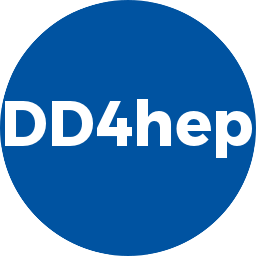 |
DD4hep
1.31.0
Detector Description Toolkit for High Energy Physics
|
Go to the documentation of this file.
23 #include <G4UIdirectory.hh>
31 size_t idx = type_name.find(delim);
32 std::string typ = type_name, nam = type_name;
33 if (idx != std::string::npos) {
34 typ = type_name.substr(0, idx);
35 nam = type_name.substr(idx + 1);
41 return split(type_name,
"/");
44 void Geant4Action::ContextUpdate::operator()(
Geant4Action* action)
const {
48 cout <<
"ERROR" << endl;
55 : m_context(ctxt), m_outputLevel(INFO), m_name(nam)
59 declareProperty(
"Name", m_name);
60 declareProperty(
"name", m_name);
61 declareProperty(
"OutputLevel", m_outputLevel);
62 declareProperty(
"Control", m_needsControl);
79 printM1(
"Geant4Action: Deleting object %s of type %s Pointer:%p",
80 m_name.c_str(),typeName(
typeid(*
this)).c_str(),(
void*)
this);
90 return (PrintLevel)old;
108 path +=
name() +
"/";
129 except(
"No control was installed for this action item.");
145 int level = std::max(
int(
outputLevel()),(
int)VERBOSE);
146 if ( level >= printLevel() ) {
149 dd4hep::printout((PrintLevel)level,
m_name.c_str(), fmt, args);
156 int level = std::max(
outputLevel()-1,(
int)VERBOSE);
157 if ( level >= printLevel() ) {
160 dd4hep::printout((PrintLevel)level,
m_name.c_str(), fmt, args);
167 int level = std::max(
outputLevel()-2,(
int)VERBOSE);
168 if ( level >= printLevel() ) {
171 dd4hep::printout((PrintLevel)level,
m_name.c_str(), fmt, args);
179 if ( level >= printLevel() ) {
182 dd4hep::printout((PrintLevel)level,
m_name.c_str(), fmt, args);
190 if ( level >= printLevel() ) {
193 dd4hep::printout((PrintLevel)level,
m_name.c_str(), fmt, args);
202 dd4hep::printout(dd4hep::ALWAYS,
m_name, fmt, args);
210 dd4hep::printout(dd4hep::DEBUG,
m_name, fmt, args);
218 dd4hep::printout(dd4hep::INFO,
m_name, fmt, args);
226 dd4hep::printout(dd4hep::WARNING,
m_name, fmt, args);
234 dd4hep::printout(dd4hep::ERROR,
m_name, fmt, args);
242 dd4hep::printout(dd4hep::ERROR,
m_name, fmt, args);
251 dd4hep::printout(dd4hep::FATAL,
m_name, fmt, args);
259 std::string err = dd4hep::format(
m_name, fmt, args);
260 dd4hep::printout(dd4hep::FATAL,
m_name, err.c_str());
262 throw std::runtime_error(err);
267 std::string desc, typ = typeName(
typeid(*
this));
268 std::string issuer =
name()+
" ["+typ+
"]";
271 desc = dd4hep::format(
"*** Geant4Action:", fmt, args);
273 G4Exception(issuer.c_str(),
exception.c_str(),RunMustBeAborted,desc.c_str());
const std::string & directoryName() const
Access the command directory.
void printP1(const char *fmt,...) const
Support for messages with variable output level using output level+1.
void printP2(const char *fmt,...) const
Support for messages with variable output level using output level+2.
bool m_needsControl
Default property: Flag to create control instance.
The property class to assign options to actions.
Geant4Action(Geant4Context *context, const std::string &nam)
Standard constructor.
void exportProperties(PropertyManager &mgr)
Export all properties to the Geant4 UI.
Geant4UIMessenger * control() const
Access to the UI messenger.
Concrete implementation of the Geant4 stacking action sequence.
virtual void installPropertyMessenger()
Install property control messenger if wanted.
