nam)
Standard constructor.
Geant4UIMessenger * control() const
Access to the UI messenger.
Concrete implementation of the Geant4 stacking action sequence.
virtual void installPropertyMessenger()
Install property control messenger if wanted.
virtual void configureFiber(Geant4Context *thread_context)
Set or update client for the use in a new thread fiber.
PrintLevel setOutputLevel(PrintLevel new_level)
Set the output level; returns previous value.
void exception(const std::string &src, const std::string &msg)
Geant4GeneratorActionSequence & generatorAction() const
Access to the main generator action sequence from the kernel object.
bool return_error(bool return_value, const char *fmt,...) const
Action to support error messages.
Functor to access elements by name.
Geant4Context * m_context
Reference to the Geant4 context.
bool filter(bool(Q::*pmf)(A0) const, A0 a0) const
Property & property(const std::string &name)
Access single property.
void abortRun(const std::string &exception, const char *fmt,...) const
Abort Geant4 Run by throwing a G4Exception with type RunMustBeAborted.
std::string m_name
Action name.
bool filter(bool(Q::*pmf)(A0, A1) const, A0 a0, A1 a1) const
int m_outputLevel
Default property: Output level.
Functor to update the context of a Geant4Action object.
TypeName(const std::string &typ, const std::string &nam)
Initializing constructor.
void warning(const char *fmt,...) const
Support of warning messages.
PropertyManager m_properties
Property pool.
void fatal(const char *fmt,...) const
Support of fatal messages. Throws exception.
bool operator()(const Geant4Action *a)
Concrete implementation of the Geant4 tracking action sequence.
void info(const char *fmt,...) const
Support of info messages.
void except(const char *fmt,...) const
Support of exceptions: Print fatal message and throw runtime_error.
void updateContext(Geant4Context *ctxt)
Context updates.
Geant4EventActionSequence & eventAction() const
Access to the main event action sequence from the kernel object.
Concrete implementation of the Geant4 generator action sequence.
void error(const char *fmt,...) const
Support of error messages.
void operator()(R(Q::*pmf)())
NON-CONST actions.
long m_refCount
Reference count. Initial value: 1.
TO fast_cast(FROM from)
Cast operator.
~ContextSwap()
Destructor.
void operator()(R(Q::*pmf)(A0) const, A0 a0) const
Geant4Action & declareProperty(const std::string &nam, T &val)
Declare property.
Concrete basic implementation of the Geant4 run action sequencer.
void printM1(const char *fmt,...) const
Support for messages with variable output level using output level-1.
void operator()(R(Q::*pmf)(A0, A1), A0 a0, A1 a1)
Default base class for all Geant 4 actions and derivates thereof.
long release()
Decrease reference count. Implicit destruction.
DDG4_DEFINE_ACTION_CONSTRUCTORS(Geant4Action)
Define standard assignments and constructors.
const std::string & name() const
Access name of the action.
TypeName(const std::pair< std::string, std::string > &c)
Copy constructor from pair.
PrintLevel outputLevel() const
Access the output level.
static TypeName split(const std::string &type_name)
Split string pair according to default delimiter ('/')
void print(const char *fmt,...) const
Support for messages with variable output level using output level.
_V::const_iterator begin() const
PropertyManager & properties()
Access to the properties of the object.
void add(const std::string &name, const Property &property)
Add a new property.
Geant4RunActionSequence & runAction() const
Access to the main run action sequence from the kernel object.
const _V * operator->() const
TypeName & operator=(const TypeName ©)=default
Assignment operator.
void setName(const std::string &new_name)
Set the object name.
Geant4UIMessenger * m_control
Control directory of this action.
long addRef()
Increase reference count.
const char * c_name() const
Access name of the action.
Manager to ease the handling of groups of properties.
virtual void installCommandMessenger()
Install command control messenger if wanted.
Namespace for the AIDA detector description toolkit.
_V::value_type get(const F &f) const
Element access by name.
TypeName()=default
Default constructor.
void debug(const char *fmt,...) const
Support of debug messages.
Helper class to handle strings of the format "type/name".
void always(const char *fmt,...) const
Support of always printed messages.
Actor class to manipulate action groups.
TypeName(const TypeName ©)=default
Copy constructor.
Concrete implementation of the Geant4 event action sequence.
Geant4Context * context
reference to the context;
virtual void enableUI()
Enable and install UI messenger.
bool hasProperty(const std::string &name) const
Check property for existence.
void operator()(R(Q::*pmf)() const) const
CONST actions.
void operator()(R(Q::*pmf)(A0), A0 a0)
Concrete implementation of the Geant4 stepping action sequence.
virtual ~Geant4Action()
Default destructor.
Geant4StackingActionSequence & stackingAction() const
Access to the main stacking action sequence from the kernel object.
virtual void installMessengers()
Install property control messenger if wanted.
FindByName(const std::string &n)
Generic implementation to export properties and actions to the Geant4 command prompt.
void printM2(const char *fmt,...) const
Support for messages with variable output level using output level-2.
Geant4SteppingActionSequence & steppingAction() const
Access to the main stepping action sequence from the kernel object.
Generic context to extend user, run and event information.
Geant4Context * context() const
Access the context.
Geant4TrackingActionSequence & trackingAction() const
Access to the main tracking action sequence from the kernel object.
ContextSwap(Geant4Action *a, Geant4Context *c)
Constructor.