Geant4RunActionSequence * runAction(bool create)
Access run action sequence.
virtual void configureFiber(Geant4Context *thread_context)
Set or update client for the use in a new thread fiber.
PrintLevel setOutputLevel(PrintLevel new_level)
Set the output level; returns previous value.
void exception(const std::string &src, const std::string &msg)
Geant4GeneratorActionSequence & generatorAction() const
Access to the main generator action sequence from the kernel object.
bool return_error(bool return_value, const char *fmt,...) const
Action to support error messages.
Geant4Context * m_context
Reference to the Geant4 context.
Geant4StackingActionSequence * stackingAction(bool create)
Access stacking action sequence.
Geant4TrackingActionSequence * trackingAction(bool create)
Access tracking action sequence.
Property & property(const std::string &name)
Access single property.
void abortRun(const std::string &exception, const char *fmt,...) const
Abort Geant4 Run by throwing a G4Exception with type RunMustBeAborted.
static void increment(T *)
Increment count according to type information.
std::string m_name
Action name.
int m_outputLevel
Default property: Output level.
void warning(const char *fmt,...) const
Support of warning messages.
PropertyManager m_properties
Property pool.
void fatal(const char *fmt,...) const
Support of fatal messages. Throws exception.
bool exists(const std::string &name) const
Check for existence.
Concrete implementation of the Geant4 tracking action sequence.
void info(const char *fmt,...) const
Support of info messages.
void except(const char *fmt,...) const
Support of exceptions: Print fatal message and throw runtime_error.
PrintLevel getOutputLevel(const std::string object) const
Retrieve the global output level of a named object.
Geant4EventActionSequence & eventAction() const
Access to the main event action sequence from the kernel object.
Concrete implementation of the Geant4 generator action sequence.
void error(const char *fmt,...) const
Support of error messages.
long m_refCount
Reference count. Initial value: 1.
Geant4EventActionSequence * eventAction(bool create)
Access run action sequence.
static void decrement(T *)
Decrement count according to type information.
Concrete basic implementation of the Geant4 run action sequencer.
void printM1(const char *fmt,...) const
Support for messages with variable output level using output level-1.
Default base class for all Geant 4 actions and derivates thereof.
long release()
Decrease reference count. Implicit destruction.
const std::string & name() const
Access name of the action.
PrintLevel outputLevel() const
Access the output level.
static TypeName split(const std::string &type_name)
Split string pair according to default delimiter ('/')
void print(const char *fmt,...) const
Support for messages with variable output level using output level.
PropertyManager & properties()
Access to the properties of the object.
Geant4RunActionSequence & runAction() const
Access to the main run action sequence from the kernel object.
Geant4UIMessenger * m_control
Control directory of this action.
long addRef()
Increase reference count.
Namespace for the Geant4 based simulation part of the AIDA detector description toolkit.
Geant4Kernel & kernel() const
Access to the kernel object.
Geant4SteppingActionSequence * steppingAction(bool create)
Access stepping action sequence.
virtual void installCommandMessenger()
Install command control messenger if wanted.
TypeName()=default
Default constructor.
void debug(const char *fmt,...) const
Support of debug messages.
Helper class to handle strings of the format "type/name".
void always(const char *fmt,...) const
Support of always printed messages.
Concrete implementation of the Geant4 event action sequence.
virtual void enableUI()
Enable and install UI messenger.
bool hasProperty(const std::string &name) const
Check property for existence.
Concrete implementation of the Geant4 stepping action sequence.
virtual ~Geant4Action()
Default destructor.
Geant4StackingActionSequence & stackingAction() const
Access to the main stacking action sequence from the kernel object.
virtual void installMessengers()
Install property control messenger if wanted.
Generic implementation to export properties and actions to the Geant4 command prompt.
void printM2(const char *fmt,...) const
Support for messages with variable output level using output level-2.
Geant4SteppingActionSequence & steppingAction() const
Access to the main stepping action sequence from the kernel object.
Generic context to extend user, run and event information.
Geant4Context * context() const
Access the context.
Geant4GeneratorActionSequence * generatorAction(bool create)
Access generator action sequence.
Geant4TrackingActionSequence & trackingAction() const
Access to the main tracking action sequence from the kernel object.